Best Practices for Using OpenAI in Flutter
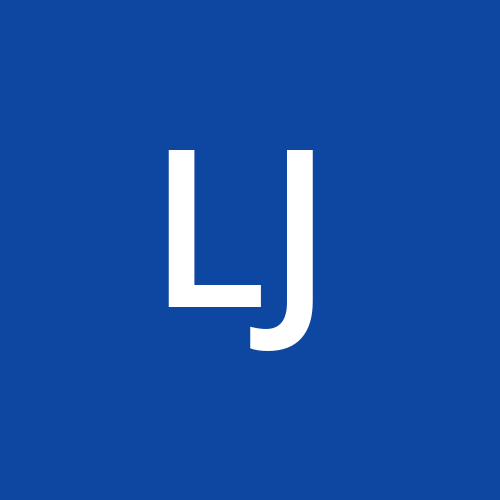
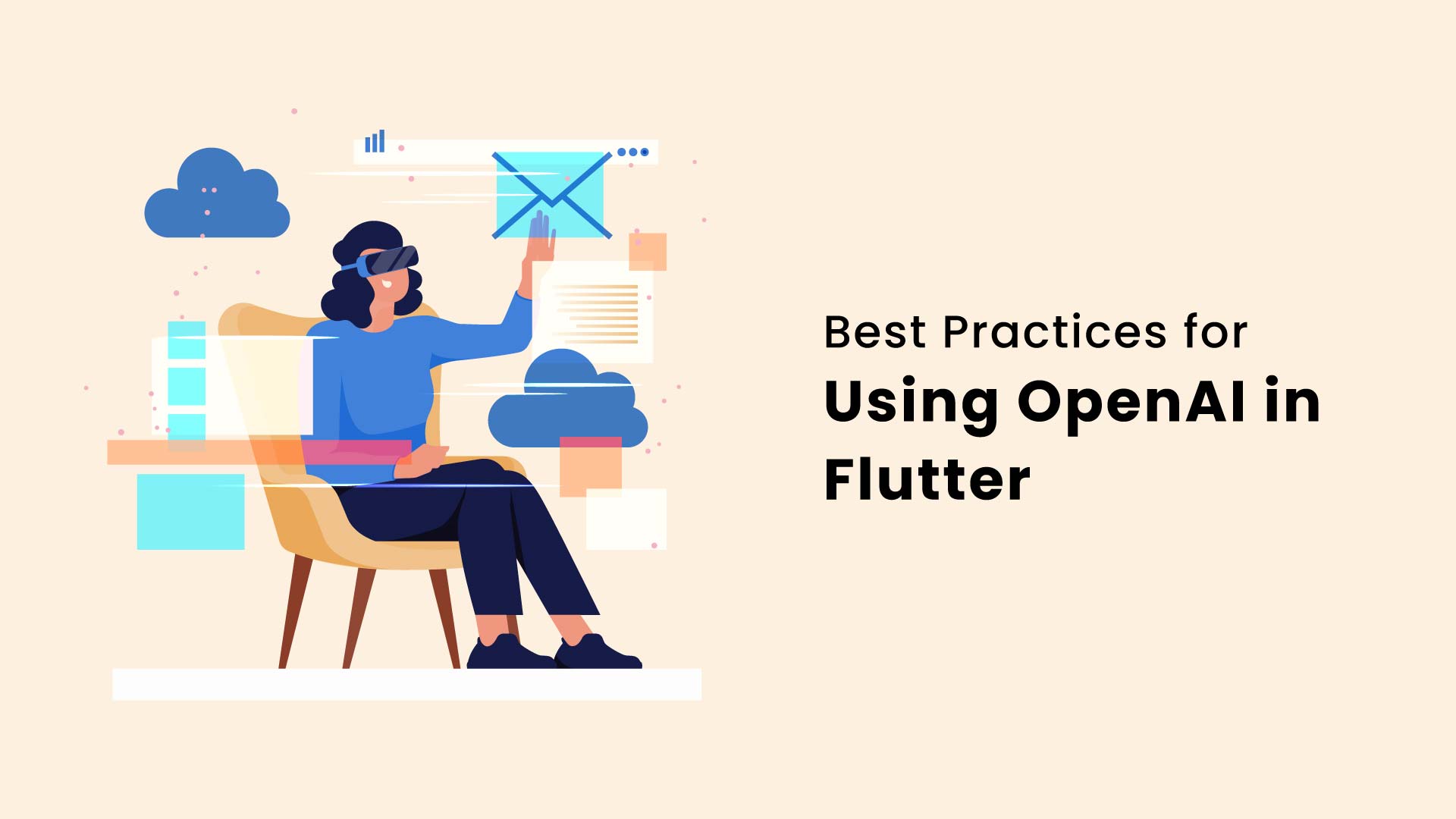
Integrating OpenAI into your Flutter app can unlock powerful features such as natural language understanding, text generation, and more. To ensure that your integration is smooth and your app remains performant and secure, it is crucial to follow best practices. This guide provides simple, beginner-friendly advice on how to effectively use OpenAI with Flutter.
