How to Deploy a Node.js App on AWS Lambda: Step-by-Step Instructions
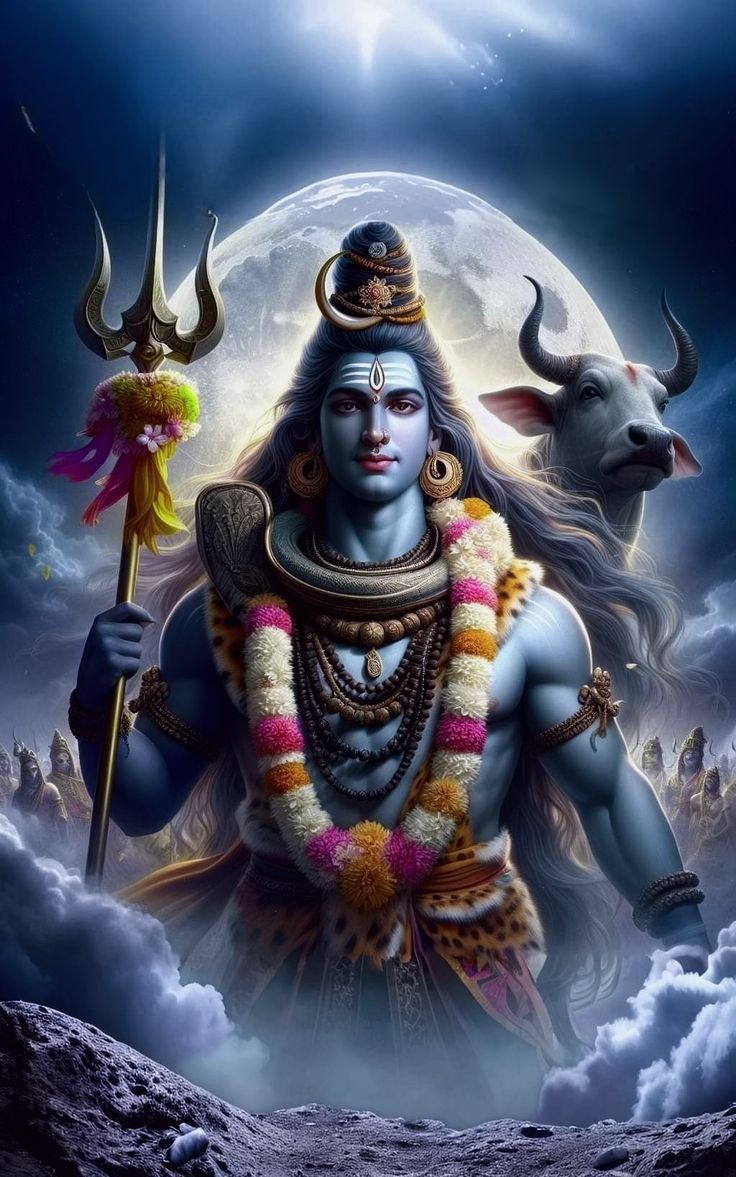
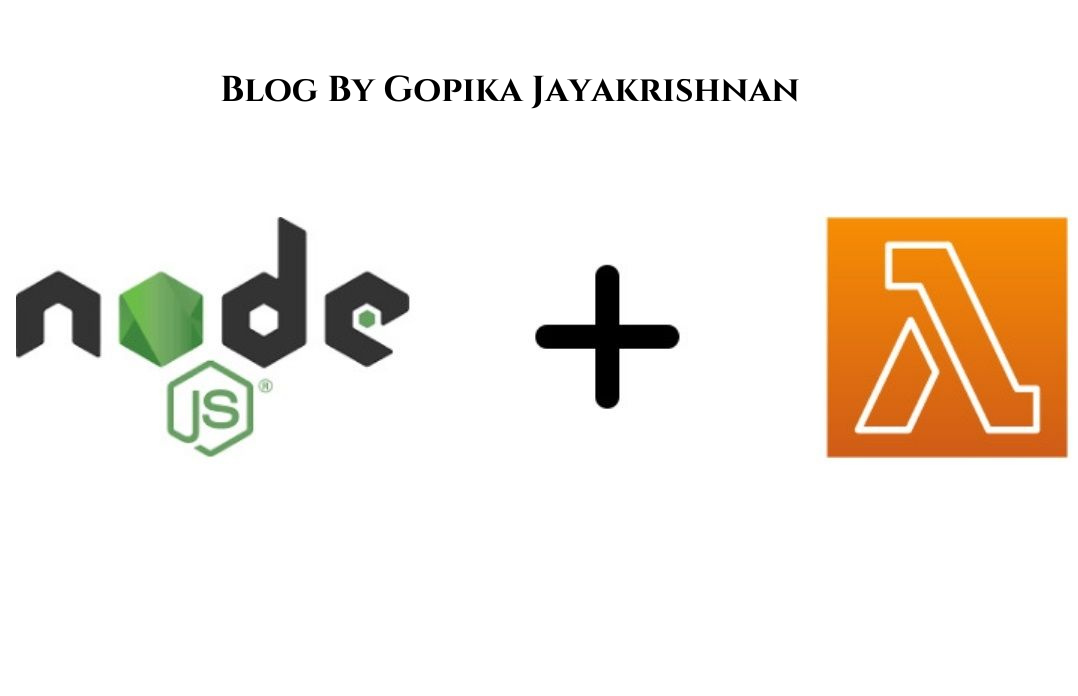
Introduction
AWS Lambda offers a serverless computing environment, enabling you to run code without the need for server management. Deploying a Node.js application to AWS Lambda can significantly enhance scalability and cost efficiency for your project. This guide will walk you through the process of deploying a Node.js app to AWS Lambda, from setting up your AWS environment to deploying and testing your application.
1. Setting Up Your AWS Environment
Create an AWS Account: If you havenβt already, sign up for an AWS account via the AWS Management Console. π
Install AWS CLI: Download and install the AWS Command Line Interface (CLI) on your local machine by following the installation instructions for your operating system here. π»
Configure AWS CLI: Open your terminal and run
aws configure
. Enter your AWS Access Key ID, Secret Access Key, default region, and default output format. π οΈ
2. Creating a Node.js Application
Initialize a Node.js Project: Create a new directory for your project and run
npm init
to set up a new Node.js project. Follow the prompts to configure your project. πInstall Dependencies: Add any necessary dependencies using
npm install
. π¦Write Your Application Code: Write your Node.js application code. For example, a simple Express.js server:
const express = require('express'); const app = express(); app.get('/', (req, res) => { res.send('Hello, AWS Lambda!'); }); app.listen(3000, () => { console.log('Server running on port 3000'); });
3. Packaging Your Application
Create a Deployment Package: Bundle your application code and dependencies into a ZIP file. Be sure to exclude development dependencies and any unnecessary files. π¦
