Creating Cross-platform Applications with C# and Xamarin
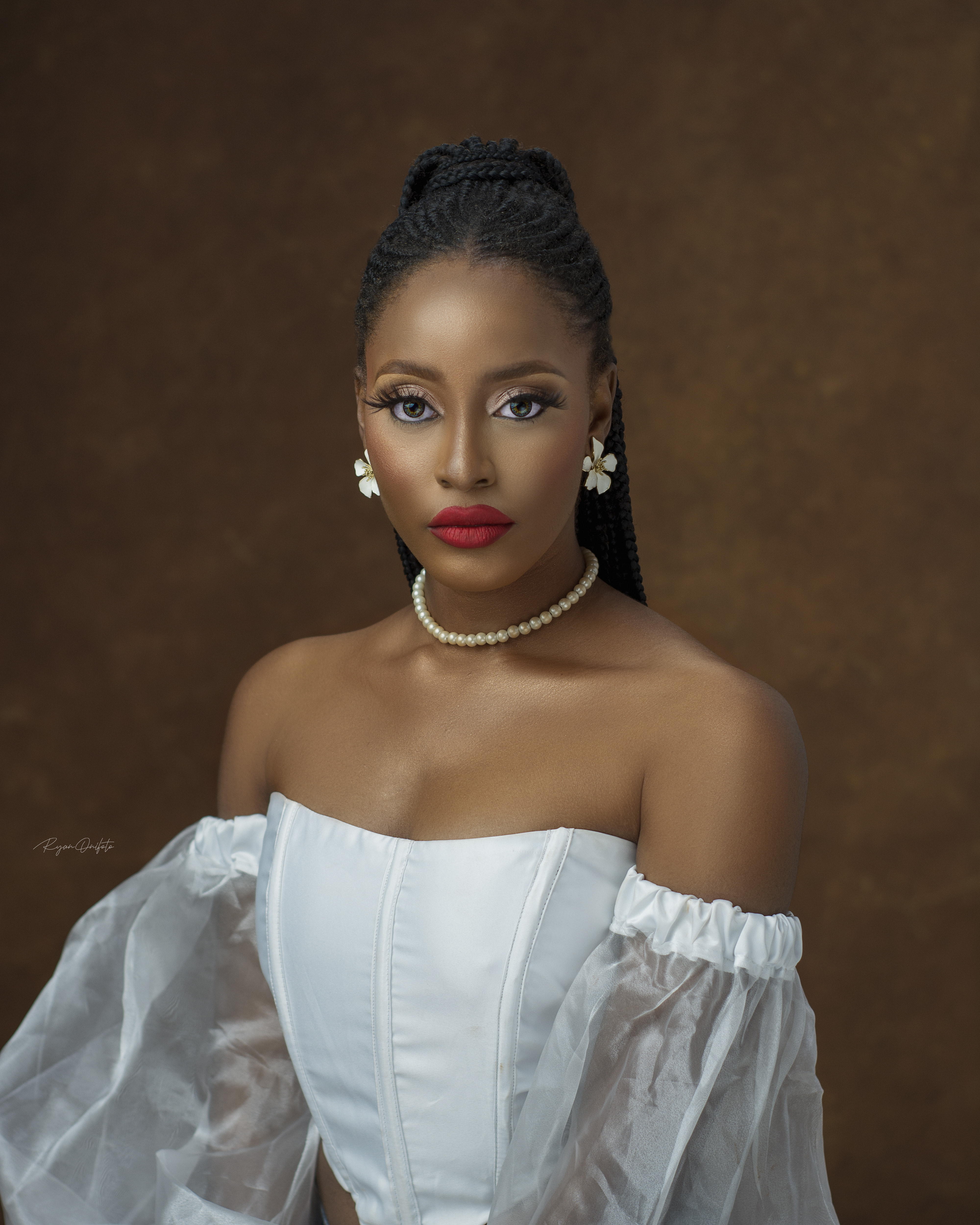
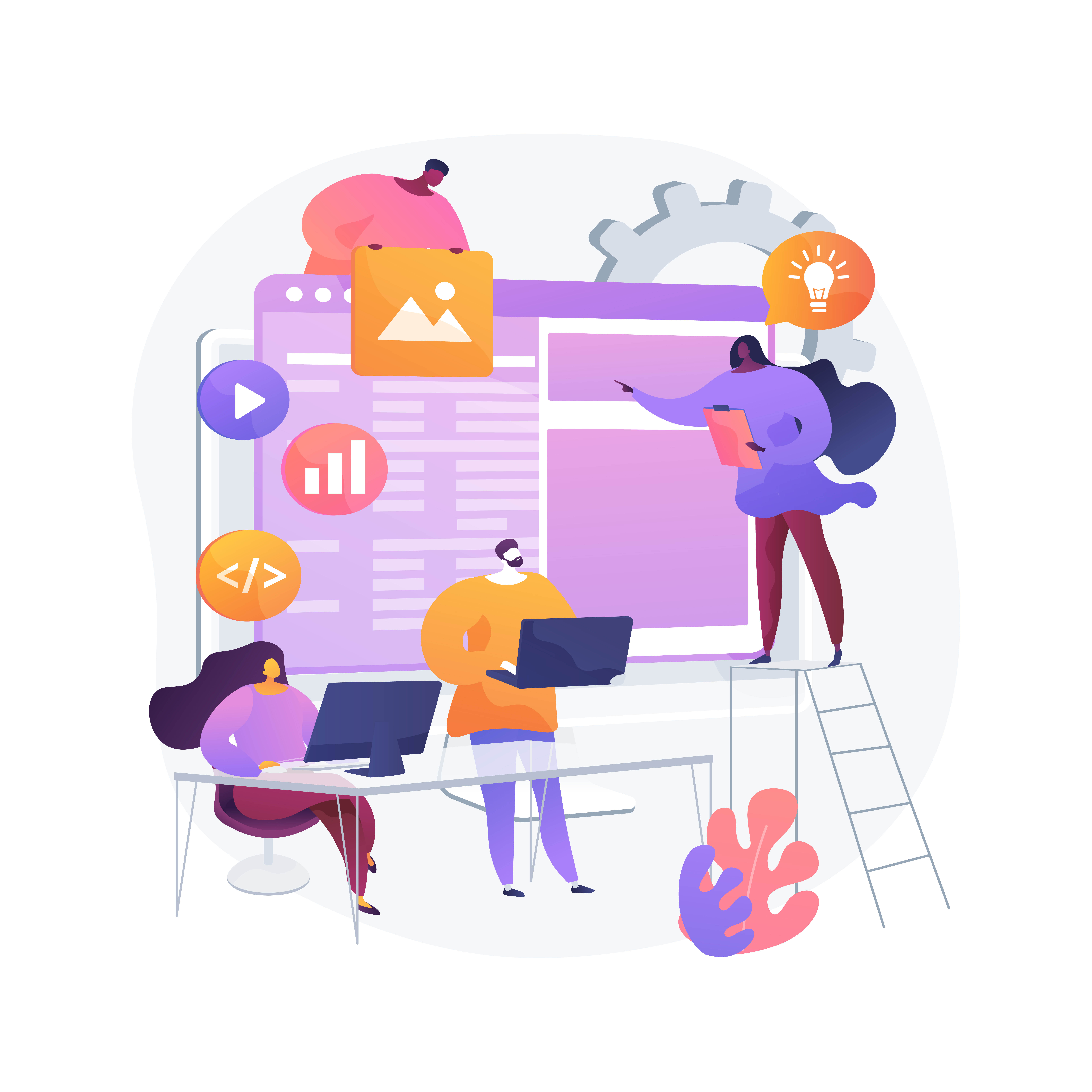
Welcome to the eighth installment of our series! Today, I will guide you through developing multi-platform apps with C# and Xamarin.
What are Multi-platform Apps?
