Quick Introduction to Spring Boot Applications
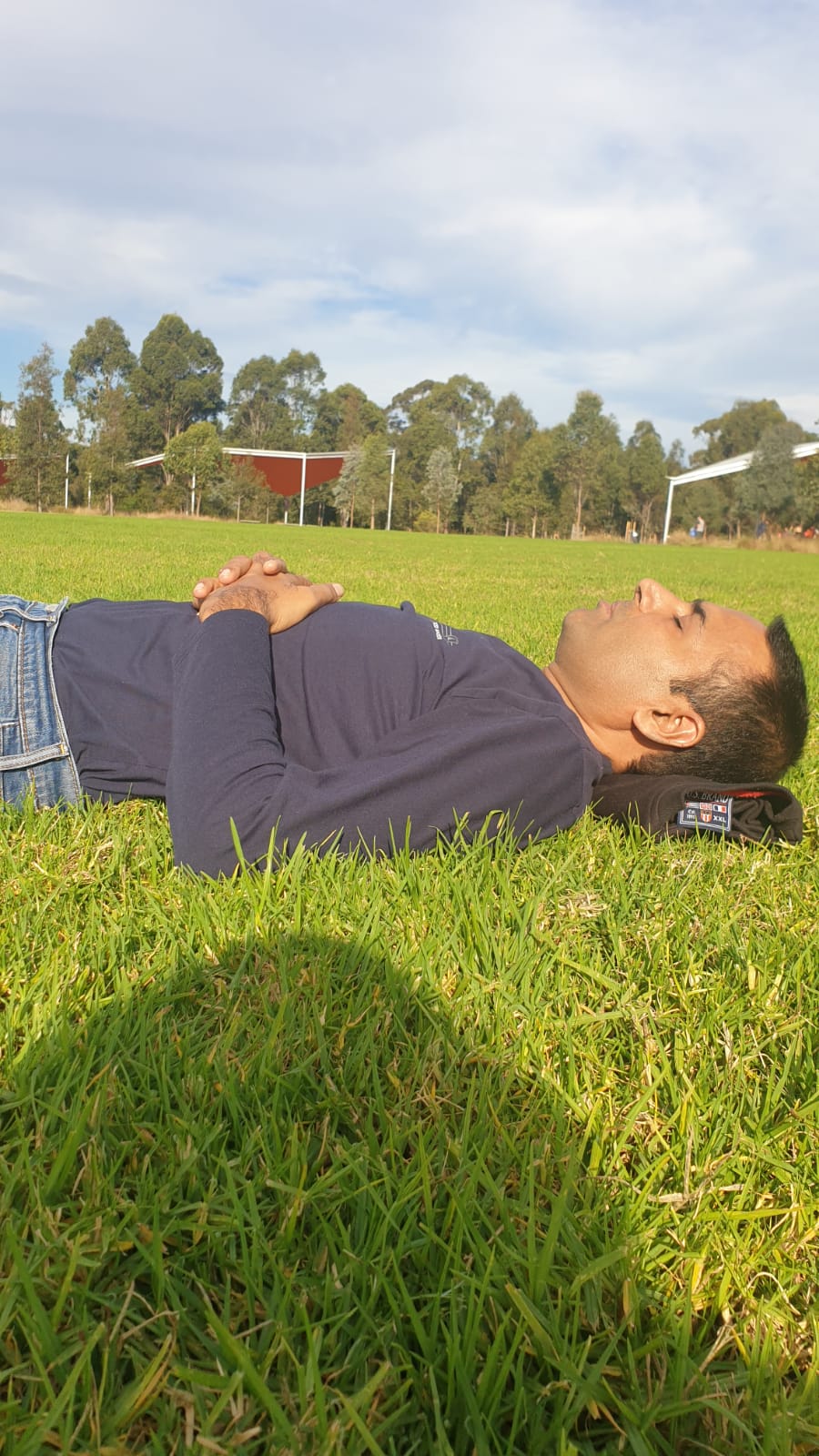
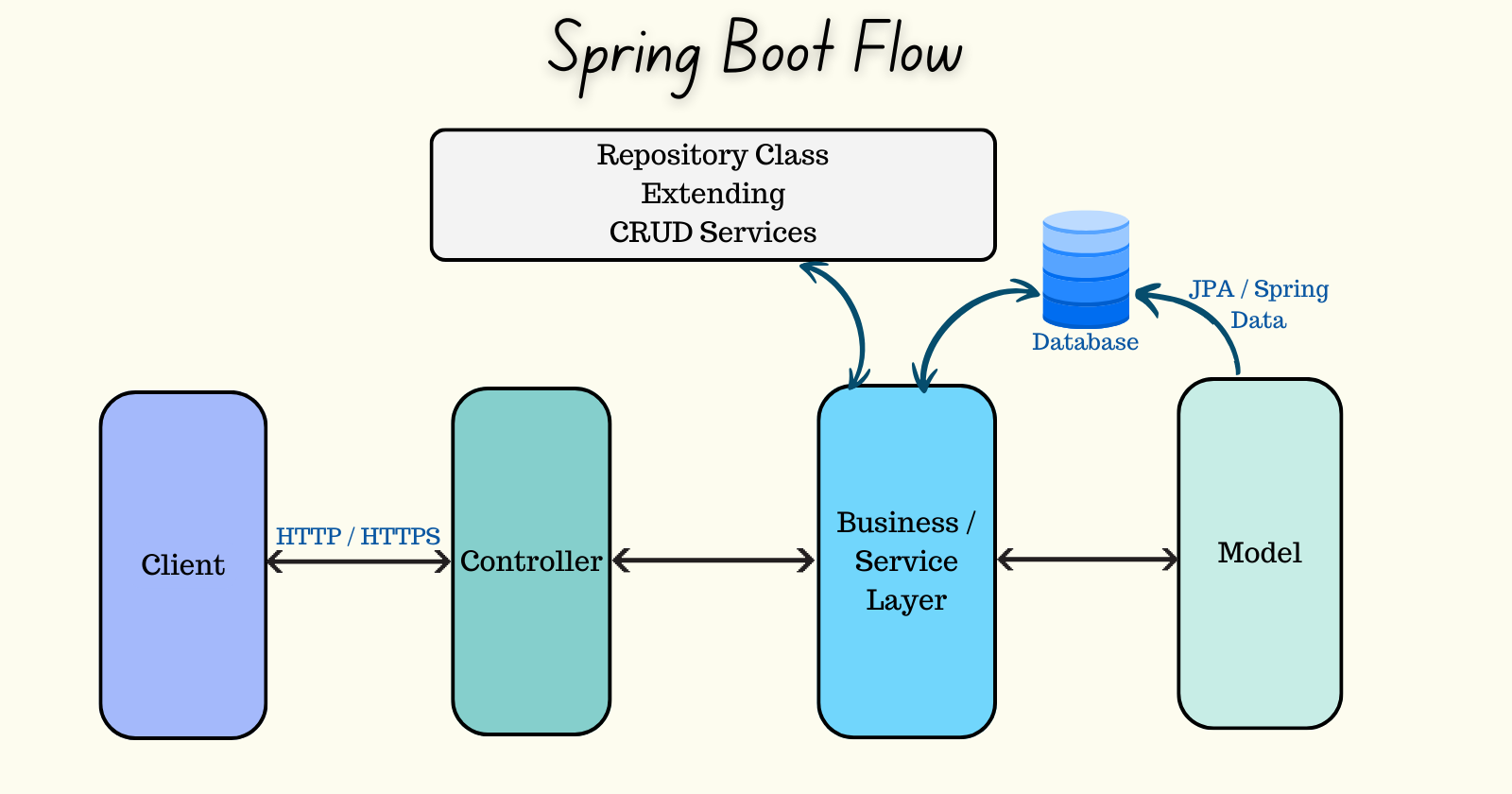
Spring Boot is a powerful framework that simplifies the setup and development of Spring applications. It provides an opinionated view of the Spring ecosystem and third-party libraries, enabling developers to create stand-alone, production-grade applications that can be run using java -jar
or traditional war deployments.
