Enhancing React Performance with Code Splitting:
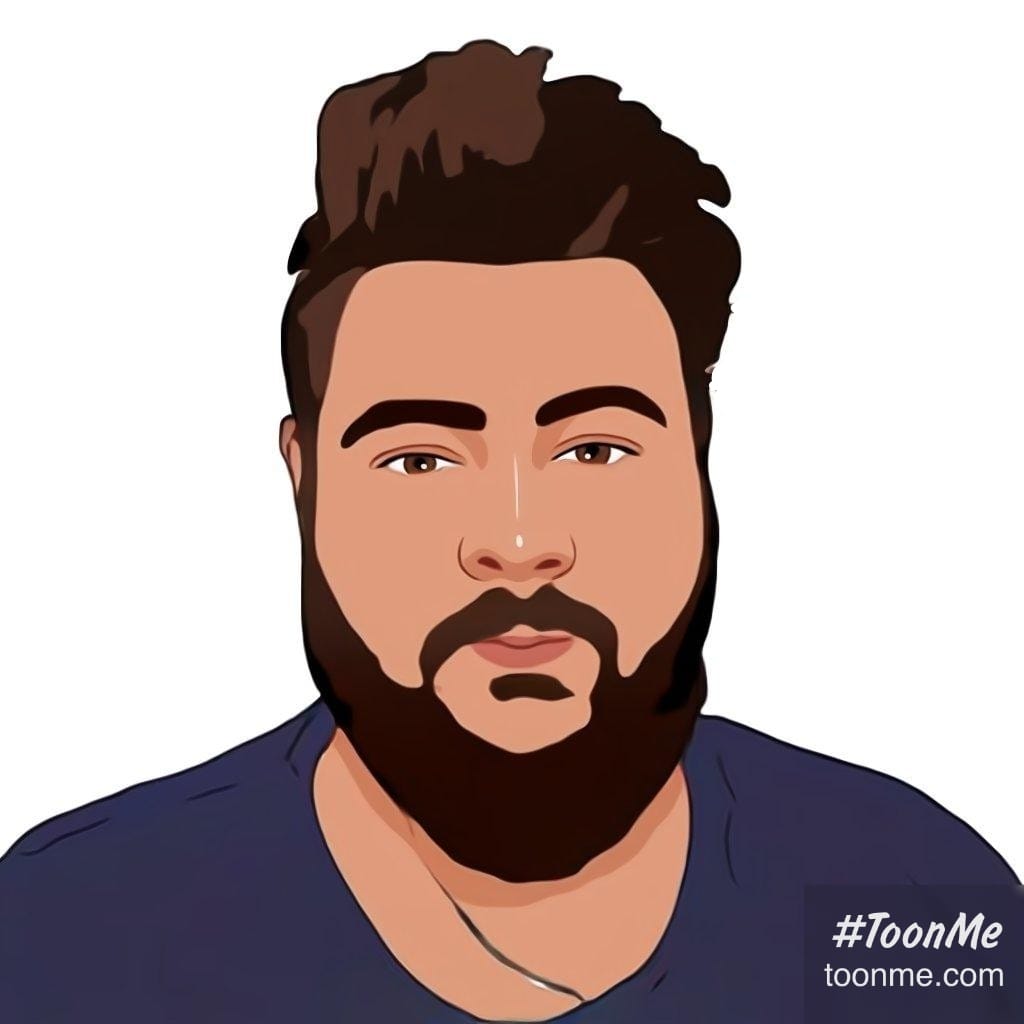

In modern web development, optimizing performance and reducing load times are crucial for creating a smooth user experience. One effective technique to achieve this in React applications is code splitting, which involves breaking down your code into smaller, manageable chunks that are loaded on demand. This article will guide you through the process of implementing code splitting using React.lazy and Suspense, using a practical example.
Understanding Code Splitting
Code splitting allows you to load only the necessary code for the initial render of your application and defer the loading of additional code until it’s needed. This can significantly reduce the initial load time, especially for large applications, and improve the overall user experience.
Using React.lazy
and Suspense
for Code Splitting
React provides a straightforward way to implement code splitting through React. Lazy and Suspense. Here’s a step-by-step process to transform a basic React application to leverage these features:
Step 1: Initial Application Setup
Consider the following initial setup of a React component:
import { useReducer } from "react";
import Admin from "./Admin";
import Calculation from "./Calculation";
function App() {
const initialState = {
showMsg: false,
showAdmin: false,
showCal: false
};
const reducer = (state, action) => {
switch (action.type) {
case "SETMSG":
return { ...state, showMsg: !state.showMsg };
case "SETADMIN":
return { ...state, showAdmin: !state.showAdmin };
case "SETCAL":
return { ...state, showCal: !state.showCal };
default:
return state;
}
};
const [{ showMsg, showAdmin, showCal }, dispatch] = useReducer(reducer, initialState);
return (
<>
<h1>Welcome to React Lazy Loading</h1>
{showMsg && <p>It's time to start! Dream it, wish it, do it.</p>}
<button onClick={() => dispatch({ type: "SETMSG" })}>View Message</button>
<button onClick={() => dispatch({ type: "SETADMIN" })}>Toggle Admin</button>
<button onClick={() => dispatch({ type: "SETCAL" })}>Calculate</button>
{showAdmin && <Admin />}
{showCal && <Calculation />}
</>
);
}
export default App;
In this setup, all components (Admin and Calculation) are imported and loaded with the initial render, regardless of whether they are needed immediately. This can lead to unnecessary loading times and wasted resources.
