Hyperparameter Tuning in Gradient Boosting: Best Practices and Common Pitfalls

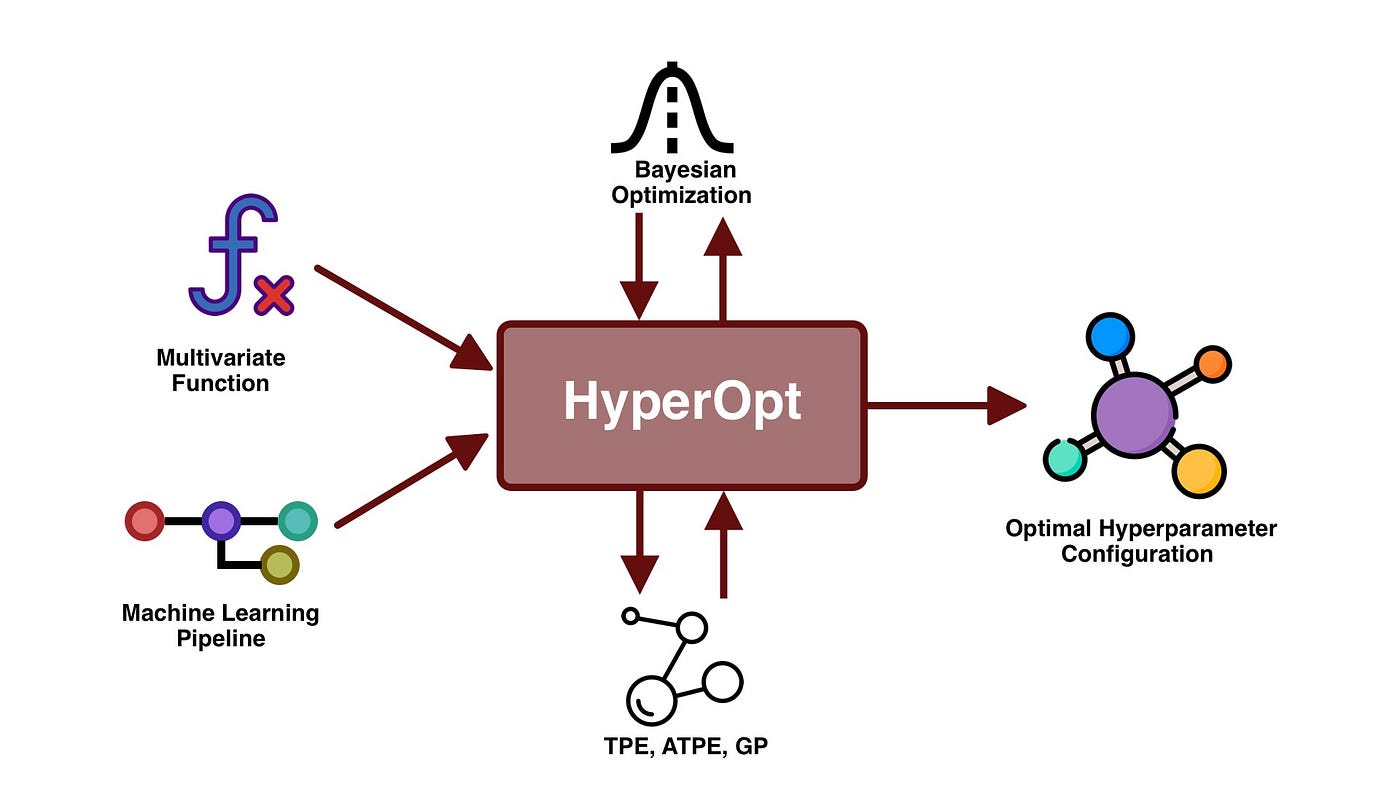
In our previous blog, we explored the fundamentals of the gradient boosting algorithm, a powerful ensemble technique used for both classification and regression tasks. Now, let's dive deeper into the topic by discussing hyperparameter tuning, which is crucial for optimizing the performance of gradient boosting models.