Multi-platform apps are designed to run on different devices and operating systems without needing separate versions. This means a single app can work on smartphones, tablets, desktops, and web browsers. Xamarin, a Microsoft-owned framework, allows you to build Android and iOS apps using C#.
Why Multi-platform Apps?
Imagine you have a favorite game on your smartphone. If it's a multi-platform app, you can play the same game on your tablet or computer without needing a different version for each device. The game’s look and feel will be consistent across all devices, making it easy and familiar to use.
Let's dive into the step-by-step process of developing multi-platform apps with C# and Xamarin.
Pre-requisites
To fully benefit from this article, readers should have the following prerequisites:
Basic Knowledge of C#:
Familiarity with C# programming is essential.Understanding of Object-Oriented Programming (OOP):
Concepts like classes, objects, inheritance, and polymorphism.Visual Studio Installed:
Ensure you have Visual Studio installed, preferably the latest version with Xamarin components.Basic Understanding of Mobile App Development:
Familiarity with mobile app concepts, UI/UX design, and app lifecycle.Xamarin Account:
Sign up for a Xamarin account if you don’t have one.Development Environment Setup:
Windows: Visual Studio with Xamarin installed.
Mac: Visual Studio for Mac with Xamarin.
Access to Android and iOS Devices or Emulators:
To test your applications on real devices use simulators/emulators.
Table of Contents
Introduction to Xamarin
Setting Up Your Development Environment
Building a Simple Cross-Platform Mobile App
Sharing Code Between Android and iOS
Creating User Interfaces with Xamarin.Forms
Data Binding and MVVM Pattern
Handling Navigation in Xamarin.Forms
Accessing Device Features
Deploying and Testing Your App
Additional Resources
Introduction to Xamarin
What is Xamarin?
Xamarin is a Microsoft-owned framework that allows you to create mobile applications that run on both Android and iOS using a single codebase written in C#. This means you can write your app once and deploy it to multiple platforms, saving time and effort.
Benefits of Using Xamarin for Cross-Platform Development
Single Codebase:
Write your app once in C# and run it on both Android and iOS.Native Performance:
Xamarin allows you to create apps with native performance and user experience.Shared Logic:
Share the core logic of your app, like data models and business rules, across platforms.Access to Native APIs:
Use platform-specific APIs and features without leaving the C# environment.Large Community and Support:
Benefit from a strong community and extensive documentation provided by Microsoft.
Setting Up Your Development Environment
Before you start building your cross-platform app with Xamarin, you'll need to set up your development environment. Don't worry, I will guide you through each step.
Installing Visual Studio with Xamarin
Visual Studio is an integrated development environment (IDE) that makes coding easier. Follow these steps to install Visual Studio with Xamarin:
Download Visual Studio:
Go to the Visual Studio website.
Choose the free Community edition (it's perfect for beginners).
Install Visual Studio:
Run the installer you just downloaded.
When prompted to choose workloads, select "Mobile development with .NET". This will include Xamarin.
Finish the Installation:
- Click on "Install" and wait for Visual Studio to complete the installation process.
Setting Up Emulators and Devices for Testing
Emulators let you test your app on a virtual device on your computer. Setting up emulators and connecting physical devices is straightforward:
Android Emulator:
Open Visual Studio and go to "Tools" > "Android" > "Android Device Manager".
Click on "New" to create a new virtual device.
Choose a device profile (like Pixel 3), select a system image, and follow the prompts to finish setting it up.
Once done, you can start the emulator by clicking "Start".
iOS Simulator (Mac required):
If you're on a Mac, the iOS Simulator is included with Xcode.
Ensure you have Xcode installed from the Mac App Store.
You can launch the simulator from Visual Studio by selecting an iOS target and running your app.
Connecting Physical Devices:
For Android:
Enable Developer Options and USB Debugging on your device. Connect it to your computer via USB. Your device should appear in the device list in Visual Studio.For iOS:
Connect your device to your Mac. Trust the computer on your device, and it should appear in the device list in Visual Studio.
