Mastering Hackathons: How to craft AI Apps using Streamlit and OpenAI API
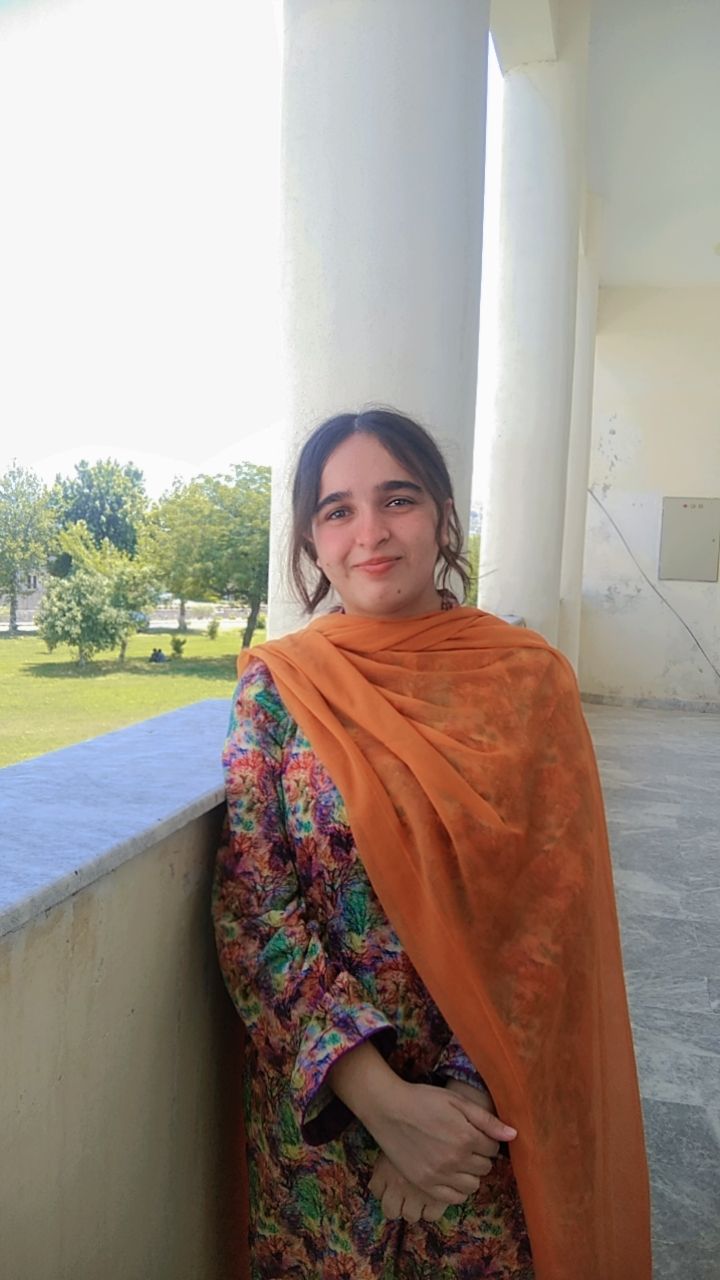
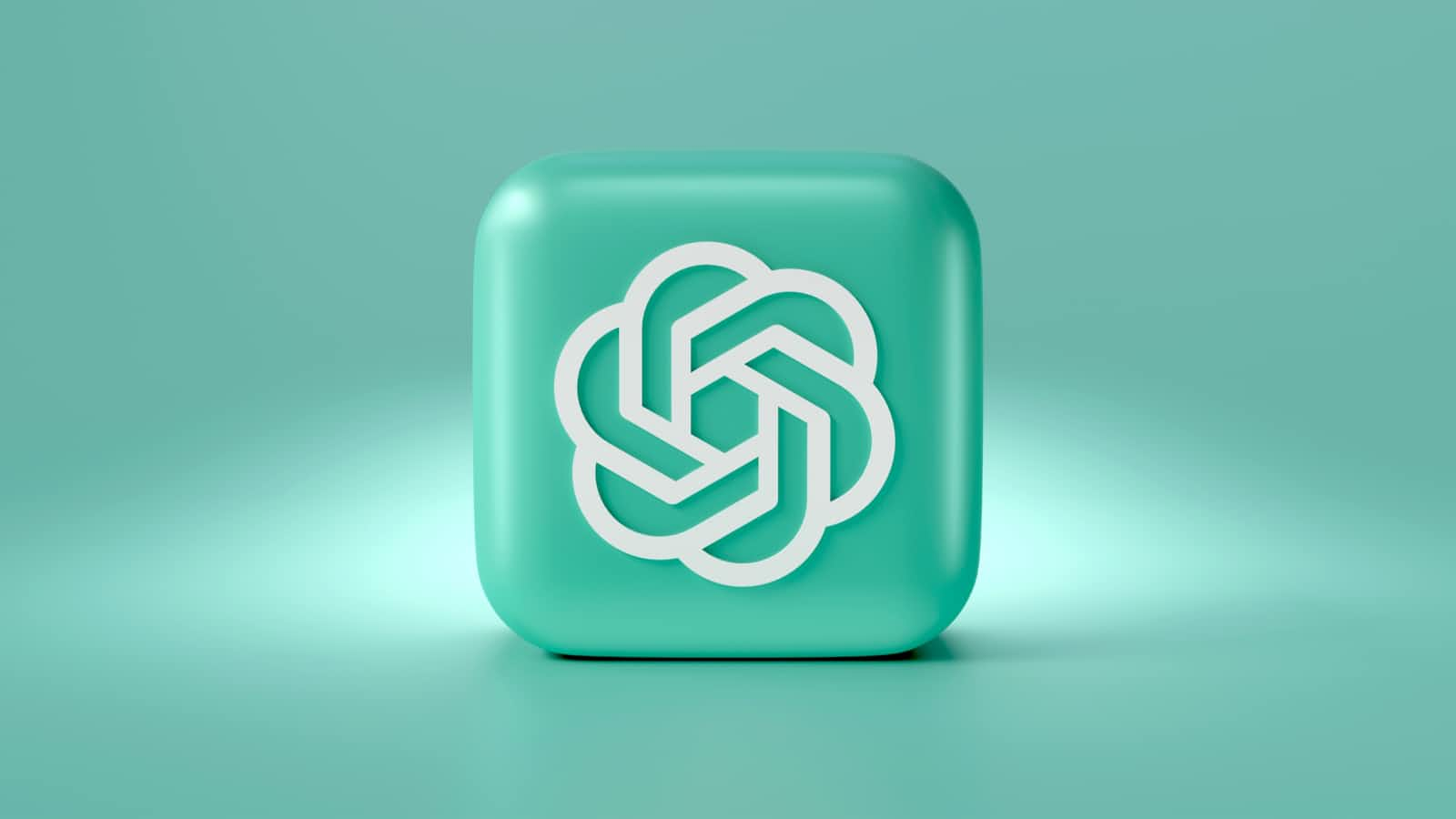
This article will help you to quickly make a streamlit app that leverages OpenAI api. For this tutorial we will make a Language Translator App using streamlit.
Here are the steps that we will follow:
Install Dependencies
Make streamlit app
Add OpenAI API Key
Run Streamlit app
Deploy to the Streamlit Cloud
now we need to install Dependencies that are
streamlit
dotenv
first of all we need to create a virtual environment for our project, for that run this command in terminal:
python -m venv env
after a folder called env
has been created in your project. you need to activate the virtual environment. for that run this command:
source env/Scripts/activate
Now That a virtual environment has been created we start installing dependencies.
Installing Dependencies
streamlit:
open your terminal in the directory of your project and run this command:
pip install streamlit
Dotenv:
I am using
dotenv
in my project to securely manage and load sensitive environment variables, such as API keys, from a centralized configuration file.To install
dotenv
run this command:pip install python-dotenv
Now you need to create a new file called
.env
in your project.and add the Open AI API credentials in it like this:
OPENAI_API_KEY = your_openai_api_key_here
Making a Streamlit App
Create a file called app.py
Now we need to load environment variables in this file so we can use them.
from dotenv import load_dotenv
import os
# Load environment variables from .env file
load_dotenv()
# Set up OpenAI client
client = OpenAI(api_key=os.getenv("OPENAI_API_KEY"))
Now we will make the Front End of the app.
st.title("Translate to Urdu")
input_text = st.text_area("Enter text in any language:")
if st.button("Translate"):
if input_text:
urdu_translation = translate_to_urdu(input_text)
st.write("Urdu Translation:")
st.write(urdu_translation)
else:
st.warning("Please enter some text to translate.")
