How to Create a Professional Resume Layout in React Using Tailwind CSS

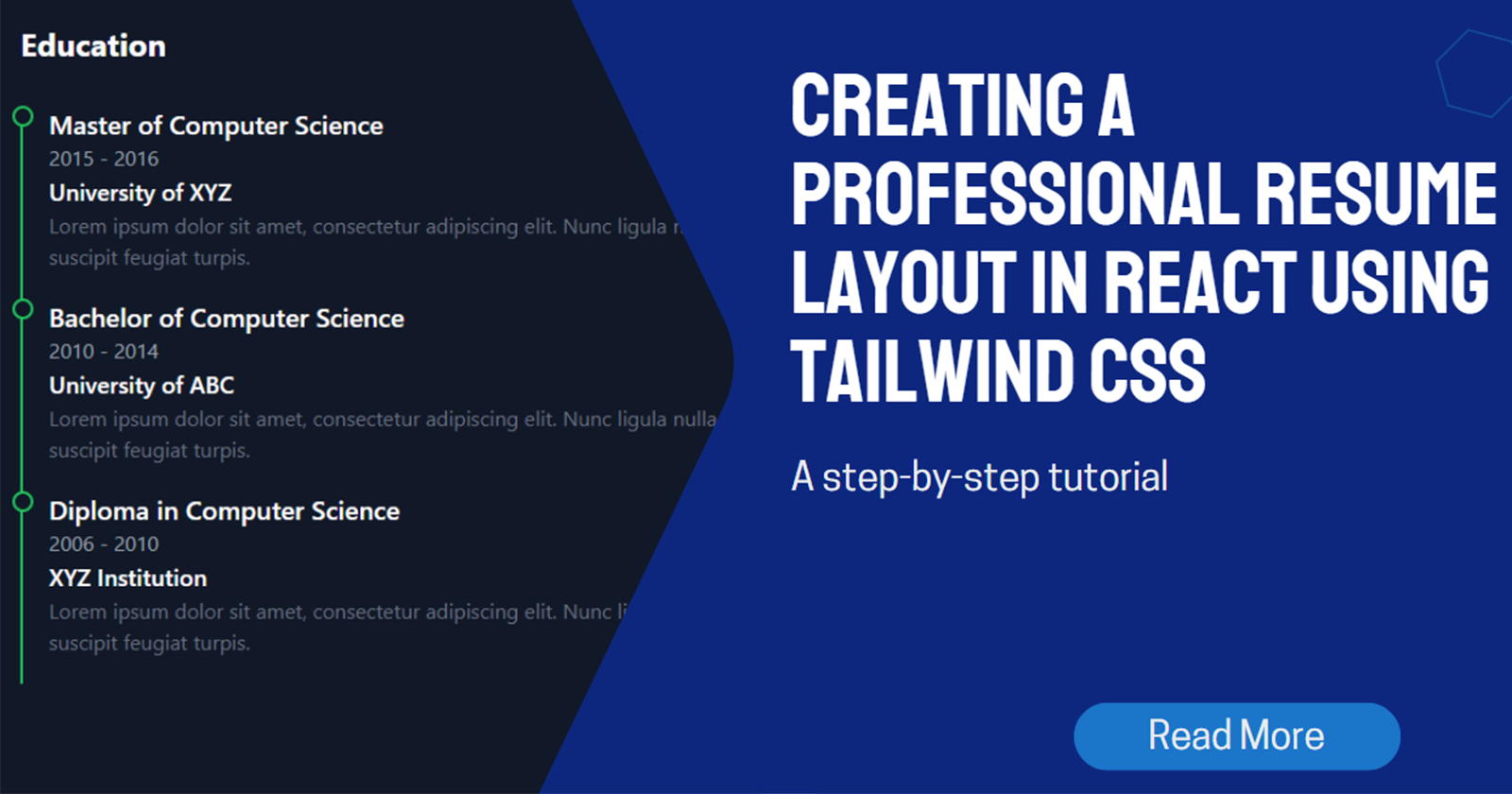
Many a times, you might have come across the resume layout style mostly used in web portfolios. You might be wondering, how can I achieve this using TailwindCSS. This article will walk you through the process, step-by-step.
Step 1: Setup Your Project
First, ensure you have a React project set up. If you haven't already, create a new React project and install Tailwind CSS.
npm create vite@latest my-resume -- --template react
cd my-resume
npm install -D tailwindcss postcss autoprefixer
npx tailwindcss init -p
Configure Tailwind by adding the content section into to your tailwind.config.js
file:
/** @type {import('tailwindcss').Config} */
export default {
content: [
"./index.html",
"./src/**/*.{js,ts,jsx,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
Add the Tailwind directives to your CSS file (src/index.css
):
@tailwind base;
@tailwind components;
@tailwind utilities;
Step 2: Create the Resume Component
Create a new file named Resume.jsx
and add the following JSX code:
We will then define 2 arrays, one having education data, another one having experience data.
