Building DynamoWave Chat: A Comprehensive Guide to a Scalable, Serverless Real-Time Chat Application
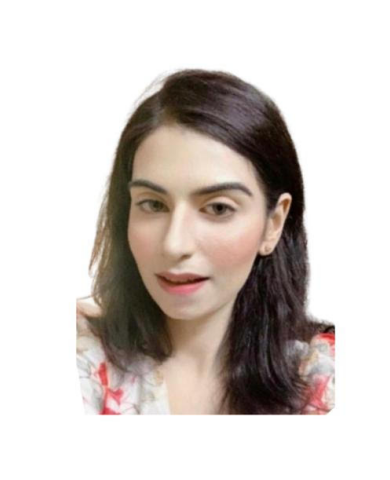
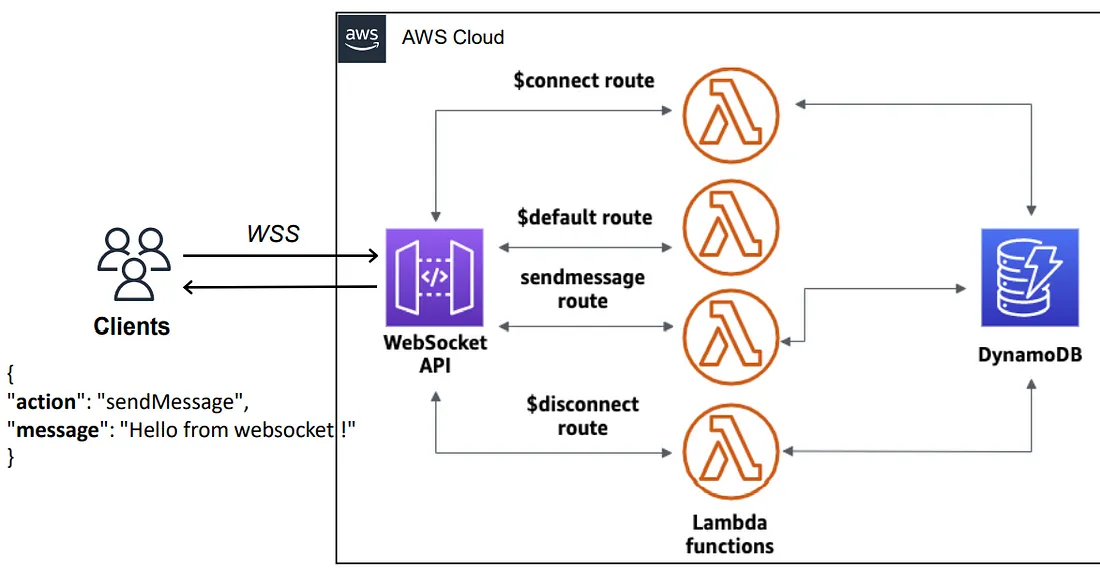
In the realm of real-time communication, creating a chat application that is both responsive and scalable is a complex yet highly rewarding challenge. In this article, I will walk you through the architecture and implementation details of DynamoWave Chat—a modern, scalable, serverless chat application I built using AWS services such as Lambda, DynamoDB, and API Gateway.
Introduction to DynamoWave Chat
DynamoWave Chat is a serverless real-time chat application designed with a core focus on enhancing non-functional requirements, making it both scalable and performant. By leveraging AWS's serverless offerings, I aimed to provide a seamless, responsive user experience.
System Architecture and Components
Workflow Overview
Establish WebSocket Connection:
API Gateway’s WebSocket API enables two-way communication between the client and the server.
The
ConnectHandler
Lambda function is triggered, inserting the connection ID into theConnectionsTable
in DynamoDB.
Client Notification:
- The client is notified upon successful connection establishment.
Message Handling:
- The
SendMessageHandler
Lambda function iterates through connection IDs and sends messages to connected clients.
- The
Session Termination:
- The
DisconnectHandler
function removes the connection ID from the registry once the session ends.
- The
Connection Closure:
- The connection is closed and resources are cleaned up.
