Understanding JavaScript String Methods: A Practical Guide
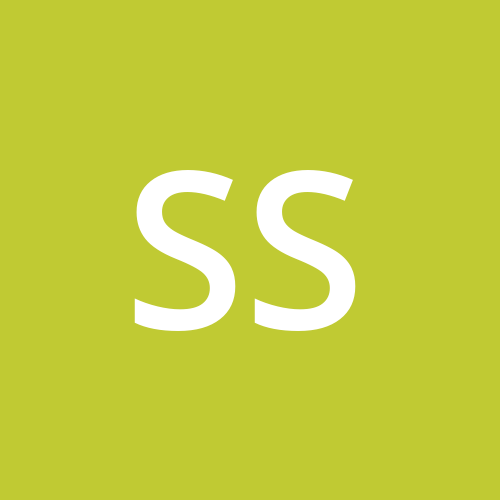
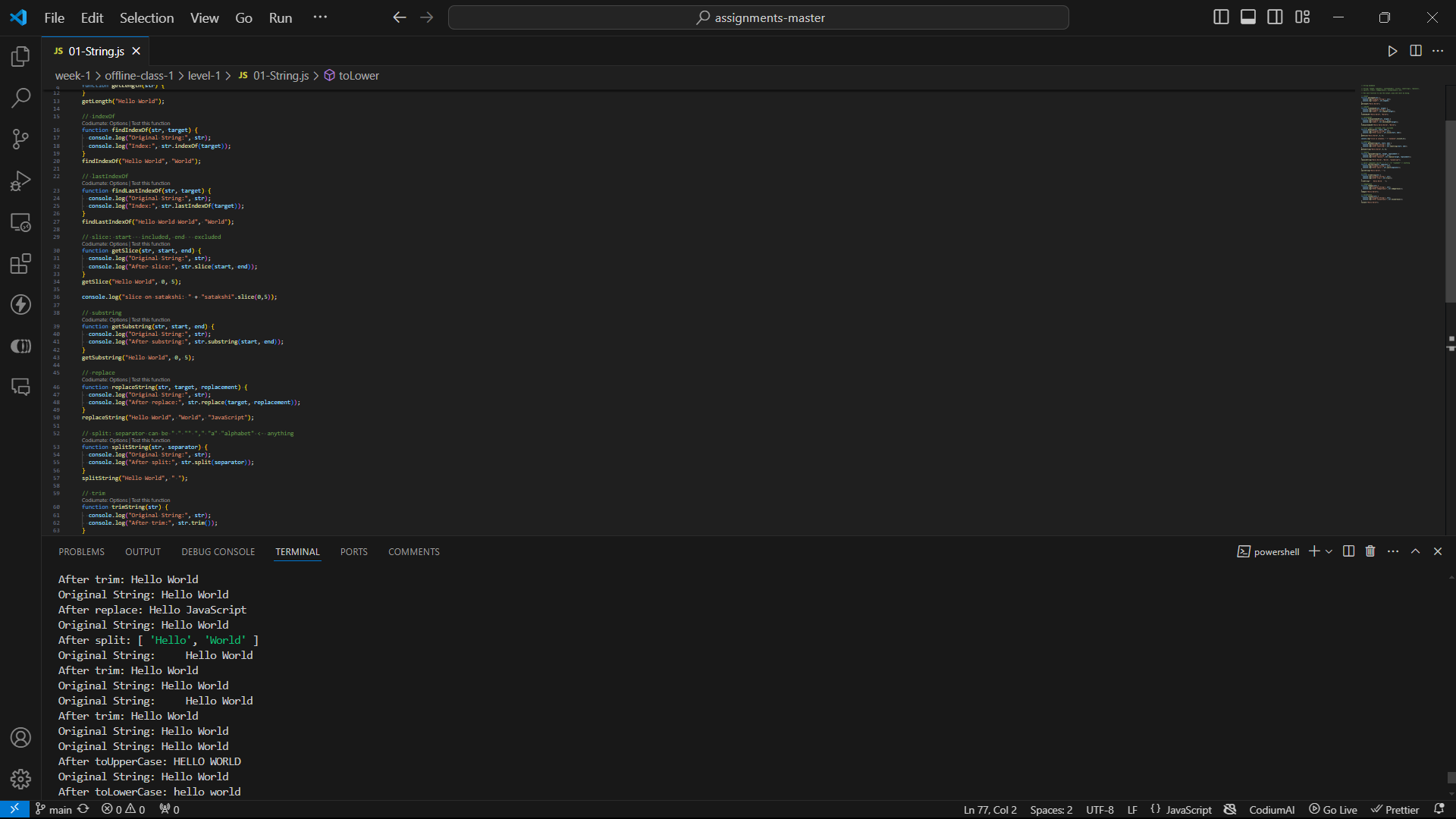
Strings are one of the most fundamental data types in JavaScript. They are used to represent and manipulate text. JavaScript provides a variety of built-in methods to handle strings efficiently.