Then we will map through these 2 arrays to achieve this in a more efficient way.
//education data array
const educationData = [
{
degree: 'Master of Computer Science',
duration: '2015 - 2016',
institution: 'University of XYZ',
description: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nunc ligula nulla, tincidunt id faucibus sed, suscipit feugiat turpis.',
},
{
degree: 'Bachelor of Computer Science',
duration: '2010 - 2014',
institution: 'University of ABC',
description: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nunc ligula nulla, tincidunt id faucibus sed, suscipit feugiat turpis.',
},
{
degree: 'Diploma in Computer Science',
duration: '2006 - 2010',
institution: 'XYZ Institution',
description: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nunc ligula nulla, tincidunt id faucibus sed, suscipit feugiat turpis.',
},
//add more items as needed
];
//experience data array
const experienceData = [
{
position: 'Senior UX/UI Designer',
duration: 'Jan 2020 - Present',
company: 'Bergnaum, Hills and Howe',
description: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nunc ligula nulla, tincidunt id faucibus sed, suscipit feugiat turpis.',
},
{
position: 'Product Designer',
duration: 'Jan 2016 - December 2019',
company: 'Langosh, Sipes and Raynor',
description: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nunc ligula nulla, tincidunt id faucibus sed, suscipit feugiat turpis.',
},
{
position: 'UI/UX Designer',
duration: 'Jan 2014 - December 2015',
company: 'Strosin, Maggio and Homenick',
description: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nunc ligula nulla, tincidunt id faucibus sed, suscipit feugiat turpis.',
},
//add more items as needed
];
const Resume = () => {
return (
<div className="bg-gray-900 text-white font-sans min-h-fit">
<div className="container mx-auto py-10 px-5">
<h1 className="text-center text-3xl font-bold mb-8">RESUME</h1>
<div className="grid grid-cols-1 md:grid-cols-2 gap-10 relative">
{/* Education Section */}
<div className="relative">
<h2 className="text-2xl font-bold mb-8">Education</h2>
{educationData.map((education, index) => (
<div key={index} className="mb-0 pb-5 border-l-2 border-green-500 pl-5 relative">
<div className="absolute left-0 -ml-2 w-4 h-4 bg-gray-900 border-2 border-green-500 rounded-full"></div>
<h3 className="text-xl font-semibold">{education.degree}</h3>
<p className="text-gray-400">{education.duration}</p>
<p className="text-lg font-semibold">{education.institution}</p>
<p className="text-gray-500">{education.description}</p>
</div>
))}
</div>
{/* Experience Section */}
<div className="relative">
<h2 className="text-2xl font-bold mb-8">Experience</h2>
{experienceData.map((experience, index) => (
<div key={index} className="mb-0 pb-5 border-l-2 border-green-500 pl-5 relative">
<div className="absolute left-0 -ml-2 w-4 h-4 bg-gray-900 border-2 border-green-500 rounded-full"></div>
<h3 className="text-xl font-semibold">{experience.position}</h3>
<p className="text-gray-400">{experience.duration}</p>
<p className="text-lg font-semibold">{experience.company}</p>
<p className="text-gray-500">{experience.description}</p>
</div>
))}
</div>
</div>
</div>
</div>
);
};
export default Resume;
Step 3: Add Tailwind CSS Classes
To add the vertical lines and dots:
Use
border-l-2 border-yellow-500
for the vertical lines.Use pseudo-elements (
div
withabsolute
positioning) for the dots:absolute left-0 -ml-2 w-4 h-4 bg-gray-900 border-2 border-yellow-500 rounded-full
Step 4: Import and Render the Component
Finally, import and render the Resume
component in your main application file (App.jsx
):
import React from 'react';
import Resume from './Resume';
import './index.css';
const App = () => {
return (
<div>
<Resume />
</div>
);
};
export default App;
Voila ๐๐ Now you have the resume component with you. Feel free to customize it. Add cool hover effects and style it to your liking.
Here is the link to the GitHub repo, Give it a start โญ if you found it helpful.
Additional Resources
Below is a list of FREE resources you can use to grasp Tailwind CSS.
TailWindCSS Docs - The official documentation to TailwindCSS. It covers every single aspect of vanilla CSS and how it is done using tailwind via utility classes.
TailwindCSS Basics for Beginners - Daily.Dev blog to get you started in case you haven't used Tailwind before
TailwindLabs (YouTube Series) - Tailwind CSS: From Zero to Production video series
Shruti Balasa on Youtube - Amazing YouTube tutorials of TailwindCSS. Definitely check it out.
Conclusion
By following these steps, you can create a professional resume layout using Tailwind CSS. This approach ensures your resume is not only visually appealing but also easy to maintain and customize. Happy coding!
Subscribe to my newsletter
Read articles from Preston Mayieka directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Preston Mayieka
Preston Mayieka
I enjoy solving technical problems, researching and developing new technologies, designing software applications for different platforms.