Pipes In Angular 🧨|✨
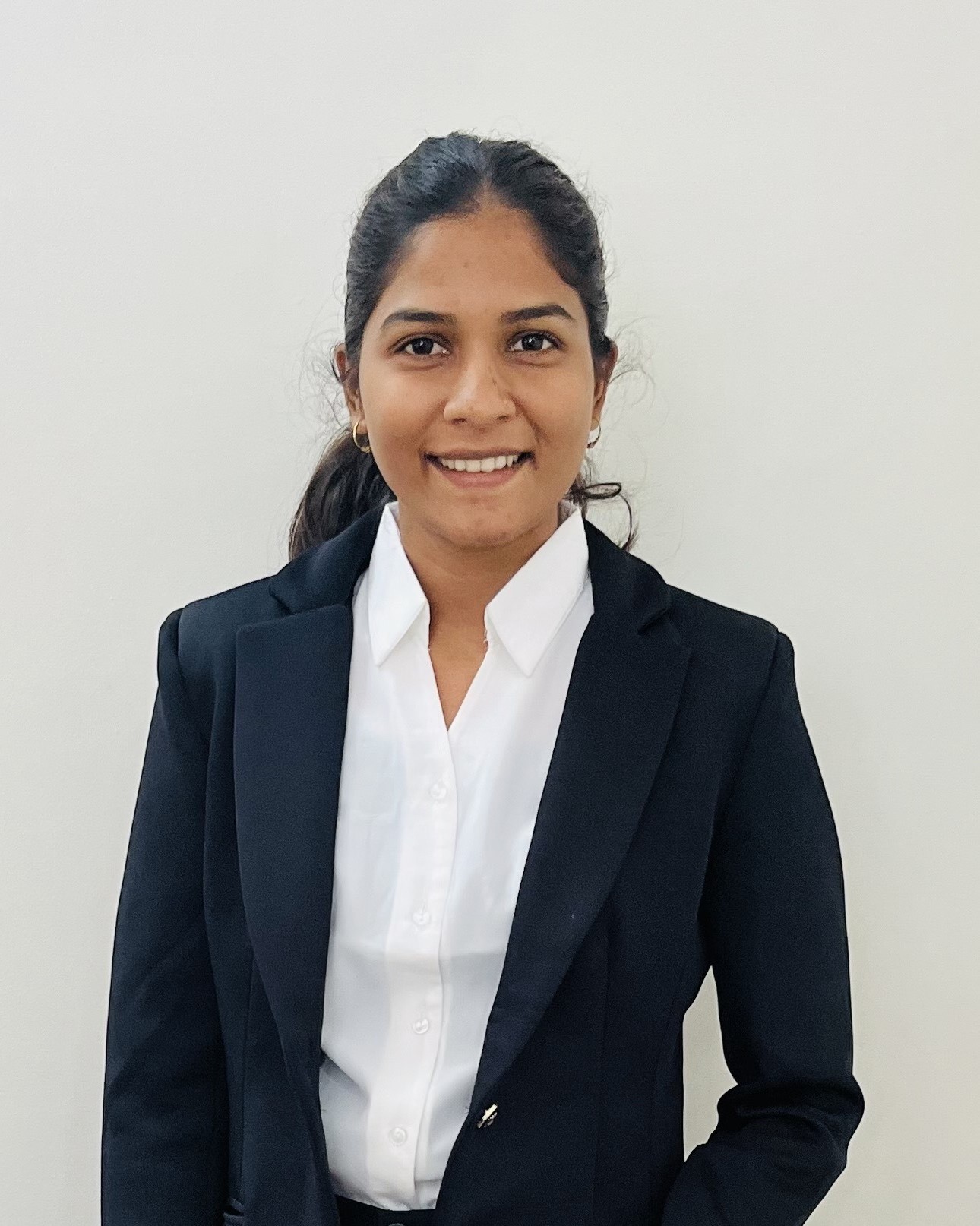
What are Angular Pipes?