Building a Simple Cross-Platform Mobile App
Creating a New Xamarin Project
Open Visual Studio:
Launch Visual Studio on your computer.Create a New Project:
Go to "File" > "New" > "Project...".Select Xamarin.Forms App:
Choose "Mobile App (Xamarin.Forms)" from the project templates.Name Your Project:
Give your project a name, choose a location to save it, and click "Create".Choose a Template:
Select the "Blank" template to start with a simple project and click "Create".
Understanding the Project Structure
Solution Explorer:
On the right side of Visual Studio, you'll see the Solution Explorer. This shows all the files and folders in your project.MainProject:
This is where your shared code lives, which works for both Android and iOS.MainPage.xaml:
This is the main user interface file where you design your app's UI.MainPage.xaml.cs:
This is the code-behind file where you add the functionality for your UI.Platform-Specific Projects:
You will see separate folders for Android and iOS, which contain platform-specific code and settings.
Running Your First App
Select an Emulator or Device:
In the toolbar, choose an emulator or a connected device to run your app.Run the App:
Click the "Start" button (looks like a play button) to build and run your app.See the Result:
Your app should launch on the selected emulator or device, showing the default "Welcome to Xamarin.Forms!" message.
Code Snippets
MainPage.xaml
This file defines the layout of your app's main page.
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="YourAppNamespace.MainPage">
<StackLayout>
<!-- Add your UI components here -->
<Label Text="Welcome to Xamarin.Forms!"
HorizontalOptions="Center"
VerticalOptions="CenterAndExpand" />
</StackLayout>
</ContentPage>
MainPage.xaml.cs
This file contains the code-behind for your main page.
using System;
using Xamarin.Forms;
namespace YourAppNamespace
{
public partial class MainPage : ContentPage
{
public MainPage()
{
InitializeComponent();
}
}
}
App.xaml.cs
This file initializes your app and sets the MainPage as the startup page.
using System;
using Xamarin.Forms;
using Xamarin.Forms.Xaml;
namespace YourAppNamespace
{
public partial class App : Application
{
public App()
{
InitializeComponent();
MainPage = new MainPage();
}
protected override void OnStart()
{
}
protected override void OnSleep()
{
}
protected override void OnResume()
{
}
}
}
Android Project
For the Android-specific part of your app, Visual Studio will automatically generate the necessary files. You typically don't need to modify these unless you have platform-specific requirements.
iOS Project
Similarly, Visual Studio generates the required files for the iOS part of your app. Modifications are only needed for platform-specific requirements.
Sharing Code Between Android and iOS
When developing apps for both Android and iOS, you don’t want to write the same code twice. Here’s how you can share code efficiently:
Using Shared Projects and Portable Class Libraries (PCL):
Shared Projects:
A shared project contains code that can be used by both Android and iOS apps. You write your common code here, and it’s included in both apps.Portable Class Libraries (PCL):
PCLs are special libraries that you can use across different platforms. They help you write code that works on Android, iOS, and other platforms, without needing to change it for each one.
Writing Platform-Independent Code:
Write code that doesn’t depend on the specifics of Android or iOS. For example, use common data models or business logic that doesn’t interact with device-specific features.
Use Xamarin’s built-in tools to ensure your code works the same way on both platforms. This means your app will look and behave consistently, whether it’s on a phone or tablet.
Utilizing Dependency Service for Platform-Specific Functionality:
Sometimes you need features that are unique to a specific platform, like accessing a phone’s camera. For these cases, you use something called a Dependency Service.
A Dependency Service allows you to write platform-specific code (like for Android or iOS) and call it from your shared code. This way, you keep most of your code common but still handle special cases for each platform.
Creating User Interfaces with Xamarin.Forms
Introduction to Xamarin.Forms
Xamarin.Forms is a framework that helps you build user interfaces (UIs) that work on both Android and iOS using a single codebase. Instead of writing separate UIs for each platform, Xamarin.Forms lets you design one UI that looks good on both.