What is Spring Boot?
Spring Boot is a module of the Spring framework designed to simplify the bootstrapping and development of new Spring applications. It uses a convention-over-configuration approach to reduce developer effort and avoid complex XML configuration.
Key Features of Spring Boot
Auto-Configuration: Spring Boot automatically configures your application based on the dependencies you have added to the project. It uses the
@SpringBootApplication
annotation to trigger auto-configuration which automatically sets up your application based on the libraries present in the classpath. For example, if Spring MVC is on your classpath, Spring Boot automatically configures an embedded Tomcat server.Standalone Nature: Spring Boot allows you to create applications that can run independently without deploying to a web server. This is achieved by embedding Tomcat, Jetty, or Undertow directly. Application can be run using
java -jar
, eliminating the need to deploy WAR files.Production Ready: Spring Boot provides built-in tools for checking the health and performance of apps. Through the Actuator module, it provides out-of-the-box endpoints for monitoring and managing production applications.
No Code Generation: Spring Boot doesn’t generate code and there’s zero requirement for XML configuration which reduces the boilerplate code.
Opinionated Defaults: Spring Boot offers ‘opinionated’ starter POMs (Project Object Model), that simplify your build configuration.
Spring Initializr: This is a tool provided by Spring Boot to quickly bootstrap a new Spring Boot application. You can select the necessary dependencies, and it will generate a project structure for you.
Actuator: It provides production-ready features like health check-up, auditing, metrics gathering, HTTP tracing etc.
Spring Boot CLI: This is a command line tool that can be used if you want to quickly prototype with Spring.
Embedded Servers: Spring Boot allows you to embed servers such as Tomcat, Jetty, or Undertow directly, which avoids the complexity of deploying your application in an external web server.
Simplified Dependencies: Spring Boot provides opinionated ‘starter’ dependencies, which simplifies the build and application configuration. This means you only need to add one dependency to get a web application up and running.
Reduced Code: Spring Boot focuses on reducing the lines of code, which makes the application easier to manage and maintain.
Spring Boot Architecture
Spring Boot follows a layered architecture where each layer communicates with the layers directly above or below it. Here’s a brief description of the architecture and the flow of an HTTP request through a Spring Boot application:
Presentation Layer (Controller): This is the top layer of the architecture, handling all HTTP requests made by clients. It translates JSON parameters to Java objects, authenticates the request, and transfers it to the business layer.
Business Layer: Also known as the Service layer, this layer handles all the business logic of the application. It consists of service classes and uses services provided by the data access layers. It also performs authorisation and validation.
Persistence Layer: This layer contains all the storage logic required and translates business objects to database rows.
Database Layer: In this layer, all the CRUD (create, read, update, delete) operations are performed.
The flow of an HTTP request in a Spring Boot application is as follows:
The client makes an HTTP request (GET, POST, PUT, DELETE) to the browser.
The request goes to the controller where all the requests are mapped and handled.
After mapping is done, all the business logic is performed in the Service layer.
It performs the logic on the data that is mapped to the Java Persistence API (JPA) using model classes.
In the repository layer, all the CRUD operations are done for the REST APIs
A HTTP response is returned to the client if no errors are there.
Creating a Simple Spring Boot Application
Creating a Spring Boot application is straightforward with the Spring Initializr. Here’s a basic step-by-step guide:
Visit the Spring Initializr website.
Choose your project options and add your dependencies.
Click “Generate” to download a zip file containing your new project.
Unzip the file and import it into your favourite IDE.
Start developing!
Here’s a simple example of a Spring Boot application:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
In conclusion, Spring Boot is a game-changer for Java developers working with the Spring framework. It simplifies configuration, enhances productivity, and ultimately allows developers to focus more on the application’s business logic rather than setup and configuration.
