Building a Personalized Study Assistant App with React, Django, and MongoDB
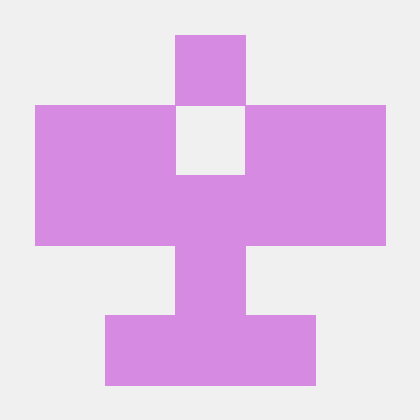
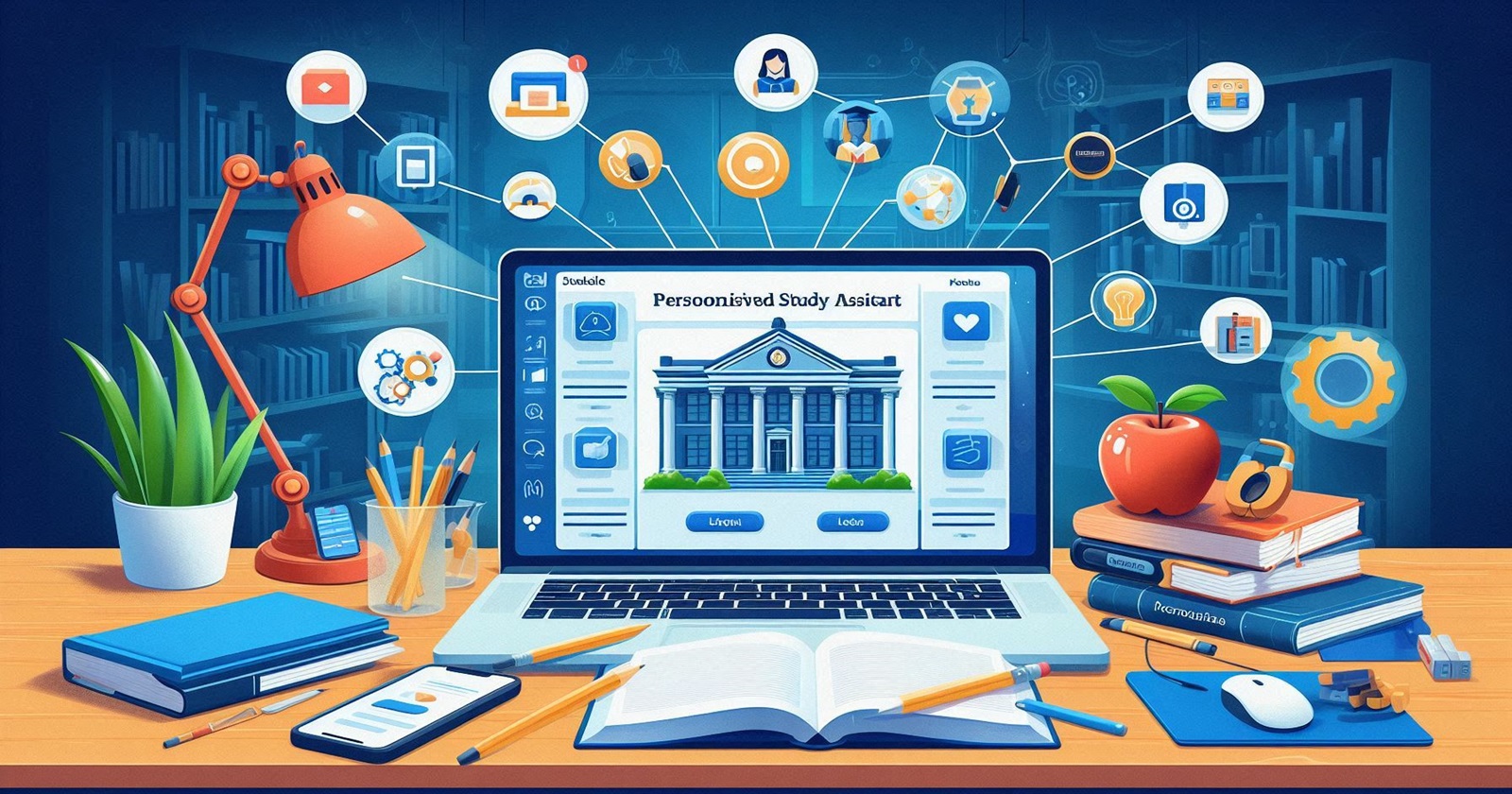
Introduction
In today's fast-paced world, personalized learning tools are becoming increasingly important. To address this need, I built a Personalized Study Assistant app. This app helps students manage their studies more effectively with features like quizzes, progress monitoring, and personalized study recommendations. In this blog post, I will walk you through the entire process of building this app, from setting up the frontend with React to creating the backend with Django and MongoDB. I'll provide detailed explanations of each step, along with the necessary code snippets where applicable.
