🚀Day 23/180 To check if the number is even or odd using Bitmanipulation...
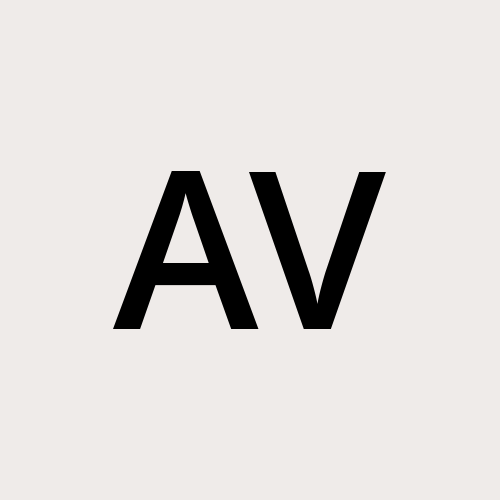
To check if the number is even or odd using Bitmanipulation...
// Now using the concept of BitManipulation we can find if the number is even or odd
// using the & operator
// So, the concept here is ==> Even & ==> then 0 at last
// Odd & ==> then 1 at last ...
import java.util.*;
public class BitManipulation {
public static String evenOdd(int n){
// I guesss
int bitMask =1;
if ((n & bitMask)==1){
return "Odd";
}
else {
return "Even";
}
}
public static void main (String args []){
Scanner scanner = new Scanner(System.in);
System.out.println ("Enter the number for which you want to check if it is even or odd");
int num = scanner.nextInt();
System.out.println(evenOdd(num));
}
}
/*
PS C:\Users\HP\OneDrive\Coding\Java\Practice01> javac BitManipulation.java
PS C:\Users\HP\OneDrive\Coding\Java\Practice01> java BitManipulation
Enter the number for which you want to check if it is even or odd
45
Odd
Let's go through a dry run of the evenOdd
method to understand how it determines if a number is even or odd using bit manipulation.
Here's the method:
public static String evenOdd(int n) {
int bitMask = 1;
if ((n & bitMask) == 1) {
return "Odd";
} else {
return "Even";
}
}
Explanation
The
&
operator is a bitwise AND operator.A bitmask is used to isolate a specific bit of a number. In this case,
bitMask = 1
which in binary is0001
.When
n
is ANDed withbitMask
, it checks the least significant bit (the rightmost bit) ofn
.If the least significant bit is
1
, the number is odd.If the least significant bit is
0
, the number is even.
Dry Run
Let's consider the example input 45
.
Convert
45
to binary:- Decimal
45
is101101
in binary.
- Decimal
Apply the bitmask:
Bitmask
0001
45
in binary101101
Perform the bitwise AND operation:
101101
& 000001
000001
- The result is `1`, which means the number is odd.
Method logic:
The condition
(n & bitMask) == 1
evaluates totrue
because45 & 1
is1
.The method returns
"Odd"
.
Testing the Code
When you run the code and input 45
, it correctly prints Odd
.
Here's the complete program for reference:
import java.util.*;
public class BitManipulation {
public static String evenOdd(int n) {
int bitMask = 1;
if ((n & bitMask) == 1) {
return "Odd";
} else {
return "Even";
}
}
public static void main(String args[]) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter the number for which you want to check if it is even or odd:");
int num = scanner.nextInt();
System.out.println(evenOdd(num));
}
}
This code correctly uses bit manipulation to determine if a number is even or odd.
Subscribe to my newsletter
Read articles from Aniket Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
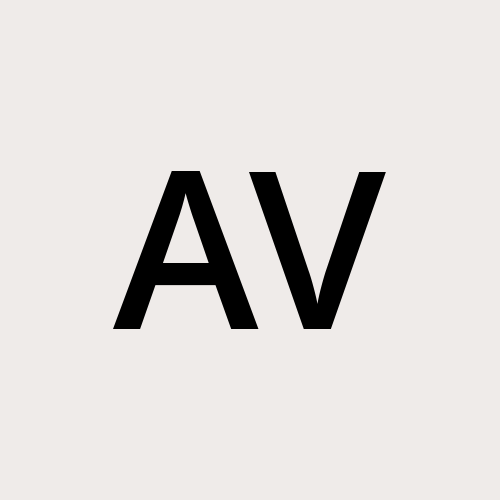