Data Structures in Competitive Programming - Hiike
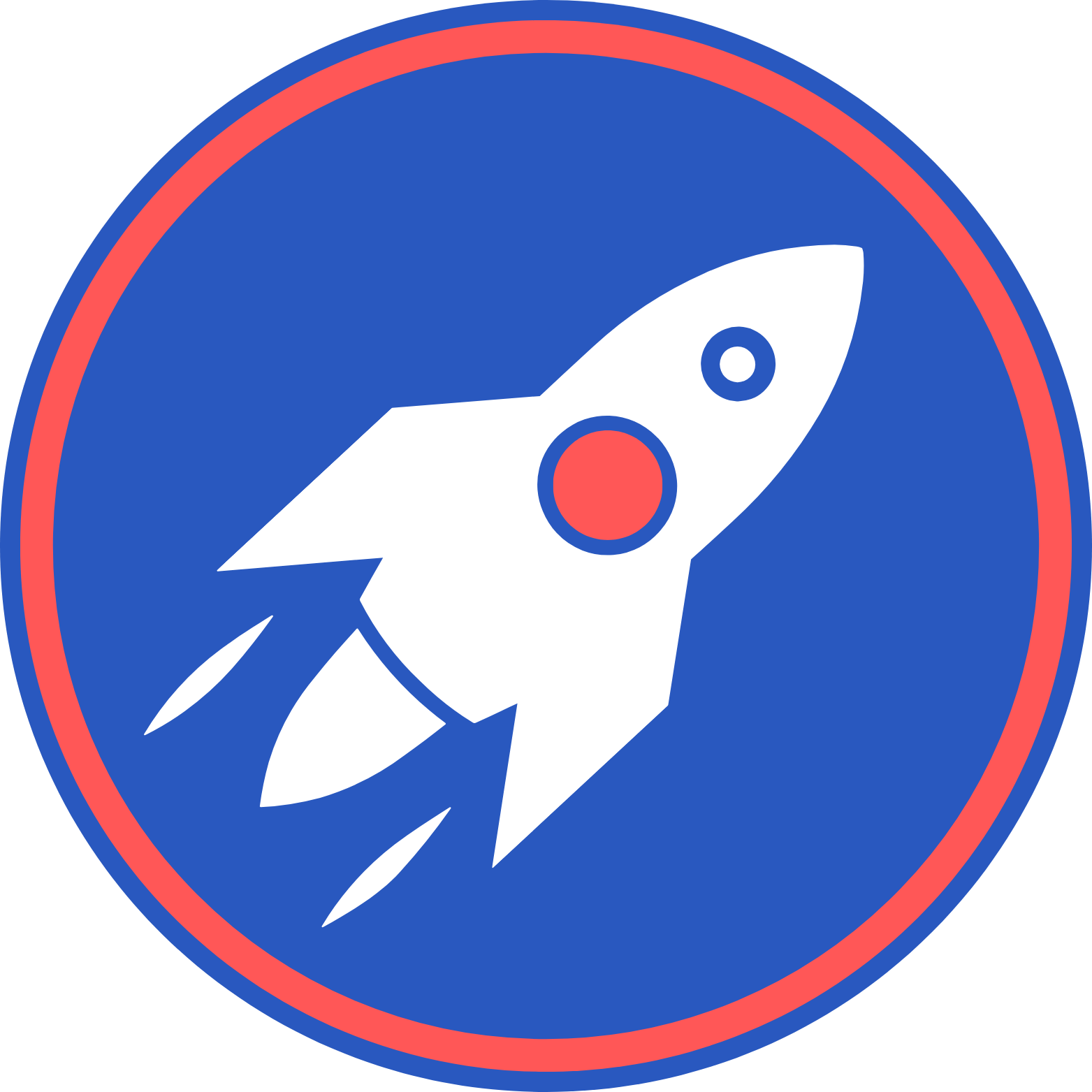
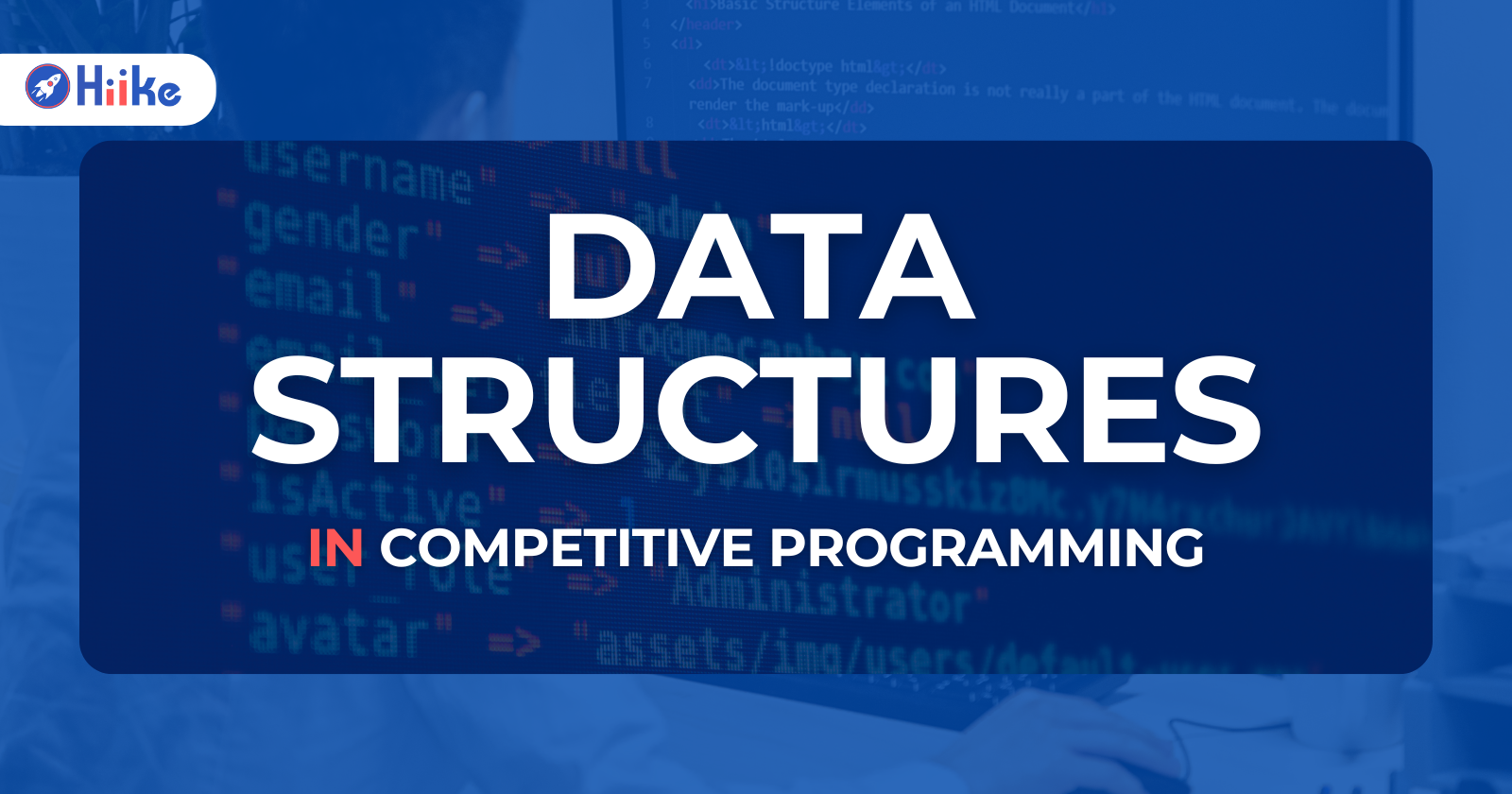
Competitive programming is like a mental marathon, pushing you to solve complex problems within a tight time frame. One of the key aspects of excelling in this field is mastering data structures, which help you organize and manage data efficiently. Let's explore some essential data structures used in competitive programming, understand their importance, and see how they can give you an edge in competitions.
Arrays and Lists
Arrays and lists are the building blocks of data structures. Arrays are fixed-size, contiguous blocks of memory where each element can be accessed in constant time O(1)O(1)O(1). They are perfect when you know the size of the data set in advance.
