How to implement OAuth 2 in Bubble?

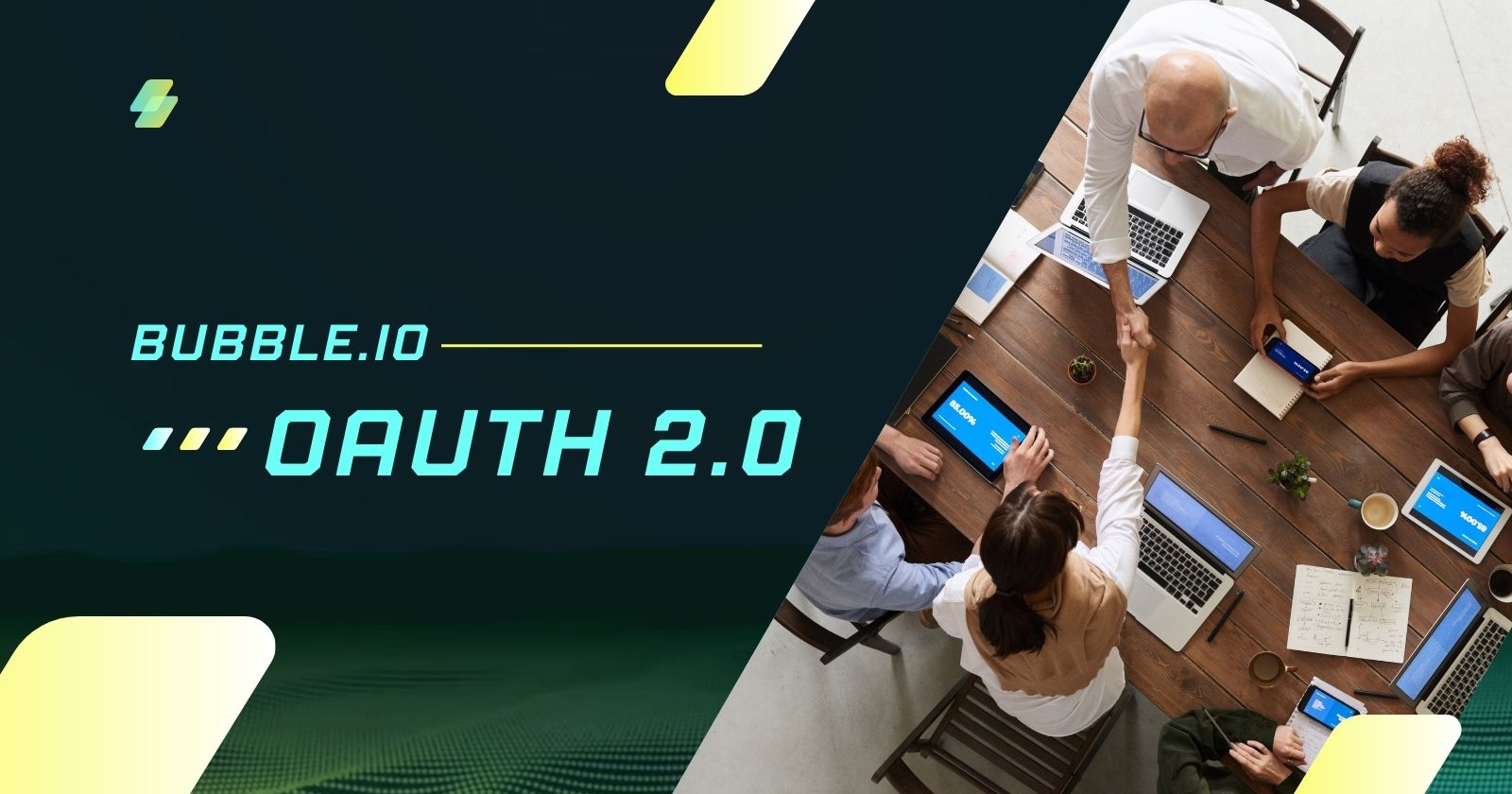
What is OAuth 2?
OAuth 2 is a way for apps to get permission to access your information without needing your password. It uses tokens, which are like temporary keys, to give specific access to your data. Imagine a valet key for your car that only allows driving, not accessing the trunk. This keeps your main password safe and only grants limited access. It's widely used by services like Google, LinkedIn, Salesforce, Zoho, and Facebook to let other apps connect securely.
How to start? With basic setup
For this, let's take an example of Salesforce CRM. Why? because there is already too much content for Google and Facebook. lol :) and on a serious note, the Concept of OAuth 2 is the same for all.
Create an account on the Salesforce website, verify your email address, and fill in all basic information. Open Salesforce OAuth API Documentation in another tab.
Now Go to Settings -> Open Advance Setup -> Home -> APPs -> APP Manager -> New Connected APP
Then set up your new application like I have mentioned below:
Now Here is some concept clarification:
How does this OAuth 2 flow work?
The bubble app will request an authorization code
Salesforce will either accept or deny the request and redirect the user to redirect URI
If Accepted, the redirect URI will have an authorization code as a query parameter
Based on the authorization code, the bubble app will ask for a token exchange
In exchange for the authorization code, the bubble app will receive an access token and a refresh token
Based on the access token, the bubble app will ask for details authorized in the API Scope
the access token will expire
bubble app will ask for details next time and if the access token is expired, bubble app will use a refresh token to get a new access token and ask for details again via a new access token
Let's make ourselves familiar with the basic definitions of keywords we are going to use.
What is redirect URI?
