Understanding Array and Object Destructuring in JavaScript
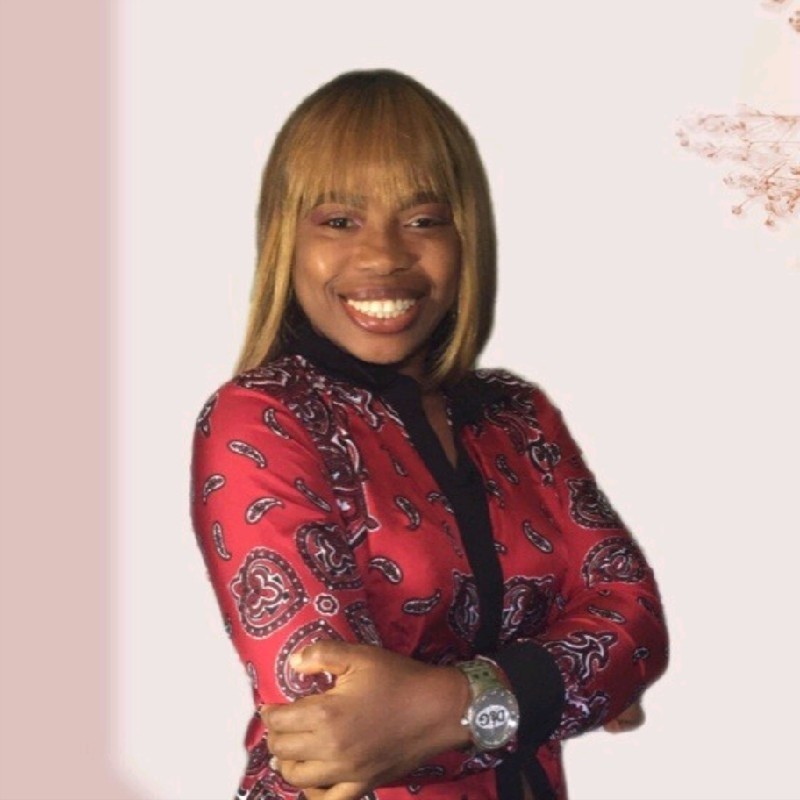
Table of contents
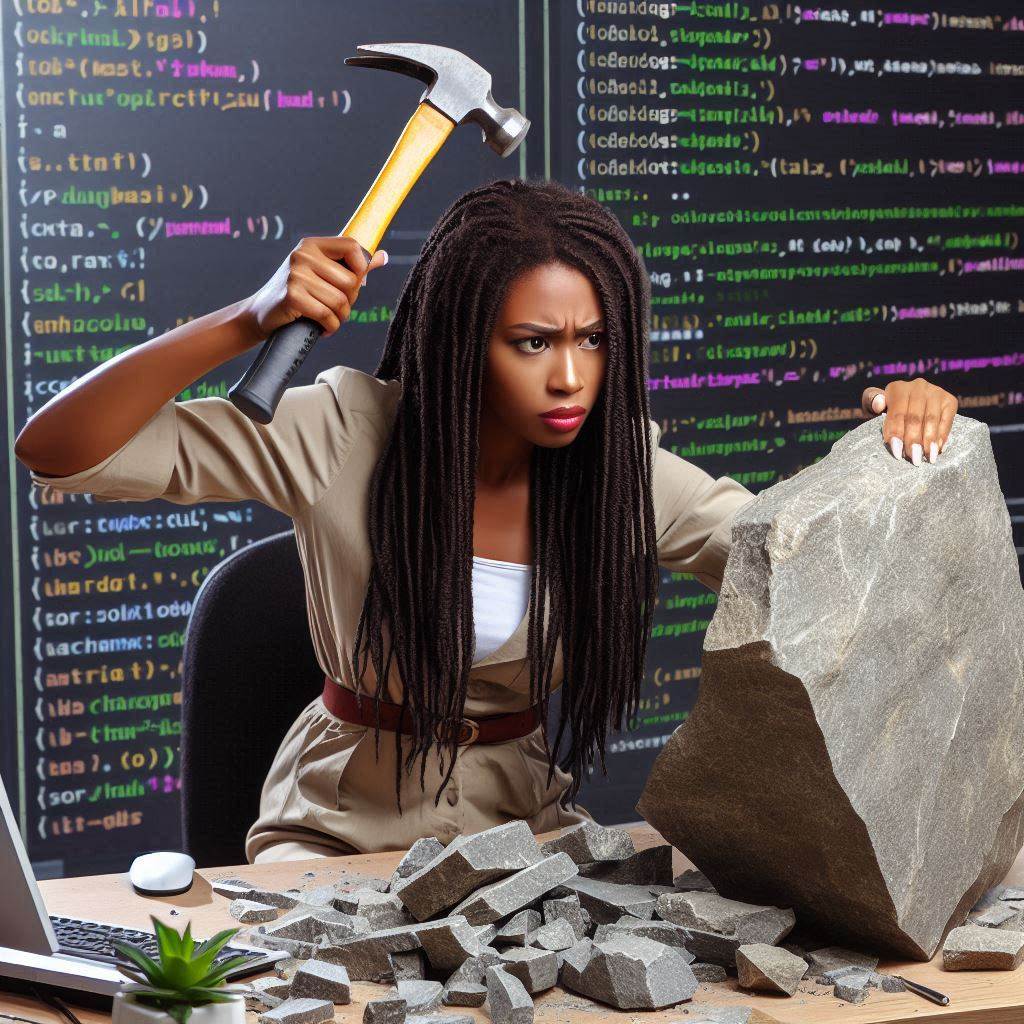
JavaScript is a versatile language, and destructuring is a powerful feature. Destructuring allows one to extract values from arrays or properties from objects and assign them to variables concisely and readably. Today, we'll explore the basics of array and object destructuring with examples to illustrate their use.
