A Beginner's Guide to Web Scraping with Python
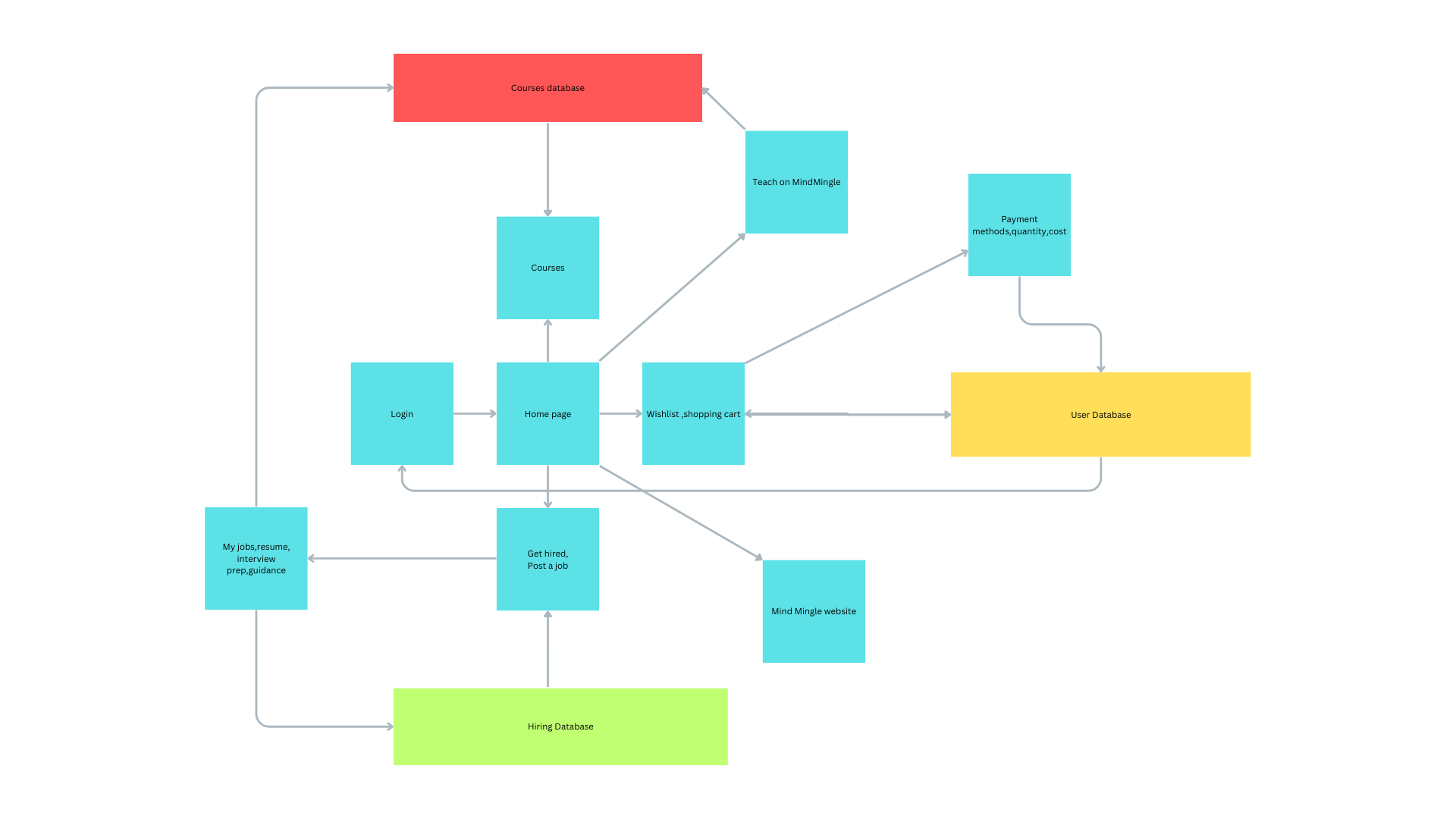
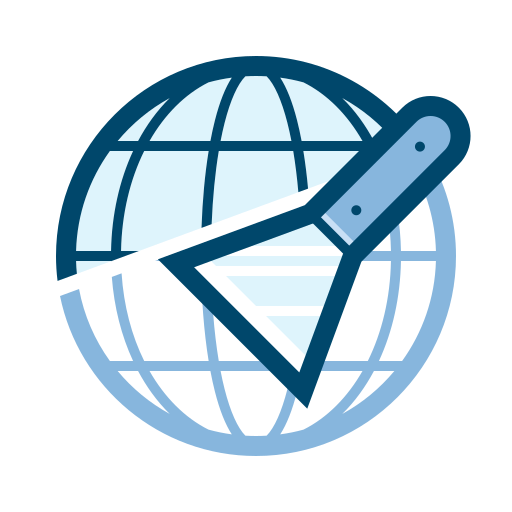
Web scraping is a powerful technique used to extract information from websites. In this article, we'll explore how to perform web scraping using Python, the requests
library, and BeautifulSoup. We'll also include a simple code snippet and its output to demonstrate the process.
What is Web Scraping?
Web scraping involves programmatically retrieving and extracting data from websites. This can be useful for various tasks such as data analysis, content aggregation, and more.
