How to Use the Spread Operator in JavaScript
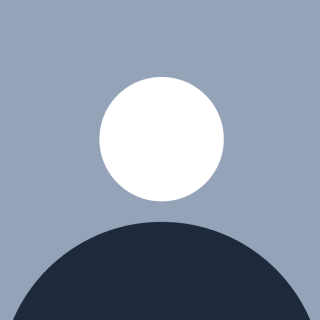
In JavaScript, the ...
(spread) syntax is used in several contexts to expand or spread iterable elements (like arrays, strings, or objects) into individual elements. It's a powerful feature introduced in ECMAScript 6 (ES6) that allows for concise and flexible handling of array-like structures. Here’s a breakdown of its uses:
1. Spread in Arrays
const arr1 = [1, 2, 3];
const arr2 = [...arr1, 4, 5];
console.log(arr2); // Output: [1, 2, 3, 4, 5]
In this example, ...arr1
spreads out the elements of arr1
into individual elements. So, arr2
becomes [1, 2, 3, 4, 5]
.
2. Spread in Function Calls
function sum(a, b, c) {
return a + b + c;
}
const numbers = [1, 2, 3];
console.log(sum(...numbers)); // Output: 6
Here, ...numbers
spreads the array numbers
into individual arguments sum(1, 2, 3)
, which are then summed up in the function.
3. Spread with Objects (Shallow Copy)
const obj1 = { foo: 'bar', x: 42 };
const obj2 = { ...obj1, y: 13 };
console.log(obj2); // Output: { foo: 'bar', x: 42, y: 13 }
When used with objects, ...
copies enumerable own properties from one object into another object. In this case, obj2
is a shallow copy of obj1
, with an additional property y
.
4. Spread in Function Arguments (Rest Parameters)
function multiply(multiplier, ...numbers) {
return numbers.map(num => num * multiplier);
}
console.log(multiply(2, 1, 2, 3)); // Output: [2, 4, 6]
In function declarations, ...
can be used as part of the rest parameters (...numbers
), which collects all remaining arguments into an array. Here, multiply(2, 1, 2, 3)
passes 2
as multiplier
and [1, 2, 3]
as numbers
.
5. Spread with Strings
const str = 'hello';
const chars = [...str];
console.log(chars); // Output: ['h', 'e', 'l', 'l', 'o']
Even though strings are not arrays, the spread syntax treats them as iterable, allowing you to spread each character of the string into an array.
Subscribe to my newsletter
Read articles from Pawan S directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Pawan S
Pawan S
Understanding technology.