Code to get the input from user.
def translate_to_urdu(text):
prompt = f"Translate the following text to Urdu: {text}"
completion = client.completions.create(
model="gpt-3.5-turbo-1106",
prompt=prompt,
max_tokens=150
)
return completion.choices[0].text.strip()
Run Streamlit App
To run the streamlit app that we just created on local host. we just have to run this command in the terminal.
streamlit run app.py
your app will start running on http://localhost:8501/
For this basic streamlit app. It will look like this.
Deploy to the Streamlit Cloud
To deploy this app on the cloud
Create a requirements.txt file
and these lines in it.
streamlit openai python-dotenv
Create a GitHub repository and commit all changes to it.
On the top right of your streamlit app click on deploy
select your preferred settings like this.
5. Click Deploy and your app will be up and running in a few minutes.
That's it, Hope this tutorial helped you.
Subscribe to my newsletter
Read articles from Amna Hassan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
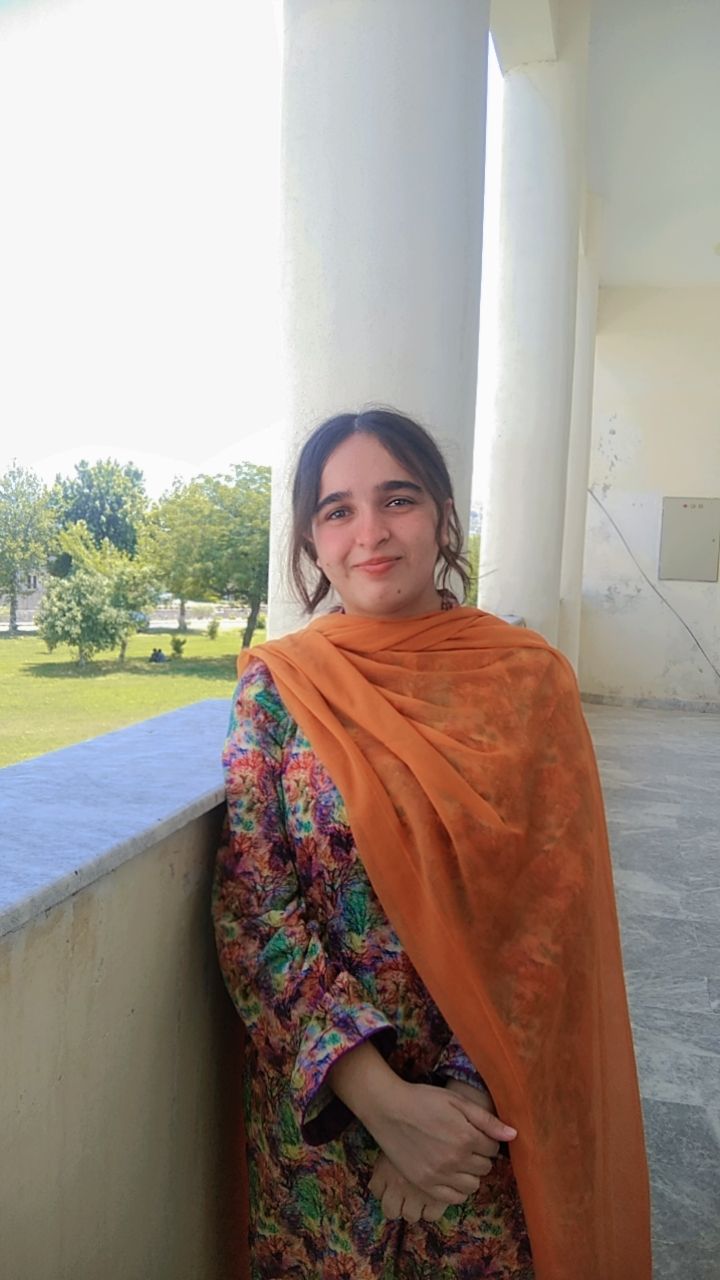
Amna Hassan
Amna Hassan
As the Section Leader for Stanford's Code In Place and a winner of the 2024 CS50 Puzzle Day at Harvard, I bring a passion for coding and problem-solving to every project I undertake. My achievements include winning a hackathon at LabLab.ai and participating in nine international hackathons, showcasing my dedication to continuous learning and innovation. As the Women in Tech Lead for GDSC at UET Taxila, I advocate for diversity and empowerment in the tech industry. With a strong background in game development, web development, and AI, I am committed to leveraging technology to create impactful solutions and drive positive change.