Exploring MySQL Queries: From Basics to Advanced Techniques
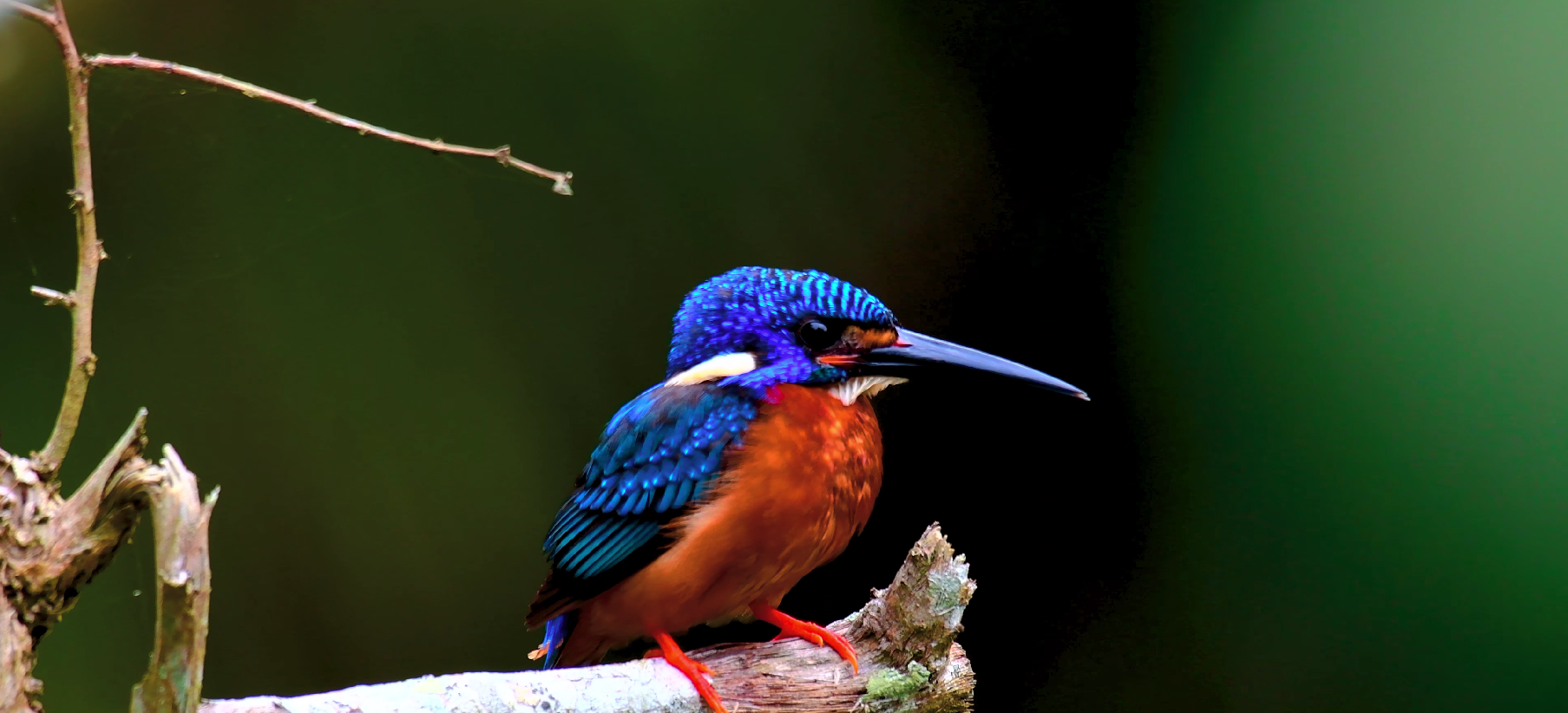
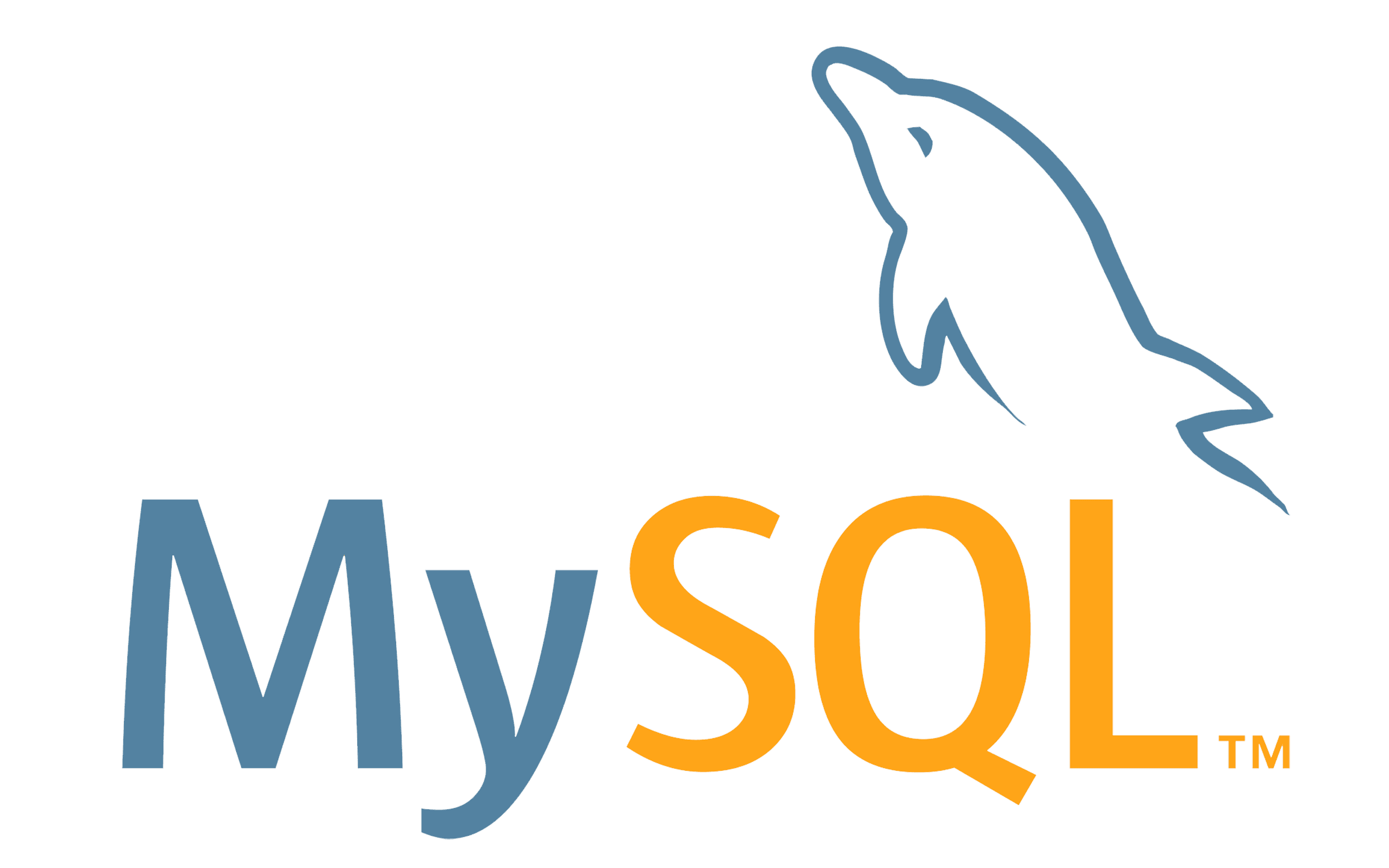
MySQL is a powerful open-source database management system that is especially popular for web applications. It uses Structured Query Language (SQL) for managing and manipulating data. Today, we'll take a journey from the basic to advanced queries in MySQL, illustrating their application with examples.
Basic Queries
SELECT: The SELECT statement is used to select data from a database. The data returned is stored in a result table, sometimes called the result set.
SELECT column1, column2 FROM table_name;
WHERE: The WHERE clause is used to filter records. It is used to extract only those records that fulfill a specified condition.
SELECT column1, column2 FROM table_name WHERE condition;
INSERT INTO: This statement is used to insert new records in a table.
INSERT INTO table_name (column1, column2) VALUES (value1, value2);
UPDATE: The UPDATE statement is used to modify the existing records in a table.
UPDATE table_name SET column1 = value1 WHERE condition;
DELETE: The DELETE statement is used to delete existing records in a table.
DELETE FROM table_name WHERE condition;
Intermediate Queries
JOIN: A JOIN clause is used to combine rows from two or more tables, based on a related column between them.
SELECT Orders.OrderID, Customers.CustomerName
FROM Orders
INNER JOIN Customers ON Orders.CustomerID = Customers.CustomerID;
GROUP BY: The GROUP BY statement groups rows that have the same values in specified columns into summary rows.
SELECT COUNT(CustomerID), Country
FROM Customers
GROUP BY Country;
HAVING: The HAVING clause was added to SQL because the WHERE keyword could not be used with aggregate functions.
SELECT COUNT(CustomerID), Country
FROM Customers
GROUP BY Country
HAVING COUNT(CustomerID) > 5;
Advanced Queries
Subqueries: A subquery is a query nested inside another query and can provide a powerful way to find the data you need.
SELECT column_name
FROM table_name
WHERE column_name IN
(SELECT column_name FROM table_name WHERE condition);
Indexes: Indexes are used to retrieve data from the database more quickly. They are very important for making searches faster.
CREATE INDEX index_name
ON table_name (column1, column2);
Transactions: Transactions are a sequence of one or more SQL operations treated as a unit. They are important for maintaining the integrity of data.
