15 ways to know which page is easy to scrape? w/ visual sample ๐งโ๐ป
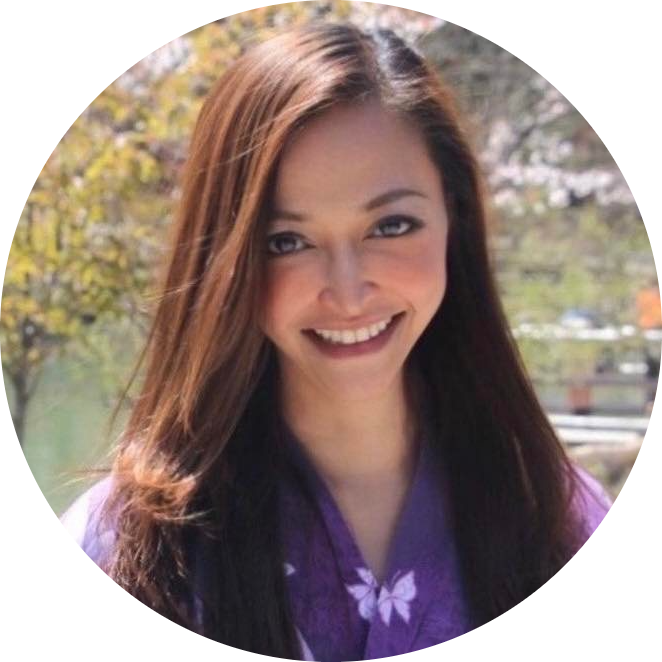
Table of contents
- 1. Static vs. Dynamic Page ๐ฟ
- 2. Consistent vs Non-consistent page layout ๐พ
- 3. Clear CSS Classes VS unclear ๐ก
- 3. Use AJAX or not?๐ค
- 4. Any Anti-bot measures? ๐คฌ
- 5. Paginated Content or not?
- 6. HTML Source
- 7. Network Tab
- 8. Console Errors
- 9. Scrolling Behavior
- 10.URL Structure
- 11.Login Requirements
- 12.Interactive Elements
- 13.Data Consistency
- 14.API Calls
- 15.Robots.txt
To determine if a website is easy or hard to scrape, you can look at its HTML structure using the "Inspect" tool in your browser. Here are some key indicators:
1. Static vs. Dynamic Page ๐ฟ
EASY: simple, static HTML:
<!DOCTYPE html>
<html>
<head>
<title>Sample Static Page</title>
</head>
<body>
<h1>Welcome to My Website</h1>
<p>This is a simple static webpage.</p>
<div class="products">
<div class="product">
<h2>Product 1</h2>
<p>Price: $10</p>
</div>
<div class="product">
<h2>Product 2</h2>
<p>Price: $20</p>
</div>
</div>
</body>
</html>
When you inspect this page, you will see the exact structure in the "Elements" tab, and the data (like product names and prices) will be visible directly in the HTML.
HARD: Dynamic Content (JavaScript-rendered)
On the other hand, websites with dynamic content often use JavaScript to load data asynchronously. The data might not be visible directly in the HTML source but instead gets loaded after the page has rendered. Here's an example:
<!DOCTYPE html>
<html>
<head>
<title>Sample Dynamic Page</title>
<script>
document.addEventListener("DOMContentLoaded", function() {
var productsDiv = document.querySelector('.products');
var products = [
{ name: 'Product 1', price: '$10' },
{ name: 'Product 2', price: '$20' }
];
products.forEach(function(product) {
var productDiv = document.createElement('div');
productDiv.className = 'product';
productDiv.innerHTML = '<h2>' + product.name + '</h2><p>Price: ' + product.price + '</p>';
productsDiv.appendChild(productDiv);
});
});
</script>
</head>
<body>
<h1>Welcome to My Website</h1>
<p>This is a dynamic webpage.</p>
<div class="products"></div>
</body>
</html>
When you inspect this page initially, the "products" div will be empty. The data will only appear after the JavaScript has executed and rendered the content. You might see something like this initially:
<div class="products"></div>
And after the JavaScript runs, it will look like this in the "Elements" tab:
<div class="products">
<div class="product">
<h2>Product 1</h2>
<p>Price: $10</p>
</div>
<div class="product">
<h2>Product 2</h2>
<p>Price: $20</p>
</div>
</div>
2. Consistent vs Non-consistent page layout ๐พ
EASY: Consistent sample
Page 1:
htmlCopy code<div class="product">
<h1 class="title">Product 1</h1>
<span class="price">$10</span>
</div>
Page 2:
htmlCopy code<div class="product">
<h1 class="title">Product 2</h1>
<span class="price">$20</span>
</div>
HARD: Non-Consistent Example:
Page 1:
htmlCopy code<div class="item">
<h2 class="name">Product 1</h2>
<p class="cost">$10</p>
</div>
Page 2:
htmlCopy code<section class="product-info">
<h1 class="title">Product 2</h1>
<span class="price">$20</span>
</section>
Consistent Layout: Same class names, tag types, and hierarchical structures across pages.
