BookStore Docker 4 : Dockerlize Spring Application & Connect to MySQL Container With Dockerfile + docker-compose.yml
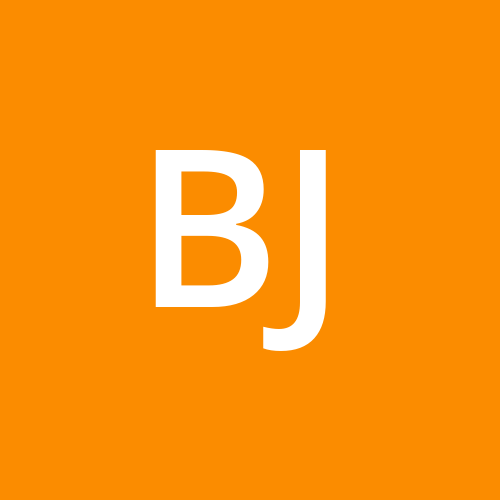
Foreword
I've tried to dockerize Spring boot application and connect it to mysql-container. But the processes were based on manual steps (only by Dockerfile
), which means hand-operated by command after building Spring Docker Image by Dockerfile
. This document will handle docker-compose.yml & Dockerfile
pair to set up these whole process automatically, including building Docker Image & Container
.
Frankly, I didn't understand the processes of using Docker. But, after I tried to create document about Docker from scratch, I finally caught the flow. If you want to see those documents, you can refer to the links below.
Dockerfile
: for building Docker Images
- This file contains instructions to build a Docker image for your Spring Boot application. It defines the environment and setup needed for the application.
docker-compose.yml
: for building Docker Containers
This file allows you to define and manage multiple Docker containers as a single service. (If your application involves multiple containers, you can use Docker Compose to manage them effectively.)
It can be used to automatically set up and connect your Spring Boot application container with a MySQL container by specifying their configurations and relationships.
Without docker-compose.yml, we need to handle this from scratch by command.
In summary, you use the Dockerfile
to build the Spring Boot application image, and then use docker-compose.yml
to orchestrate the containers and establish connections between them.
When Docker Compose docker-compose.yml
Doesn’t Need to Be Pushed
docker-compose.yml is for managing multiplie containers.
Kubernetes
- Use Kubernetes manifests (
.yaml
files for deployments, services, etc.) instead ofdocker-compose.yml
.
- Use Kubernetes manifests (
AWS ECS(Elastic Container Service)
- Use Kubernetes manifests (
.yaml
files for deployments, services, etc.) instead ofdocker-compose.yml
.
- Use Kubernetes manifests (
Docker Swarm
- You can use
docker-compose.yml
for defining stack deployments in Docker Swarm, but it’s specific to Docker Swarm. (docker-compose.yml is tailered for Docker Swarm.)
- You can use
Reference Link
Docker Basic, Installation
MySQL Monitoring With Docker
: https://joo.hashnode.dev/book-store-docker-2-mysql-monitoring
Dockerizing Spring Boot & Connect to mysql-container
Prerequisite
You need to install Docker Desktop.
These Dockerfile & docker-compose.yml file's processes for building Docker Images and Containers are very simple and easy, maybe you can't understand how it works. if you do, you can refer to this manual steps. (Frankly, since I tried all manual steps and organized that documents, I can understand this auto-configured processes.)
Ensure Docker Images are created (mysql, spring)Ensure Docker Containers are created (mysql, spring)Ensure Both containers are running
After I tried to build Docker Images and Containers with docker-compose.yml & Dockerfile
, it turned out that building Docker Container & Images aren't prerequisite. docker-compose.yml & Dockerfile
can build it automatically.
- But Commands are useful, you can refer to it.
Ensure Docker Image & Container are ready
Commands
Check Docker Images
docker images
Check the current containers that are running
docker ps
Check all Status for Docker (Optional), including stopped container
docker ps -a
Even though I stopped spring container, I can see all of them, While docker ps only shows currently running container.
Docker Desktop
Docker Image (Blueprint for Container)
Docker Container (Status -> Running)
Create : Dockerfile
& docker-compose.yml
: We can automatically dockerize Spring boot application & Connect to mysql container by Using Dockerfile & docker-compose.yml
, If we don't use docker-compose.yml
, we need to create bridge network and other things for building Docker Container, which means all processes isn't simple without it. If you want to know whole process, you can refer to link i mentioned.
1.Create Dockerfile on root directory
# Use the official OpenJDK image as a parent image
FROM openjdk:17-jdk-alpine
#Base Image: openjdk:17-jdk-alpine provides a lightweight environment with JDK 17.
# Set the working directory in the container
WORKDIR /app
#Working Directory: /app is set as the working directory inside the container.