- Example Package Command:
zip -r my-node-app.zip index.js node_modules
4. Deploying Your Application to AWS Lambda
Create an IAM Role: In the AWS Management Console, go to IAM and create a new IAM role for your Lambda function. Attach the
AWSLambdaBasicExecutionRole
policy to this role. π‘οΈDeploy Your Function: Use the AWS CLI to deploy your function to AWS Lambda. Replace
<function-name>
with a unique name for your function.
aws lambda create-function --function-name <function-name> --zip-file fileb://my-node-app.zip --handler index.handler --runtime nodejs14.x --role arn:aws:iam::<your-account-id>:role/<your-role-name>
- Test Your Function: Use the AWS Lambda console to test your function. You can also invoke your function using the AWS CLI. π
5. Updating Your Function
Update Your Application Code: Make necessary changes to your application code. βοΈ
Update Your Deployment Package: Create a new ZIP file with your updated code. π¦
Update Your Function: Use the AWS CLI to update your function with the new deployment package.
aws lambda update-function-code --function-name <function-name> --zip-file fileb://my-node-app.zip
Conclusion
Deploying a Node.js application to AWS Lambda simplifies server management and boosts scalability and cost efficiency. By following these steps, youβve set up your environment, created your application, and deployed it to Lambda. Enjoy the benefits of a serverless architecture and focus more on your code. π
Happy coding! If you have any questions or need further assistance, feel free to reach out. π
If you found this information helpful, please like, follow and share to spread the knowledge Thank You !!π€β¨.