OpenAI
OpenAI is a cutting-edge technology that can enhance your app with advanced features like natural language processing and generation. Whether you're building a chatbot, content generator, or a smart assistant, integrating OpenAI into your Flutter app can greatly improve its functionality. However, to make the most out of this integration, it's important to follow certain best practices. These practices will help you optimize performance, handle errors gracefully, and secure sensitive information.
1. Optimize API Requests
Making too many API requests can slow down your app and lead to higher costs. It's important to manage how frequently you interact with the OpenAI API to ensure a smooth user experience.
Batching Requests
If possible, group multiple requests into one. This reduces the number of times your app communicates with the API, which can enhance performance and reduce costs.
Caching Results
Store results from the API to avoid making the same request multiple times. This can save time and reduce the load on the API. Here’s how you can implement caching in your Flutter app:
import 'dart:convert';
import 'package:http/http.dart' as http;
class OpenAIService {
final String apiKey = 'YOUR_API_KEY';
final http.Client client = http.Client();
final Map<String, String> _cache = {};
Future<String> generateText(String prompt) async {
if (_cache.containsKey(prompt)) {
return _cache[prompt];
}
final response = await client.post(
Uri.parse('https://api.openai.com/v1/engines/davinci-codex/completions'),
headers: {
'Authorization': 'Bearer $apiKey',
'Content-Type': 'application/json',
},
body: jsonEncode({
'prompt': prompt,
'max_tokens': 150,
}),
);
if (response.statusCode == 200) {
final data = jsonDecode(response.body);
_cache[prompt] = data['choices'][0]['text'].trim();
return _cache[prompt];
} else {
throw Exception('Failed to generate text');
}
}
}
2. Handle Errors Gracefully
Errors can occur due to network issues, API problems, or other unexpected factors. Proper error handling ensures that your app remains functional and user-friendly even when things go wrong.
Show User-Friendly Messages
Display helpful messages to users when errors occur instead of letting the app crash. This improves the user experience by providing clarity and assistance. Here’s how you can handle errors gracefully:
Future<String> generateText(String prompt) async {
try {
final response = await client.post(
Uri.parse('https://api.openai.com/v1/engines/davinci-codex/completions'),
headers: {
'Authorization': 'Bearer $apiKey',
'Content-Type': 'application/json',
},
body: jsonEncode({
'prompt': prompt,
'max_tokens': 150,
}),
);
if (response.statusCode == 200) {
final data = jsonDecode(response.body);
return data['choices'][0]['text'].trim();
} else {
throw Exception('Failed to generate text');
}
} catch (error) {
return 'An error occurred: $error';
}
}
3. Secure Your API Key
Your OpenAI API key is sensitive information that should be protected. Exposing it can lead to unauthorized access and potential misuse.
