smart component and dumb component in angular
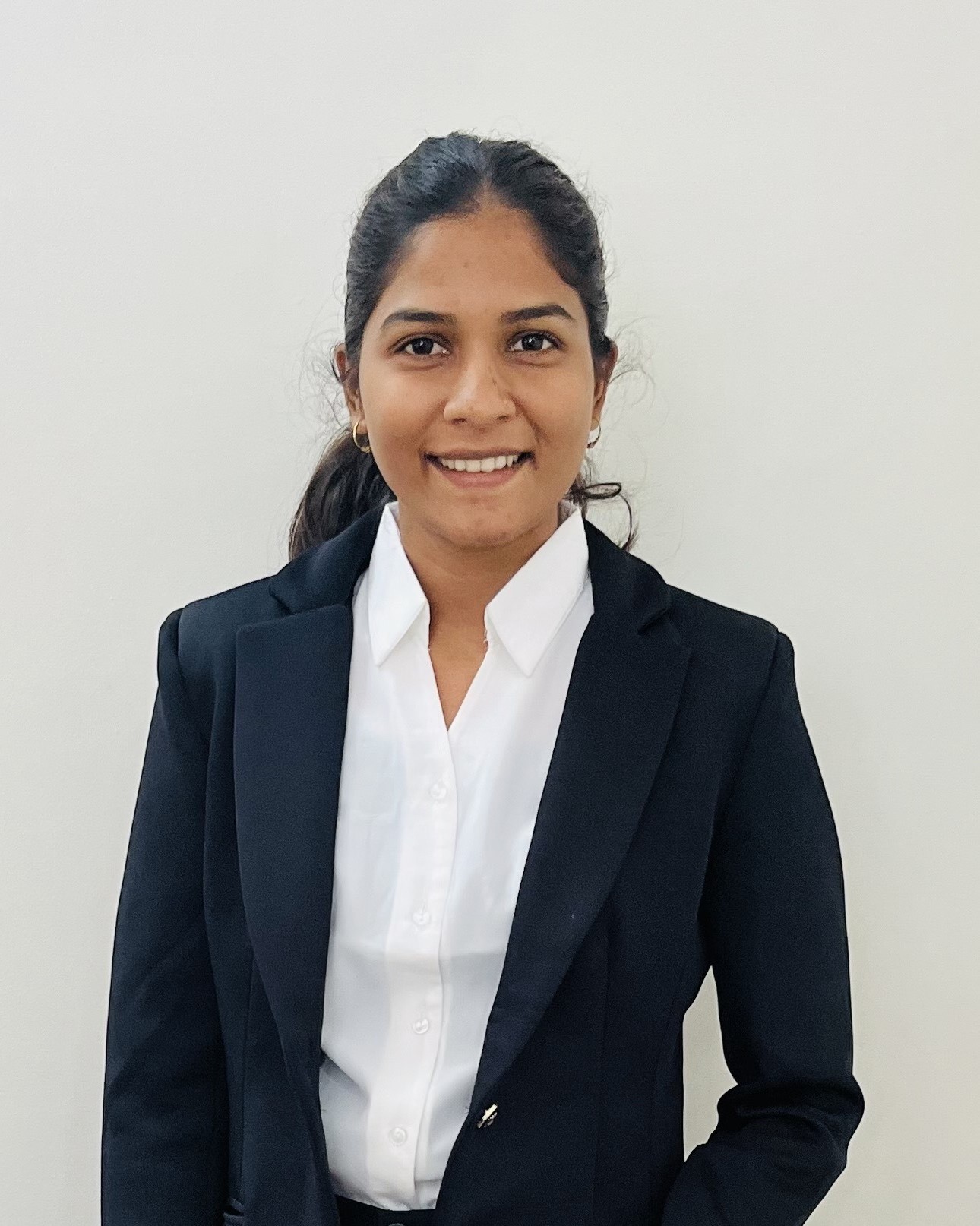
Smart Components:👍
Definition:
Smart components, also known as container components, are responsible for managing data and state. They handle the logic and interactions of the application, often dealing with services, APIs, and state management.
Characteristics:
State Management: They manage the state of the application and decide how data should be fetched, transformed, and stored.
Dependency Injection: They inject services to fetch or manipulate data.
Event Handling: They handle user interactions and decide what actions need to be taken, such as fetching data or navigating to a different route.
Minimal UI Logic: While they can include some UI logic, this should be kept minimal. The focus is on data and state.
Communication: They pass data down to dumb components and react to events emitted by dumb components.
Example:import { Component, OnInit } from '@angular/core'; import { UserService } from './user.service'; @Component({ selector: 'app-smart', template: ` <app-dumb [user]="user" (update)="onUpdate($event)"></app-dumb> ` }) export class SmartComponent implements OnInit { user: any; constructor(private userService: UserService) {} ngOnInit() { this.userService.getUser().subscribe(data => { this.user = data; }); } onUpdate(updatedUser: any) { this.userService.updateUser(updatedUser).subscribe(); } }
Dumb Components:👎
Definition:
Dumb components, also known as presentational components, are focused on displaying data and emitting events. They are unaware of the application's state and do not directly interact with services or APIs.