Non-Consistent Layout: Different class names, tag types, and hierarchical structures across pages.Clear CSS Classes or IDs
3. Clear CSS Classes VS unclear ๐ก
EASY: Clear CSS Classes or IDs
Clear CSS classes or IDs make it easy to identify and extract specific elements on a webpage. They are descriptive and specific to the content they are targeting.
Example of Clear CSS Classes/IDs
htmlCopy code<!DOCTYPE html>
<html>
<head>
<title>Product Page</title>
</head>
<body>
<div class="product">
<h1 id="product-title">Product 1</h1>
<p class="product-price">$10</p>
<div class="product-description">
<p>This is the description for Product 1.</p>
</div>
</div>
</body>
</html>
Product title:
id="product-title"
Product price:
class="product-price"
Product description:
class="product-description"
HARD: Unclear CSS Classes or IDs
Unclear CSS classes or IDs are generic and non-descriptive, making it difficult to identify the specific content they target. They may not provide any indication of the element's purpose or content.
Example of Unclear CSS Classes/IDs
htmlCopy code<!DOCTYPE html>
<html>
<head>
<title>Product Page</title>
</head>
<body>
<div class="content">
<h1 class="header">Product 1</h1>
<p class="info">$10</p>
<div class="text">
<p>This is the description for Product 1.</p>
</div>
</div>
</body>
</html>
Product title:
class="header"
Product price:
class="info"
Product description:
class="text"
3. Use AJAX or not?๐ค
HARD: Example of a Page Using AJAX
HTML (Initial Load)
<!DOCTYPE html>
<html>
<head>
<title>Product Page</title>
</head>
<body>
<h1>Product List</h1>
<div id="product-list">
<!-- Products will be loaded here by AJAX -->
</div>
<script>
document.addEventListener("DOMContentLoaded", function() {
// Simulating an AJAX call
setTimeout(function() {
var products = [
{ id: 1, name: "Product 1", price: "$10" },
{ id: 2, name: "Product 2", price: "$20" }
];
var productList = document.getElementById("product-list");
products.forEach(function(product) {
var productDiv = document.createElement("div");
productDiv.className = "product";
productDiv.innerHTML = "<h2>" + product.name + "</h2><p>" + product.price + "</p>";
productList.appendChild(productDiv);
});
}, 1000); // Simulating delay
});
</script>
</body>
</html>
When you first load this page and inspect the element, the #product-list
div will be empty. After the JavaScript executes, it will be populated with the product data.
Loaded Content (After AJAX Call)
<div id="product-list">
<div class="product">
<h2>Product 1</h2>
<p>$10</p>
</div>
<div class="product">
<h2>Product 2</h2>
<p>$20</p>
</div>
</div>
EASY: Example of a Page Without AJAX
HTML (Static Content)
<!DOCTYPE html>
<html>
<head>
<title>Product Page</title>
</head>
<body>
<h1>Product List</h1>
<div id="product-list">
<div class="product">
<h2>Product 1</h2>
<p>$10</p>
</div>
<div class="product">
<h2>Product 2</h2>
<p>$20</p>
</div>
</div>
</body>
</html>
When you inspect this page, the product data is already present in the HTML and doesn't require JavaScript to load.
Indicators of AJAX Usage
Empty Containers: When you inspect the HTML and see empty containers (like
<div id="product-list"></div>
), it might indicate that content is loaded via AJAX.JavaScript Code: Look for JavaScript code that performs data fetching, such as:
fetch()
XMLHttpRequest
$.ajax
(for jQuery)axios.get()
Network Activity: Use the browser's developer tools to monitor network activity. If you see network requests fetching data after the initial page load, it indicates AJAX usage.