Services and Their Purposes
Service | Identifier | Purpose |
API Gateway | WebSocket API | Real-time communication in the application |
DynamoDB | ConnectionsTable | Tracking and managing connections |
AWS Lambda | ConnectHandler | Recording new connections |
DisconnectHandler | Removing inactive connections | |
SendMessageHandler | Ensuring reliable communication across clients | |
DefaultHandler | Notifying the client upon successful connection establishment |
Design Considerations
Enhancing Availability and Reliability
Reserved Concurrency for Lambdas:
- Allocating reserved concurrency ensures that critical Lambda functions always have sufficient compute resources, preventing throttling during peak times.
Point-In-Time Recovery (PITR) for DynamoDB:
- Enabling PITR allows data restoration to any point in the last 35 days, improving data availability and fault tolerance.
API Throttling and Rate Limiting:
- Implementing throttling and rate limiting ensures that the backend services are not overwhelmed, maintaining the API's responsiveness and preventing potential DDoS attacks.
Regional Resilience:
- For critical applications requiring high availability across regions, consider using DynamoDB global tables to replicate data across multiple regions.
Code Optimizations for Lambda Reliability
Error Handling Mechanisms:
- Incorporating error handling within Lambda functions prevents cascading failures and ensures application stability.
Retry Logic with Exponential Backoff:
- Implementing retry logic with exponential backoff increases the probability of successful operation completion while reducing system load.
Dead Letter Queues (DLQs):
- Using DLQs for failed messages ensures zero data loss and provides opportunities for re-processing and analysis.
Enhancing API Gateway Availability
Regional Redundancy:
- Deploy API Gateway in multiple regions and use Route 53 for DNS failover to ensure availability in case of regional failures.
Cost-Effective Scalability
Adaptive Auto-Scaling for DynamoDB:
- Enable adaptive auto-scaling to automatically adjust capacity based on workload, optimizing resource utilization and cost.
Custom Lambda Warmer:
- Implement a custom Lambda warmer to reduce cold starts without incurring the constant cost of provisioned concurrency.
Security Considerations
HTTPS Enforcement:
- Use API Gateway resource policies to enforce HTTPS, ensuring secure communication.
Data Encryption:
- Enable KMS encryption for DynamoDB to secure data at rest.
Least Privilege IAM Policies:
- Apply the principle of least privilege to IAM policies, granting only the necessary permissions to Lambda functions and other components.
Detailed Functional Overview
1. Establishing Connection
When a client connects to the WebSocket API, API Gateway establishes a connection and assigns a unique connection ID. This ID is crucial for identifying and managing the connection throughout the session.
Example: ConnectHandler Lambda
import json
import boto3
import time
dynamodb = boto3.resource('dynamodb')
connection_table = dynamodb.Table('ConnectionsTable')
def lambda_handler(event, context):
connection_id = event['requestContext']['connectionId']
connection_table.put_item(Item={
'ConnectionID': connection_id,
'Timestamp': int(time.time())
})
return {
'statusCode': 200,
'body': json.dumps('Connected')
}
2. Sending Messages
Clients send messages through the WebSocket connection. API Gateway receives these messages and invokes the corresponding Lambda function based on the route selection expression (request.body.action
).
Example: SendMessageHandler Lambda
import json
import boto3
dynamodb = boto3.resource('dynamodb')
api_gateway = boto3.client('apigatewaymanagementapi', endpoint_url="https://your-api-id.execute-api.region.amazonaws.com/your-stage")
def lambda_handler(event, context):
connection_table = dynamodb.Table('ConnectionsTable')
body = json.loads(event['body'])
action = body.get('action')
if action == 'sendMessage':
message = body.get('message')
sender_id = event['requestContext']['connectionId']
# Retrieve all connection IDs
response = connection_table.scan()
for item in response['Items']:
connection_id = item['ConnectionID']
send_message(connection_id, message)
return {
'statusCode': 200,
'body': json.dumps('Message sent')
}
def send_message(connection_id, message):
try:
api_gateway.post_to_connection(
ConnectionId=connection_id,
Data=json.dumps({"message": message})
)
except Exception as e:
print(f"Failed to send message to {connection_id}: {str(e)}")
3. Disconnecting
When a client disconnects from the WebSocket API, the DisconnectHandler
Lambda function removes the connection ID from the registry.