Getting Started
To start web scraping in Python, you'll need to install the requests
and BeautifulSoup
libraries. You can install them using pip:
pip install requests beautifulsoup4
Example Code
Let's write a simple Python script to scrape the title of the homepage of The New York Times.
import requests
from bs4 import BeautifulSoup
# Send a GET request to the website
req = requests.get('https://www.nytimes.com/')
# Parse the content of the request with BeautifulSoup
soup = BeautifulSoup(req.content, 'html.parser')
# Find the title of the webpage
res = soup.title
# Print the title text
print(res.getText())
Explanation
Import Libraries: We start by importing the necessary libraries,
requests
for sending HTTP requests andBeautifulSoup
for parsing HTML content.Send a Request: We send a GET request to the website URL using
requests.get()
.Parse the Content: We parse the HTML content of the webpage using BeautifulSoup.
Extract the Title: We find the title tag of the webpage using
soup.title
.Print the Title: Finally, we print the text inside the title tag.
Output
When you run the script, it will print the title of The New York Times homepage:
The New York Times - Breaking News, US News, World News and Videos
Conclusion
Web scraping is a handy tool for extracting data from websites. By using the requests
library to fetch web pages and BeautifulSoup to parse and extract the needed information, you can automate many data collection tasks. This example demonstrates a simple way to get started with web scraping in Python.
Feel free to try this code and modify it to scrape other types of data from different websites. Remember to always respect the website's robots.txt
file and terms of service when scraping data.
Happy scraping!
Subscribe to my newsletter
Read articles from Rahul Boney directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
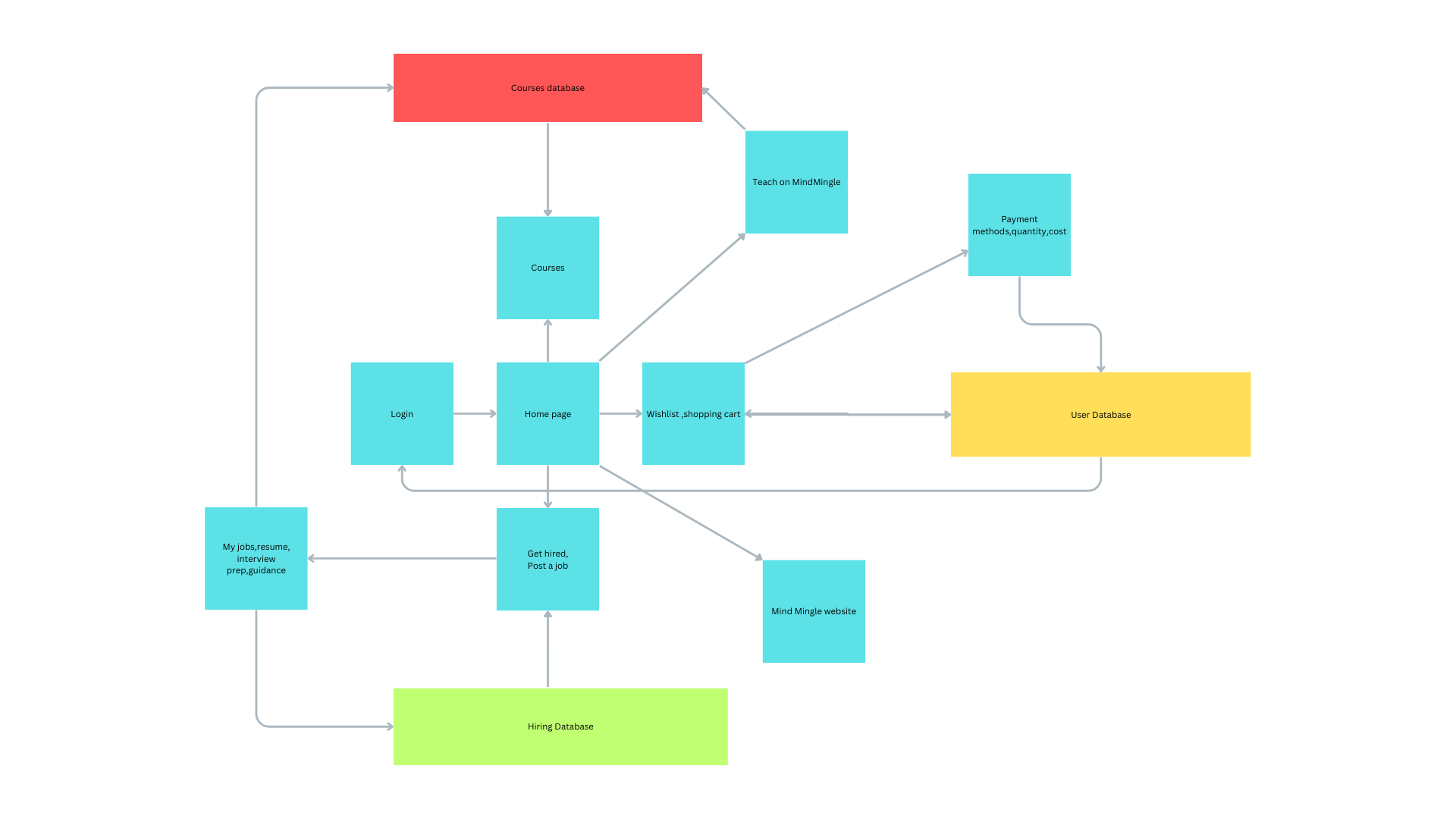
Rahul Boney
Rahul Boney
Hey, I'm Rahul Boney, really into Computer Science and Engineering. I love working on backend development, exploring machine learning, and diving into AI. I am always excited about learning and building new things.