Understanding Callbacks, Promises, and Async/Await in JavaScript
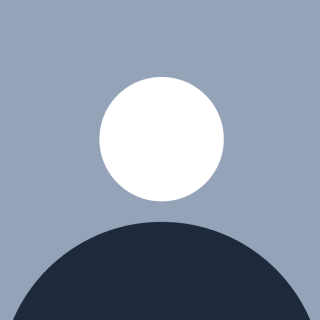
Table of contents
Callbacks
A callback is a function passed as an argument to another function, to be "called back" later. Callbacks are a fundamental concept in asynchronous programming in JavaScript, allowing you to execute code after a long-running operation, like an API call, completes.
function bakeCake(callback) {
console.log("Cake is baking...");
// Simulate cake baking time
callback();
}
function notify() {
console.log("Cake is ready!");
}
bakeCake(notify);
Here, notify
is a callback function passed to bakeCake
. When bakeCake
finishes its task, it calls notify
.
Promises
Promises provide a cleaner and more robust way to handle asynchronous operations compared to callbacks, especially for chaining multiple asynchronous calls.
function bakeCake() {
return new Promise((resolve, reject) => {
console.log("Cake is baking...");
let success = true; // Simulate successful cake baking
if (success) {
resolve("Cake is ready!");
} else {
reject("Cake burned!");
}
});
}
bakeCake()
.then((message) => {
console.log(message); // If promise is resolved
})
.catch((error) => {
console.error(error); // If promise is rejected
});
In this example, bakeCake
returns a promise. If the cake bakes successfully, the promise is resolved with a success message. If something goes wrong, the promise is rejected with an error message.
Async/Await
async
and await
are syntactic features in JavaScript that allow you to work with promises more comfortably. An async
function always returns a promise, and await
pauses the execution of the function, waiting for the promise to resolve or reject.
async function bakeCake() {
console.log("Cake is baking...");
let success = true; // Simulate successful cake baking
if (success) {
return "Cake is ready!";
} else {
throw new Error("Cake burned!");
}
}
async function makeCake() {
try {
let result = await bakeCake();
console.log(result); // If cake bakes successfully
} catch (error) {
console.error(error.message); // If cake baking fails
}
}
makeCake();
Here, bakeCake
is an asynchronous function that returns a promise. The makeCake
function uses await
to wait for the promise to resolve. If the cake bakes successfully, it logs the result. If it fails, it catches the error.
Subscribe to my newsletter
Read articles from Pawan S directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Pawan S
Pawan S
Understanding technology.