Step-by-Step Guide to Validations in Spring Boot Starter
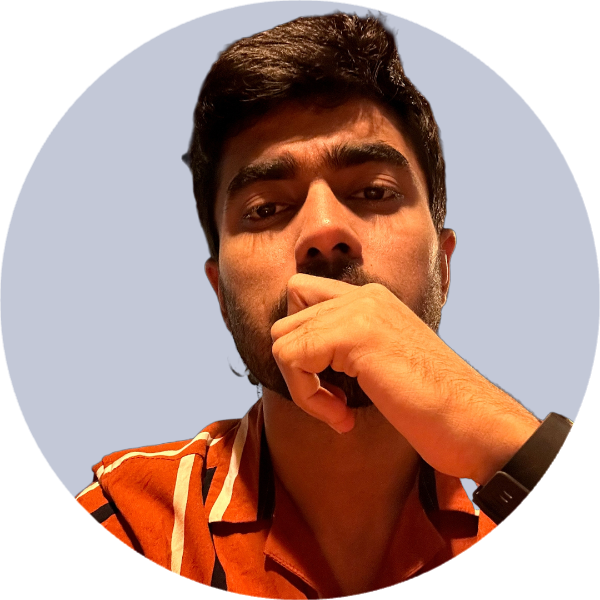
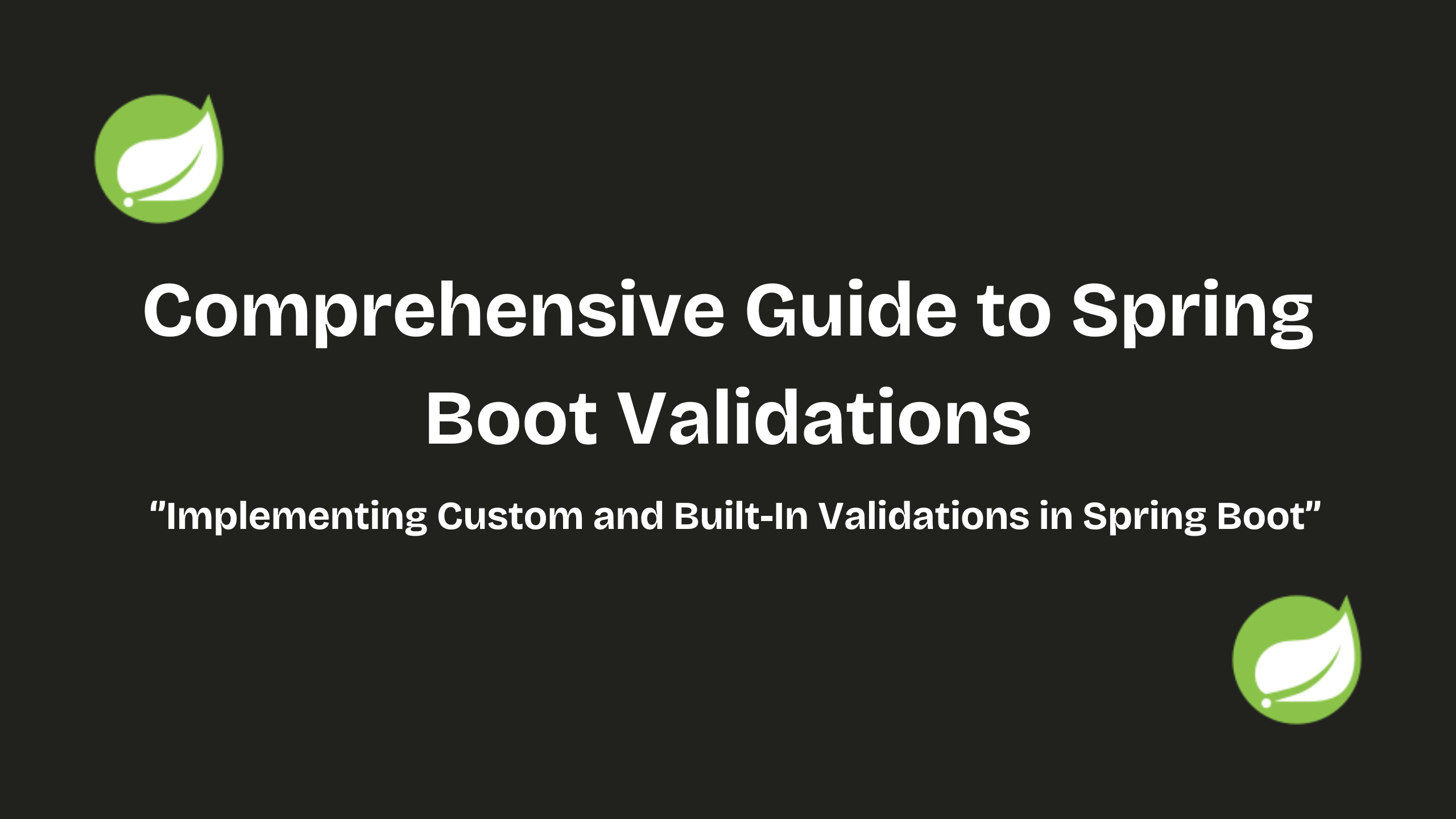
Introduction:
When developing a Spring Boot application that accepts user data, it's crucial to ensure the data is secure, maintains integrity, and meets specific constraints. For example, a user's age should be between 0 and 150 years. To handle these requirements, Spring Boot offers the spring-boot-starter-validation
framework, which can validate user input and return either a success or failure response based on the input. This guide provides a simple approach to setting up and using validations in your Spring Boot applications.
Why Validations Matter
Validations help:
Ensure Data Integrity: Prevent incorrect data from entering the system.
Enhance Security: Mitigate risks of malicious data injections.
Improve User Experience: Provide immediate feedback to users.
Setting Up Spring Boot for Validations
To start using validations in Spring Boot, you need to include the necessary dependencies and configure your application correctly.
Step 1: Add Dependencies
Include the following dependencies in your pom.xml
if you're using Maven:
For Gradle, add these to your build.gradle
:
Step 2: Create a Model with Validation Annotation
Create User.java
model class, which includes both built-in validation annotations and our custom password validation annotation:
Understanding Validation Annotations in Spring Boot
Spring Boot provides several other built-in validation annotations that are frequently used to ensure data integrity and correctness. Here are some of the most commonly used annotations:
NotBlank
Purpose: Ensures that the annotated field is not null and the trimmed length is greater than zero.
Example:
Size
Purpose: Validates that the size of the annotated field is within the specified range.
Example:
Email
Purpose: Validates that the annotated field is a well-formed email address.
Example:
Min
Purpose: Validates that the annotated field is a number with a value greater than or equal to the specified minimum.
Example:
Max
Purpose: Validates that the annotated field is a number with a value less than or equal to the specified maximum.
Example:
NotNull
Purpose: Ensures that the annotated field is not null.
Example:
NotEmpty
Purpose: Ensures that the annotated collection, map, or string is not null and not empty.
Example:
Positive
Purpose: Ensures that the annotated field is a positive number.
Example:
Negative
Purpose: Ensures that the annotated field is a negative number.
Example:
Future
Purpose: Validates that the annotated date or time is in the future.
Example:
Past
Purpose: Validates that the annotated date or time is in the past.
Example:
Pattern
Purpose: Validates that the annotated string matches the specified regular expression.
Example:
These annotations provide a powerful way to enforce data validation rules at the model level, ensuring that your application receives valid and correctly formatted data. By leveraging these built-in annotations, you can significantly reduce the amount of boilerplate code and enhance the robustness of your application.
