Challenges and Solutions I've Found in Managing Inheritance Hierarchies in Object-Oriented Programming
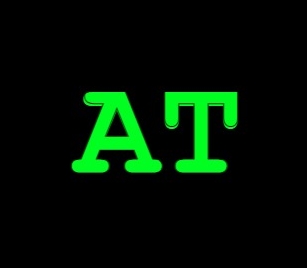
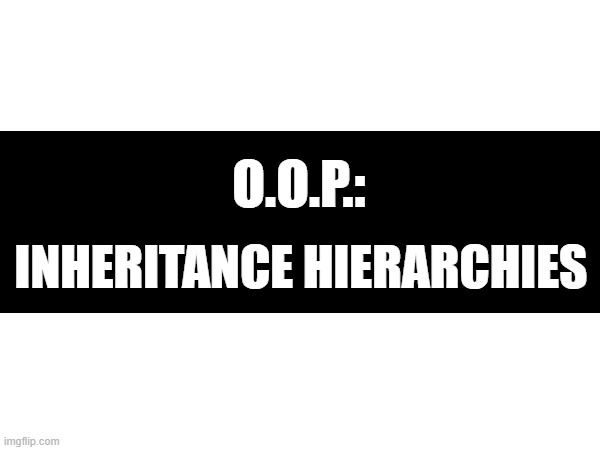
Like others, I use OOP to organize software around objects that interact using interfaces. The inheritance concept is key to OOP, allowing new classes to be based on existing ones and inherit their features and behaviors. Focusing on inheritance helps me enable code reuse, to create modular, maintainable systems. Here are some tips I have for handling the challenge of inheritance hierarchies especially in large and complex systems.
The Challenges of Inheritance Hierarchies
Tight Coupling: When a superclass changes, its subclasses can also be affected, making it hard to keep code separate and manageable.
Fragility: I make superclass changes backward compatible, to enhance robustness in updating many subclasses.
Complexity: As the hierarchy grows, which is mostly unavoidable, it becomes a challenge to understand the increasingly complex class relationships.
Inheritance vs. Composition: I find that deciding between inheritance (for "is-a" relationships) and composition (for "has-a" relationships) can be tricky.
Overriding and Overloading: Changing methods in subclasses can cause confusion, especially when methods with the same name and parameters are overridden in various places.
The Diamond Problem: Multiple inheritance can lead to conflicts when a class has two ancestors with a common ancestor, creating a diamond-shaped hierarchy.
Single Responsibility Principle (SRP) Violations: Inheritance might force a subclass to handle tasks it doesn't need, making it bloated and hard to manage.
Dependency Inversion Principle (DIP) Violations: Inheritance can cause high-level modules to rely on low-level ones, which goes against the goal of having a flexible system structure.
Common Inheritance Hierarchy Management Challenges
Deep Hierarchies: I've found that having many inheritance levels creates complex structures with hard-to-manage small, specialized classes.
Overriding Methods: I look to prevent mismanaged overrides because they can lead to unexpected behavior and difficulties in ensuring the correct runtime performance.
Static vs. Dynamic Polymorphism: I find that it's often a challenge to choose between performance-friendly static polymorphism and flexible dynamic polymorphism.
The Liskov Substitution Principle (LSP): I try to make subclasses interchangeable with their superclasses without causing errors. Violating this principle can lead to bugs and maintenance headaches.
Inheritance and Encapsulation: Inheritance can expose a superclass's details, making it hard to keep classes separate and maintainable.
Inheritance and Testing: Shared state and behavior can make it difficult to test individual classes without affecting others.
Easier Ways I've Found to Handle Inheritance Hierarchies
Prefer Composition: I use composition to build flexible classes by combining other objects, rather than extending them.
Stick to Single Responsibility Principle (SRP): I code to ensure each class has one main job to keep things simple and manageable.
Keep Hierarchies Shallow: I try to keep it simple and avoid deep inheritance trees to maintain clear class dependencies and simplicity.
Use Interfaces and Abstract Classes Sensibly: I define common contracts with interfaces and provide basic implementations with abstract classes, but don't overuse them.
Follow Dependency Inversion Principle (DIP): Depend on simple ideas rather than complex ones to keep design flexible.
Apply LSP: I design subclasses to fit in with superclasses without causing problems when used in place of them.
Clarify Interfaces: I make sure to clearly define class interfaces to reduce the impact of changes in subclasses.
Use Design Patterns: Patterns like Template Method, Strategy, and Factory help manage behavior and inheritance without making things too complicated.
Refactor Regularly: I keep checking and improving class structure to remove unnecessary parts and make it easier to maintain and scale.
Embrace Unit Testing and Mocking: I write tests for each class and use mock objects to isolate them from the inheritance hierarchy, to make them less sensitive to changes in superclasses.
Conclusion
Applying these insights and tips to these challenges can help to create robust, scalable, and maintainable systems. It's crucial to balance inheritance's benefits with the need for adaptable, modular code.
Subscribe to my newsletter
Read articles from Andrew Tetzeli directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
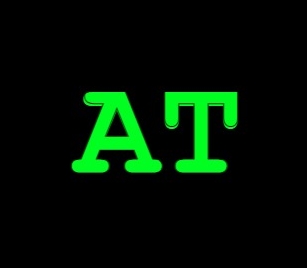
Andrew Tetzeli
Andrew Tetzeli
I provide bespoke software development and other IT solutions, including sysadmin, network management, and cybersecurity. I work in and on iOS, Android, macOS, Windows, Linux development; Python; C/#/++; Swift; Java (!); JavaScript; PHP; SQL...