Inspiration
As part of a hackathon, I was inspired to create this project after observing the struggles students face in managing their studies effectively. With the increasing amount of information and resources available, students often find it difficult to focus on what matters most. My goal was to develop an app that not only helps students manage their study schedules but also provides personalized assistance to enhance their understanding and performance. This project aims to simplify and optimize the learning process, making it easier for students to achieve their academic goals.
Problem-Solving Approach
The approach to solving this problem involved several key steps:
Identifying Core Features: Determining the essential features that would make the app valuable to students, such as topic explanations, study plans, quizzes, progress tracking, and notifications.
Designing the User Interface: Ensuring the UI/UX design is intuitive and user-friendly to enhance the overall user experience.
Choosing the Technology Stack: Selecting React for the frontend, Django for the backend, and MongoDB for database management to ensure a robust and scalable application.
Implementing the Features: Developing each feature with detailed explanations and necessary code.
Testing and Refining: Continuously testing the app and refining the features based on user feedback. Frontend Structure with React
The frontend of my app is built using React, a popular JavaScript library for building user interfaces. Here's a brief overview of the structure:
Project Setup
First, I created a new React app using Create React App:
npx create-react-app personalized-study-assistant
I then installed the necessary dependencies, including Firebase for authentication:
npm install firebase
Directory Structure
The directory structure of the React app is as follows:
personalized-study-assistant/
│
├── public/
│
├── src/
│ ├── components/
│ │ ├── Dashboard.js
│ │ ├── LoginPage.js
│ │ ├── ProgressMonitor.js
│ │ ├── QuizPage.js
│ │ └── ...
│ ├── firebaseConfig.js
│ ├── App.js
│ ├── index.js
│ └── ...
│
├── package.json
├── ...
Firebase Configuration
To enable user authentication, I set up Firebase. In firebaseConfig.js
, I initialized the Firebase app and set up authentication:
// src/firebaseConfig.js
import { initializeApp } from 'firebase/app';
import { getAuth } from 'firebase/auth';
const firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_AUTH_DOMAIN",
projectId: "YOUR_PROJECT_ID",
storageBucket: "YOUR_STORAGE_BUCKET",
messagingSenderId: "YOUR_MESSAGING_SENDER_ID",
appId: "YOUR_APP_ID",
};
const app = initializeApp(firebaseConfig);
const auth = getAuth(app);
export { auth };
User Authentication
For user authentication, I created the LoginPage.js
component. This component handles user login using Firebase's authentication service.
Dashboard and Other Components
The Dashboard.js
component serves as the main interface after login, where users can access various features like quizzes and progress monitoring. The QuizPage.js
component displays quizzes to the user, and the ProgressMonitor.js
component allows users to monitor their progress.
Backend Structure with Django and MongoDB
The backend of the app is built using Django, a powerful web framework, and MongoDB, a NoSQL database. Here’s how I structured the backend:
Setting Up Django
First, I created a new Django project and app:
django-admin startproject backend
cd backend
django-admin startapp study_assistant
I installed the necessary dependencies, including djongo
to integrate MongoDB with Django:
pip install djongo djangorestframework
Directory Structure
The directory structure of the Django backend is as follows:
backend/
│
├── study_assistant/
│ ├── migrations/
│ ├── __init__.py
│ ├── admin.py
│ ├── apps.py
│ ├── models.py
│ ├── serializers.py
│ ├── tests.py
│ ├── views.py
│ └── ...
│
├── backend/
│ ├── __init__.py
│ ├── settings.py
│ ├── urls.py
│ ├── wsgi.py
│ └── ...
│
├── manage.py
└── ...
