Understanding SCSS

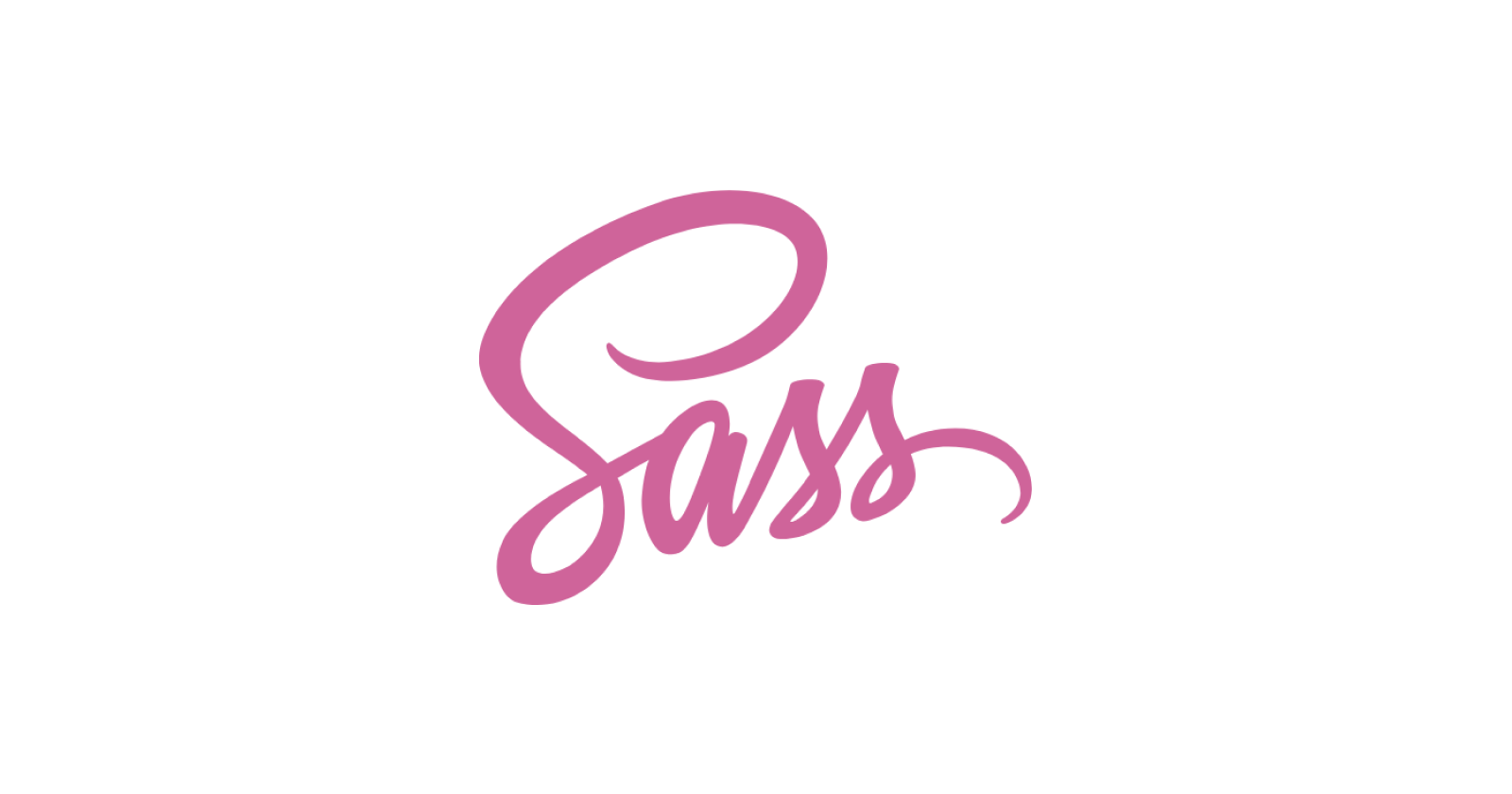
SCSS (Sassy CSS) is a preprocessor scripting language that is interpreted or compiled into CSS. It allows you to use variables, nested rules, mixins, functions, and more. Let's start with the basics and gradually move to more advanced concepts.
1. Variables
SCSS allows you to use variables to store values that you want to reuse throughout your stylesheet. This makes it easy to manage colors, fonts, or any CSS value.
Example:
code// Define variables
$primary-color: #3498db;
$font-stack: Helvetica, sans-serif;
body {
font: 100% $font-stack;
color: $primary-color;
}
2. Nesting
SCSS allows you to nest your CSS selectors in a way that follows the same visual hierarchy of your HTML.
Example:
codenav {
ul {
margin: 0;
padding: 0;
list-style: none;
}
li { display: inline-block; }
a {
display: block;
padding: 6px 12px;
text-decoration: none;
}
}
3. Partials and Import
You can create partial SCSS files that contain little snippets of CSS that you can include in other SCSS files. A partial is created by naming a file with a leading underscore.
Example:
//_colors.scss (file name)
code$primary-color: #3498db;
$secondary-color: #2ecc71;
//styles.scss (file name)
code@import 'colors';
body {
color: $primary-color;
}
4. Mixins
Mixins allow you to create reusable chunks of code. You can pass variables to mixins, making them even more flexible.
Example:
code@mixin border-radius($radius) {
-webkit-border-radius: $radius;
-moz-border-radius: $radius;
-ms-border-radius: $radius;
border-radius: $radius;
}
.box { @include border-radius(10px); }
5. Inheritance (Extend)
You can use the @extend
directive to share a set of CSS properties from one selector to another.
Example:
code.message {
border: 1px solid #ccc;
padding: 10px;
color: #333;
}
.success {
@extend .message;
border-color: #2ecc71;
}
.error {
@extend .message;
border-color: #e74c3c;
}
6. Operators
SCSS supports mathematical operations, which can be very handy.
Example:
code.container {
width: 100%;
}
.content {
width: 600px / 960px * 100%; // Converts to percentage
}
7. Functions
You can use built-in functions in SCSS to manipulate values.
Example:
code$color: #3498db;
.container {
background-color: lighten($color, 10%);
border: 1px solid darken($color, 10%);
}
8. Loops
SCSS supports loops for repetitive CSS tasks.
Example:
code@for $i from 1 through 3 {
.col-#{$i} {
width: 100% / 3 * $i;
}
}
This is a basic introduction to SCSS. There are more advanced topics like conditionals, functions, and more complex mixins and loops.
Subscribe to my newsletter
Read articles from Biswnath Mukherjee directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