Example: DisconnectHandler Lambda
import json
import boto3
dynamodb = boto3.resource('dynamodb')
connection_table = dynamodb.Table('ConnectionsTable')
def lambda_handler(event, context):
connection_id = event['requestContext']['connectionId']
connection_table.delete_item(
Key={'ConnectionID': connection_id}
)
return {
'statusCode': 200,
'body': json.dumps('Disconnected')
}
Real-Time Communication Workflow
Client Connection:
- When a client connects to the WebSocket API, API Gateway establishes a connection and assigns a unique connection ID for tracking and managing the connection.
Message Sending:
Clients send messages through the WebSocket connection.
API Gateway receives these messages and invokes the corresponding Lambda function based on the route selection expression (
request.body.action
).The Lambda function processes the message, retrieves the connection IDs from DynamoDB, and iterates through the list, sending the message to each connected client.
Real-Time Communication:
- The message is broadcasted to all connected clients, ensuring real-time communication across the platform.
Consistency and Collaboration for Real-Time Low Latency Communication
Consistency and Collaboration:
DynamoDB for State Management:
DynamoDB ensures that the connection state is consistently maintained.
Any changes (e.g., new connections, disconnections) are immediately reflected in the table.
Event-Driven Architecture:
- The use of Lambda functions triggered by events (e.g., messages, connections) ensures a responsive and scalable system.
API Gateway:
Manages WebSocket connections efficiently, ensuring low latency.
Provides a scalable entry point for client requests.
Error Handling and Retries:
Incorporating error handling and retries ensures that transient issues do not disrupt the communication flow.
Exponential backoff strategies help in managing retries effectively.
By maintaining a consistent state in DynamoDB, leveraging AWS Lambda for processing, and using API Gateway for efficient communication, the DynamoWave Chat application achieves real-time, low-latency communication, ensuring a seamless user experience.
Conclusion
DynamoWave Chat demonstrates the power and flexibility of AWS's serverless services in building a real-time, scalable chat application. By leveraging API Gateway, Lambda, and DynamoDB, I created a responsive and reliable platform that meets modern communication needs.
Building this application provided me with valuable insights into serverless architecture, real-time communication, and the challenges of scaling such a platform. I hope this deep dive into DynamoWave Chat inspires you to explore serverless solutions for your projects.
For more details and code examples, you can check out the DynamoWave Chat repository on GitHub.
Feel free to leave your thoughts and questions in the comments below. Happy coding!
By Tanishka Marrott
Certified AWS Solutions Architect | DevSecOps Engineer | Cloud Enthusiast
For more tech insights and project walkthroughs, follow me on LinkedIn and GitHub.
Subscribe to my newsletter
Read articles from Tanishka Marrott directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
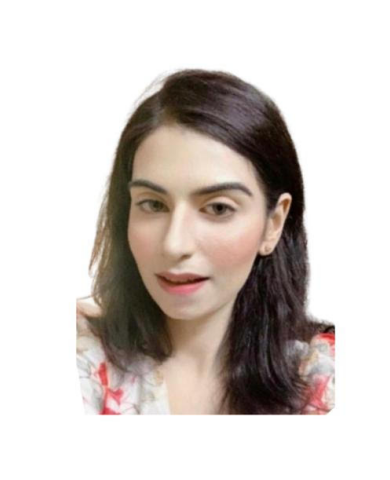
Tanishka Marrott
Tanishka Marrott
I'm a results-oriented cloud architect passionate about designing resilient cloud solutions. I specialize in building scalable architectures that meet business needs and are agile. With a strong focus on scalability, performance, and security, I ensure solutions are adaptable. My DevSecOps foundation allows me to embed security into CI/CD pipelines, optimizing deployments for security and efficiency. At Quantiphi, I led security initiatives, boosting compliance from 65% to 90%. Expertise in data engineering, system design, serverless solutions, and real-time data analytics drives my enthusiasm for transforming ideas into impactful solutions. I'm dedicated to refining cloud infrastructures and continuously improving designs. If our goals align, feel free to message me. I'd be happy to connect!