Today, we'll explore some essential string methods, including length
, indexOf()
, lastIndexOf()
, slice()
, substring()
, replace()
, split()
, trim()
, toUpperCase()
, and toLowerCase()
.
Let's dive in and see how these methods work through practical examples.
String Length
The length
property returns the number of characters in a string.
function getLength(str) {
console.log("Original String:", str);
console.log("Length:", str.length);
}
getLength("Hello World");
Output:
Original String: Hello World
Length: 11
Finding Index of a Substring
The indexOf()
method returns the index of the first occurrence of a specified substring.
function findIndexOf(str, target) {
console.log("Original String:", str);
console.log("Index:", str.indexOf(target));
}
findIndexOf("Hello World", "World");
Output:
Original String: Hello World
Index: 6
Finding Last Index of a Substring
The lastIndexOf()
method returns the index of the last occurrence of a specified substring.
function findLastIndexOf(str, target) {
console.log("Original String:", str);
console.log("Index:", str.lastIndexOf(target));
}
findLastIndexOf("Hello World World", "World");
Output:
Original String: Hello World World
Index: 12
Extracting a Portion of a String
The slice()
method extracts a section of a string and returns it as a new string. The start
index is inclusive, and the end
index is exclusive.
function getSlice(str, start, end) {
console.log("Original String:", str);
console.log("After slice:", str.slice(start, end));
}
getSlice("Hello World", 0, 5);
Output:
Original String: Hello World
After slice: Hello
Extracting a Substring
The substring()
method is similar to slice()
, but it doesn't accept negative indices.
function getSubstring(str, start, end) {
console.log("Original String:", str);
console.log("After substring:", str.substring(start, end));
}
getSubstring("Hello World", 0, 5);
Output:
Original String: Hello World
After substring: Hello
Replacing Substrings
The replace()
method searches for a specified value or a regular expression in a string and returns a new string with the specified value replaced.
function replaceString(str, target, replacement) {
console.log("Original String:", str);
console.log("After replace:", str.replace(target, replacement));
}
replaceString("Hello World", "World", "JavaScript");
Output:
Original String: Hello World
After replace: Hello JavaScript
Splitting a String into an Array
The split()
method divides a string into an array of substrings using a specified separator.
function splitString(str, separator) {
console.log("Original String:", str);
console.log("After split:", str.split(separator));
}
splitString("Hello World", " ");
Output:
Original String: Hello World
After split: ["Hello", "World"]
Trimming Whitespace
The trim()
method removes whitespace from both ends of a string.
function trimString(str) {
console.log("Original String:", str);
console.log("After trim:", str.trim());
}
trimString(" Hello World ");
Output:
Original String: Hello World
After trim: Hello World
Converting to Uppercase
The toUpperCase()
method converts a string to uppercase letters.
function toUpper(str) {
console.log("Original String:", str);
console.log("After toUpperCase:", str.toUpperCase());
}
toUpper("Hello World");
Output:
Original String: Hello World
After toUpperCase: HELLO WORLD
Converting to Lowercase
The toLowerCase()
method converts a string to lowercase letters.
function toLower(str) {
console.log("Original String:", str);
console.log("After toLowerCase:", str.toLowerCase());
}
toLower("Hello World");
Output:
Original String: Hello World
After toLowerCase: hello world
Subscribe to my newsletter
Read articles from Satakshi Shanvi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
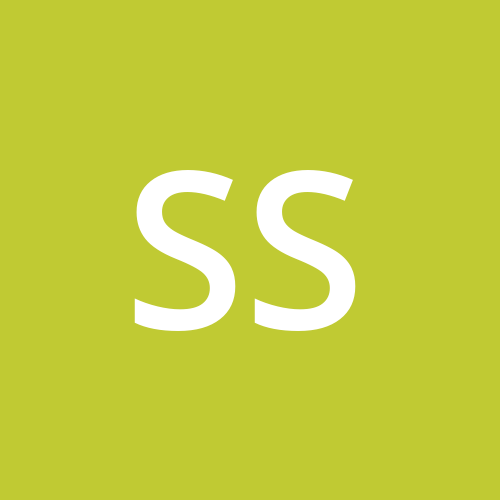