Subscribe to my newsletter
Read articles from Sandeep Choudhary directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
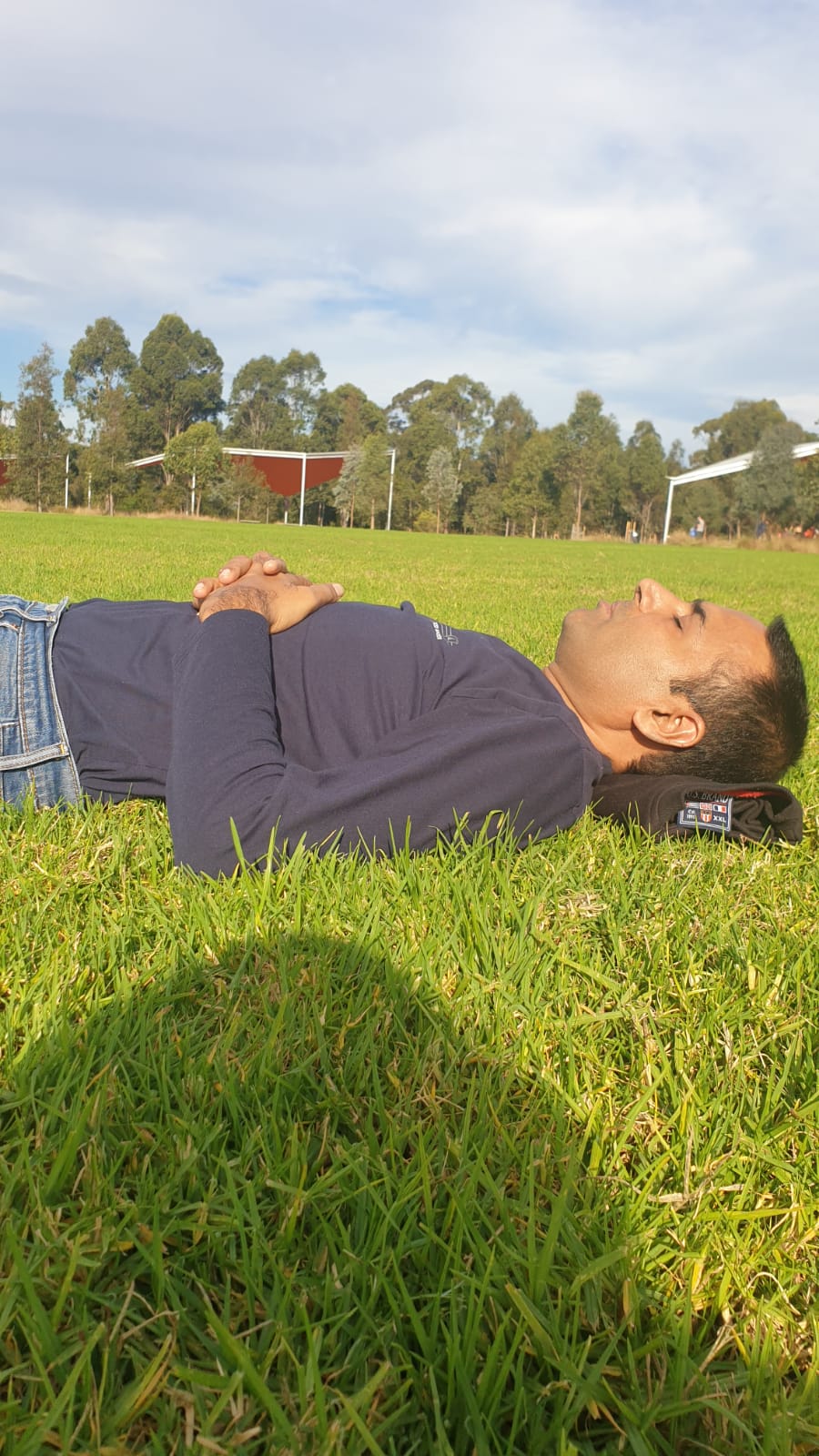