Mastering Higher Order Functions in OCaml: A Deep Dive
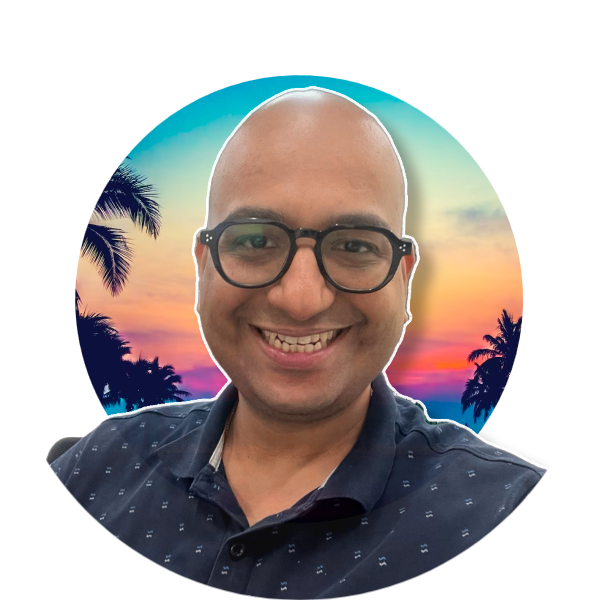
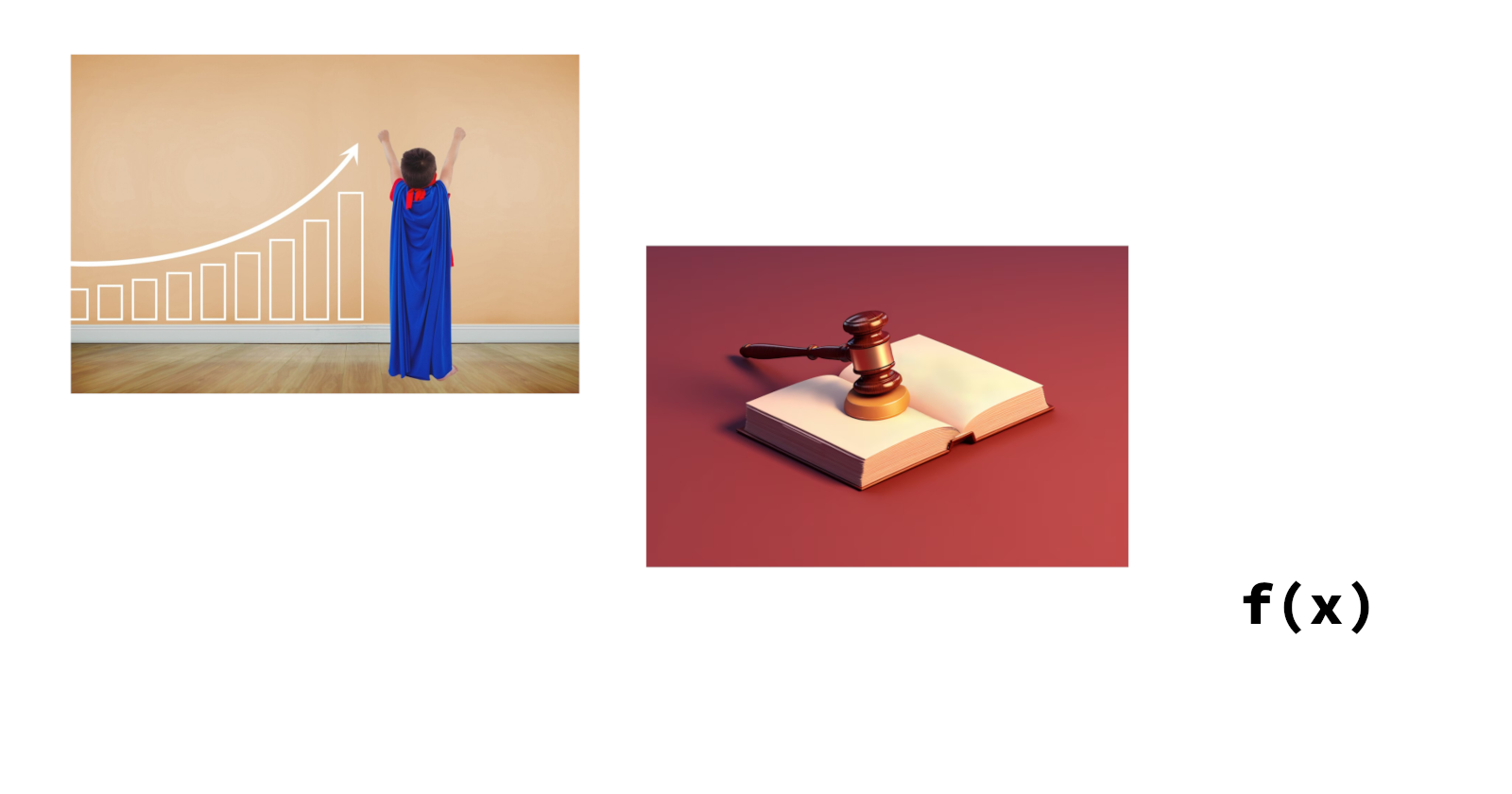
Introduction
Higher Order Functions (HOFs) are a cornerstone of functional programming, and OCaml, as a multi-paradigm language with strong functional capabilities, provides excellent support for them. These functions, which can accept other functions as arguments or return functions as results, are powerful tools that enable developers to write more abstract, reusable, and expressive code.
