Why I Emphasize KISS and DRY Over SOLID Principles in Software Development π
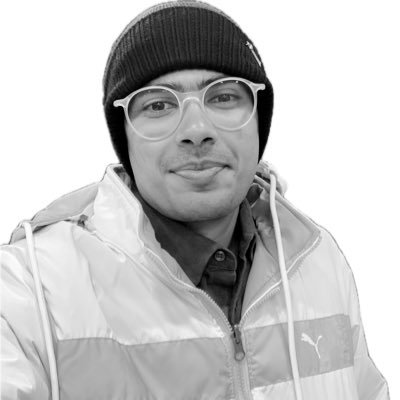
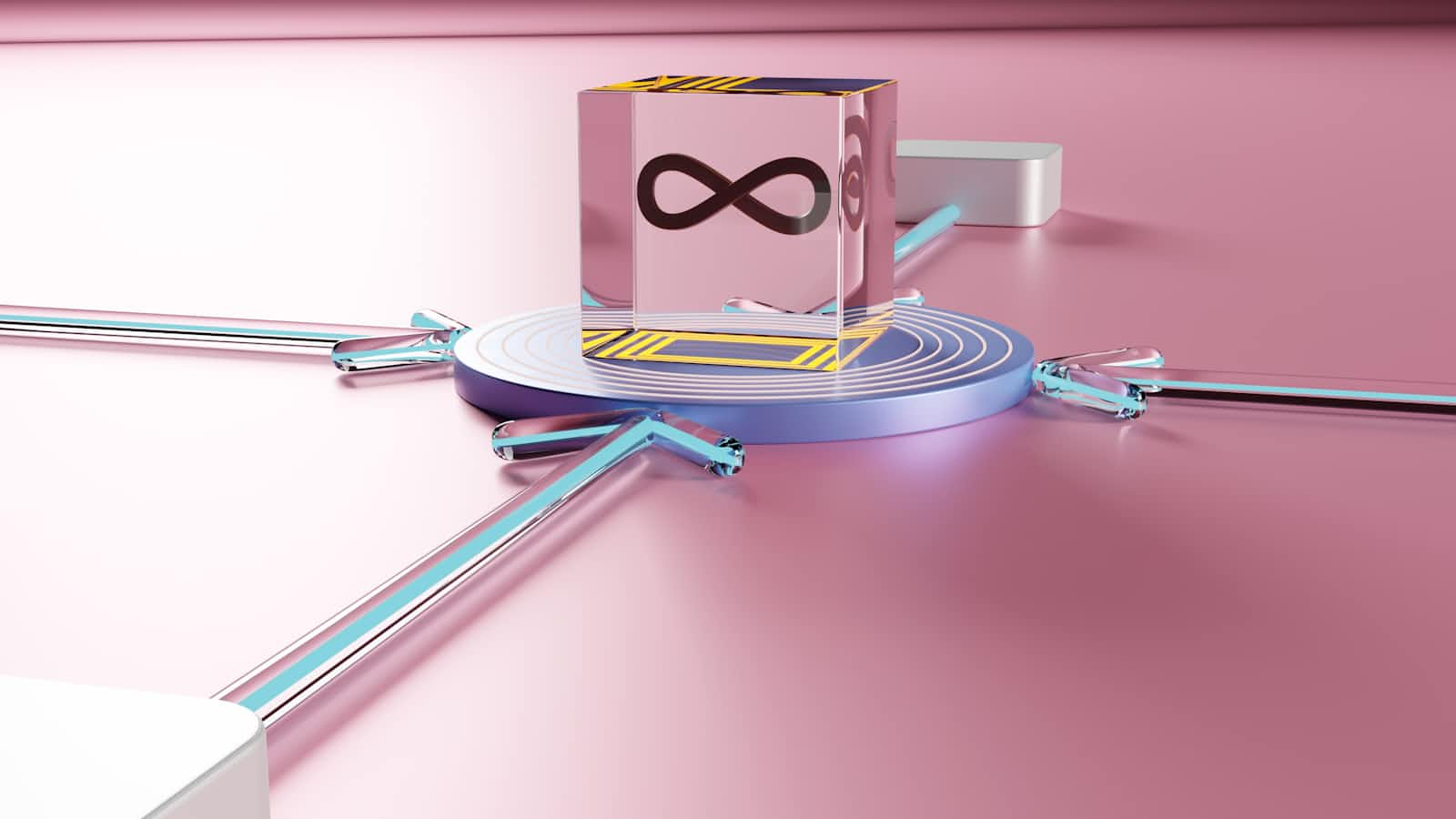
In the world of software development, principles and methodologies are essential for writing clean, maintainable, and efficient code. Among the various principles, KISS (Keep It Simple, Stupid), DRY (Donβt Repeat Yourself), and SOLID principles are often discussed and implemented by developers. While SOLID principles are critical for object-oriented design, I have found that emphasizing KISS and DRY principles often leads to better overall outcomes in my projects. In this blog post, Iβll share why I prioritize KISS and DRY over SOLID principles and how this approach benefits my development process.
