Mastering Binary Search: An Easy Guide for Beginners
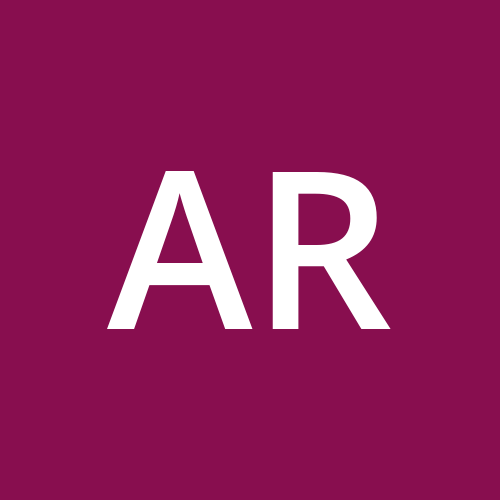
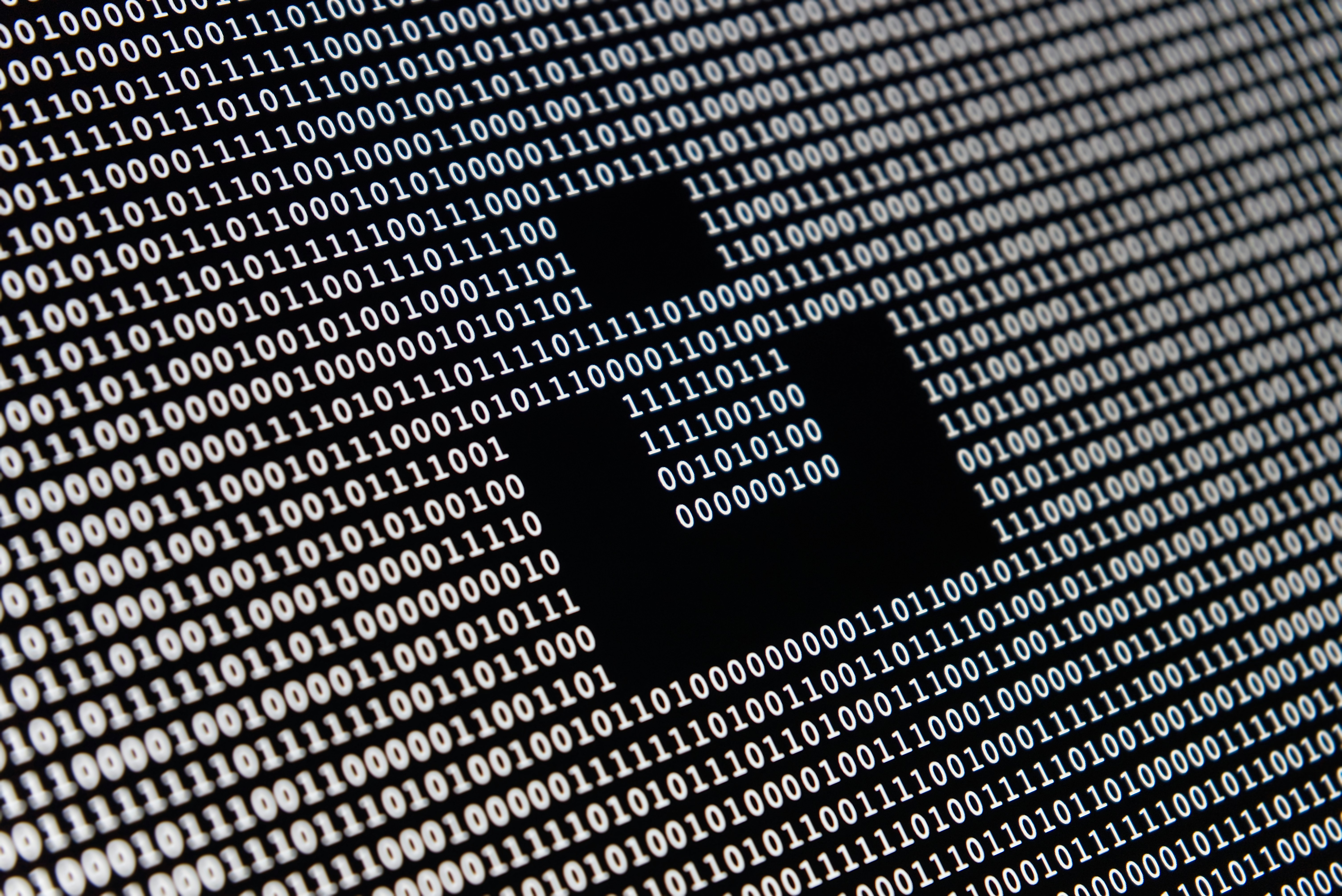
Introduction
Hey there, fellow coders! Today, we're diving into the world of searching algorithms with one of the most efficient and powerful methods: Binary Search. Searching for an item in a sorted list can be done quickly and efficiently with this algorithm. Let's break down Binary Search in a way that's easy to understand, even for beginners!
What is Binary Search?
