Exploratory Data Analysis (EDA): A Crucial Step Before Applying Machine Learning
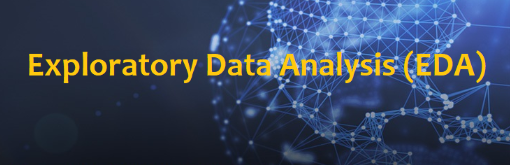
Exploratory Data Analysis (EDA) is a fundamental process in the data science pipeline, performed before applying machine learning algorithms. It involves analyzing and summarizing the main characteristics of a dataset to gain insights, detect anomalies, and understand the underlying patterns. In this blog post, we'll delve into the importance of EDA, the key steps involved, and provide Python code examples to illustrate the process.
Importance of Exploratory Data Analysis
EDA is crucial for several reasons:
Understanding the Data: EDA helps in understanding the structure, distribution, and quality of the data. This knowledge is essential for making informed decisions about data preprocessing and feature selection.
Identifying Patterns: By visualizing and summarizing the data, EDA allows us to identify patterns, trends, and relationships between variables.
Detecting Anomalies: EDA helps in identifying outliers, missing values, and errors in the dataset, which need to be addressed before applying machine learning models.
Guiding Feature Engineering: Insights gained from EDA guide the creation of new features, which can improve the performance of machine learning models.
Choosing the Right Models: EDA provides a basis for selecting appropriate machine learning algorithms based on the characteristics of the data.
Key Steps in Exploratory Data Analysis
Data Collection and Loading
The first step is to collect and load the data into your environment. This can involve reading data from CSV files, databases, or APIs.
Example:
pythonCopy codeimport pandas as pd # Load dataset df = pd.read_csv('your_dataset.csv')
Data Inspection
Inspect the dataset to understand its structure, data types, and basic statistics.
Example:
pythonCopy code# Display the first few rows of the dataset print(df.head()) # Display the summary statistics print(df.describe()) # Display data types and non-null counts print(df.info())
Handling Missing Values
Identify and handle missing values, which can significantly impact the performance of machine learning models.
Example:
pythonCopy code# Check for missing values print(df.isnull().sum()) # Fill missing values with mean (for numerical columns) df['column_name'].fillna(df['column_name'].mean(), inplace=True) # Drop rows with missing values df.dropna(inplace=True)
Data Visualization
Visualize the data to identify patterns, distributions, and relationships between variables. Common visualization techniques include histograms, box plots, scatter plots, and heatmaps.
Example:
pythonCopy codeimport matplotlib.pyplot as plt import seaborn as sns # Histogram plt.figure(figsize=(10, 6)) sns.histplot(df['numerical_column'], bins=30, kde=True) plt.title('Distribution of Numerical Column') plt.show() # Box plot plt.figure(figsize=(10, 6)) sns.boxplot(x='categorical_column', y='numerical_column', data=df) plt.title('Box Plot of Numerical Column by Categorical Column') plt.show() # Scatter plot plt.figure(figsize=(10, 6)) sns.scatterplot(x='feature1', y='feature2', data=df, hue='target') plt.title('Scatter Plot of Feature1 vs Feature2') plt.show() # Heatmap plt.figure(figsize=(12, 8)) sns.heatmap(df.corr(), annot=True, cmap='coolwarm') plt.title('Correlation Matrix') plt.show()
Feature Engineering
Create new features based on the insights gained from the data. This can involve combining existing features, creating interaction terms, or transforming features.
Example:
pythonCopy code# Create a new feature as the ratio of two existing features df['new_feature'] = df['feature1'] / df['feature2'] # Log transform a skewed feature df['log_feature'] = np.log1p(df['skewed_feature'])
Outlier Detection
Identify and handle outliers, which can distort the results of machine learning models.
Example:
pythonCopy code# Identify outliers using the IQR method Q1 = df['feature'].quantile(0.25) Q3 = df['feature'].quantile(0.75) IQR = Q3 - Q1 outliers = df[(df['feature'] < Q1 - 1.5 * IQR) | (df['feature'] > Q3 + 1.5 * IQR)] # Remove outliers df = df[~df.index.isin(outliers.index)]
Feature Scaling
Scale the features to ensure that they are on a similar scale, which is important for many machine learning algorithms.
Example:
pythonCopy codefrom sklearn.preprocessing import StandardScaler # Scale numerical features scaler = StandardScaler() df[['feature1', 'feature2']] = scaler.fit_transform(df[['feature1', 'feature2']])
Conclusion
Exploratory Data Analysis is an essential step in the data science workflow. It provides valuable insights into the data, guiding the preprocessing, feature engineering, and model selection processes. By thoroughly exploring and understanding the data, we can improve the accuracy and robustness of our machine learning models.
EDA is not just a one-time process but an iterative approach. As we progress with our analysis and modeling, we often revisit EDA to refine our understanding and make necessary adjustments. This iterative nature ensures that we build models that are well-suited to the data and capable of delivering accurate and reliable predictions.
By following the steps outlined in this blog post and utilizing the provided Python code examples, you can effectively perform EDA on your datasets and set a solid foundation for successful machine learning projects.
Subscribe to my newsletter
Read articles from Prakhar Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by