Understanding KISS and DRY Principles π§
KISS (Keep It Simple, Stupid) π¨
The KISS principle emphasizes simplicity in design and implementation. The core idea is to keep the code as straightforward and uncomplicated as possible. Simplicity ensures that the code is easier to read, understand, and maintain.
DRY (Donβt Repeat Yourself) π
The DRY principle aims to reduce redundancy by ensuring that each piece of knowledge or logic is written only once. This approach minimizes duplication and promotes reusability, making the codebase more efficient and easier to manage.
Understanding SOLID Principles ποΈ
SOLID principles are a set of five design principles intended to make software designs more understandable, flexible, and maintainable. They are:
Single Responsibility Principle (SRP): A class should have only one reason to change.
Open/Closed Principle (OCP): Software entities should be open for extension but closed for modification.
Liskov Substitution Principle (LSP): Objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program.
Interface Segregation Principle (ISP): Clients should not be forced to depend on interfaces they do not use.
Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules; both should depend on abstractions.
Why I Emphasize KISS and DRY Over SOLID π
1. Simplicity and Readability π
KISS ensures that the code remains simple and readable, which is crucial for maintaining a clean codebase. When the code is straightforward, new developers can easily understand and contribute to the project, reducing onboarding time and increasing productivity.
// Example of KISS principle
function calculateTotal(price, quantity) {
return price * quantity;
}
2. Reduced Redundancy ποΈ
DRY reduces code duplication, making it easier to update and maintain. When changes are needed, they can be made in a single place, reducing the risk of introducing bugs and inconsistencies.
// Example of DRY principle
const TAX_RATE = 0.1;
function calculateTax(amount) {
return amount * TAX_RATE;
}
function calculateTotal(price, quantity) {
const subtotal = price * quantity;
const tax = calculateTax(subtotal);
return subtotal + tax;
}
3. Flexibility and Maintainability π§
While SOLID principles aim to enhance flexibility and maintainability, they can sometimes lead to overly complex designs, especially in smaller projects. By focusing on KISS and DRY, I achieve a balance between simplicity and flexibility, ensuring the code is easy to modify without unnecessary complexity.
// Example of balancing simplicity with flexibility
class Product {
constructor(name, price) {
this.name = name;
this.price = price;
}
getPriceWithTax() {
return this.price * (1 + TAX_RATE);
}
}
const apple = new Product('Apple', 1.2);
console.log(apple.getPriceWithTax()); // 1.32
4. Speed and Efficiency β‘
KISS and DRY principles often result in faster development cycles. By keeping the code simple and avoiding duplication, developers can implement features and fix bugs more quickly, leading to more efficient project delivery.
// Example of fast development with simple functions
function fetchData(url) {
return fetch(url)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error fetching data:', error));
}
fetchData('https://api.example.com/data');
5. Practicality in Real-World Projects π
In real-world projects, especially with tight deadlines and limited resources, itβs often more practical to prioritize simplicity and reduce redundancy. While SOLID principles are beneficial, they can sometimes be overkill for certain applications. Emphasizing KISS and DRY helps in delivering functional and maintainable software within constraints.
// Example of practical simplicity
const formatDate = date => date.toISOString().split('T')[0];
console.log(formatDate(new Date())); // '2024-07-03'
Balancing SOLID with KISS and DRY βοΈ
Itβs important to note that KISS, DRY, and SOLID principles are not mutually exclusive. In fact, they can complement each other when applied judiciously. The key is to strike a balance that fits the projectβs needs. Here are some tips for balancing these principles:
Start Simple: Begin with KISS and DRY principles to establish a clear and maintainable codebase.
Refactor When Needed: As the project grows, refactor the code to incorporate relevant SOLID principles.
Avoid Premature Optimization: Donβt over-engineer the codebase from the start. Apply SOLID principles incrementally as the need arises.
Keep the User in Mind: Always prioritize user needs and project requirements over strict adherence to any set of principles.
While SOLID principles are vital for object-oriented design, I have found that emphasizing KISS and DRY principles often leads to better outcomes in my projects. Simplicity and reduced redundancy not only make the code easier to maintain but also speed up the development process and enhance flexibility. By striking a balance between these principles, developers can create clean, efficient, and maintainable codebases that meet real-world needs.
Happy coding π
Subscribe to my newsletter
Read articles from Vivek directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
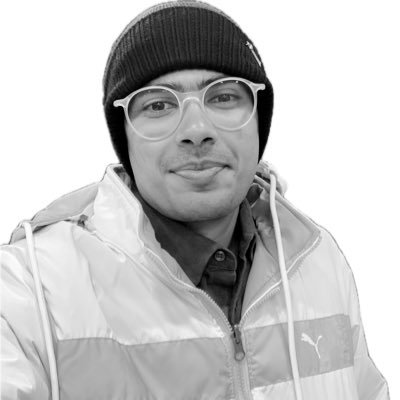
Vivek
Vivek
Curious Full Stack Developer wanting to try hands on β¨οΈ new technologies and frameworks. More leaning towards React these days - Next, Blitz, Remix π¨π»βπ»