Terraform Module 1: Create VPC and Launch EC2 Instance with remote Backend on S3 bucket.
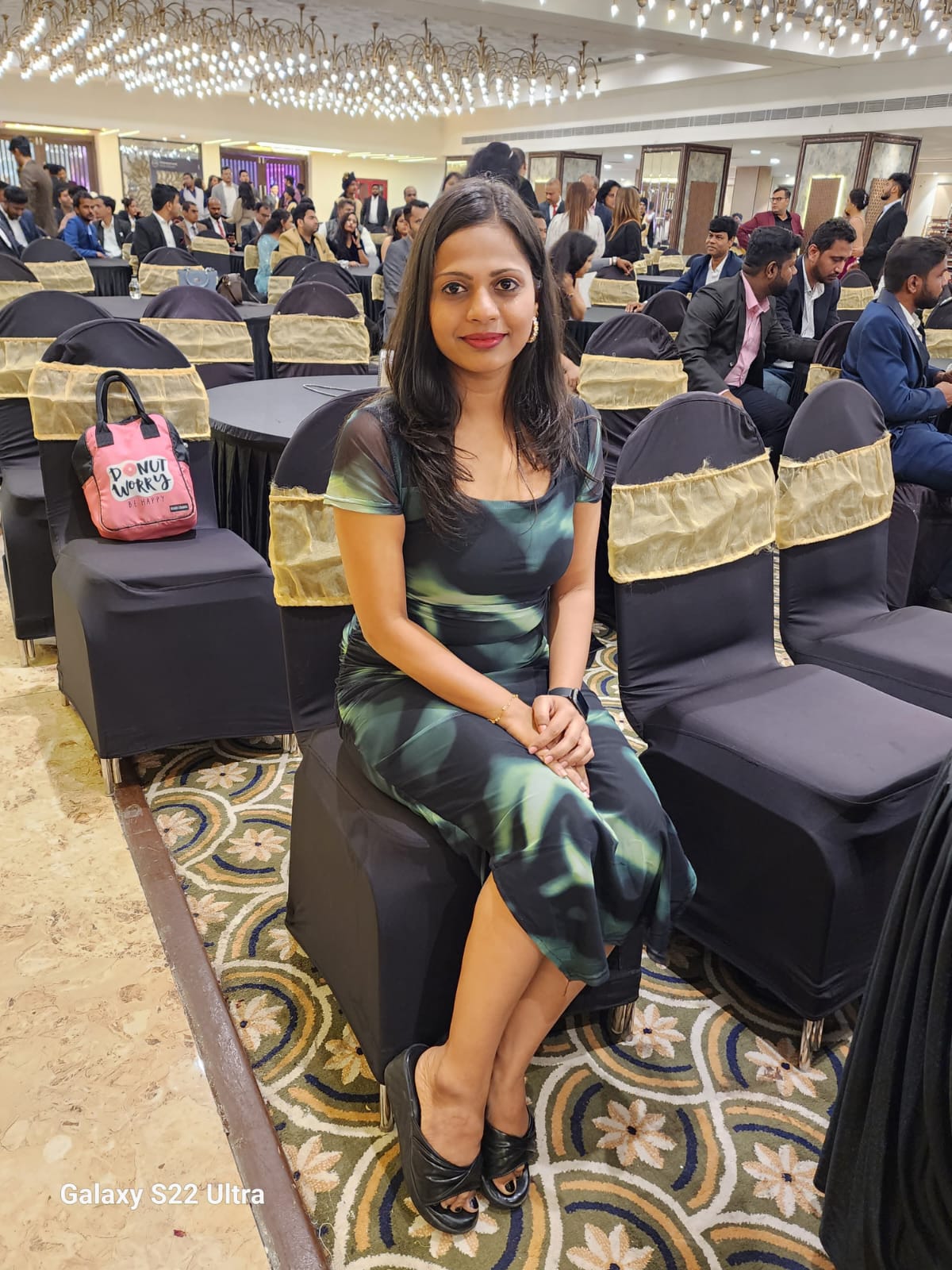
Introduction
This Terraform project is designed to provision and manage AWS infrastructure using Infrastructure as Code (IaC). The project includes modules for configuring a Virtual Private Cloud (VPC) and EC2 instances. The VPC module defines network resources such as subnets, route tables, and an internet gateway, while the EC2 module provisions virtual machines within this VPC. The project also uses a backend.tf file to configure remote state storage, ensuring safe and consistent management of the infrastructure state.