Characteristics:
Presentation Focused: They are primarily concerned with how the data is displayed.
Receive Inputs: They receive data via
@Input
properties from their parent components.Emit Outputs: They use
@Output
properties to emit events to the parent components.Stateless: They generally do not maintain their own state, other than local UI state.
Reusable: They are highly reusable because they do not depend on the application’s state or services.
Example:
import { Component, Input, Output, EventEmitter } from '@angular/core';
@Component({
selector: 'app-dumb',
template: `
<div>
<p>{{ user.name }}</p>
<button (click)="updateUser()">Update</button>
</div>
`
})
export class DumbComponent {
@Input() user: any;
@Output() update = new EventEmitter<any>();
updateUser() {
this.update.emit({ name: 'Updated User' });
}
}
Summary of Differences:🤷♀️
conclution👌:
In practice, most of your components will be dumb components, handling the display and basic interactions. A smaller number of smart components will manage the overall state and logic, creating a clear and manageable architecture for your Angular application.
Subscribe to my newsletter
Read articles from priyanka chaudhari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
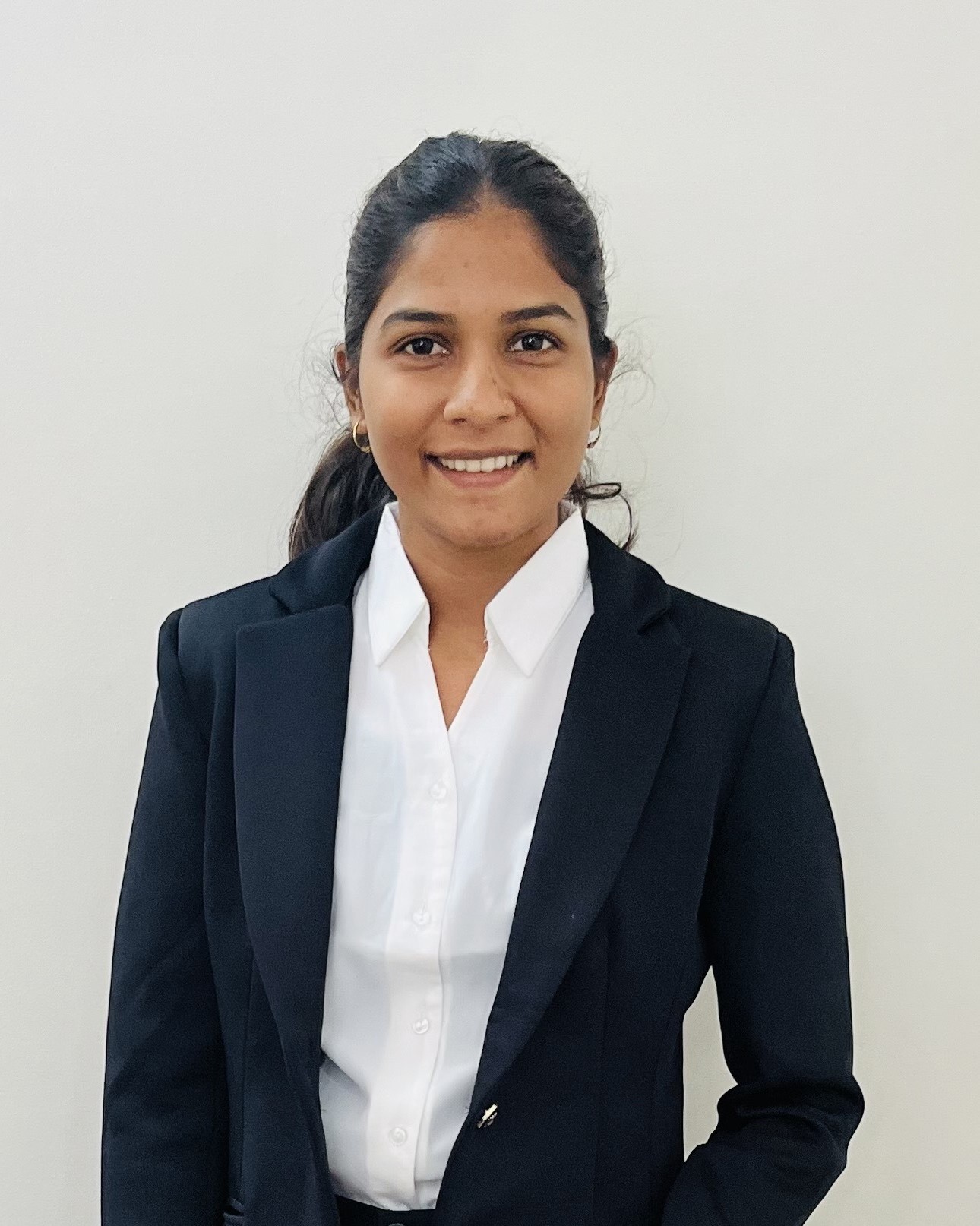
priyanka chaudhari
priyanka chaudhari
i am a full stack web developer and i write code....