Make Your 'Boring' Java Console App Stand Out👨🎓🏫
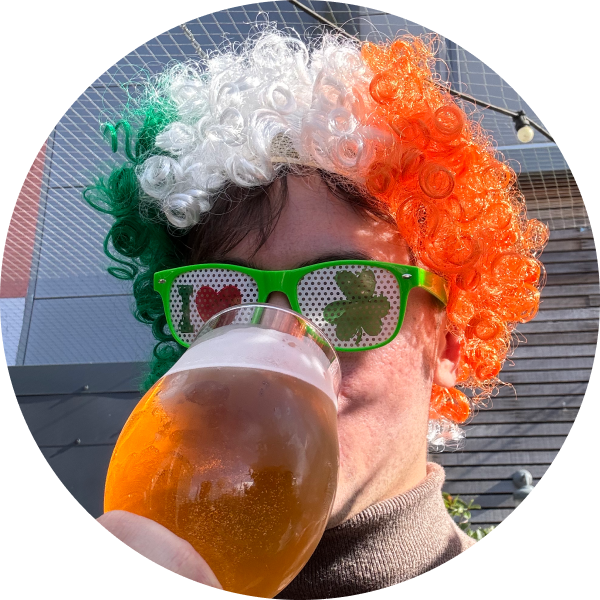
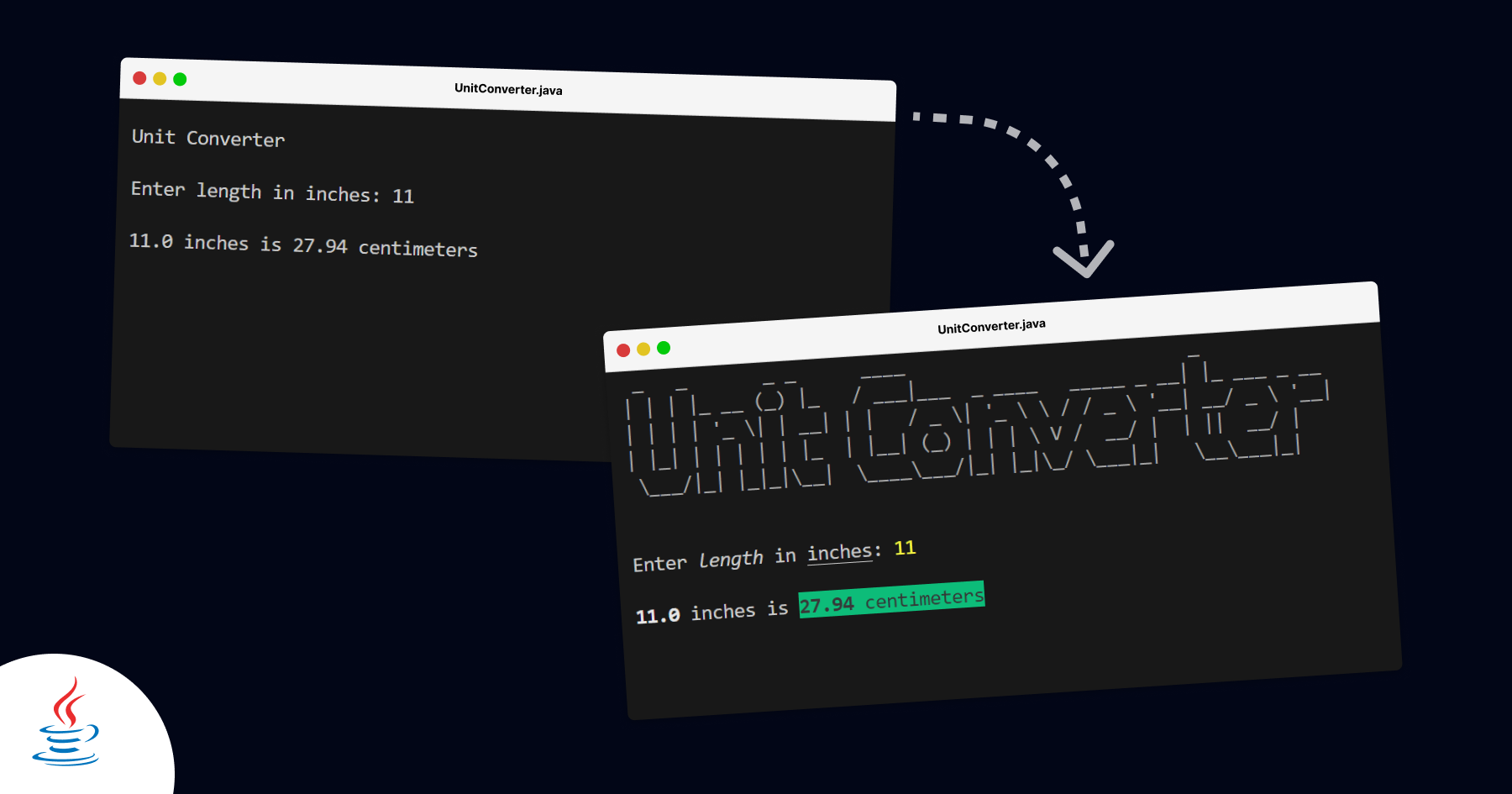
Introduction 👋
As a college student learning Java, I've spent countless hours on simple console applications. While the main goal is to grasp the underlying logic, I often wished I could make the "user interface" more exciting. That's when I discovered ANSI escape codes. If you want to make your console apps pop, these codes might be just what you need.