Building UI with XAML
XAML (eXtensible Application Markup Language) is a way to design your app's interface using simple markup. Think of it as writing HTML for mobile apps. You describe what you want your app to look like and Xamarin.Forms turns that into a working UI. Here’s an example of a simple XAML code to create a button:
<Button Text="Click Me" />
Working with Common Controls and Layouts
In Xamarin.Forms, you use controls and layouts to build your app’s UI. Common controls include buttons, labels, and text inputs, while layouts help you arrange these controls on the screen. For instance:
Button: A clickable element.
Label: Displays text.
Entry: A text input field.
Layouts like StackLayout
and Grid
help organize your controls. For example, StackLayout
arranges items in a vertical or horizontal stack:
<StackLayout>
<Label Text="Welcome to Xamarin.Forms!" />
<Button Text="Press me" />
</StackLayout>
By using Xamarin.Forms, you can create an app that looks and works well on both Android and iOS without needing to design separate interfaces for each.
Data Binding and MVVM Pattern
Understanding the Model-View-ViewModel (MVVM) Pattern
The MVVM pattern helps you separate different parts of your app to make it easier to manage and test. Think of it like this:
Model: This is the data of your app. For example, if your app shows a list of contacts, the model is where you store information about each contact.
View: This is what users see on their screen. It includes things like buttons, text boxes, and labels.
ViewModel: This acts as a bridge between the model and the view. It holds the logic and processes the data that the view displays.
Implementing Data Binding in Xamarin.Forms
Data binding connects your view (UI) to your ViewModel so that changes in one are automatically reflected in the other. For example, if your contact list updates, the view updates automatically without you having to write extra code.
Here’s how you can use data binding:
Set Up Your Model: Define the data structure for your app (e.g., a Contact class with properties like Name and PhoneNumber).
Create Your ViewModel: This is where you put the code to handle the data. For instance, you might have a
ContactViewModel
that manages a list of contacts.Bind Your View to the ViewModel: In your XAML file (where you define your UI), link your UI elements to the properties in your ViewModel. For example, if you have a label that should show a contact's name, you bind it to the
Name
property in your ViewModel.
Here's a quick example in Xamarin.Forms:
Model:
public class Contact { public string Name { get; set; } public string PhoneNumber { get; set; } }
ViewModel:
public class ContactViewModel : INotifyPropertyChanged { private Contact _contact; public Contact Contact { get { return _contact; } set { _contact = value; OnPropertyChanged(); } } public event PropertyChangedEventHandler PropertyChanged; protected void OnPropertyChanged([CallerMemberName] string propertyName = null) { PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName)); } }
View (XAML):
<Label Text="{Binding Contact.Name}" />
In this setup, whenever Contact.Name
in your ViewModel changes, the Label
in your view updates automatically.
By using MVVM and data binding, you make your code cleaner and easier to manage, especially as your app grows.
Handling Navigation in Xamarin.Forms
Navigation Basics:
Navigation in Xamarin.Forms allows users to move between different screens (pages) in your app. Think of it like flipping through pages in a book. There are two main ways to navigate between pages: pushing and popping.
Push: Adds a new page on top of the current page, so users can go forward in your app.
Pop: Removes the top page, taking users back to the previous page.
Using NavigationPage:
A NavigationPage
is a special kind of page that helps manage navigation in your app. It provides a navigation bar at the top with a back button and title.
Here’s how to use it:
Wrap your main page in a NavigationPage to enable navigation:
var navigationPage = new NavigationPage(new MainPage());
Push a new page when navigating forward:
await Navigation.PushAsync(new SecondPage());
Pop a page to go back:
await Navigation.PopAsync();
Using TabbedPage:
A TabbedPage
is used to create an app with multiple tabs, where each tab shows a different page. It’s like having several different pages accessible from the bottom of the screen.
Here’s how to use it:
Create a TabbedPage and add tabs:
var tabbedPage = new TabbedPage(); tabbedPage.Children.Add(new HomePage()); tabbedPage.Children.Add(new SettingsPage());
Set the TabbedPage as the main page of your app:
MainPage = tabbedPage;
With NavigationPage
and TabbedPage
, you can easily manage how users move around in your Xamarin.Forms app. Use NavigationPage
for stack-based navigation (like going deeper into a section), and TabbedPage
for quick access to different sections from the bottom of the screen.