Step 2: Implementing Code Splitting with React.lazy
To implement code splitting, replace the direct imports with React.lazy and wrap the lazy-loaded components with Suspense to handle the loading state.
import { useReducer, lazy, Suspense } from "react";
const Admin = lazy(() => import("./Admin"));
const Calculation = lazy(() => import("./Calculation"));
function App() {
const initialState = {
showMsg: false,
showAdmin: false,
showCal: false
};
const reducer = (state, action) => {
switch (action.type) {
case "SETMSG":
return { ...state, showMsg: !state.showMsg };
case "SETADMIN":
return { ...state, showAdmin: !state.showAdmin };
case "SETCAL":
return { ...state, showCal: !state.showCal };
default:
return state;
}
};
const [{ showMsg, showAdmin, showCal }, dispatch] = useReducer(reducer, initialState);
return (
<>
<h1>Welcome to React Lazy Loading</h1>
{showMsg && <p>It's time to start! Dream it, wish it, do it.</p>}
<button onClick={() => dispatch({ type: "SETMSG" })}>View Message</button>
<button onClick={() => dispatch({ type: "SETADMIN" })}>Toggle Admin</button>
<button onClick={() => dispatch({ type: "SETCAL" })}>Calculate</button>
<Suspense fallback={<div>Loading...</div>}>
{showAdmin && <Admin />}
{showCal && <Calculation />}
</Suspense>
</>
);
}
export default App;
Key Changes:
Lazy Loading Components:
const Admin = lazy(() => import(“./Admin”));
const Calculation = lazy(() => import(“./Calculation”));
These lines delay the loading of Admin and Calculation components until they are needed.
Suspense Component:
The Suspense component is used to wrap the lazy-loaded components and provide a fallback UI (<div>Loading…</div>) while they are being loaded.
Conclusion
By implementing code splitting with React.lazy and Suspense, you can optimize the performance of your React application. This technique ensures that your users only download the code they need, leading to faster load times and a better overall experience.
Happy coding!
Subscribe to my newsletter
Read articles from Random Dude directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
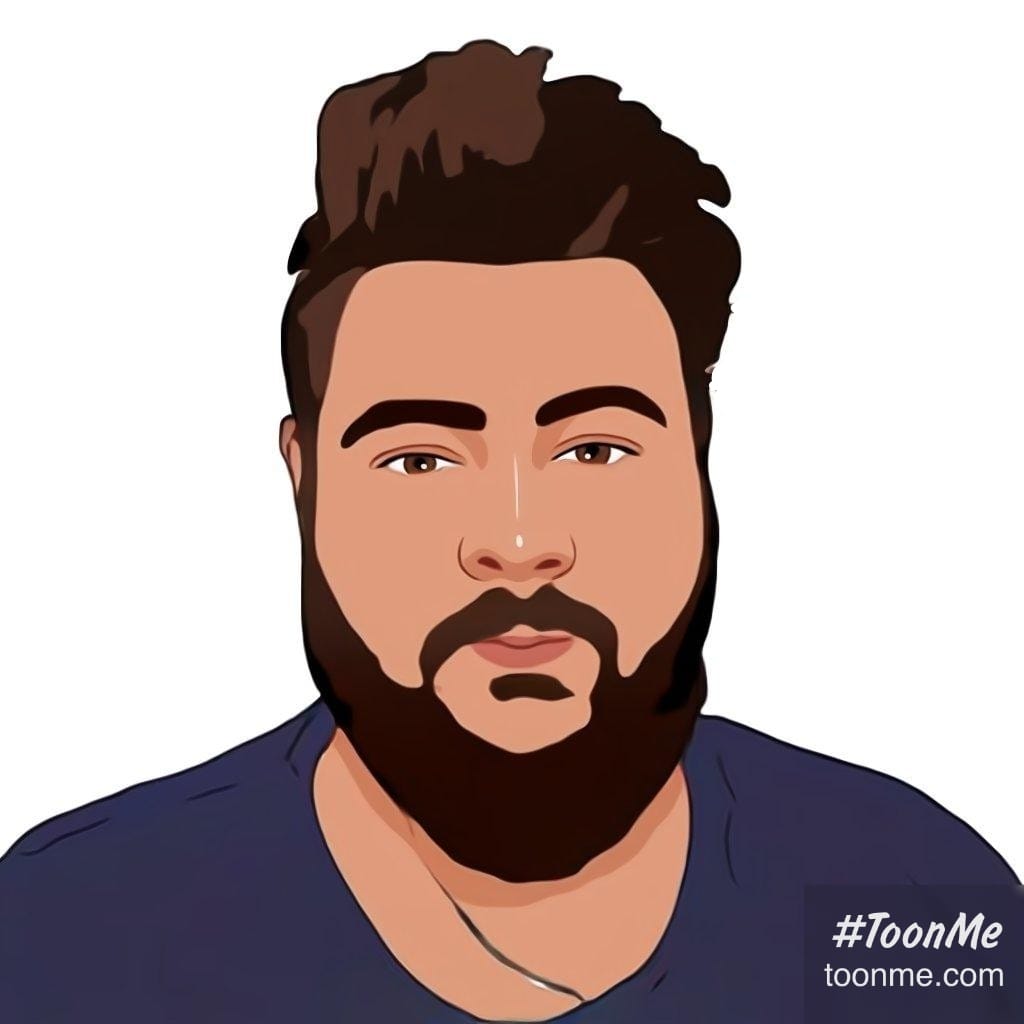
Random Dude
Random Dude
Passionate Software Engineer with 4 years of experience in developing web applications and backend systems using MERN stack. Skilled at writing clear, concise code that is easy to maintain and troubleshoot. Experienced in working with both small and large teams across multiple projects and companies. Able to work independently of remote locations or in office environments as needed by the company.