Getting Started with Golang: A Beginner's Guide
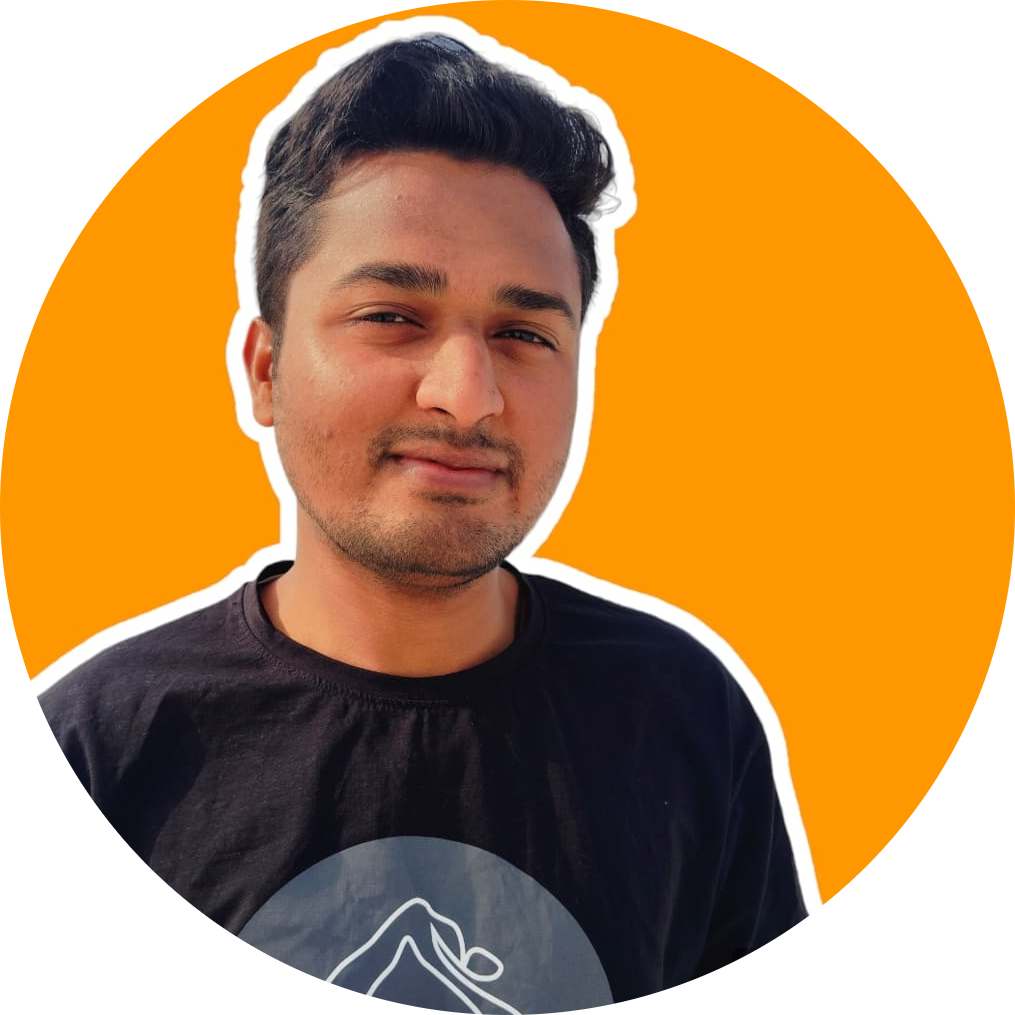
Introduction
Golang, or Go, is an open-source programming language designed by Google. It is known for its simplicity, efficiency, and strong concurrency support. In this blog series, we'll cover the basics of Golang, starting with its syntax, data types, and basic operations.
Setting Up the Environment
Before we start coding, ensure you have Go installed on your system. You can download and install it from the official website. also you can check my detailed instruction of golang Installation and Basics.
To verify the installation, open a terminal and run:
go version
You should see the installed Go version.
Hello, World!
Let's start with a simple "Hello, World!" program to get familiar with the basic structure of a Go program.
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
package main
: Defines the package name. Themain
package is a special package in Go.import "fmt"
: Imports thefmt
package, which contains functions for formatting text.func main()
: Defines themain
function, which is the entry point of the program.
Variables and Data Types
Go is a statically typed language, meaning the type of a variable is known at compile time.
Declaring Variables
You can declare variables using the var
keyword.
package main
import "fmt"
func main() {
var name string = "Alice"
var age int = 25
var height float64 = 5.8
var isStudent bool = true
fmt.Println("Name:", name)
fmt.Println("Age:", age)
fmt.Println("Height:", height)
fmt.Println("Is Student:", isStudent)
}
Go also supports type inference, allowing you to omit the type if it can be inferred from the value.
package main
import "fmt"
func main() {
name := "Bob"
age := 30
height := 6.1
isStudent := false
fmt.Println("Name:", name)
fmt.Println("Age:", age)
fmt.Println("Height:", height)
fmt.Println("Is Student:", isStudent)
}
Constants
Constants are declared using the const
keyword and cannot be changed after their declaration.
package main
import "fmt"
func main() {
const pi = 3.14
fmt.Println("Pi:", pi)
}
Basic Operations
Go supports various arithmetic, comparison, and logical operations.