ANSI Escape Sequence
ANSI escape codes control text formatting, colors, and other options on text terminals. Each ANSI escape sequence consists of four parts:
Escape Character (
\033
in octal)Control Sequence Introducer (
[
)Parameter (specifies what we want to change - text style, text color, background color)
Final Byte (
m
specifies that the preceding numbers are related to graphic rendering modes)
For example, an ANSI escape sequence to change the text color to red looks like this: \033[31m
As you'll see, the only part we'll be changing is the parameter, which represents the changes in appearance we want to apply.
Basic Syntax
System.out.println(ANSI_CODE + "This text is colored" + ANSI_RESET);
ANSI_CODE
is the ANSI escape sequenceANSI_RESET
is a "special" ANSI escape sequence (\033[0m
) that turns off all ANSI attributes, returning the console to its default appearance (white text on a black background).
Text Style ⌨️
Before we start playing with colors, let's try to change the style of the text. Here are the styles we can choose from:
Bold:
\033[1m
Italicized:
\033[3m
Underline:
\033[4m
public class TextStyles {
public static final String ANSI_RESET = "\033[0m";
public static final String TEXT_BOLD = "\033[1m";
public static final String TEXT_ITALIC = "\033[3m";
public static final String TEXT_UNDERLINE = "\033[4m";
public static void main(String[] args) {
System.out.println(TEXT_BOLD + "Hello World" + ANSI_RESET);
System.out.println(TEXT_ITALIC + "Hello World" + ANSI_RESET);
System.out.println(TEXT_UNDERLINE + "Hello World" + ANSI_RESET);
}
}
💡 We could write the ANSI escape sequences directly into the 'Hello World' string, but I prefer to store them as constants. It makes the code easier to read and understand.
Colors 🎨
We can also use ANSI codes to change the text and background colors.
Text Color
Color | Standard | Bright |
Black | \033[30m | \033[90m |
Red | \033[31m | \033[91m |
Green | \033[32m | \033[92m |
Yellow | \033[33m | \033[93m |
Blue | \033[34m | \033[94m |
Magenta | \033[35m | \033[95m |
Cyan | \033[36m | \033[96m |
White | \033[37m | \033[97m |
public class Foreground {
public static final String CYAN_COLOR = "\033[36m";
public static final String BRIGHT_YELLOW = "\033[93m";
public static final String ANSI_RESET = "\033[0m";
public static void main (String[] args){
System.out.println(CYAN_COLOR + "Hello World" + ANSI_RESET);
System.out.println(BRIGHT_YELLOW + "Hello World" + ANSI_RESET);
}
}
Background Color
Color | Standard | Bright |
Black | \033[40m | \033[100m |
Red | \033[41m | \033[101m |
Green | \033[42m | \033[102m |
Yellow | \033[43m | \033[103m |
Blue | \033[44m | \033[104m |
Magenta | \033[45m | \033[105m |
Cyan | \033[46m | \033[106m |
White | \033[47m | \033[107m |
public class Background {
public static final String RED_COLOR = "\033[41m";
public static final String BRIGHT_GREEN_COLOR = "\033[102m";
public static final String ANSI_RESET = "\033[0m";
public static void main (String[] args){
System.out.println(RED_COLOR + "Hello World" + ANSI_RESET);
System.out.println(BRIGHT_GREEN_COLOR + "Hello World" + ANSI_RESET);
}
}
256-Color Mode
To use the 256 color codes:
foreground:
\033[38;5;{color_code}m
background:
\033[48;5;{color_code}m
Where {color_code}
ranges from 0 to 255.