Step 3: Create a Custom Annotation
Sometimes, built-in annotations aren't sufficient. You can create custom validators for more complex validation logic.
Here is our custom annotation :
Step 4: Implement the Constraint Validator
This class defines the rules for our custom @PasswordValidator
annotation and ensures that the password meets the specified criteria.
Here is the PasswordValidatorConstraint
class:
Step 5: Implementing the REST Controller
This controller will expose an endpoint to receive user data and trigger validation.
Step 6: Implementing the Global Exception Handler
Create a global exception handler using @RestControllerAdvice
to manage validation errors and format the error responses consistently.
Step 7: Creating the ErrorResponse Class
This class helps in formatting the validation errors with additional context such as the timestamp, request path, HTTP status, status code, and the detailed error messages.
Testing the Setup
Send a POST request to the /receive
endpoint with an invalid User
payload. For example:
The response should be similar to (ignore timestamp):
Conclusion
In this guide, we've explored how to implement robust validation mechanisms in a Spring Boot application using the spring-boot-starter-validation
framework. By following the steps outlined, you can ensure that the data entering your application adheres to predefined constraints, enhancing data integrity, security, and user experience.
Here's a quick recap of what we've covered:
Adding Dependencies: Included the necessary dependencies for validation in your project.
Defining the Model: Created a
User
model with built-in validation annotations.Creating Custom Annotations: Defined a custom password validation annotation.
Implementing the Constraint Validator: Wrote the logic for the custom password validator.
Setting Up the REST Controller: Created a REST controller to handle user input.
Handling Validation Errors: Implemented a global exception handler to manage validation errors and format error responses.
Custom Error Response: Designed a custom error response class to ensure consistent error message formatting.
By integrating these components, your Spring Boot application can effectively validate user inputs, providing clear feedback when validation fails. This not only helps in maintaining data integrity and security but also improves the overall user experience by ensuring that users are aware of and can correct their input errors promptly.
For a complete implementation, you can check out the GitHub project.
Feel free to clone the repository and explore the code to better understand how these validations are implemented in a real-world project.
Subscribe to my newsletter
Read articles from Sudarshan Doiphode directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
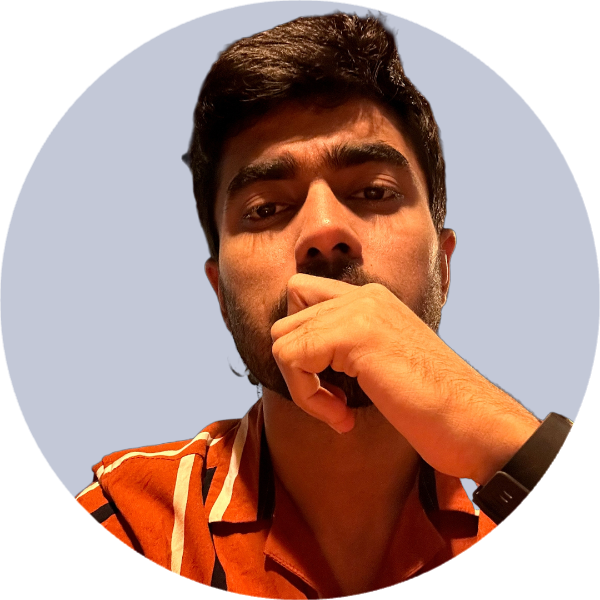
Sudarshan Doiphode
Sudarshan Doiphode
๐ Hey there! I'm Sudarshan, a tech enthusiast with a focus on: โข ๐ Spring Boot โข ๐ผ Spring โข ๐ Spring Security โข ๐ฑ Hibernate โข โ Java 8 & Java โข ๐ Apache Kafka โข ๐ข๏ธ Apache Maven โข ๐ณ Docker โข ๐ Git โข ๐๏ธ Design Patterns โข And more tech adventures! Follow for insights and join the journey. ๐๐จโ๐ป #TechEnthusiast