In OCaml, HOFs are not just a feature but a fundamental part of the language's design philosophy. They allow programmers to work at a higher level of abstraction, manipulating and composing functions as easily as they would manipulate data. This capability is particularly valuable in OCaml, where the type system ensures that these function manipulations are performed safely and efficiently.
The use of HOFs in OCaml leads to more concise and readable code, reduces redundancy, and promotes a style of programming that aligns closely with mathematical thinking. Whether you're processing lists, creating custom control structures, or implementing complex algorithms, HOFs provide a elegant and powerful approach. In this article, we'll explore the concept of Higher Order Functions in OCaml, examine some common examples, discuss their use cases, and highlight their key features and benefits.
Examples
- Map function:
let map f list =
let rec map_aux acc = function
| [] -> List.rev acc
| x :: xs -> map_aux (f x :: acc) xs
in
map_aux [] list
let squares = map (fun x -> x * x) [1; 2; 3; 4; 5]
(* Result: [1; 4; 9; 16; 25] *)
The map
function is a classic example of a higher order function. It takes a function f
and a list, applying f
to each element of the list and returning a new list with the results.
- Fold (reduce) function:
let fold_left f init list =
let rec fold_aux acc = function
| [] -> acc
| x :: xs -> fold_aux (f acc x) xs
in
fold_aux init list
let sum = fold_left (+) 0 [1; 2; 3; 4; 5]
(* Result: 15 *)
fold_left
is another powerful HOF that reduces a list to a single value by applying a function f
to each element and an accumulator.
- Function composition:
let (>>) f g x = g (f x)
let increment x = x + 1
let double x = x * 2
let increment_then_double = increment >> double
let result = increment_then_double 5
(* Result: 12 *)
This example demonstrates how HOFs can be used to compose functions, creating new functions from existing ones.
- Partial application:
let add x y = x + y
let add5 = add 5
let result = add5 3
(* Result: 8 *)
Partial application, a feature closely related to HOFs, allows us to create new functions by fixing some arguments of existing functions.
Use cases
List processing Higher order functions like map, filter, and fold are invaluable for processing lists and other collections. They allow for expressive and concise data transformations and aggregations.
Callback mechanisms HOFs enable the implementation of callback systems, where functions are passed as arguments to be called at a later time or under specific conditions. This is particularly useful in event-driven programming or when working with asynchronous operations.
Strategy pattern implementation The strategy pattern, which allows selecting an algorithm at runtime, can be elegantly implemented using HOFs. Different strategies can be represented as functions and easily swapped.
Creating domain-specific languages (DSLs) HOFs can be used to build expressive DSLs within OCaml. By combining and composing functions, you can create a vocabulary of operations specific to a particular domain.
Lazy evaluation and streams HOFs are crucial in implementing lazy evaluation strategies and working with potentially infinite streams of data. They allow for the definition of computation that is only executed when needed.
Features
In OCaml, Higher Order Functions come with several key features that make them particularly powerful:
Type inference: OCaml's strong type system, combined with its powerful type inference, ensures that HOFs are used correctly and safely. The compiler can often deduce the types of function arguments and return values without explicit annotations.
Currying: All functions in OCaml are automatically curried, which means that a function taking multiple arguments can be partially applied, returning a new function that takes the remaining arguments. This feature works seamlessly with HOFs.
First-class functions: Functions in OCaml are first-class citizens, meaning they can be passed as arguments, returned from other functions, and assigned to variables just like any other value.
Polymorphism: OCaml's parametric polymorphism allows writing HOFs that work with functions of various types, increasing their reusability and expressiveness.
Tail-call optimization: OCaml optimizes tail-recursive calls, which is particularly useful when implementing HOFs that process large data structures or perform recursive computations.
Take-away
Higher Order Functions in OCaml represent a powerful paradigm that elevates the language's expressive capabilities. By treating functions as first-class citizens, OCaml enables developers to write more abstract, reusable, and elegant code. HOFs facilitate a declarative programming style, allowing developers to focus on what needs to be done rather than how to do it. The combination of HOFs with OCaml's strong type system and efficient compilation results in code that is not only expressive and flexible but also safe and performant. As you delve deeper into OCaml programming, mastering Higher Order Functions will undoubtedly enhance your ability to solve complex problems with clarity and efficiency.
Image Attribution
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
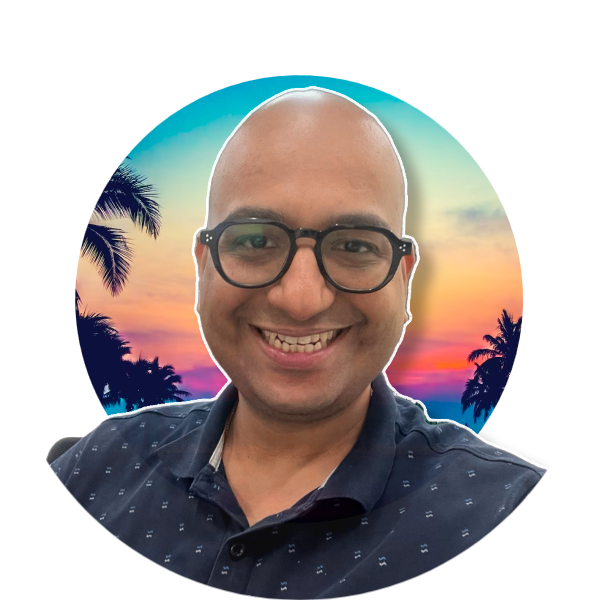
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.