Scheduling Generative AI Jobs Inside Azure Container Apps

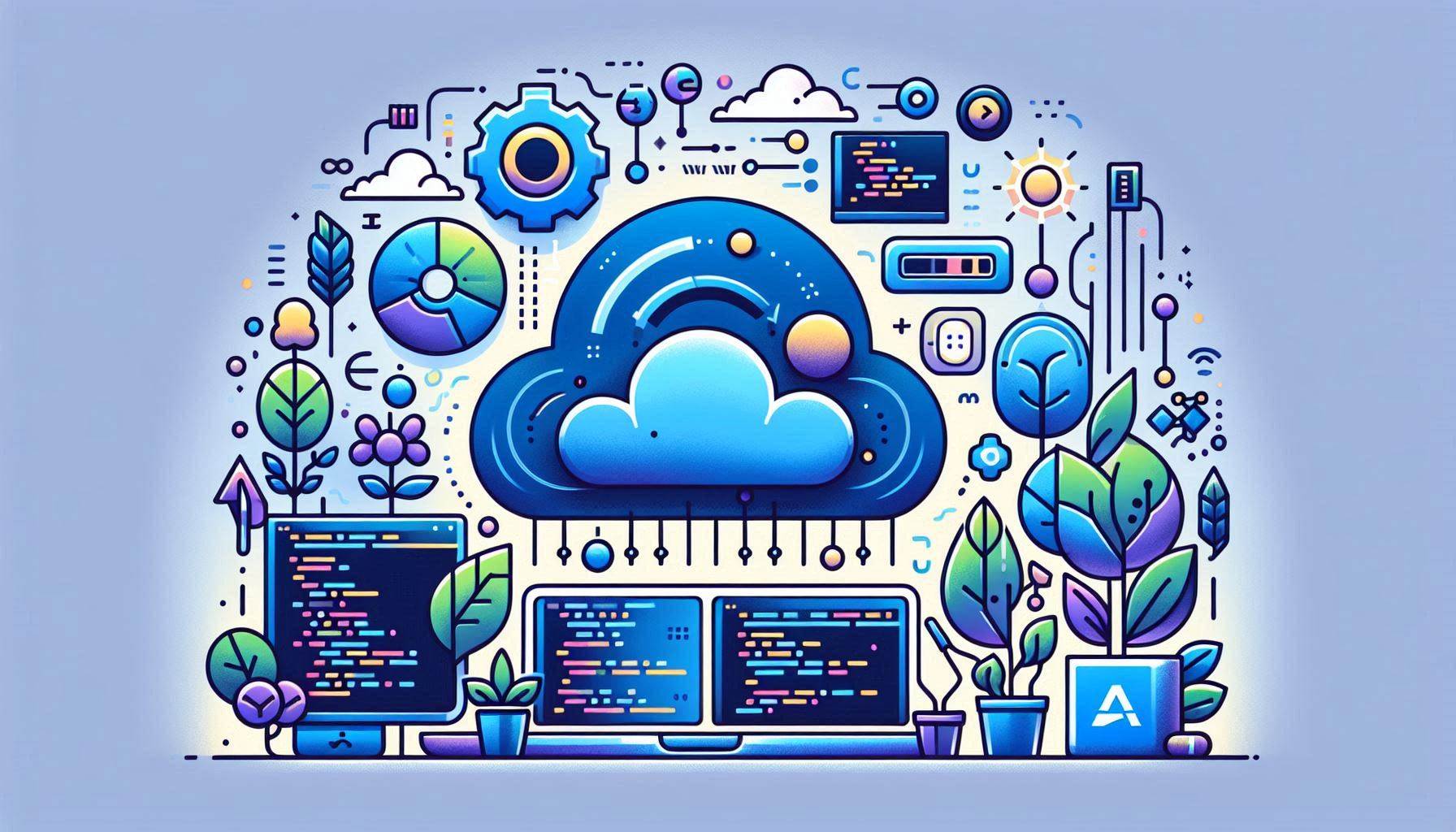
As you build a generative AI application, you might need to schedule jobs to perform tasks such as data processing, model training, or output generation. In this blog post, we'll explore how to use the CronScheduler.AspNetCore library to schedule jobs inside an Azure Container App.