Subscribe to my newsletter
Read articles from Gopika Jayakrishnan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
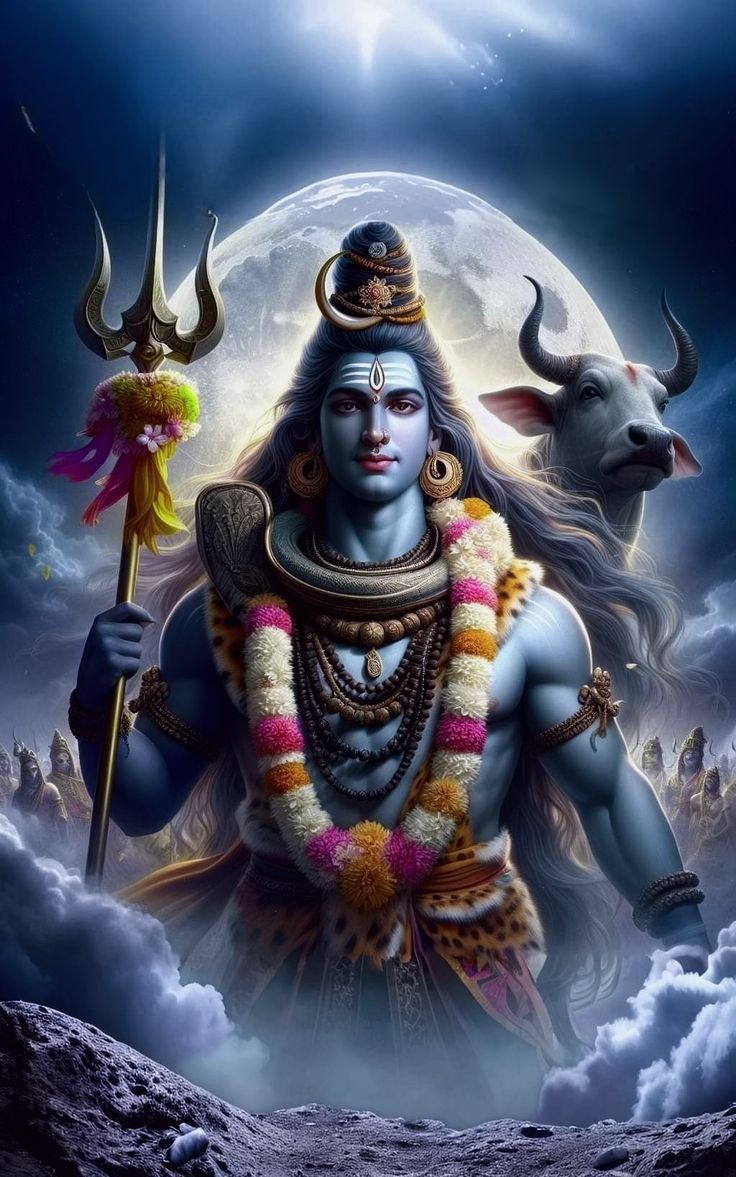
Gopika Jayakrishnan
Gopika Jayakrishnan
π I'm a Cloud DevOps engineer βοΈ. I develop, deploy, and maintain software applications in cloud environments, ensuring they are scalable, reliable, and secure π. My expertise lies in programming languages and cloud platforms, and I work collaboratively to deliver innovative solutions that meet business needs π.