Binary Search is a highly efficient algorithm for finding an item in a sorted list. Unlike a linear search, which checks each item one by one, binary search works by repeatedly dividing the list in half, significantly reducing the search area with each step. This method greatly reduces the number of comparisons needed, making it much faster than linear search, especially for large datasets.
How It Works?
Let's walk through the steps of Binary Search with a simple example:
Start with Two Pointers:
- Set one pointer (
low
) at the beginning of the list and the other (high
) at the end.
- Set one pointer (
Calculate the Middle:
- Find the middle index of the list:
middle = (low + high) // 2
.
- Find the middle index of the list:
Compare the Middle Element:
- Compare the middle element with the target item.
Adjust Pointers:
If the middle element is equal to the target, you've found your item.
If the middle element is less than the target, move the
low
pointer tomiddle + 1
to search the right half.If the middle element is greater than the target, move the
high
pointer tomiddle - 1
to search the left half.
Repeat:
- Continue the process until
low
is greater thanhigh
or the target is found.
- Continue the process until
Example
Let's use Binary Search to find the target 7
in the sorted array [1, 3, 5, 7, 9, 11, 13]
:
Initial pointers:
low = 0
,high = 6
.Calculate middle:
middle = (0 + 6) // 2 = 3
.Compare middle element:
array[3] = 7
, which is equal to the target7
.Target found at index
3
.
Time Complexity
Best Case: ๐(1) - When the target is found at the first middle element comparison.
Average Case: ๐(log ๐) - Due to the halving of the search area with each step.
Worst Case: ๐(log ๐) - When the target is found after several halvings.
Space Complexity
Iterative Version: ๐(1) - Requires a constant amount of additional space for pointers and the middle index.
Recursive Version: ๐(log ๐) - Due to the call stack space used by recursive calls.
Implementation in JavaScript
Here's how you can implement Binary Search in JavaScript:
const array = [1, 3, 5, 7, 9, 11, 13, 15];
function binarySearch(array, target) {
let low = 0;
let high = array.length - 1;
while (low <= high) {
const middle = Math.floor((low + high) / 2);
if (array[middle] === target) {
return middle; // Target found
} else if (array[middle] < target) {
low = middle + 1; // Search the right half
} else {
high = middle - 1; // Search the left half
}
}
return -1; // Target not found
}
// Example usage
const target = 7;
const result = binarySearch(array, target);
console.log(result); // Output: 3
Implementation in Python
And here's how you can do it in Python:
array = [1, 3, 5, 7, 9, 11, 13, 15]
def binary_search(array, target):
low = 0
high = len(array) - 1
while low <= high:
middle = (low + high) // 2
if array[middle] == target:
return middle # Target found
elif array[middle] < target:
low = middle + 1 # Search the right half
else:
high = middle - 1 # Search the left half
return -1 # Target not found
# Example usage
target = 7
result = binary_search(array, target)
print(result) # Output: 3
Use Cases
Efficient Search in Large Data Sets:
- Quickly find specific entries in large, sorted datasets, such as logs, transaction records, or customer databases.
Databases:
- Quickly locate a record in a sorted database.
Libraries:
- Search for a book in an alphabetically sorted list of book titles or authors.
Problem Solving in Competitive Programming:
- Frequently used in competitive programming and coding interviews for problems involving sorted data or requiring efficient search techniques.
E-commerce and Retail:
- Locate products quickly in a sorted list of items, such as prices, ratings, or names. Quickly find items in a sorted inventory list to check stock levels or update details.
Networking:
- Search for IP addresses in sorted routing tables to efficiently determine the best path for data packets.
Version Control Systems:
- Quickly find specific revisions or commits in a sorted list of version histories.
More About Algorithms
If you enjoyed learning about Binary Search, you might also find these articles interesting:
Understanding Merge Sort: A Simple Guide for Aspiring Coders
Cracking the Code: Understanding Quick Sort Algorithm in Simple Steps
Bubble Sort Demystified: A Beginner's Guide to Sorting Algorithms
These posts will help you further explore different sorting algorithms and improve your coding skills!
Conclusion
Binary Search is a powerful and efficient algorithm for finding an item in a sorted list. Its divide-and-conquer strategy significantly reduces the number of comparisons needed, making it much faster than a linear search. By understanding and implementing Binary Search, you can improve the performance of your search operations, especially in large datasets.
If you found this post helpful, please give it a like and share it with others who might benefit from it. Happy coding!
Subscribe to my newsletter
Read articles from Artur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
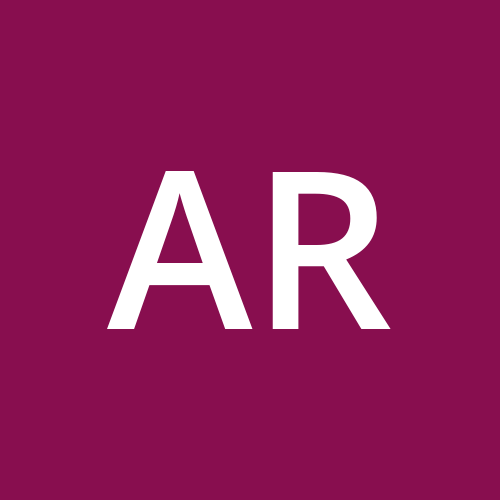