Dynamic Content Loading: If the content appears on the page after a delay or after certain user actions (like scrolling or clicking a button), it might be loaded via AJAX.
4. Any Anti-bot measures? ๐คฌ
CAPTCHAs
What You See:
- CAPTCHAs are designed to distinguish between human users and automated bots. You might see images or text-based challenges that require user interaction.
Example:
htmlCopy code<!DOCTYPE html>
<html>
<head>
<title>CAPTCHA Example</title>
</head>
<body>
<h1>Verify You're Human</h1>
<form action="/verify" method="post">
<div class="captcha">
<img src="/captcha-image" alt="CAPTCHA Image">
<input type="text" name="captcha-response" placeholder="Enter the text">
</div>
<button type="submit">Submit</button>
</form>
</body>
</html>
Indicators:
Presence of
<img>
tags with CAPTCHA images.Forms asking for CAPTCHA responses (e.g.,
<input type="text" name="captcha-response">
).
IP Blocking
What You See:
- IP blocking prevents your IP address from accessing the website after a certain number of requests. This might result in HTTP 403 Forbidden or 429 Too Many Requests errors.
Example:
htmlCopy code<!DOCTYPE html>
<html>
<head>
<title>Access Denied</title>
</head>
<body>
<h1>403 Forbidden</h1>
<p>Your IP address has been blocked due to excessive requests.</p>
</body>
</html>
Indicators:
Receiving HTTP status codes 403 or 429.
Error messages indicating IP blocking or access denial.
Obfuscation
What You See:
- Obfuscation involves hiding or scrambling HTML content to make it difficult to parse. JavaScript might be used to obfuscate element attributes or content.
Example:
htmlCopy code<!DOCTYPE html>
<html>
<head>
<title>Obfuscation Example</title>
<script>
document.addEventListener("DOMContentLoaded", function() {
var hiddenContent = "UHJvZHVjdCAxIC0gJDEw"; // Base64 encoded
var decodedContent = atob(hiddenContent);
document.getElementById('product').textContent = decodedContent;
});
</script>
</head>
<body>
<h1>Products</h1>
<div id="product"></div>
</body>
</html>
Indicators:
JavaScript functions decoding or transforming data.
Encrypted or encoded data within the HTML (e.g., Base64 encoding).
Honeypots
Visual Indication:
- Hidden fields in forms that should not be filled out by users but can trap bots.
Example:
htmlCopy code<!DOCTYPE html>
<html>
<head>
<title>Form with Honeypot</title>
<style>
.hidden { display: none; }
</style>
</head>
<body>
<form action="/submit" method="post">
<input type="text" name="username" placeholder="Username">
<input type="password" name="password" placeholder="Password">
<input type="text" name="email" class="hidden" placeholder="Email"> <!-- Honeypot field -->
<button type="submit">Submit</button>
</form>
</body>
</html>
Indicators:
Hidden fields (e.g.,
<input type="text" name="email" class="hidden">
) that should not be filled out by humans.CSS classes like
display: none
orvisibility: hidden
JavaScript Challenges
Visual Indication:
JavaScript code that performs checks before loading the main content.
Redirects or delays in loading content that require JavaScript execution.
Example:
htmlCopy code<!DOCTYPE html>
<html>
<head>
<title>JavaScript Challenge</title>
<script>
document.addEventListener("DOMContentLoaded", function() {
if (someCondition()) {
window.location.href = "/main-content";
} else {
document.body.innerHTML = "<h1>Access Denied</h1>";
}
});
function someCondition() {
// Some complex JavaScript checks
return true;
}
</script>
</head>
<body>
<noscript>
<h1>JavaScript is required to view this page.</h1>
</noscript>
</body>
</html>
Indicators:
Content or redirects dependent on JavaScript execution.
Messages requiring JavaScript to be enabled.
Rate Limiting
Visual Indication:
Error messages indicating that you have exceeded the number of allowed requests.
HTTP status code 429 (Too Many Requests).
Example:
htmlCopy code<!DOCTYPE html>
<html>
<head>
<title>Rate Limiting</title>
</head>
<body>
<h1>429 Too Many Requests</h1>
<p>You have exceeded the number of allowed requests. Please try again later.</p>
</body>
</html>
Indicators:
HTTP status code 429.
