Mastering XGBoost: A Comprehensive Guide to Hyperparameter Tuning

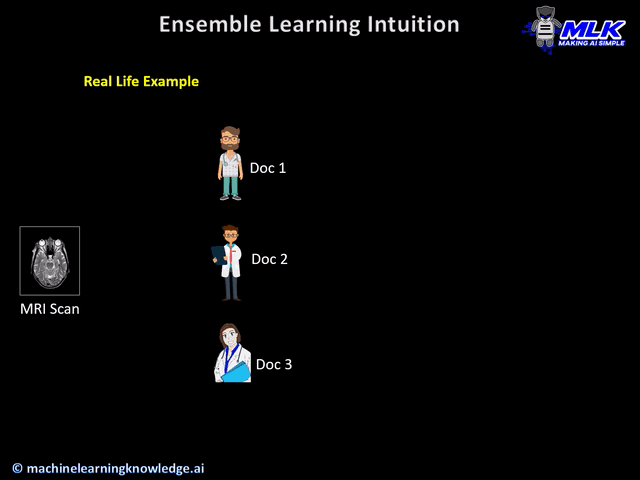
Introduction
Welcome back, fellow data enthusiasts! In our last blog, we explored the intricacies of hyperparameter tuning in Gradient Boosting Machines (GBM). Today, we are going to take a step further and dive into XGBoost (Extreme Gradient Boosting), a more advanced and efficient implementation of gradient boosting. XGBoost has gained immense popularity due to its speed and performance, making it a go-to choice for many data scientists and machine learning practitioners.
In this blog, we will cover the following:
Introduction to XGBoost
Key hyperparameters in XGBoost
Practical examples with code
Real-life applications and case studies
Additional resources for further learning
What is XGBoost?
XGBoost is an optimized distributed gradient boosting library designed to be highly efficient, flexible, and portable. It implements machine learning algorithms under the Gradient Boosting framework. XGBoost provides a parallel tree boosting (also known as GBDT, GBM) that solves many data science problems in a fast and accurate way.
Key Hyperparameters in XGBoost
Understanding and tuning hyperparameters is crucial for getting the best performance out of XGBoost. Here are some of the most important hyperparameters:
Learning Rate (
eta
): Controls the step size at each iteration while moving towards a minimum of the loss function. Lower values make the model more robust to overfitting but require more trees.Number of Trees (
n_estimators
): The number of boosting rounds.Maximum Depth (
max_depth
): The maximum depth of a tree. Increasing this value makes the model more complex and more likely to overfit.Subsample: The fraction of samples to be used for fitting the individual base learners.
Colsample_bytree: The fraction of features to be used for fitting the individual base learners.
Gamma: Minimum loss reduction required to make a further partition on a leaf node of the tree.
Lambda: L2 regularization term on weights (analogous to Ridge regression).
Alpha: L1 regularization term on weights (analogous to Lasso regression).
Practical Examples with Code
Let's dive into some practical examples to see how these hyperparameters can be tuned for optimal performance.
Example: Predicting House Prices
We'll use the famous Boston Housing dataset for this example.
import xgboost as xgb
from sklearn.model_selection import train_test_split, GridSearchCV
from sklearn.datasets import load_boston
from sklearn.metrics import mean_squared_error
# Load dataset
boston = load_boston()
X, y = boston.data, boston.target
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Initialize XGBoost model
xg_reg = xgb.XGBRegressor(objective='reg:squarederror')
# Define hyperparameter grid
param_grid = {
'learning_rate': [0.01, 0.1, 0.2],
'max_depth': [3, 5, 7],
'n_estimators': [100, 200, 300],
'subsample': [0.8, 1.0],
'colsample_bytree': [0.8, 1.0]
}
# Perform grid search
grid_search = GridSearchCV(estimator=xg_reg, param_grid=param_grid, scoring='neg_mean_squared_error', cv=5, verbose=1)
grid_search.fit(X_train, y_train)
# Best parameters
print("Best parameters found: ", grid_search.best_params_)
# Train model with best parameters
best_xg_reg = grid_search.best_estimator_
best_xg_reg.fit(X_train, y_train)
# Predict and evaluate
y_pred = best_xg_reg.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
print(f"Mean Squared Error: {mse}")
Real-Life Applications and Case Studies
XGBoost is used in a variety of real-life applications, from predicting customer churn to classifying images. Here are a few case studies:
Kaggle Competitions: XGBoost has been a key algorithm in many winning solutions on Kaggle.
Finance: Used for credit scoring and fraud detection.
Healthcare: Predicting patient outcomes and disease progression.
Additional Resources
To further enhance your understanding of XGBoost and hyperparameter tuning, here are some valuable resources:
Conclusion
XGBoost is a powerful tool in the machine learning toolkit, and mastering its hyperparameters can significantly boost your model's performance. We hope this guide has provided you with a solid foundation to start experimenting with XGBoost in your projects.
Happy coding ! !
Happy coding Inferno ! !
Subscribe to my newsletter
Read articles from Sujit Nirmal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sujit Nirmal
Sujit Nirmal
๐ Hi there! I'm Sujit Nirmal, a AI /ML Developer with a passion for creating intelligent, seamless ML applications. With a strong foundation in both machine learning and Deep Learning I thrive at the intersection of data and technology.