Array Destructuring
Array destructuring is simply extracting values from an array into distinct variables. It is versatile and can be applied in various ways, such as skipping elements, assigning default values, and swapping variables.
const numbers = [1, 2, 3];
const [one, two, three] = numbers;
console.log(one); // 1
console.log(two); // 2
console.log(three); // 3
Skipping elements
Items can be skipped in the array by simply leaving the corresponding position empty:
const [one, , three] = numbers;
console.log(one); // 1
console.log(three); // 3
Using Default Values
Default values can also be assigned to variables during restructuring:
const [x, y, z = 5] = [10, 20];
console.log(x); // 10
console.log(y); // 20
console.log(z); // 5
Swapping Variables
Destructuring makes swapping variables easier:
let x = 1, y = 2;
[x, y] = [y, x];
console.log(x); // 2
console.log(y); // 1
One might ask, "Why should I use destructuring instead of just accessing array elements directly?" Mastering array and object destructuring minimizes repetitive code and enhances maintainability by creating room for more efficient and concise JavaScript. For instance, consider the task of swapping variables; let's compare and see how this can be achieved without using restructuring.
let x = 1;
let y = 2;
// Swapping values without destructuring
let temp = x;
x = y;
y = temp;
console.log(x); // 2
console.log(y); // 1
// Swapping values by destructuring
let x = 1, y = 2;
[x, y] = [y, x];
console.log(x); // 2
console.log(y); // 1
We can see that destructuring is a powerful feature in JavaScript that can make your code cleaner and more readable. Whether you're working with simple arrays or complex nested objects (more on this later), destructuring is a tool every JavaScript developer should have in their toolkit.
Subscribe to my newsletter
Read articles from Onuora Chidimma Blessing directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
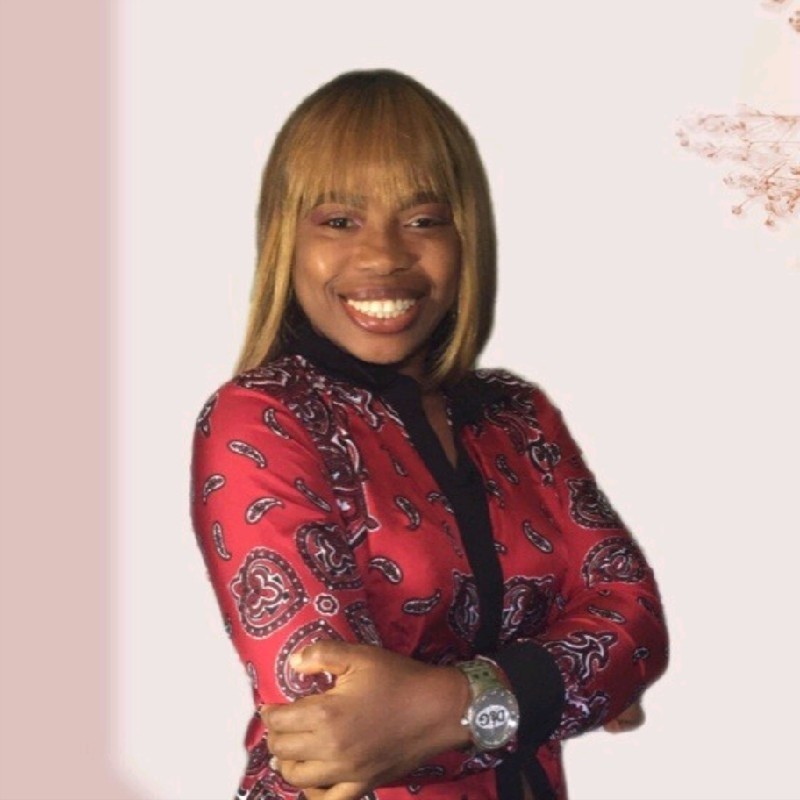
Onuora Chidimma Blessing
Onuora Chidimma Blessing
I'm Chidimma, a passionate software engineering and front-end web development enthusiast. I'm constantly learning and exploring these fields' latest technologies and tools. Through my blog on Hashnode, I'll be sharing my journey as I know and grow in my skills, and writing about concepts and topics I've come across. I hope my articles will help you learn something new or inspire you to take your skills to the next level!