Purpose
The purpose of this Terraform project is to automate the setup of AWS infrastructure with a focus on network isolation and compute resources. By using Terraform modules, the project provides reusable and organized code for deploying a secure and scalable network environment, along with EC2 instances for application hosting.
Resources Used
AWS VPC: Creates a virtual network to host resources.
Subnets: Two subnets are created, with one being a public subnet attached to an internet gateway.
Internet Gateway: Allows communication between the VPC and the internet.
Route Table: Manages routing for network traffic.
EC2 Instances: Provision virtual machines within the VPC.
Terraform Configurations
Backend Configuration: Manages state files using a remote backend for better collaboration and consistency.
Configuration of Files
main.tf
The main.tf file is the central configuration file in a Terraform project, which typically includes the definition of the provider, the instantiation of modules, and the configuration of resources.
provider "aws" { region = "us-east-1" } module "vpc" { source = "./vpc" } module "ec2" { source = "./ec2" }
backend.tf
Configures the remote backend for storing the Terraform state file. This file sets up an S3 bucket and DynamoDB table for state management and locking, ensuring that the state file is safely stored and prevents concurrent modifications.
terraform { backend "s3" { bucket = "tf-remote-8611a32" key = "terraform.tfstate" region = "us-east-1" dynamodb_endpoint = "tf-my-lock-table" encrypt = true } }
VPC Module
- vpc.tf (within the VPC module):
Defines the core VPC resources including the VPC itself, subnets, route tables, and internet gateway. It sets up the network environment, configuring both public and private subnets, and establishes routing for internet access through the internet gateway.
locals {
cidr_block = "10.0.0.0/16"
}
resource "aws_vpc" "main" {
cidr_block = local.cidr_block
tags = {
Name = "tf-vpc-27-07"
}
}
data "aws_availability_zones" "az" {
}
resource "aws_subnet" "public-subnet" {
vpc_id = aws_vpc.main.id
cidr_block = "10.0.1.0/24"
availability_zone = data.aws_availability_zones.az.names[0]
tags = {
Name = "public-subnet"
}
}
resource "aws_subnet" "private-subnet" {
vpc_id = aws_vpc.main.id
cidr_block = "10.0.2.0/24"
availability_zone = data.aws_availability_zones.az.names[1]
tags = {
Name = "private-subnet"
}
}
output "vpc_id" {
value = aws_vpc.main.cidr_block
}
- ig.tf (within the VPC module):
Specifically manages the internet gateway configuration. It attaches the internet gateway to the VPC, enabling outbound internet access for resources in the public subnet.
resource "aws_internet_gateway" "gw" {
vpc_id = aws_vpc.main.id
tags = {
Name = "tf-ig"
}
}
resource "aws_route_table" "example" {
vpc_id = aws_vpc.main.id
route {
cidr_block = "0.0.0.0/0"
gateway_id = aws_internet_gateway.gw.id
}
tags = {
Name = "tf-public-rt"
}
}
resource "aws_route_table_association" "public-ig" {
subnet_id = aws_subnet.public-subnet.id
route_table_id = aws_route_table.example.id
}
EC2 Module
ec2.tf (within the EC2 module):
Configures EC2 instances within the specified VPC. This file defines the instance type, AMI, and network configuration, provisioning virtual machines based on the defined specifications.
resource "aws_instance" "web" { ami = "ami-0427090fd1714168b" instance_type = "t2.micro" tags = { Name = "tf-instance-26-07" } } output "instance_ami" { value = aws_instance.web.ami }
Conclusion
This Terraform project efficiently sets up a well-structured AWS infrastructure using modular code. By employing VPC and EC2 modules, the project ensures a scalable and maintainable setup for network and compute resources. The use of a remote backend for state management enhances collaboration and state consistency, making the project suitable for both development and production environments.