START TRANSACTION;
INSERT INTO table_name VALUES(value1, value2);
UPDATE table_name SET column_name = value WHERE condition;
COMMIT;
Demonstrating the Application of Queries
Let's consider a simple table Employees
with columns ID
, Name
, Position
, and Department
.
ID | Name | Position | Department |
1 | John Doe | Software Eng. | IT |
2 | Jane Smith | Project Manager | Marketing |
To select all employees from the IT department, we would use:
SELECT * FROM Employees WHERE Department = 'IT';
To add a new employee to the table:
INSERT INTO Employees (ID, Name, Position, Department) VALUES (3, 'Alice Brown', 'Data Analyst', 'IT');
To update the position of an employee:
UPDATE Employees SET Position = 'Senior Software Eng.' WHERE ID = 1;
To delete an employee from the records:
DELETE FROM Employees WHERE ID = 2;
Understanding and mastering these queries can significantly enhance your ability to work with databases. Whether you're a beginner or an advanced user, there's always more to learn in the world of MySQL. Keep practicing, and soon you'll be writing complex queries with ease. Happy querying!
Subscribe to my newsletter
Read articles from SANTOSH SINGH directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
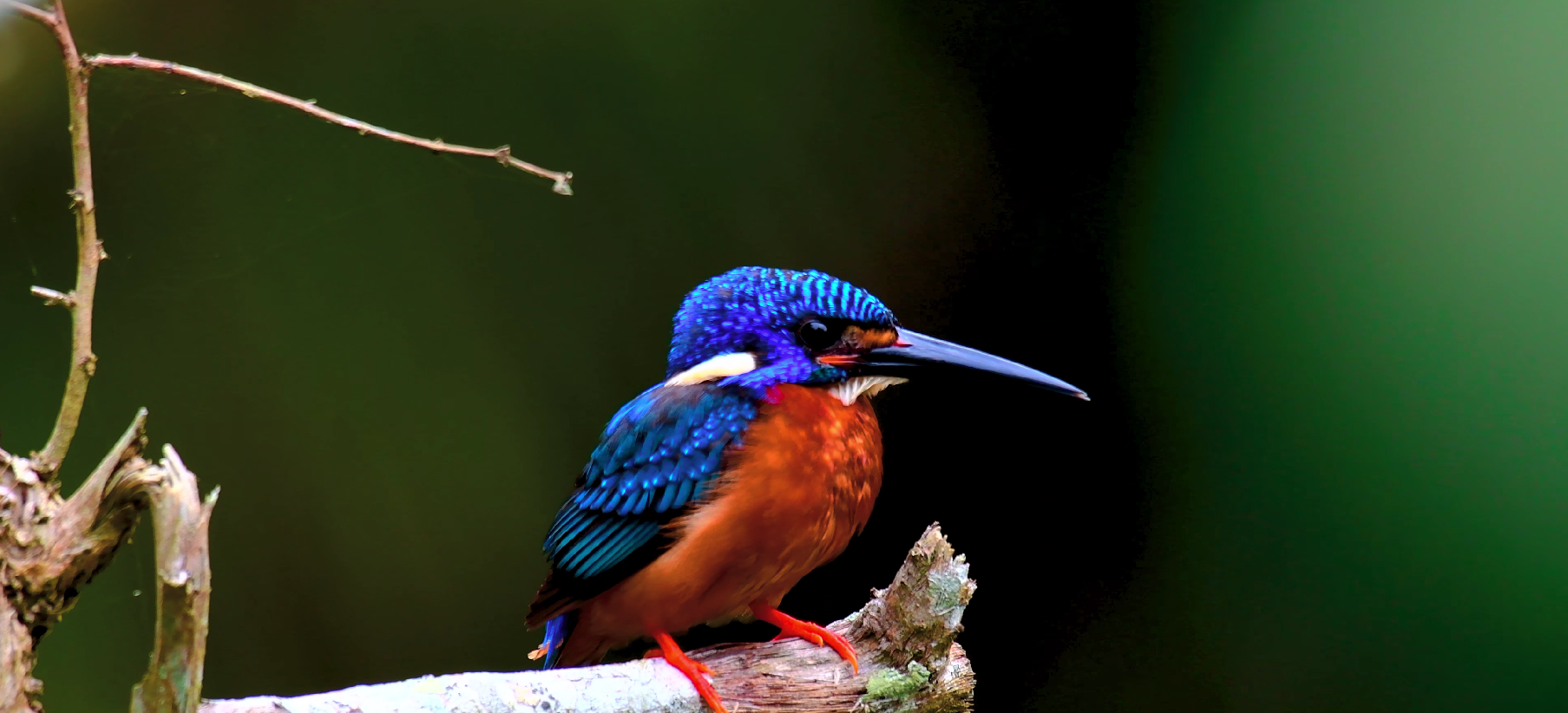