Why Hyperparameter Tuning Matters
Hyperparameters are the settings that control the learning process of a machine learning algorithm. Unlike model parameters, which are learned from the data, hyperparameters need to be set before training. Proper tuning of these hyperparameters can significantly improve the model's performance.
Key Hyperparameters in Gradient Boosting
Learning Rate: Controls the contribution of each tree to the final model. A smaller learning rate requires more trees but can lead to better performance.
Number of Trees (n_estimators): The number of boosting stages to be run. More trees can improve performance but also increase the risk of overfitting.
Maximum Depth (max_depth): The maximum depth of each tree. Deeper trees can capture more complex patterns but are more prone to overfitting.
Minimum Samples Split (min_samples_split): The minimum number of samples required to split an internal node. Higher values prevent overfitting.
Minimum Samples Leaf (min_samples_leaf): The minimum number of samples required to be at a leaf node. Higher values can smooth the model.
Subsample: The fraction of samples to be used for fitting each tree. Lower values can reduce overfitting.
Best Practices for Hyperparameter Tuning
Start with a Baseline Model: Begin with default hyperparameters to establish a baseline performance.
Use Grid Search or Random Search: Systematically explore a range of hyperparameter values using techniques like Grid Search or Random Search.
Cross-Validation: Use cross-validation to evaluate the performance of different hyperparameter combinations and avoid overfitting.
Monitor Performance Metrics: Track metrics such as accuracy, precision, recall, or mean squared error to guide your tuning process.
Iterative Approach: Gradually refine your hyperparameters based on the results of your initial searches.
Common Pitfalls to Avoid
Overfitting: Be cautious of overfitting, especially with high values of
n_estimators
andmax_depth
. Use techniques like early stopping to mitigate this risk.Ignoring Learning Rate: A common mistake is to overlook the learning rate. A smaller learning rate with more trees often yields better results.
Not Using Cross-Validation: Relying solely on training data performance can lead to overfitting. Always use cross-validation.
Inadequate Search Space: Limiting the range of hyperparameters can prevent finding the optimal settings. Ensure a broad and comprehensive search space.
Example Code for Hyperparameter Tuning
Here is an example of how to perform hyperparameter tuning using Grid Search with Scikit-Learn:
from sklearn.ensemble import GradientBoostingClassifier
from sklearn.model_selection import GridSearchCV
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
# Load dataset
data = load_iris()
X_train, X_test, y_train, y_test = train_test_split(data.data, data.target, test_size=0.2, random_state=42)
# Define the model
model = GradientBoostingClassifier()
# Define the parameter grid
param_grid = {
'learning_rate': [0.01, 0.1, 0.2],
'n_estimators': [100, 200, 300],
'max_depth': [3, 4, 5],
'min_samples_split': [2, 5, 10],
'min_samples_leaf': [1, 2, 4],
'subsample': [0.8, 0.9, 1.0]
}
# Perform Grid Search
grid_search = GridSearchCV(estimator=model, param_grid=param_grid, cv=5, scoring='accuracy', n_jobs=-1)
grid_search.fit(X_train, y_train)
# Print the best parameters and score
print("Best Parameters:", grid_search.best_params_)
print("Best Score:", grid_search.best_score_)
Additional Resources
By following these best practices and avoiding common pitfalls, you can effectively tune the hyperparameters of your gradient boosting models and achieve better performance.
Happy tuning!!
Happy Coding!!
Happy Coding Inferno!!
Subscribe to my newsletter
Read articles from Sujit Nirmal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sujit Nirmal
Sujit Nirmal
๐ Hi there! I'm Sujit Nirmal, a AI /ML Developer with a passion for creating intelligent, seamless ML applications. With a strong foundation in both machine learning and Deep Learning I thrive at the intersection of data and technology.