Configuring MongoDB
I configured the MongoDB database in settings.py
:
# backend/settings.py
DATABASES = {
'default': {
'ENGINE': 'djongo',
'NAME': 'study_assistant_db',
}
}
Creating Models
I defined the models for quizzes and progress tracking in models.py
:
# study_assistant/models.py
from djongo import models
class Quiz(models.Model):
question = models.TextField()
option1 = models.CharField(max_length=100)
option2 = models.CharField(max_length=100)
option3 = models.CharField(max_length=100)
option4 = models.CharField(max_length=100)
answer = models.CharField(max_length=100)
class Progress(models.Model):
user = models.CharField(max_length=100)
quiz = models.ForeignKey(Quiz, on_delete=models.CASCADE)
score = models.IntegerField()
Creating Views and Serializers
To handle API requests, I created serializers and views. Serializers convert complex data types, like querysets and model instances, into native Python datatypes that can then be easily rendered into JSON or XML. Views handle the HTTP requests and responses.
Routing
I set up the routing for the API endpoints in urls.py
:
# backend/urls.py
from django.contrib import admin
from django.urls import path, include
from rest_framework import routers
from study_assistant.views import QuizViewSet, ProgressViewSet
router = routers.DefaultRouter()
router.register(r'quizzes', QuizViewSet)
router.register(r'progress', ProgressViewSet)
urlpatterns = [
path('admin/', admin.site.urls),
path('api/', include(router.urls)),
]
Integrating Frontend and Backend
To integrate the frontend with the backend, I used Axios for making API requests. For example, to fetch quizzes, I created a function in the QuizPage.js
component that sends a GET request to the backend API and updates the state with the received data.
Additional Features
Quizzes
The quiz feature allows users to take quizzes and get instant feedback. This feature is implemented with React state management and Axios for API requests. Users can select their answers, and the app calculates their score based on the correctness of their answers.
Progress Monitoring
The progress monitoring feature tracks the user's performance over time. This feature is implemented in the ProgressMonitor.js
component, which fetches the user's progress data from the backend and displays it in a user-friendly format. This helps users identify their strengths and areas for improvement.
The entire process of building the Personalized Study Assistant app:
The development of the Personalized Study Assistant app began with the inspiration to address the challenges students face in managing their studies. The process started by identifying core features such as topic explanations, study plans, quizzes, progress tracking, performance scoring, focus areas, and notifications. The next step involved designing an intuitive and user-friendly interface using React for the frontend, ensuring smooth navigation and interaction. For the backend, Django was chosen to handle data and business logic, while MongoDB was used for database management to ensure scalability and flexibility. Each component, from Topic Explanation to Notification, was meticulously developed with detailed explanations and necessary code to provide personalized assistance to students. Additional features like scoring overall performance, identifying focus areas, and notifying important exam dates were incorporated to enhance the app's real-world usefulness. Throughout the project, continuous testing and refinement were carried out to ensure feature completeness and an excellent user experience. This comprehensive approach not only solved the initial problem but also created an impactful tool to support students in their educational journey.
Impact
The Personalized Study Assistant app aims to revolutionize the way students approach their studies. By providing a comprehensive and personalized tool, the app helps students manage their study schedules, understand complex topics, track their progress, and stay updated with important dates. This holistic approach ensures that students can focus on their learning effectively and efficiently.
Conclusion
In this project, I have built a Personalized Study Assistant app using React, Django, and MongoDB. The app includes features such as topic explanations, study plans, quizzes, progress tracking, performance scoring, focus areas, and notifications. Each feature is designed to enhance the student's learning experience by providing personalized assistance and insights. The journey of creating this app involved careful planning, designing, and implementing each feature to ensure a user-friendly and impactful tool.
By working on this project, I gained deeper insights into full-stack development, API integration, and deploying machine learning models in real-world applications. This journey has been both challenging and rewarding, and I look forward to further enhancing this application with more features and improvements.
Subscribe to my newsletter
Read articles from Amey Pote directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
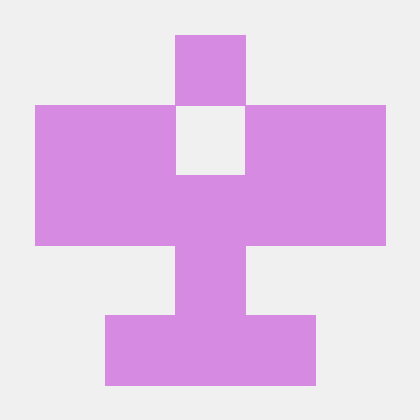