useContext() - React Hooks
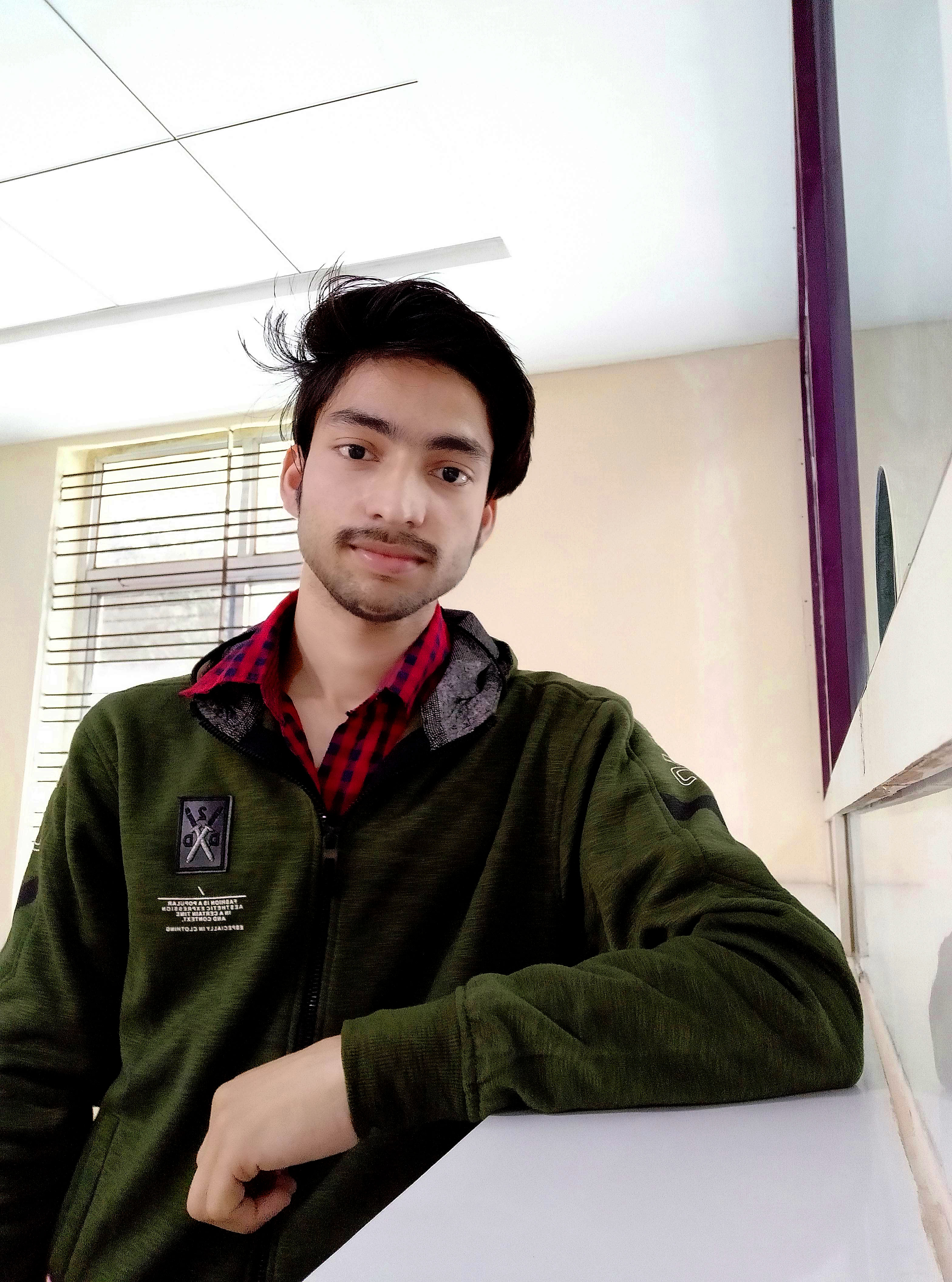
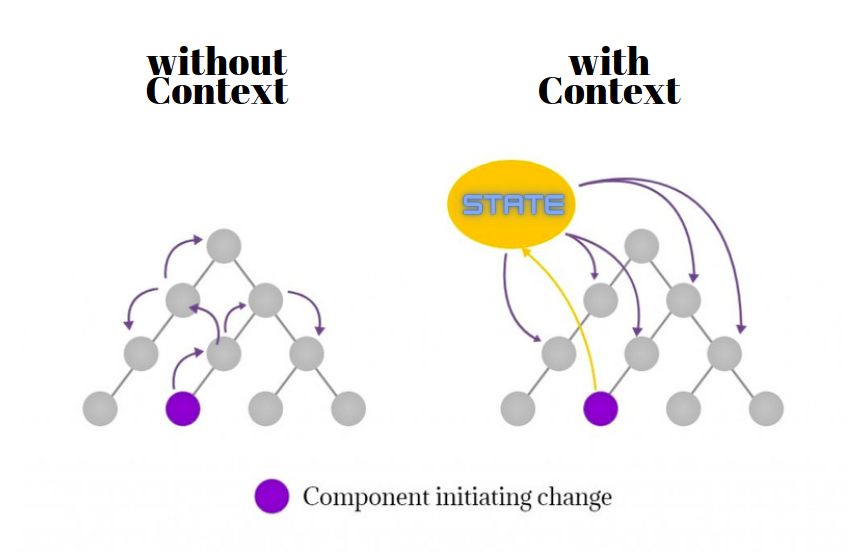
1. Set Up the Context
create a file called ThemeContext.js
and add the below code
// 📂 context/ThemeContext.js
import React, { useState, createContext } from "react";
// Create context object
// const ThemeContext = React.createContext();
const ThemeContext = createContext(null);
// const ThemeProvider = (props) => {
const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState("light");
const toggleTheme = () => setTheme(theme === "light" ? "dark" : "light");
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{/* 2️⃣ {props.children} */}
{children}
</ThemeContext.Provider>
);
};
export {ThemeContext};
export default ThemeProvider;
Okay, here we create a context object known as ThemeContext
, and then we're having a state that holds the current theme. And at last, we're wrapping the children around the Provider component from ThemeContext. Any component wrapped by this Provider will have access to the ThemeContext
object.