Accessing Device Features
In this section, we will learn how to access features on a mobile device, like the camera or GPS, using Xamarin.
Using Platform-Specific APIs
Sometimes, you need to access features that are specific to the platform, such as Android or iOS. Xamarin provides a way to do this using platform-specific APIs. This means you can write code that works differently depending on whether your app is running on an Android phone or an iPhone.
Accessing the Camera
To take photos or videos with the device’s camera, you can use Xamarin’s built-in features. Here’s a simple way to do it:
Android: Use the
Intent
class to start the camera app and capture a photo.iOS: Use the
UIImagePickerController
to present the camera interface.Using GPS to Get Location
To get the device’s location, you can use the GPS. Here’s how it works:
Android and iOS: Use the
Geolocation
API in Xamarin.Forms to request the device’s current location. This allows you to get the latitude and longitude coordinates.Example: Accessing the Camera
Here’s a basic example to help you get started:
// Request permission to access the camera
await Permissions.RequestAsync<Permissions.Camera>();
// Launch the camera to take a photo
var photo = await MediaPicker.CapturePhotoAsync();
Example: Getting Location
Here’s how you can get the device’s location:
// Request permission to access location
await Permissions.RequestAsync<Permissions.LocationWhenInUse>();
// Get the current location
var location = await Geolocation.GetLastKnownLocationAsync();
Deploying and Testing Your App
Debugging and Testing Your App
Emulators:
Emulators are virtual devices on your computer that simulate real phones or tablets. You can use them to test your app without needing an actual device. To use an emulator, select it from the list in Visual Studio and run your app. This lets you see how it behaves on different screen sizes and operating systems.Physical Devices:
Testing on real devices helps you catch issues that might not show up on emulators. Connect your phone or tablet to your computer using a USB cable, and then select it as the test device in Visual Studio. This way, you can check how your app works in real-world conditions.
Preparing Your App for App Stores
Check for Errors:
Before you upload your app to an app store, make sure it’s free of errors. Test all features thoroughly to ensure they work correctly and fix any bugs you find.App Settings:
Configure important settings for your app, such as its name, version number, and icon. This information is required for app stores and helps users identify your app.Build for Release:
Create a release version of your app, which is optimized for performance and stability. In Visual Studio, you can switch to the “Release” build configuration to prepare your app for publishing.
Publishing to Google Play and Apple App Store
Google Play Store:
To publish your app on Google Play, create a developer account and follow the guidelines for app submission. Upload your APK file (the app file), add details like screenshots and descriptions, and submit it for review. Once approved, your app will be available on the Play Store.Apple App Store:
For the Apple App Store, you need an Apple Developer account. Prepare your app by following Apple’s submission guidelines, including providing screenshots and descriptions. Use Xcode or App Store Connect to upload your app and submit it for review. After Apple approves it, your app will be available on the App Store.
Additional Resources
Recommended Readings and Tutorials:
Official Xamarin Documentation:
The official guide provides detailed explanations and examples. Xamarin DocumentationBeginner's Guide to Xamarin:
A helpful book or online guide that covers the basics of Xamarin. Search for beginner-friendly resources like "Xamarin for Beginners" on platforms like Amazon or Google Books.Online Tutorials and Courses:
Websites like Udemy, Coursera, or Pluralsight offer courses on Xamarin. Look for beginner courses with good reviews.
Community Forums and Support:
Stack Overflow:
A great place to ask questions and find answers related to Xamarin development. Just search for "Xamarin" and you’ll find plenty of discussions and solutions.Xamarin Community Forums:
Join the Xamarin forums to connect with other developers and get support from the community. Xamarin ForumsReddit:
The r/xamarin subreddit is a helpful space to share your experiences, ask questions, and get advice. r/xamarin
I hope you found this guide helpful and learned something new. Stay tuned for the next article in the "Mastering C#" series: A Deep Dive into ASP.NET Core Middleware
Happy coding!