Arithmetic Operations
package main
import "fmt"
func main() {
a := 10
b := 3
fmt.Println("Addition:", a+b)
fmt.Println("Subtraction:", a-b)
fmt.Println("Multiplication:", a*b)
fmt.Println("Division:", a/b)
fmt.Println("Modulus:", a%b)
}
Comparison Operations
package main
import "fmt"
func main() {
a := 10
b := 3
fmt.Println("Equal:", a == b)
fmt.Println("Not Equal:", a != b)
fmt.Println("Greater Than:", a > b)
fmt.Println("Less Than:", a < b)
fmt.Println("Greater or Equal:", a >= b)
fmt.Println("Less or Equal:", a <= b)
}
Logical Operations
package main
import "fmt"
func main() {
a := true
b := false
fmt.Println("AND:", a && b)
fmt.Println("OR:", a || b)
fmt.Println("NOT A:", !a)
}
Conclusion
In this first part of the Golang series, we've covered the basic syntax, variables, constants, and basic operations. Understanding these basics is crucial as they form the foundation of more complex concepts. In the next part, we'll explore control structures and functions in Golang. Stay tuned!
Let me know if you need any changes or additional information!
Subscribe to my newsletter
Read articles from Dhruvkumar Maisuria directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
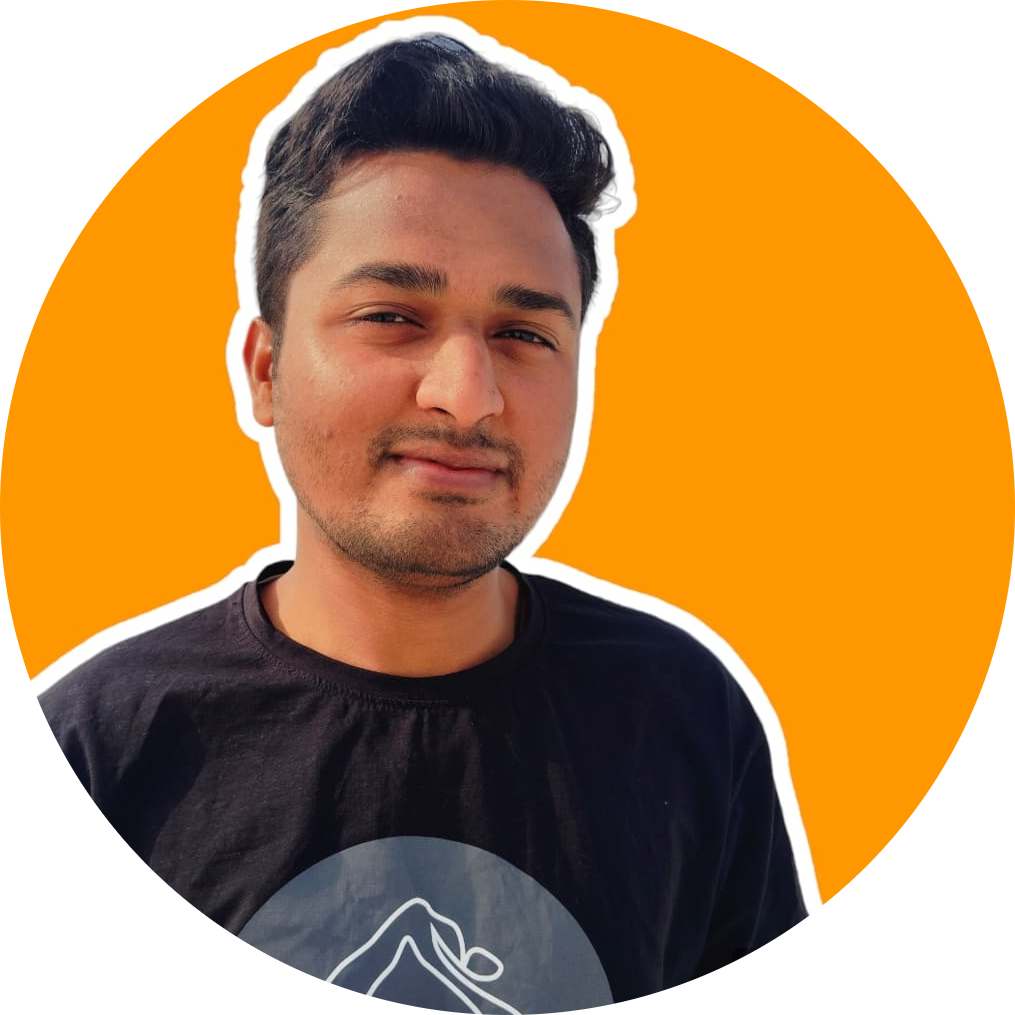
Dhruvkumar Maisuria
Dhruvkumar Maisuria
About Me As a dedicated tech enthusiast with a passion for continuous learning, I possess a robust background in software development and network engineering. My journey in the tech world is marked by an insatiable curiosity and a drive to solve complex problems through innovative solutions. I have honed my skills across a diverse array of technologies, including Python, AWS, SQL, and Golang, and have a strong foundation in web development, API testing, and cloud computing. My hands-on experience ranges from creating sophisticated applications to optimizing network performance, underscored by a commitment to excellence and a meticulous attention to detail. In addition to my technical prowess, I am an avid advocate for knowledge sharing, regularly contributing to the tech community through blogs and open-source projects. My proactive approach to professional development is demonstrated by my ongoing exploration of advanced concepts in programming and networking, ensuring that I stay at the forefront of industry trends. My professional journey is a testament to my adaptability and eagerness to embrace new challenges, making me a valuable asset in any dynamic, forward-thinking team. Whether working on a collaborative project or independently, I bring a blend of analytical thinking, creative problem-solving, and a deep-seated passion for technology to every endeavor.