Lists, in contrast, are flexible and can expand or contract as required. In Python, lists are implemented as dynamic arrays, providing O(1)O(1)O(1) time complexity for indexing. However, appending elements is amortized O(1)O(1)O(1) and removing elements can be O(n)O(n)O(n).
Applications:
Arrays: Static data storage, quick lookups, and when the number of elements is known beforehand.
Lists: Dynamic data storage, where the size of the data can change.
Stacks and Queues
Stacks and queues are abstract data types implemented using arrays or linked lists. A stack operates on a Last In, First Out (LIFO) basis, whereas a queue adheres to the First In, First Out (FIFO) method.
Applications:
Stacks: Undo mechanisms in text editors, parsing expressions (like in calculators), and backtracking algorithms (like solving mazes).
Queues: Breadth-First Search (BFS) algorithms, task scheduling, and handling requests in web servers.
Trees
Trees are hierarchical structures with nodes, each containing a value and pointers to its children. The binary tree is the most prevalent type, characterized by each node having a maximum of two children. Special types include binary search trees (BSTs), heaps, AVL trees, and segment trees.
Binary Search Trees (BSTs): Maintain sorted elements, allowing efficient searching, insertion, and deletion, typically in O(logn)O(\log n)O(logn) time.
Heaps: Implement priority queues, supporting efficient extraction of the minimum or maximum element.
AVL Trees: Self-balancing BSTs that ensure the tree remains balanced after insertions and deletions, maintaining O(logn)O(\log n)O(logn) operations.
Segment Trees: Answer range queries efficiently, such as finding the sum or minimum of a subarray.
Applications:
BSTs: Database indexing, sorting algorithms, and dynamic data sets.
Heaps: Priority queues, Dijkstra's algorithm for shortest paths.
AVL Trees: Frequent insertions and deletions, ensuring the tree remains balanced.
Segment Trees: Range queries in computational geometry and large datasets.
Hash Tables
Hash tables store key-value pairs efficiently, offering average-case O(1)O(1)O(1) time complexity for insertions, deletions, and lookups. They employ a hash function to calculate an index within an array of buckets or slots.
Applications:
- Hash tables: Database indexing, caching, implementing sets, and scenarios requiring fast lookups and insertions.
Graphs
Graphs represent networks of interconnected nodes (vertices) and edges. They can be represented in two main ways: adjacency lists and adjacency matrices.
Adjacency List: Each vertex stores a list of adjacent vertices, space-efficient for sparse graphs.
Adjacency Matrix: A 2D array where each cell (i,j)(i, j)(i,j) indicates the presence or absence of an edge between vertices iii and jjj, space-efficient for dense graphs.
Applications:
- Graphs: Social network analysis, shortest path algorithms (like Dijkstra’s and Floyd-Warshall), network flow problems, and scheduling.
Tries
Tries, or prefix trees, are search trees used to store a dynamic set of strings, providing fast prefix-based searches.
Applications:
- Tries: Autocomplete systems, spell checkers, and IP routing.
Advanced Data Structures
Beyond the basics, competitive programmers often use advanced structures like Fenwick trees (Binary Indexed Trees) and Disjoint Set Union (DSU).
Fenwick Trees (BIT): Efficiently update elements and calculate prefix sums in a table of numbers.
Disjoint Set Union (DSU): Also known as Union-Find, tracks partitions of a set into disjoint subsets, supporting efficient union and find operations. Widely used in network connectivity and Kruskal's algorithm for finding the Minimum Spanning Tree (MST).
Conclusion
Mastering data structures is crucial for excelling in competitive programming. Each data structure has its strengths and is suited for different problems. By understanding and practicing these tools, you can solve complex problems more efficiently and improve your performance in competitions.
Author Bio
Pranjal Jain is the co-founder of Hiike, an innovative edtech startup dedicated to upskilling software engineers. A gold medalist from IIT Kanpur, Pranjal has a distinguished career with previous roles at Samsung and Microsoft. He personally teaches Data Structures, Algorithms, and System Design, demonstrating his deep passion and dedication to education. Pranjal’s commitment to teaching ensures that complex technical concepts are accessible and engaging for learners. He is also an active voice in the tech education community, sharing valuable insights and resources through Hiike’s YouTube channel and LinkedIn profile. Connect with him on LinkedIn — https://www.linkedin.com/in/techpranjal/ .