2.Wrap Your App with ThemeProvider
Now go to the App.js
file
And import the ThemeProvider component from the ThemeContext.js
file
import { ThemeProvider } from "./ThemeContext";
export default function App() {
return (
<ThemeProvider>
<App />
</ThemeProvider>
);
}
So now our setup is complete, we now access the context object, okay then let's build a component to work with the context object.
3. Use the Context in Components
create a file TogglePage.js
and add the following code
import React, { useContext } from "react";
// importing the context object that we created
import { ThemeContext } from "./ThemeContext";
const ToggleTheme = () => {
// Access the context object
const { theme, toggleTheme } = useContext(ThemeContext);
return (
<>
<button onClick={toggleTheme}>Toggle Theme</button>
<div className={theme === "light" ? "light" : "dark"}>
The current theme is <span>{theme}</span>
</div>
</>
);
};
export default ToggleTheme;
Subscribe to my newsletter
Read articles from Ayush Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
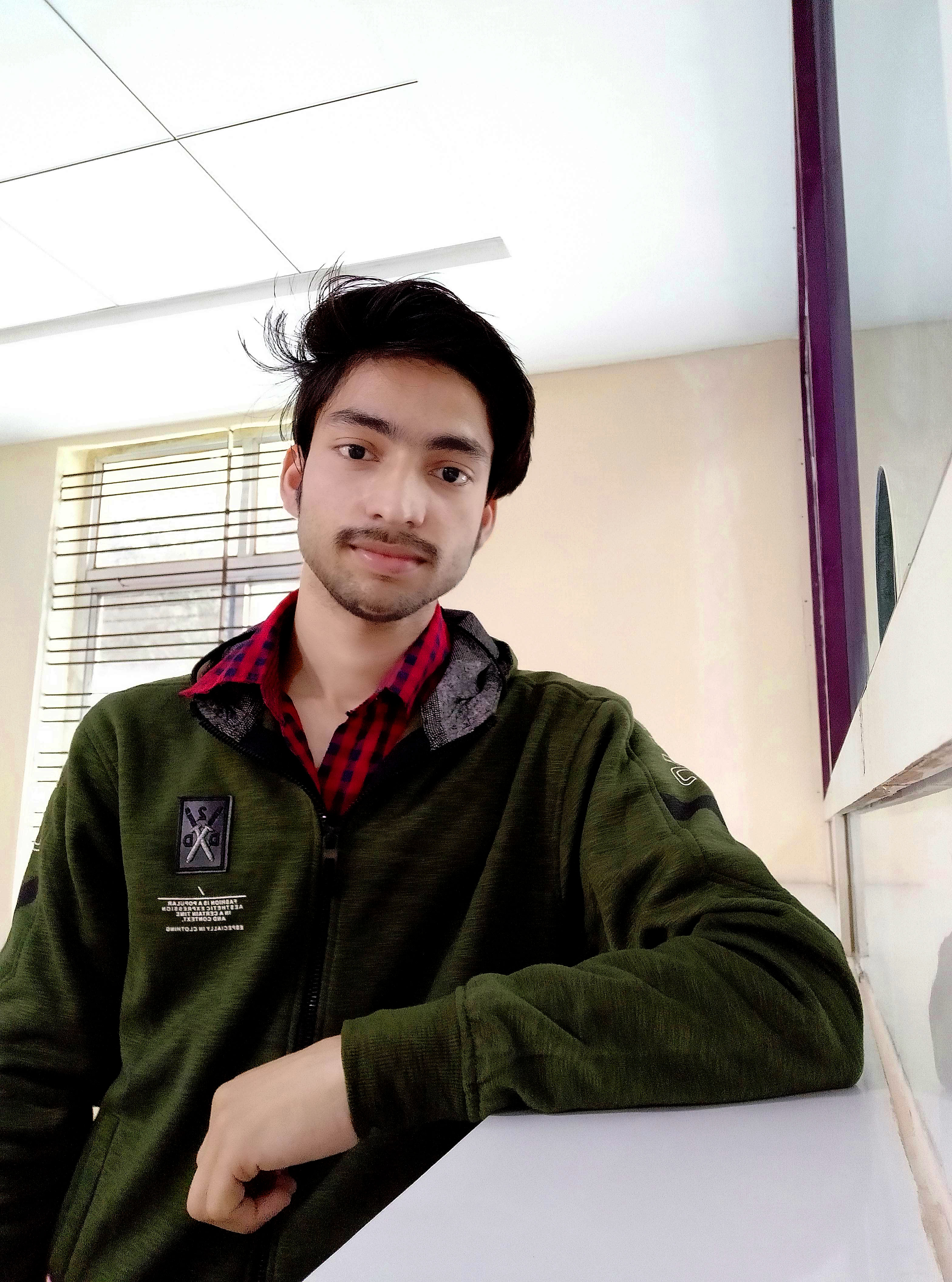
Ayush Kumar
Ayush Kumar
A passionate MERN Stack Developer from India I am a full stack web developer with experience in building responsive websites and applications using JavaScript frameworks like ReactJS, NodeJs, Express etc.,