Subscribe to my newsletter
Read articles from Shraddha Suryawanshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
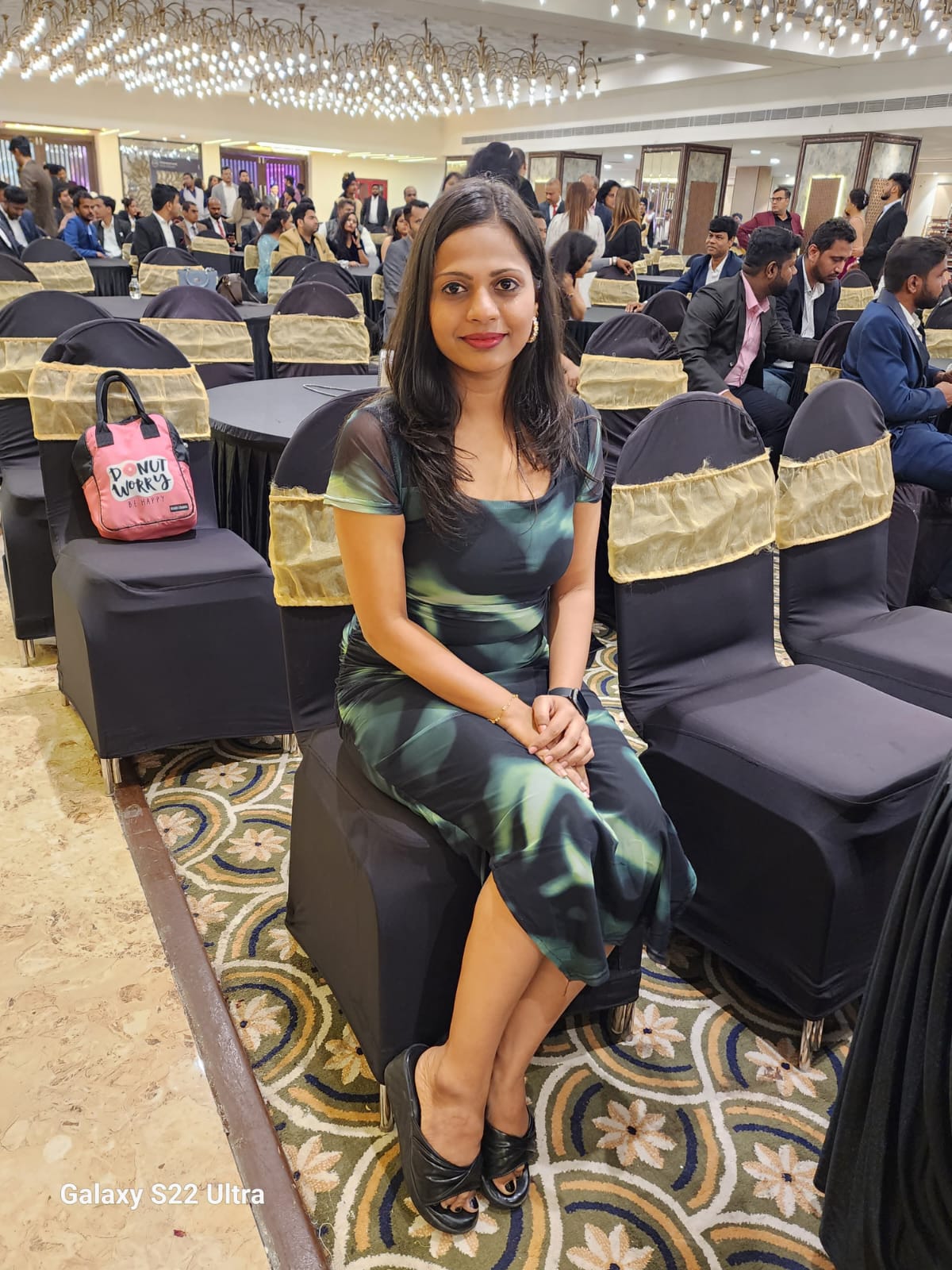