Angular Pipes are a way to transform the format of output data for display. The data can be strings, currency amounts, dates, etc. Pipes are simple functions that accept an input and return a transformed value in a more technical understanding. They do not alter the data but change into the required format to display in the browser. Angular provides many built-in pipes for typical data transformation. You can also create custom pipes if you want to do custom transformation.
Built-in Pipes🎭:
Angular provides several built-in pipes that cover common use cases:
DatePipe: Formats a date value according to locale rules.
htmlCopy code{{ dateValue | date:'short' }}
UpperCasePipe: Transforms text to uppercase.
htmlCopy code{{ 'hello' | uppercase }}
LowerCasePipe: Transforms text to lowercase.
htmlCopy code{{ 'HELLO' | lowercase }}
CurrencyPipe: Formats a number as a currency.
htmlCopy code{{ amount | currency:'USD':'symbol':'1.2-2' }}
DecimalPipe: Formats a number with a specified number of decimal places.
htmlCopy code{{ 12345.6789 | number:'1.2-2' }}
PercentPipe: Formats a number as a percentage.
htmlCopy code{{ 0.85 | percent }}
JsonPipe: Converts a value into JSON string.
htmlCopy code{{ object | json }}
Custom Pipes:🎃
You can create custom pipes to handle specific transformations that are not covered by the built-in pipes.
Creating a Custom Pipe
Define the Pipe:
typescriptCopy codeimport { Pipe, PipeTransform } from '@angular/core'; @Pipe({ name: 'customPipe' }) export class CustomPipe implements PipeTransform { transform(value: any, ...args: any[]): any { // Custom transformation logic return transformedValue; } }
Register the Pipe: Add the pipe to your module's declarations.
typescriptCopy codeimport { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { AppComponent } from './app.component'; import { CustomPipe } from './custom.pipe'; @NgModule({ declarations: [ AppComponent, CustomPipe ], imports: [ BrowserModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
Use the Pipe:
htmlCopy code{{ value | customPipe:arg1:arg2 }}
Pipe Chaining:🚝
You can chain multiple pipes together to apply multiple transformations to the same data.
htmlCopy code{{ value | pipe1 | pipe2 | pipe3 }}
Angular, pipes are a way to transform data in your templates. There are two types of pipes: pure and impure.
Pure Pipes
Definition: Pure pipes are stateless, meaning they do not have side effects and do not depend on the application's state or any external variables.
Execution: Angular executes pure pipes only when the inputs to the pipe change. If the same input is provided, the pipe is not executed again.
Use Case: Ideal for situations where the input data is immutable or changes are infrequent, such as simple formatting or basic transformations.
Example:
typescriptCopy codeimport { Pipe, PipeTransform } from '@angular/core'; @Pipe({ name: 'purePipe' }) export class PurePipe implements PipeTransform { transform(value: any, ...args: any[]): any { // transformation logic return transformedValue; } }
Impure Pipes
Definition: Impure pipes can have side effects and may depend on the application's state or external variables.
Execution: Angular executes impure pipes on every change detection cycle, regardless of whether the input data changes.
Use Case: Useful for scenarios where the data is mutable or can change frequently, such as arrays, objects, or data from external sources.
Example:
typescriptCopy codeimport { Pipe, PipeTransform } from '@angular/core'; @Pipe({ name: 'impurePipe', pure: false // explicitly marks the pipe as impure }) export class ImpurePipe implements PipeTransform { transform(value: any, ...args: any[]): any { // transformation logic return transformedValue; } }
Key Differences
Change Detection: Pure pipes are called only when input values change, while impure pipes are called on every change detection cycle.
Performance: Pure pipes are more performance-efficient because they are executed less frequently. Impure pipes can impact performance due to their frequent execution.
State Management: Pure pipes are stateless and suitable for simple transformations, while impure pipes are stateful and suitable for complex scenarios where the data changes frequently.
When to Use Each
Pure Pipes: Use pure pipes for most cases where the data transformations are straightforward and the input data does not change often.
Impure Pipes: Use impure pipes when dealing with complex data structures that change frequently, such as filtering or sorting arrays, or when the transformation depends on external factors.
By choosing the appropriate type of pipe, you can ensure your Angular application remains performant and efficient.