Use Environment Variables
Store your API key in a secure location rather than hardcoding it into your source code. Using environment variables is a safe method. Here’s how to do it:
Add the flutter_dotenv
package to your project:
dependencies:
flutter:
sdk: flutter
flutter_dotenv: ^5.0.2
Create a .env file in your project’s root directory:
OPENAI_API_KEY=your_openai_api_key
Load and use the environment variable in your app:
import 'package:flutter_dotenv/flutter_dotenv.dart';
void main() async {
await dotenv.load(fileName: ".env");
runApp(MyApp());
}
class OpenAIService {
final String apiKey = dotenv.env['OPENAI_API_KEY'];
// rest of the code
}
4. Monitor Usage and Costs
Using the OpenAI API can incur costs based on usage. Keeping track of your API calls helps manage expenses and ensures you don’t exceed your budget.
Use the OpenAI Dashboard
Regularly check the OpenAI dashboard to monitor your usage and set up alerts for any unusual activity. This helps in keeping track of costs and making adjustments as needed.
5. Improve Performance
A smooth and responsive app provides a better user experience. Performance optimization ensures that your app runs efficiently and quickly.
Optimize UI and Reduce Computations
Make sure your app's user interface is optimized and avoid unnecessary computations on the main thread. Use Flutter’s performance tools to identify and fix any bottlenecks.
6. Provide User Feedback
Informing users about ongoing processes, such as API requests, improves their experience by keeping them updated on the app’s status.
Show Loading Indicators
Display loading indicators of progress bars while waiting for the API response. This helps users understand that the app is processing their request. Here’s an example:
import 'package:flutter/material.dart';
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
final OpenAIService openAIService = OpenAIService();
final TextEditingController _controller = TextEditingController();
String _generatedText = '';
bool _isLoading = false;
void _generateText() async {
setState(() {
_isLoading = true;
});
try {
final text = await openAIService.generateText(_controller.text);
setState(() {
_generatedText = text;
});
} catch (error) {
setState(() {
_generatedText = 'Error: $error';
});
} finally {
setState(() {
_isLoading = false;
});
}
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('OpenAI in Flutter')),
body: Padding(
padding: EdgeInsets.all(16.0),
child: Column(
children: [
TextField(
controller: _controller,
decoration: InputDecoration(labelText: 'Enter a prompt'),
),
SizedBox(height: 20),
ElevatedButton(
onPressed: _generateText,
child: Text('Generate Text'),
),
SizedBox(height: 20),
_isLoading ? CircularProgressIndicator() : Text(_generatedText),
],
),
),
),
);
}
}
Conclusion
Integrating OpenAI into your Flutter app opens up many exciting possibilities, but it requires careful planning and execution. By following these best practices—optimizing API requests, handling errors gracefully, securing your API key, monitoring usage, improving performance, and providing user feedback—you can ensure a seamless and efficient integration. This will not only enhance the functionality of your app but also provide a better experience for your users.
Happy coding!
Subscribe to my newsletter
Read articles from Liam Johnson directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
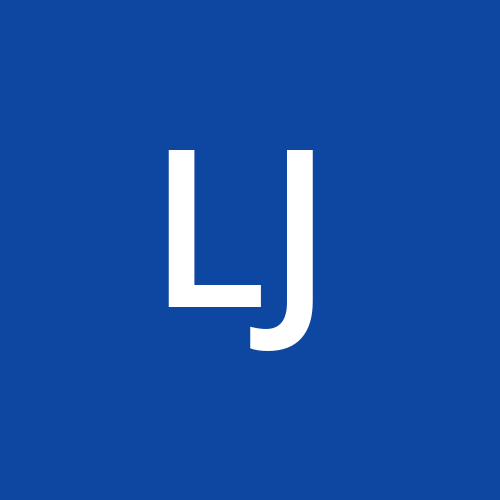
Liam Johnson
Liam Johnson
Hey there, I am Liam Johnson and I am a passionate Digital Marketer who is keen to know the hows of an ad and a website. Right now I am working at https://digimonksolutions.com/