What is NodeJs
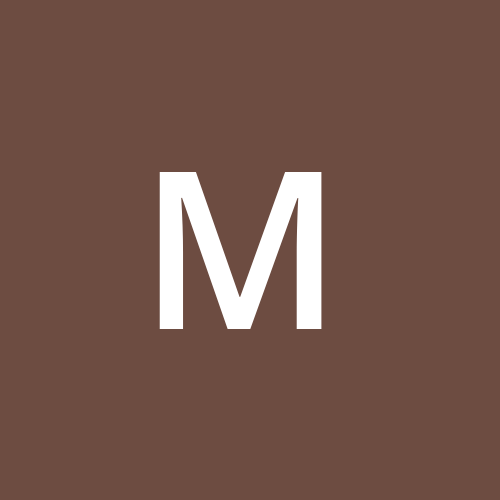
Table of Contents
ABOUT NODEJS
Node.js is an open-source JavaScript runtime environment that executes code outside web browsers, functioning as a standalone application. It supports cross-platform, which means it works on Windows, OSX, Linux, etc. It is a lightweight and easy-to-use framework perfect for beginners to get their hands on. Big corporations like Netflix and Uber use Node.js. This article will help you understand the architecture, dependencies, and features, showcasing why Node.js is a preferred choice for scalable server-side applications.
NODEJS ARCHITECTURE
Nodejs has important dependencies (Dependencies are libraries). Nodejs relies on dependencies to work properly. The two main dependencies are the V8 engine and LIB UV.
V8 engine is a JavaScript engine developed by the Chrome team at Google. It is at the heart of both Node.js and the Chrome browser. V8 is responsible for interpreting and executing JavaScript code. It converts JavaScript code into machine code for faster execution. V8 ensures optimal performance using JIT and garbage collection for memory management. It is open source and is written in C++.
Lib UV is an open-source library written in C++ that deals with asynchronous I/O. LIBUV gives node access to the underlying computer OS, File System, Networks, and many more. LIB UV has 2 main features, event loop and thread pool.
Event Loop: Responsible for executing tasks like callback functions, and Network IOS.
Thread Pool: It processes tasks like file access, compression, and cryptography like hashing passwords, DNS lookups, etc.
The main thread executes easy tasks and the heavy tasks are offloaded to the thread pool so the main thread isn’t blocked. The thread pool is separated from the main thread and usually 4 thread pools are in use but you can configure up to 1024 threads in a thread pool.
Key features of Nodejs
Single Threaded: The code runs in a single execution thread, nodeJS uses a single main thread to handle requests. That means it handles multiple instances without creating a new thread for every request.
Asynchronous I/0: when an I/O operation is initiated, instead of blocking the entire thread, the operation is delegated to the underlying system. Node.js continues executing other tasks or handling additional incoming requests while waiting for the I/O operation to complete.
A Simple Analogy (Comparing Asynchronous and Synchronous)
Synchronous: You're at the checkout counter with a cashier. The cashier scans each item, you have to wait for each item to be processed before moving on to the next item. While the cashier is dealing with your items, no other customers can start their checkout process. It's a linear operation.
Asynchronous: You're at a self-checkout station with multiple lanes. You start scanning your items (initiating an I/O operation i.e, reading from a file, making a network request), while each item is being scanned, the system asynchronously processes the information (initiate the next operation while the system processes the current one). While the system is handling one item, you can continue scanning (initiate the next operation while the system processes the current one) the next one without waiting for the previous one to be fully processed.
Meanwhile, other customers are using different self-checkout lanes simultaneously. Each customer's checkout process is asynchronous, allowing multiple customers to proceed independently. (processing multiple operations concurrently)
Note: Asynchronous functions will run and execute only after all the synchronous functions have been executed. Until then, they will be processed in the background.
How Event Looping Works
Events, like keyboard presses or mouse clicks, trigger actions in a system. The event loop, a continuous background process, listens for events without blocking the main thread. In Node.js, tasks are delegated to the native system, allowing concurrent execution. The main thread manages easy executions, while the event loop handles callback functions like file access and crypto hashing, offloaded to threads in the thread pool.
Phases of Event Loops
The event Loop has multiple phases, and each phase has a separate callback queue. There are separate callback queues for different types of events.
The event loop contains six phases, each functioning as a First In, First Out (FIFO) queue. When the event loop enters a given phase, it executes callbacks until the queue is exhausted or the maximum number of callbacks is run and moves to the next stage.
1) Timers: This is the first phase in the event loop. The event loop checks for timers, setTimeout(), and setInterval() whose time has expired. If a timer has expired, its associated callback function is executed.
2) I/O Callbacks: The I/O callback executes callback functions related to I/O events. Callbacks such as file I/O, network I/O, and other asynchronous operations.
3) Idle, Prepare: They are only used internally. The idle phase runs setImmediate scheduled callbacks, while the prepare phase handles system-specific preparations.
4) Poll Phase: It calculates the blocking time in every iteration to handle I/O callbacks. It checks for new I/O events (For example: Incoming requests) and executes their associated callbacks. If there are no I/O events, The event loop might wait for events to arrive or for timers to expire.
5) Check Phase: The check phase executes callbacks scheduled by setImmediate. The callbacks will be executed immediately once the Poll phase becomes idle.
6) Close Phase: The close callbacks phase is responsible for executing callbacks registered by close events, such as closing a socket or a file.
Npm is the official package manager for Node.js, it is wholly written in JavaScript. Npm manages all the packages and modules for Node.js and allows you to install, manage, and share packages or libraries. The command line is client is npm
. To install a package npm install packageName
.
The package.json file is a key component of Node.js projects. Package.json can install as well as uninstall files. It contains metadata about the project, such as its name, version, dependencies, and scripts.
To install packages globally ( if you install globally you can use the tools and utilities from the package across different projects.
npm install -g packageName
To install locally (local packages are project-specific that is, they can only be used in that particular project.
npm install packageName
To check the version of the npm package
npm -v
To update to the latest version
npm update npm@latest -g.
To uninstall a package
npm uninstall
Uninstall globally
npm uninstall package_name -g
These are just some of the client command lines, you can explore more in the official document.
Summary
In this article, we saw in detail about node.js, the whole architecture of node.js which includes key components like the V8 engine, and LIBUV (event loop, thread pool). Asynchronous I/O. Event loop in detail in 6 phases. And NPM manager and some of its important client line commands.
Subscribe to my newsletter
Read articles from Mosaddeka Mallick directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
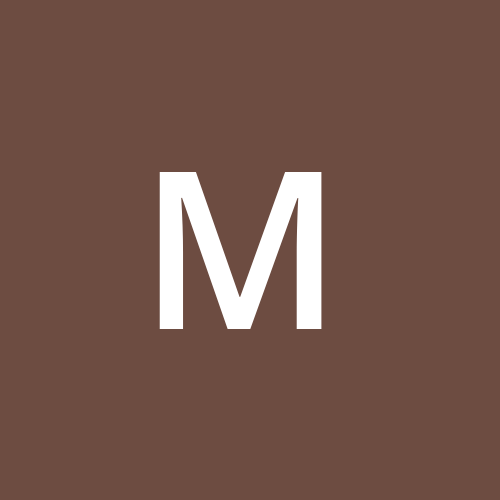