In OAuth 2, a redirect URI (Uniform Resource Identifier) is the URL to which the authorization server sends the user back after they have granted or denied permission to the application. It acts as a callback URL where the authorization code or access token is sent. This URI must be pre-registered with the authorization server to ensure the response is sent to a trusted destination, enhancing security. It's like a return address on a letter, telling the server where to send the response. This address must be registered in advance to ensure it’s safe and trusted.
What is an authorization code?
An authorization code in OAuth 2 is a short-lived code that an app receives after a user grants permission. The app exchanges this code for an access token, which it uses to access the user's data. This process keeps the user's credentials secure because the app never sees the user's password directly.
What is an access token?
An access token in OAuth 2 is a security credential that allows an app to access a user's data from another service. After the user grants permission, the app receives this token and uses it to make authorized requests. It's temporary and limited to specific actions, ensuring secure and controlled access to the user's information. The app uses this key to do specific things, like read your emails or access your photos, without needing your password. It's temporary and only works for the actions you allow via scope.
What is a refresh token?
A refresh token in OAuth 2 is a special token that allows an app to obtain a new access token after the current one expires. This helps the app maintain access to the user's data without needing them to log in again. It's used to ensure continuous and secure access over a longer period.
What is the scope in OAuth 2?
In OAuth 2, a scope is a parameter that specifies the level of access the app is requesting from the user. It defines what actions the app can perform and what data it can access. For example, a scope might allow an app to read your emails but not send them. This helps users understand and control what permissions they are granting to the app.
What is client ID in OAuth 2?
In OAuth 2, a client ID is a unique identifier assigned to an app when it registers with an authorization server. It's used to identify the app requesting the OAuth flow. Think of it like a username for the app, which helps the authorization server know which app is requesting access to user data.
What is client secret in OAuth 2?
In OAuth 2, a client secret is a confidential key assigned to an app when it registers with an authorization server. It's used along with the client ID to authenticate the app and ensure that the request is coming from a trusted source. Think of it like a password for the app, helping to keep the communication secure.
You can find the client ID and secret in the manage connected app section of settings shown below
What does content type as application/x-www-form-urlencoded mean?
The content type application/x-www-form-urlencoded
indicates that data sent in an HTTP request is encoded as key-value pairs, separated by &
and with each key and value separated by =
. This format is commonly used when submitting form data via HTTP POST requests. For example, name=John&age=30
is a typical representation of this content type.
How to Setup up OAuth API calls in Bubble APP?
Step 1: Request authorization code
Here is the API doc link for this.
In the bubble app, Create a button from which you need to initiate authorization and create the action 'Open an external website'. The destination will be similar to that shown in the image below
Now 'MyDomainName' is the same as your salesforce account subdomain which you can see in the URL when you open your your salesforce account.
When the user clicks on this button, the bubble app will redirect you to this URL and it will ask you to log into a Salesforce account then it will redirect to redirect URI you had set up and added in the button link.
This URL will have a query parameter named 'code'. For me, it was like this: https://unicotasky.bubbleapps.io/version-test/salesforce?code=aPrxI5eherH6PXZaIC0jwHd4esNOZqUHE_ub76CHD.JJj5W5iU54fhOCG87qYsSA3d8e65UtOA%3D%3D
Step 2: Request access token
Now we have to set up the request access token. Here is the Salesforce API Doc
The header will have the content type as application/x-www-form-urlencoded
Body parameters will have grant_type(static), code(dynamic), client_id (static), client_secret(static) and redirect_url(static).
In the Bubble API connector, it will look like this
Now put the code here and initialize the call, then save the response.
Make sure to convert the code as URL Parameter to text.
This means code=aPrxI5eherH6PXZaIC0jwHd4ehTG6AIWy5hT6F7Wa10T5A.btFkMQdrYdJ2j.pA714_oiXiw6g%3D%3D
is code=aPrxI5eherH6PXZaIC0jwHd4ehTG6AIWy5hT6F7Wa10T5A.btFkMQdrYdJ2j.pA714_oiXiw6g==
when you are using this in the body of an API call.
Successfully initialized call will look like this
Once initialized, Create a workflow on your redirect URL Page which you can execute either on 'Page load' or 'Do when condition is true'
Then I save the response to this action into the database in which I need access, token, refresh token, and expired by. Make sure to protect this data with privacy rules!
Step 3: Get Data before the access token expires
Here is the Salesforce API Doc reference.
Now Suppose you want to get data on accounts from the salesforce account of your user. You have an access token in DB that is not expired.
Here is the endpoint you need to use for this:
In Bubble, the API Setup will be like this:
and When successfully initialised call will look like this
Now I am creating a button which will only show If I have completed Step 2 successfully. As you can see, the data is empty, I haven't fetched it yet.
Now I am checking whether the token is expired or not.
When it is not expired, I will fetch the data from salesforce and show that in Repeating group.
Vola! Data from salesforce is visible now:
Step 4: Get Data after the access token expires
When you click the button and that time is greater than expire time, it means that access token is expired and we need new access token. So we will use refresh token to get it. Here is what the workflow will look like
This is how OAuth 2 works for all the platforms. There might be minor changes so always refer to API documentation to understand the nitty-gritty of their APIs.
Hope this will help you learn more about OAuth 2 and APIs in Bubble.
Help me!
If you enjoyed this post and found it helpful, Kindly consider supporting my work by buying me a coffee! Your support helps me create more valuable content and continue sharing useful resources. Thank you!
These API calls are not secure. Click here to find out how to secure them.
Subscribe to my newsletter
Read articles from Anish Gandhi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anish Gandhi
Anish Gandhi
✔️ Certified Bubble.io Developer with 2+ Years of experience in creating scalable responsive web applications. ✔️ Top Rated Plus Upwork Freelancer ✔️ Canvas framework expert