Subscribe to my newsletter
Read articles from Opaluwa Emidowo-ojo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
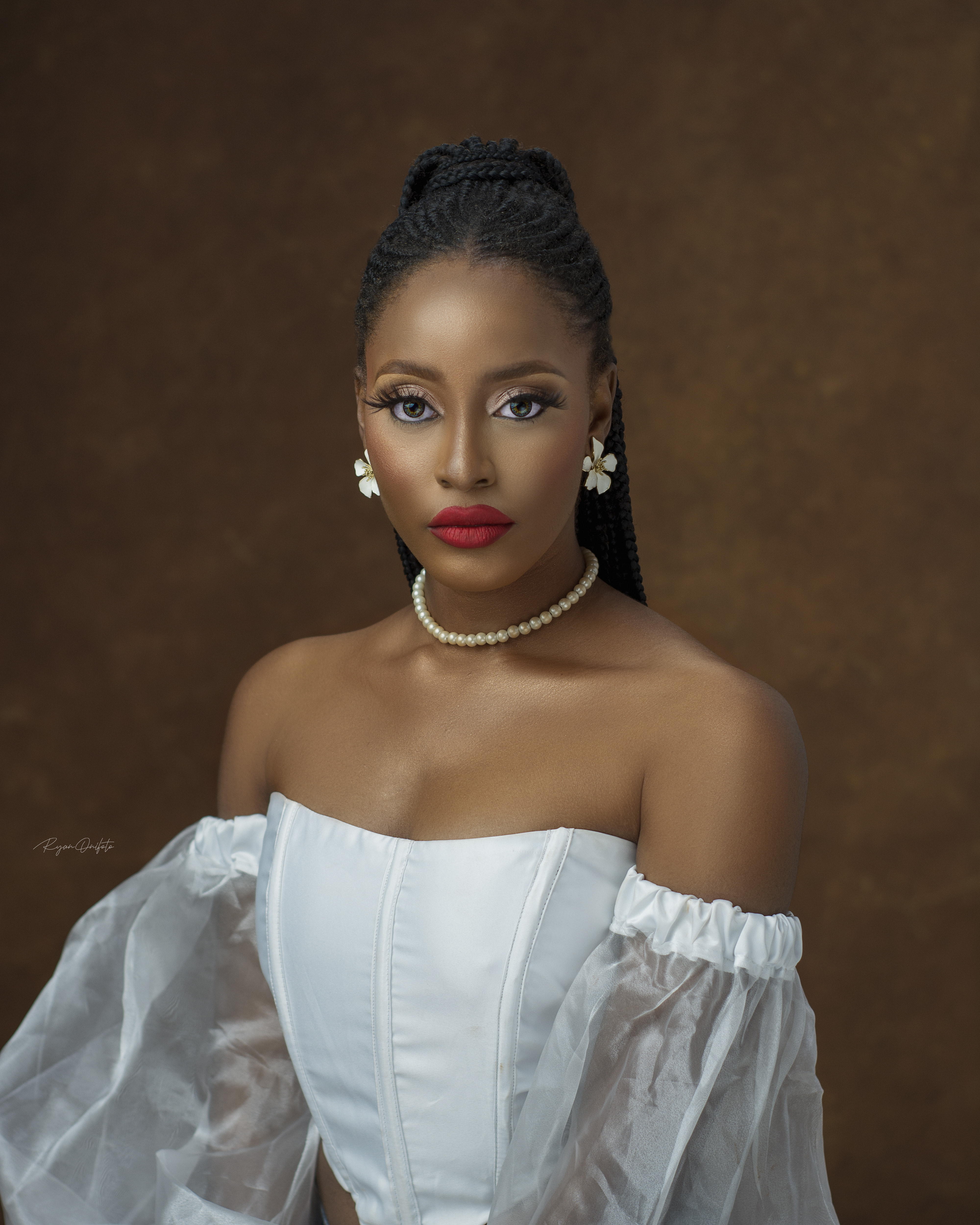