Error messages mentioning request limits.
Anti-Scraping Headers
- Headers in the HTTP response that indicate anti-scraping measures, such as
X-Robots-Tag: noindex, nofollow
.
Example:
- In the HTTP response headers:
X-Robots-Tag: noindex, nofollow
.
Indicators:
- Specific headers indicating that the content should not be indexed or followed by bots.
5. Paginated Content or not?
What You See:
- Navigation elements like "Next" or numbered page links indicating data is spread across multiple pages.
Example HTML:
htmlCopy code<!DOCTYPE html>
<html>
<head>
<title>Paginated Content</title>
</head>
<body>
<div class="articles">
<article>Article 1</article>
<article>Article 2</article>
<!-- More articles -->
</div>
<div class="pagination">
<a href="?page=1">1</a>
<a href="?page=2">2</a>
<a href="?page=next">Next</a>
</div>
</body>
</html>
6. HTML Source
Easy: Content is directly in the HTML.
- Example: Static pages where all content is visible in the HTML source.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Quote Example</title>
</head>
<body>
<div class="quote">
<span class="text">"The world as we have created it is a process of our thinking. It cannot be changed without changing our thinking."</span>
<span>by <small class="author">Albert Einstein</small></span>
</div>
</body>
</html>
Hard: Content requires JavaScript execution.
- Example: Pages where content is dynamically loaded by JavaScript.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Dynamic Content Example</title>
</head>
<body>
<div id="content-placeholder"></div>
<script>
document.addEventListener('DOMContentLoaded', () => {
document.getElementById('content-placeholder').innerHTML = '<div class="quote"><span class="text">"Dynamic content loaded"</span><span>by <small class="author">John Doe</small></span></div>';
});
</script>
</body>
</html>
7. Network Tab
Easy: Few requests, mainly static content.
- Few requests, mostly for static resources like HTML, CSS, and images.
Request URL: http://example.com/
Request Method: GET
Status Code: 200 OK
Hard: Many requests, including dynamic content loading.
- Numerous requests, often including AJAX calls to load data dynamically.
Request URL: http://example.com/api/data
Request Method: GET
Status Code: 200 OK
8. Console Errors
Easy: Few to none.
- Minimal or no errors in the console.
[Log] Document loaded successfully.
Hard: Possible errors related to content loading.
- Errors indicating issues with dynamic content loading.
[Error] Failed to load resource: the server responded with a status of 404 (Not Found)
[Error] Uncaught TypeError: Cannot read property 'data' of undefined
9. Scrolling Behavior
Easy: Standard scrolling.
- All content is loaded at once, no additional requests on scroll.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Standard Scrolling Example</title>
</head>
<body>
<div class="content">
<p>Content block 1</p>
<p>Content block 2</p>
<p>Content block 3</p>
</div>
</body>
</html>
Hard: Infinite scroll or lazy loading.
- Content loads dynamically as the user scrolls.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Infinite Scroll Example</title>
<script>
document.addEventListener('scroll', () => {
if (window.innerHeight + window.scrollY >= document.body.offsetHeight) {
// Load more content dynamically
fetch('/api/more-content')
.then(response => response.text())
.then(data => {
document.body.insertAdjacentHTML('beforeend', data);
});
}
});
</script>
</head>
<body>
<div class="content">
<p>Initial content block</p>
</div>
</body>
</html>
10.URL Structure
Easy: Clean and predictable.
- URLs follow a consistent and logical pattern.
http://example.com/page/1
http://example.com/page/2
http://example.com/page/3
Hard: Dynamic or complex URL changes.
- URLs include dynamic parameters or session-based data.
http://example.com/page?session_id=abc123
http://example.com/search?query=data&page=1
11.Login Requirements
Easy: Typically none.
- Content is publicly accessible without authentication.
http://example.com/news
http://example.com/blog
Hard: Might require login.
- Requires user authentication to access content.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Login Example</title>
</head>
<body>
<form action="/login" method="post">
<label for="username">Username:</label>
<input type="text" id="username" name="username">
<label for="password">Password:</label>
<input type="password" id="password" name="password">
<button type="submit">Login</button>
</form>
</body>
</html>
12.Interactive Elements
Easy: Few.
