MyStudyMate: AI Tool for Optimizing Your Study Schedule

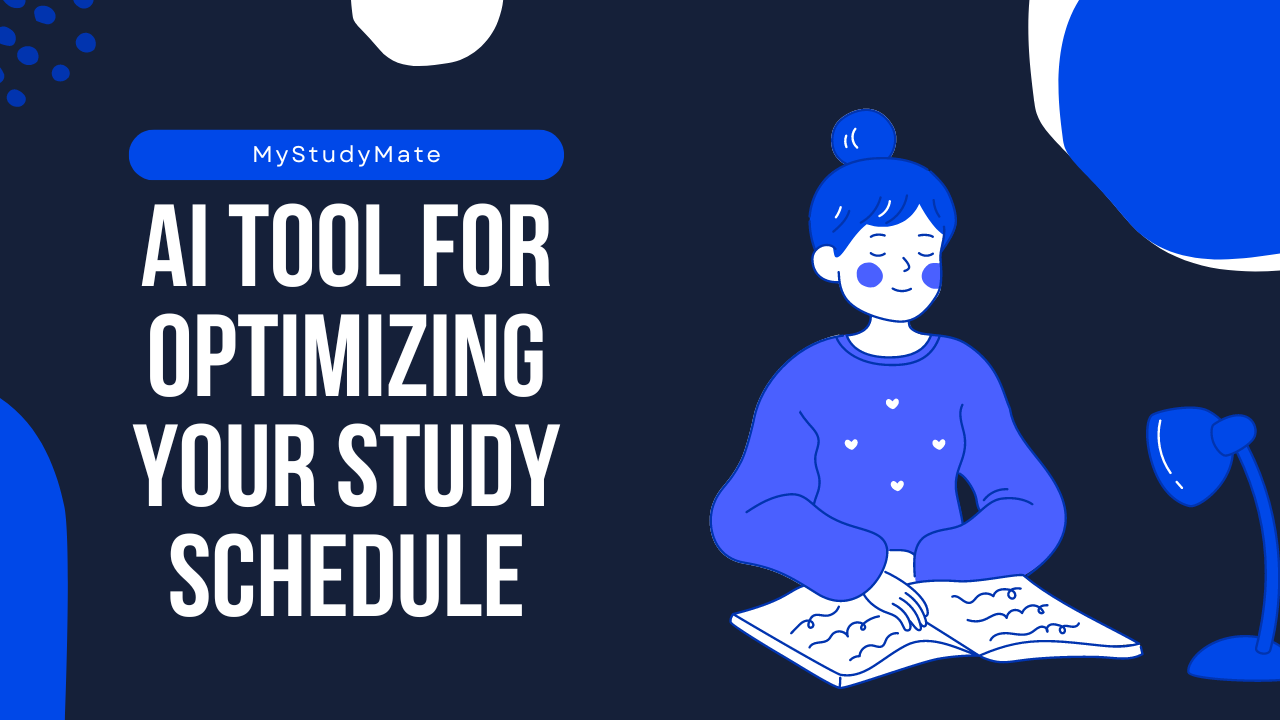
Hi All,
This article is about a project I worked on this week.
First, I will explain the main idea behind the project, how I got inspired, the solution I created, and then we will review the code.
We will also discuss the project's real-world usefulness and some future improvements that can be made.
So, let's dive in.
Introduction
MyStudyMate is an AI-powered study scheduling application designed to help students manage their study time more effectively. It automates the creation of personalized study schedules based on individual preferences and subject outlines, ensuring that students can focus on their studies without the hassle of manual planning. It also notifies the user when it is time for a study session.
GitHub Repository: https://github.com/HussnainAhmad1606/MyStudyMate
Inspiration
Managing your studies and finding time for learning can become quite challenging at times. Balancing university lectures, assignments, and projects can be overwhelming. Often, you might find yourself in a situation where you have to sacrifice one task to ensure the other doesn't suffer.
Personally, I encountered this issue frequently while trying to juggle my university coursework with my development projects. There were numerous instances where I had to put my development work on hold to focus on completing my university assignments and projects. This constant struggle to maintain a balance between academic responsibilities and personal projects inspired me to create a solution that could help others facing similar challenges.
Solution
That's when the idea of MyStudyMate came to me. It's a tool where I just provide the outline of the subject I'm currently enrolled in, and AI handles the rest. The AI creates a personalized schedule based on the subject outline and my initial understanding of the project.
This helps me manage both my university work and my development projects. I will have set times for what I need to do, instead of manually deciding which task to work on first.
Design Choices
1 - Mockups
First, I started with making the mockups of ExcaliDraw.
First I try to make how scheduling will work with AI. At start, I want to make it like table with output of AI at side of same table.
But, when I try to do this in figma, it wasn't looking good so I changed the whole design.
This was the mockup for session scheduling process.
I also try to draw analytics page to get a feel of how I need to arrange the elements.
I made these mockups just to get ideas from my head to the canvas so I can check whether ideas are good or I need to rearrange them.
2 - Color Scheme
The goal was to design an interface that is visually appealing, easy to navigate, and conducive to a focused study environment.
I saw many inspirations on Dribbble, Behance and Pinterest. I also try some study apps and website to get to know more about picking colors for education type project.
Primary Colors:
- Orange (#FF7F50): Orange was chosen for its vibrant and energetic qualities. It is used for buttons, highlights, and important call-to-action elements. This color brings a sense of enthusiasm and motivation, encouraging users to engage with their study schedules actively.
Secondary Colors:
Black (#000000): Black provides a strong contrast and is used for text, icons, and critical interface elements.