What is CronSchedulerApp?
CronSchedulerApp is a .NET Core web application that demonstrates various scheduled job scenarios using the CronScheduler.AspNetCore library. It includes examples of background tasks, startup jobs, and scheduled jobs that can be used in your own applications.
Benefits of Using Scheduled Jobs
Scheduled jobs offer several benefits for your generative AI application:
Efficient processing: By scheduling jobs to run at specific times or intervals, you can ensure that computationally intensive tasks are executed during off-peak hours or when system resources are available.
Improved reliability: Scheduled jobs can be configured to retry failed tasks or execute alternative tasks if the primary job fails.
Enhanced scalability: Azure Container Apps provide a scalable platform for your application. By scheduling jobs, you can take advantage of this scalability and ensure that your application can handle increased traffic or processing demands.
Getting Started with CronSchedulerApp
To get started with CronSchedulerApp, follow these steps:
Install the NuGet package: Install the
CronScheduler.AspNetCore
NuGet package in your .NET Core project.Configure the scheduler: In your
Program.cs
, configure the scheduler using theAddScheduler
method. This method allows you to add jobs and customize job options, such as the run interval, maximum retries, and error handling.
Example Job: TorahQuoteJob
Let's take a look at an example job, TorahQuoteJob
, which retrieves a random verse from the Torah and updates the current verses in the TorahVerses
service:
public class TorahQuoteJob : IScheduledJob
{
private readonly ILogger<TorahQuoteJob> _logger;
private readonly ITorahVersesService _torahVersesService;
public TorahQuoteJob(ILogger<TorahQuoteJob> logger, ITorahVersesService torahVersesService)
{
_logger = logger;
_torahVersesService = torahVersesService;
}
public string Name { get; } = nameof(TorahQuoteJob);
public async Task ExecuteAsync(CancellationToken cancellationToken)
{
_logger.LogInformation("Executing scheduled job: {jobName}", Name);
// Retrieve a random verse from the Torah
var verse = await _torahVersesService.GetRandomVerse();
// Update the current verses in the `TorahVerses` service
await _torahVersesService.UpdateCurrentVerses(verse);
await Task.CompletedTask;
}
}
Configuring Job Options
You can configure job options using a custom class that inherits from SchedulerOptions
. For example:
public class TorahQuoteJobOptions : SchedulerOptions
{
public string SomeOption { get; set; } = string.Empty;
}
Registering the Job
To register the TorahQuoteJob
with the scheduler, use the following code:
builder.Services.AddScheduler(builder =>
{
builder.Services.AddSingleton<TorahVerses>();
builder.Services
.AddHttpClient<TorahService>()
.AddTransientHttpErrorPolicy(p => p.RetryAsync());
builder.AddJob<TorahQuoteJob, TorahQuoteJobOptions>();
builder.Services.AddScoped<UserService>();
builder.AddJob<UserJob, UserJobOptions>();
builder.AddUnobservedTaskExceptionHandler(sp =>
{
var logger = sp.GetRequiredService<ILoggerFactory>().CreateLogger("CronJobs");
return (sender, args) =>
{
logger?.LogError(args.Exception?.Message);
args.SetObserved();
};
});
});
Running the Application
To run your application with scheduled jobs, follow these steps:
Build and deploy: Build and deploy your .NET Core project to Azure Container Apps.
Configure the scheduler: Configure the scheduler using the
AddScheduler
method in yourProgram.cs
.Run the application: Run your application to execute scheduled jobs based on their configured schedules.
In this blog post, we've explored how to use CronScheduler.AspNetCore library with CronSchedulerApp to schedule generative AI jobs inside Azure Container Apps. By leveraging scheduled jobs, you can improve the efficiency, reliability, and scalability of your application.
Happy coding..
Subscribe to my newsletter
Read articles from King David Consulting LLC directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

King David Consulting LLC
King David Consulting LLC
A highly skilled Cloud Solutions Architect with 20+ years of experience in software application development across diverse industries. Offering expertise in Cloud Computing and Artificial Intelligence. Passionate about designing and implementing innovative cloud-based solutions, migrating applications to the cloud, and integrating third-party platforms. Dedicated to collaborating with other developers and contributing to open-source projects to enhance software application functionality and performance