- Minimal interactive elements like simple forms and links.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Simple Interaction Example</title>
</head>
<body>
<a href="/about">About Us</a>
<form action="/subscribe" method="post">
<label for="email">Email:</label>
<input type="email" id="email" name="email">
<button type="submit">Subscribe</button>
</form>
</body>
</html>
Hard: Numerous, relying on JavaScript.
- Complex interactive elements like modals, carousels, and dynamic forms.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Interactive Elements Example</title>
<script>
document.addEventListener('DOMContentLoaded', () => {
document.getElementById('load-more').addEventListener('click', () => {
fetch('/api/more-content')
.then(response => response.json())
.then(data => {
const container = document.getElementById('content');
data.forEach(item => {
const p = document.createElement('p');
p.textContent = item.text;
container.appendChild(p);
});
});
});
});
</script>
</head>
<body>
<div id="content">
<p>Initial content</p>
</div>
<button id="load-more">Load More</button>
</body>
</html>
13.Data Consistency
Easy: Consistently presented.
- Data follows a predictable structure across the page.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Consistent Data Example</title>
</head>
<body>
<div class="item">
<h2 class="name">Item 1</h2>
<p class="description">Description for item 1</p>
</div>
<div class="item">
<h2 class="name">Item 2</h2>
<p class="description">Description for item 2</p>
</div>
</body>
</html>
Hard: Requires interaction or varies.
- Data structure varies or requires user interaction to load.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Variable Data Example</title>
<script>
function showTab(tabId) {
document.querySelectorAll('.tab-content').forEach(tab => tab.style.display = 'none');
document.getElementById(tabId).style.display = 'block';
}
</script>
</head>
<body>
<div class="tabs">
<button onclick="showTab('tab1')">Tab 1</button>
<button onclick="showTab('tab2')">Tab 2</button>
</div>
<div id="tab1" class="tab-content">Content for Tab 1</div>
<div id="tab2" class="tab-content" style="display:none;">Content for Tab 2</div>
</body>
</html>
14.API Calls
Easy: Minimal or none.
- Content is static and doesn't require API calls.
Request URL: http://example.com/
Request Method: GET
Status Code: 200 OK
Hard: Numerous, requiring analysis.
- Content is dynamically loaded through multiple API calls.
Request URL: http://example.com/api/data
Request Method: GET
Status Code: 200 OK
15.Robots.txt
Easy: Typically allows.
- The robots.txt file allows web scraping.
User-agent: *
Disallow:
Hard: Possible restrictions.
- The robots.txt file restricts web scraping.
User-agent: *
Disallow: /private/
Conclusion
Understanding these key differences can help you determine the complexity of a web scraping task and choose the appropriate tools and techniques. Easy scraping scenarios involve straightforward HTML and predictable patterns, while hard scenarios require handling dynamic content, complex interactions, and potentially restrictive robots.txt files. Use the provided examples and code snippets to guide your scraping projects effectively.
Subscribe to my newsletter
Read articles from Anix Lynch directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
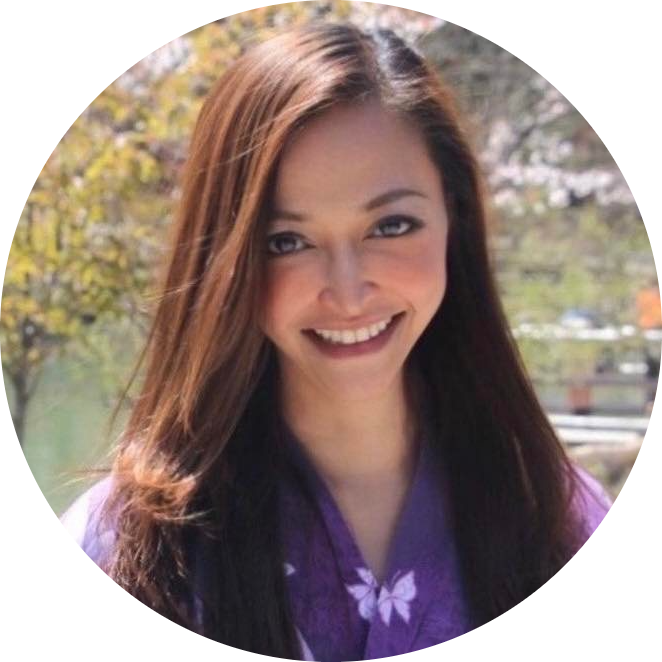