RGB
To use RGB codes:
foreground:
\033[38;2;{r};{g};{b}m
background:
\033[48;2;{r};{g};{b}m
Where {r}
, {g}
, and {b}
are the red, green, and blue color values, respectively, ranging from 0 to 255.
Combining Properties
ANSI escape sequence also allows us to specify multiple parameters separated by semicolons. For example, to make the text italicized and red:
public class CombinedStyles {
// 3 - Italicized
// 31 - Red text color
public static final String ITALIC_RED = "\033[3;31m";
public static final String ANSI_RESET = "\033[0m";
public static void main(String[] args) {
System.out.println(ITALIC_RED + "Hello World" + ANSI_RESET);
}
}
Or we can concatenate multiple escape sequences:
public class CombinedStyles {
public static final String BOLD = "\033[1m";
public static final String RED_TEXT = "\033[31m";
public static final String YELLOW_BACKGROUND = "\033[43m";
public static final String ANSI_RESET = "\033[0m";
public static void main(String[] args) {
System.out.println(BOLD + RED_TEXT + YELLOW_BACKGROUND + "Hello World" + ANSI_RESET);
}
}
Extra Tip - Using ASCII Art🖌️
To add some extra flair, you can use text-to-ASCII art generators for titles. I recommend the ASCII Art Archive generator. Type your desired text, click 'Copy to Clipboard,' and paste it into your print statement.
public class Title {
public static void main(String[] args) {
System.out.print(" _ _ _ _ __ __ _ _ _ \r\n" + //
"| | | | ___| | | ___ \\ \\ / /__ _ __| | __| | |\r\n" + //
"| |_| |/ _ \\ | |/ _ \\ \\ \\ /\\ / / _ \\| '__| |/ _` | |\r\n" + //
"| _ | __/ | | (_) | \\ V V / (_) | | | | (_| |_|\r\n" + //
"|_| |_|\\___|_|_|\\___/ \\_/\\_/ \\___/|_| |_|\\__,_(_)");
}
}
Conclusion
I hope these tips help you add some flair to your college projects by enhancing the appearance of your console applications. While these changes won't necessarily improve your grades or the functionality of your apps, they can make your work more visually appealing and fun to create. So, if you have some spare time, why not make your app stand out a bit? 😎
Sources🔗
📄 How to Print Colored Text in Java Console? (geeksforgeeks)
🛠️ ASCII Art Archive (Text to ASCII Art Generator)
Subscribe to my newsletter
Read articles from Filip Melka directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
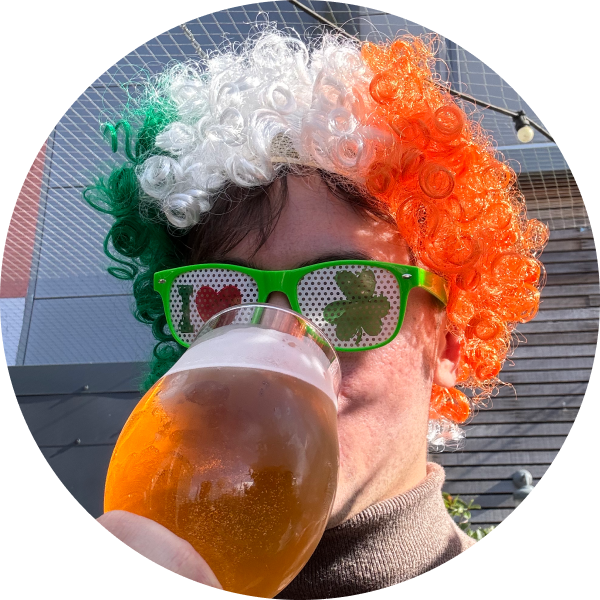
Filip Melka
Filip Melka
Hi there!👋 I’m Filip, an enthusiastic software development student👨🎓 My passion for learning led me to pursue a path in software development. I believe software has the incredible potential to revolutionize the learning experience, making it more accessible, engaging, and rewarding. With the rapid advancements in AI and other technologies, I'm excited about the potential to make a positive impact in education, and I’m eager to be a part of this transformation. Why did I start this blog? Mainly for two reasons. First, it keeps me motivated. As an aspiring software developer, I encounter 🐞 every day and sometimes feel like giving up. Writing about my side projects and what I’m learning keeps me going💪 Second, writing helps me organize my thoughts and grasp complex topics more thoroughly. If my posts help someone else too, that’s a huge win!