# Copy the JAR file into the container
COPY build/libs/BookStore-0.0.1-SNAPSHOT.jar app.jar
# Copy JAR: Copies the built JAR file from the build/libs directory into the container.
# 'app.jar' in the Dockerfile is a way of renaming the JAR file inside the Docker image to a consistent name.
# Expose the port that the application runs on
EXPOSE 8080
# Expose Port: Exposes port 8080, which is the default port for Spring Boot applications.
# Define the command to run the application
ENTRYPOINT ["java", "-jar", "app.jar"]
# Run Command: Uses java -jar to run the JAR file.
2.Create docker-compose.yml on root directory
docker-compose.yml configuration
#version: '3.8' ==> this is obsolete
services:
bookstore:
build:
context: .
dockerfile: Dockerfile
ports:
- "8080:8080"
environment:
- SPRING_PROFILES_ACTIVE=default
- SPRING_DATASOURCE_URL=${SPRING_DATASOURCE_URL}
- SPRING_DATASOURCE_USERNAME=${SPRING_DATASOURCE_USERNAME}
- SPRING_DATASOURCE_PASSWORD=${SPRING_DATASOURCE_PASSWORD}
depends_on:
- mysql #To connect Spring Docker Container to mysql Container, we need Bridge Container.
networks:
- bookstore-network #bridge network name, where containers communicate
mysql:
image: mysql:latest
environment:
MYSQL_ROOT_PASSWORD: ${MYSQL_ROOT_PASSWORD}
MYSQL_DATABASE: ${MYSQL_DATABASE}
MYSQL_USER: ${MYSQL_USER}
MYSQL_PASSWORD: ${MYSQL_PASSWORD}
ports:
- "13306:3306"
networks:
- bookstore-network #bridge network name, where containers communicate
networks:
bookstore-network: #bridge network name, where containers communicate
driver: bridge
services
: Defines the services you want to run (your Spring application and the MySQL database).bookstore
: Your Spring Boot application.build
: Specifies the build context and Dockerfile.ports
: Maps container port8080
to host port8080
.environment
(for.env
): Sets environment variables for your Spring Boot application. Replaceyour_database_name
,your_username
, andyour_password
with your actual values.depends_on
: Ensures that themysql
service starts beforebookstore
.networks
: Connects the service to thebookstore-network
network.
mysql
: MySQL database container.image
: Specifies the MySQL Docker image.environment
: Sets environment variables required for MySQL. Replace with your desired values.ports
: Maps container port3306
to host port13306
.networks
: Connects the service to themy-network
network.
networks
: Defines a custom network (bookstore-network
) that both services will use.\==> It's a bridge network, where containers can communicate.
(Without those files, we need to create this in person for the Container)
.env
# For application.yml db connection
SPRING_DATASOURCE_URL=jdbc:mysql://mysql:3306/BookStore
SPRING_DATASOURCE_USERNAME=root (your_db_usename)
SPRING_DATASOURCE_PASSWORD=your_DBroot_password
# For Docker Image mysql:latest (For Regular User, not for Root use)
# MySQL Container Configuration
MYSQL_ROOT_PASSWORD=your_DBroot_password
MYSQL_DATABASE=BookStore
MYSQL_USER=ByungJoo
MYSQL_PASSWORD=yourPersonalPassword
application.yml
spring:
datasource:
url: ${SPRING_DATASOURCE_URL}
username: ${SPRING_DATASOURCE_USERNAME}
password: ${SPRING_DATASOURCE_PASSWORD}
driver-class-name: com.mysql.cj.jdbc.Driver
jpa:
database-platform: org.hibernate.dialect.MySQLDialect
hibernate:
ddl-auto: create
show-sql: true
Commands for running docker-compose.yml
- Run in detached mode (run in the background) - (Recommended):
-d
docker-compose up -d --build
Start the containers with Docker Compose:
docker-compose up --build
: to check currently running docker container
docker ps
Based on the current directorybe-bookstore
, Containers and Images are built.
- Stop and remove the containers:
docker-compose down
Containers & Network (Bridge Network) Removed
Containers (bookstore / mysql) & Network (bookstore-network)
+Command To check the network
container:
docker network ls
bookstore-bridgenetwork
is the one that I created for connecting spring-bookstore container to mysql-container while I am creating my documentation about manual steps. (It's a user-defined network
.)
+Command To remove the user-defined network
Once no containers are using specific network, you can remove it using the docker network rm
command.
docker network rm bookstore-bridgenetwork
Subscribe to my newsletter
Read articles from Byung Joo Jeong directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
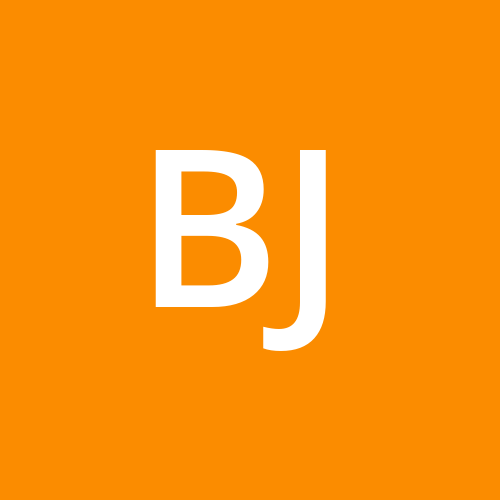