White (#FFFFFF): White is used for the background and other major sections, providing a clean, uncluttered look and helps to keep the interface simple.
3 - Figma Design
Then, I moved to figma to design the pages of the website to get feel of the website.
As, I already had drawn the mockups so I just have to try some variations of it to get to a point where the design look visually appealing & easy to navigate with study focused enviroment.
Project Overview
Currently, MyStudyMate has minimal features that a student needs to generate schedule based on subject outline and initial understanding.
It has following key features:
1 - Calendar View
Users can view all their scheduled study sessions on a calendar, complete with details for each session. This helps in visualizing the study plan and making adjustments as needed.
2 - Auto-Generated Schedules
Users can input outlines for each subject with initial understanding of user with the subject, which are then used to create personalized study plans.
AI will generate schedule based on outline topics and give personalized schedule.
3 - Session Timings
Users can set the times they want to study. This helps the AI generate a schedule within those times.
4 - Notifications
The website sends reminders and push notifications to keep users on track with their study seshsions.
5 - Analytics
MyStudyBuddy provides insights into study sessions, helping users see their statistics of study sessions.
Analytics also includes creating monthly study graph inspired by GitHub (this feature is still in development, that's why UI is not that good).
Code Review
Tech Stack
The application is built using Next.js for both the front-end and back-end, with MongoDB as the database. Gemini AI is integrated for schedule generation, Firebase Messaging is used for notifications, and custom analytics are implemented to track study performance. A Cron Job is set up to ensure users receive timely notifications.
Schedule Generation
For generating the schedule, I am sending the outline that user enters, with the study session timings that user previously entered along with a prompt.
Gemini gives the output as JSON and I am simply parsing it to show it to Frontend. User can add that sessions to his calendar by clicking on Add to Schedule Button or regenerate the schedule.
let msg = req.body.prompt;
const genAI = new GoogleGenerativeAI(process.env.GEMINI_API);
const model = genAI.getGenerativeModel({ model: "gemini-pro" });
const chat = model.startChat({
generationConfig: {
maxOutputTokens: 350,
},
});
const result = await chat.sendMessage(msg);
const response = await result.response;
const text = response.text();
User Authentication
For user authentication, I am using JWT Tokens. This method is highly secure and efficient for managing user sessions. When a user logs in, a token is generated and sent to the client. This token is then stored on the client side in localStorage.
import jwt from 'jsonwebtoken';
export async function loginUser(req, res) {
const { email, password } = req.body;
const user = await User.findOne({ email });
if (user && (await user.comparePassword(password))) {
const token = jwt.sign({ id: user._id }, process.env.JWT_SECRET, {
expiresIn: '1h',
});
res.status(200).json({ token });
} else {
res.status(401).json({ message: 'Invalid email or password' });
}
}
Notification System
For the notification system, I am using Firebase Messaging to send push notifications to users when it's time for a session.
Here is a setup for sending notifications:
import admin from "firebase-admin";
import { Message } from "firebase-admin/messaging";
if (!admin.apps.length) {
admin.initializeApp({
credential: admin.credential.cert(SERVICE_KEY),
});
}
const notificationHandler = async (request, response) => {
const { token, title, message, link } = request.body;
const payload = {
token,
notification: {
title: title,
body: message,
},
};
try {
await admin.messaging().send(payload);
return response.status(200).json({ type:"success", message: "Notification sent!" });
} catch (error) {
console.log(error)
return response.status(500).json({ type:"error", message: `Error: ${error}` });
}
}
export default notificationHandler;
Analytics
For the analytics, I am getting the info about the user, like sessions that user currently have and sessions that user completed along with whole time spend of all completed sessions.
Then, I am showing that data on frontend with some progress bars.
const timeSpend = await User.findOne({username: id}).select('timeSpend');
const sessions = await Session.find({ username: id });
const totalSessions = sessions.length;
const completedSessions = sessions.filter(session => session.status === "completed").length;
return res.status(200).json({ type: "success", timeSpend: timeSpend.timeSpend, totalSessions: totalSessions, completedSessions: completedSessions });
Real-World Usefulness
Better Time Management: MyStudyBuddy helps students manage their time better by automatically creating personalized study schedules.
Calendar View for Planning: The calendar view shows all scheduled study sessions with details, helping students plan their week, make changes, and track progress.
Less Stress: With automated scheduling, notifications, and detailed planning, MyStudyBuddy reduces the stress associated with managing study time. Students can focus more on learning and less on planning.
Consistent Study Habits: The app helps students keep a consistent study routine by sending regular reminders and notifications.
Future Scope
Enhanced Analytics: Expanding the analytics feature to include time spent on each subject, productivity trends, performance comparisons over different periods, and recommendations based on study habits.
Gamification: Adding gamification elements like rewards, badges, and leaderboards to motivate students and make studying more engaging.
Collaborative Features: Introducing features that allow students to form study groups, share study plans, and work together on projects. This can create a sense of community and provide extra support and motivation through peer interaction.
Mobile Application: Creating a mobile app so users can manage their study schedules and receive notifications on the their mobiles.
Conclusion
MyStudyBuddy is more than just a study scheduling app; it's a complete tool designed to change how students approach their studies. It addresses the common problems students face in managing their study time effectively.
Using advanced technologies like Next.js, MongoDB, Gemini AI, and Firebase Messaging, MyStudyBuddy is both powerful and easy to use.
In conclusion, MyStudyBuddy is a comprehensive study scheduling tool that helps students manage their study time effectively. It creates personalized schedules, sends reminders, and provides useful insights through analytics.
Subscribe to my newsletter
Read articles from Hussnain Ahmad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Hussnain Ahmad
Hussnain Ahmad
Psycho Here ;-)