Subscribe to my newsletter
Read articles from Hiike directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
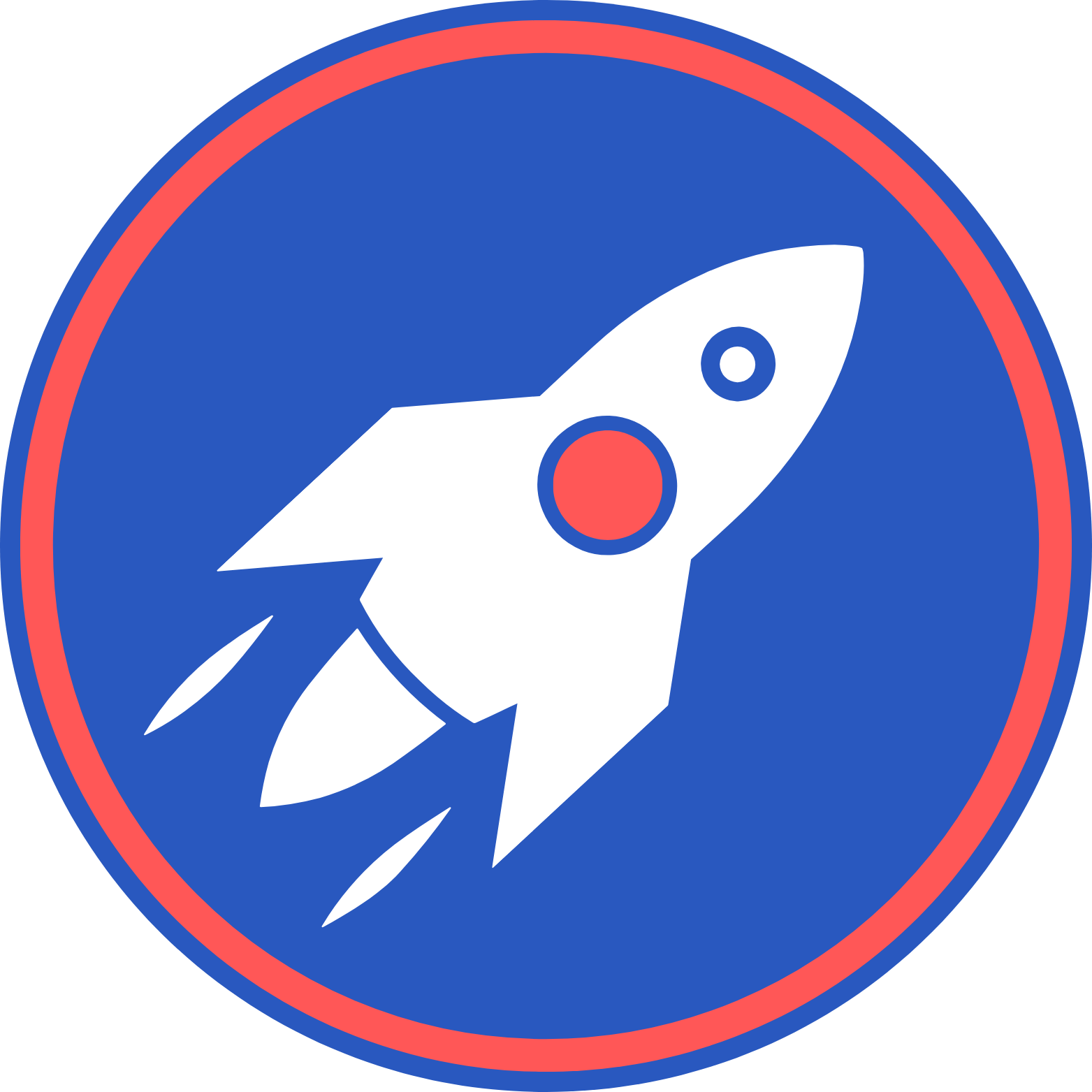
Hiike
Hiike
🌟𝐇𝐢𝐢𝐤𝐞 - Crafting Your Tech Odyssey! 🚀 Join Hiike for immersive learning, expert mentorship, and accelerated career growth. Our IITian-led courses and vibrant community ensure you excel in Data Structures, Algorithms, and System Design. Transform your tech career with Hiike today!