Subscribe to my newsletter
Read articles from priyanka chaudhari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
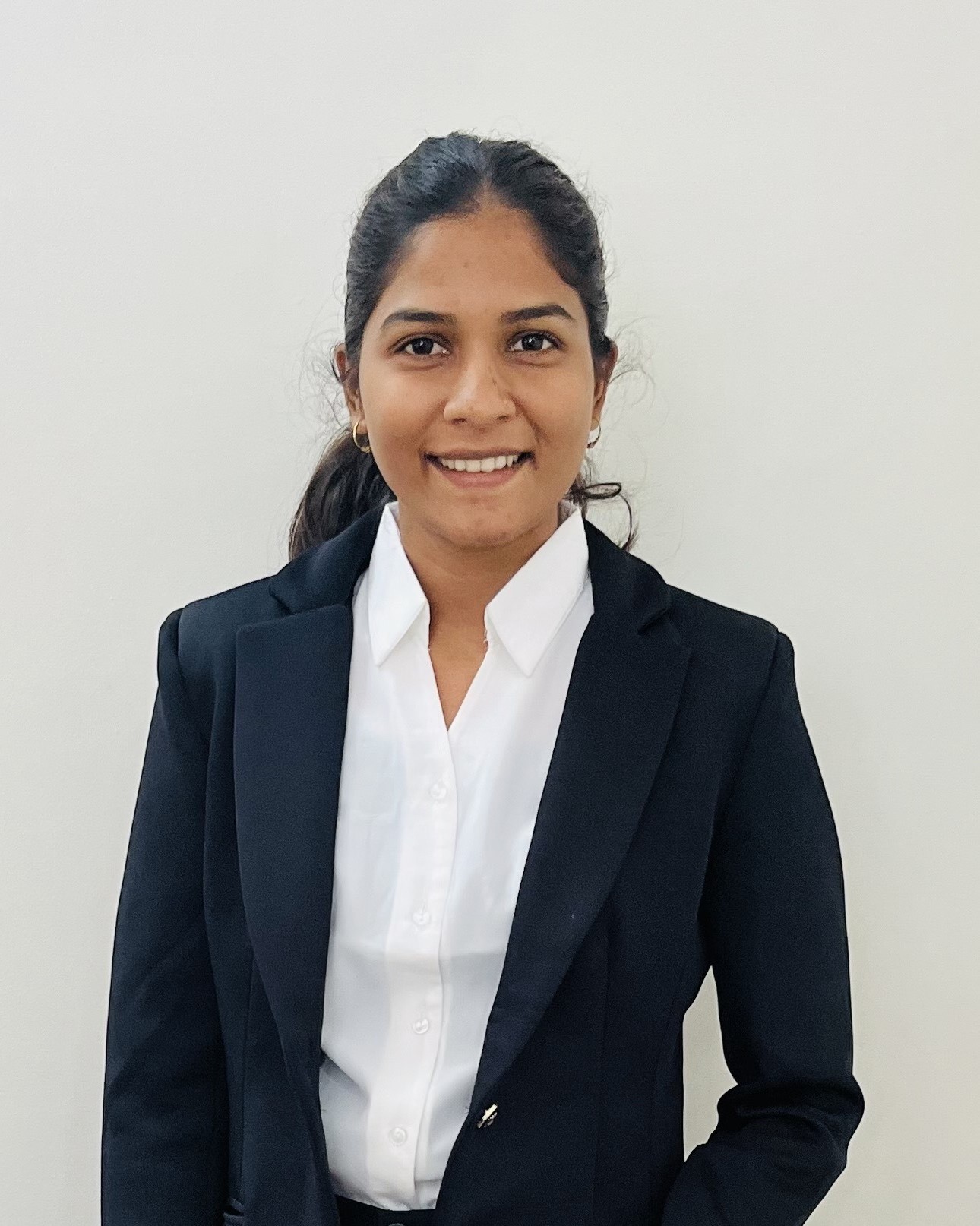
priyanka chaudhari
priyanka chaudhari
i am a full stack web developer and i write code....