Understanding Retrieval-Augmented Generation (RAG) and Fine-Tuning Approaches

Table of contents
- 1. Introduction
- 2. Understanding Retrieval-Augmented Generation (RAG) Models
- 3. Dense Passage Retrieval (DPR)
- 4. Implementing Dense Passage Retrieval (DPR)
- 5. Code Implementation: Dense Passage Retrieval
- 6. Benefits of Dense Passage Retrieval (DPR)
- 7. Hybrid Retrieval Methods
- 8. Implementing Hybrid Retrieval Methods
- 9. Code Implementation: Hybrid Retrieval Method
- 10. Understanding Pinecone Vector Database
- 11. Implementing Pinecone Vector Database in DPR
- 12. Fine-Tuning Language Models
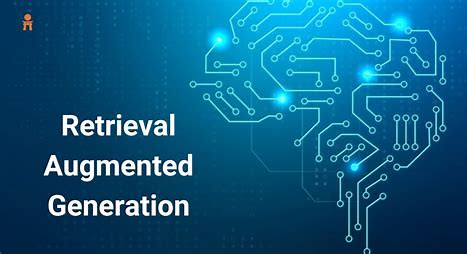
1. Introduction
Retrieval-Augmented Generation (RAG) models combine retrieval and generation techniques to improve the accuracy and relevance of generated responses in question-answering contexts. By leveraging both retrieval and generation, RAG models can provide more precise and contextually appropriate answers. While RAG models significantly enhance performance, optimizing these models can lead to even better results. This document outlines two innovative techniques for optimizing RAG models: Dense Passage Retrieval (DPR) and Hybrid Retrieval Methods. The goal is to leverage the strengths of each approach to achieve superior performance and accuracy in RAG models.
2. Understanding Retrieval-Augmented Generation (RAG) Models
Definition and Components of RAG Models
RAG models are designed to handle question-answering tasks by integrating two key components:
Retrieval Component: This part of the model fetches relevant passages or documents from a large corpus based on the given query.
Generation Component: Using the retrieved passages, this component generates a coherent and contextually relevant response to the query.
How RAG Models Work in Question-Answering Contexts
Query Processing: The model first processes the user's query to understand the context and intent.
Retrieval Step: The processed query is then used to retrieve relevant passages from a pre-indexed knowledge base. This retrieval can be done using dense or sparse methods.
Passage Encoding: The retrieved passages are encoded to represent their semantic content.
Generation Step: The encoded passages are then fed into a generative model that constructs a response, integrating the information from the retrieved documents.
Response Delivery: The final step involves delivering the generated response to the user.
Benefits of Using RAG Models
Enhanced Contextual Understanding: By combining retrieval with generation, RAG models can leverage extensive knowledge bases to provide more accurate and contextually relevant answers.
Scalability: RAG models can handle large corpora of documents, making them suitable for a wide range of applications.
Flexibility: These models can be fine-tuned for specific tasks, allowing for customization based on the particular needs of the application.
3. Dense Passage Retrieval (DPR)
Overview of Dense Passage Retrieval
Dense Passage Retrieval (DPR) is an advanced retrieval technique that uses dense vectors to capture the semantic meaning of passages. Unlike traditional sparse retrieval methods, such as TF-IDF and BM25, which rely on keyword matching, DPR models are trained to understand the deeper semantic relationships between words and passages.
Differences Between Dense and Sparse Retrieval Methods
Sparse Retrieval: Methods like TF-IDF and BM25 represent documents and queries as sparse vectors based on word frequencies. These methods are fast but may miss relevant documents that do not share exact keywords with the query.
Dense Retrieval: DPR represents documents and queries as dense vectors in a continuous vector space. This allows the model to capture semantic similarities even when the exact keywords are not present, leading to more relevant retrieval results.
Benefits of DPR in Improving Semantic Understanding
Semantic Matching: DPR excels at understanding the context and meaning behind the words, leading to more accurate retrieval of relevant passages.
Higher Relevance: By focusing on semantic content rather than keyword matching, DPR can retrieve passages that are contextually relevant, even if they use different vocabulary.
Improved Accuracy: The enhanced semantic understanding provided by DPR leads to better context retrieval, which in turn improves the accuracy of the generated answers.
4. Implementing Dense Passage Retrieval (DPR)
Pre-trained Models for DPR
DPR utilizes pre-trained models from Hugging Face's Transformers library, specifically designed for question encoding and passage encoding.
from transformers import DPRQuestionEncoder, DPRContextEncoder, DPRQuestionEncoderTokenizer, DPRContextEncoderTokenizer
# Load pre-trained models and tokenizers
question_encoder = DPRQuestionEncoder.from_pretrained("facebook/dpr-question_encoder-single-nq-base")
context_encoder = DPRContextEncoder.from_pretrained("facebook/dpr-ctx_encoder-single-nq-base")
question_tokenizer = DPRQuestionEncoderTokenizer.from_pretrained("facebook/dpr-question_encoder-single-nq-base")
context_tokenizer = DPRContextEncoderTokenizer.from_pretrained("facebook/dpr-ctx_encoder-single-nq-base")
Encoding Passages with DPR
Passages in the knowledge base are encoded using the context encoder, and these embeddings are stored in the Pinecone index.
def encode_passages(passages):
inputs = context_tokenizer(passages, return_tensors='pt', padding=True, truncation=True)
embeddings = context_encoder(**inputs).pooler_output.detach().numpy()
return embeddings
passages = ["Your text data here..."]
passage_embeddings = encode_passages(passages)
vectors = [{"id": str(i), "values": embedding.tolist(), "metadata": {"context": passage}} for i, (passage, embedding) in enumerate(zip(passages, passage_embeddings))]
index.upsert(vectors)
Query Encoding and Retrieval
Queries are encoded using the question encoder, and relevant passages are retrieved from the Pinecone index.
def retrieve_relevant_docs_dpr(query):
inputs = question_tokenizer(query, return_tensors='pt', padding=True, truncation=True)
query_embedding = question_encoder(**inputs).pooler_output.detach().numpy()[0]
result = index.query(vector=query_embedding.tolist(), top_k=5, include_metadata=True)
return [match['metadata']['context'] for match in result['matches']]
5. Code Implementation: Dense Passage Retrieval
Sample Code for Implementing DPR
Here's the complete code for implementing DPR, integrating the steps mentioned above.
from transformers import DPRQuestionEncoder, DPRContextEncoder, DPRQuestionEncoderTokenizer, DPRContextEncoderTokenizer
from pinecone import Pinecone
import numpy as np
# Initialize Pinecone
pinecone.init(api_key="your-pinecone-api-key")
index_name = 'your-index-name'
if index_name not in pinecone.list_indexes():
pinecone.create_index(index_name, dimension=768, metric='cosine')
index = pinecone.Index(index_name)
# Load pre-trained models and tokenizers
question_encoder = DPRQuestionEncoder.from_pretrained("facebook/dpr-question_encoder-single-nq-base")
context_encoder = DPRContextEncoder.from_pretrained("facebook/dpr-ctx_encoder-single-nq-base")
question_tokenizer = DPRQuestionEncoderTokenizer.from_pretrained("facebook/dpr-question_encoder-single-nq-base")
context_tokenizer = DPRContextEncoderTokenizer.from_pretrained("facebook/dpr-ctx_encoder-single-nq-base")
def encode_passages(passages):
inputs = context_tokenizer(passages, return_tensors='pt', padding=True, truncation=True)
embeddings = context_encoder(**inputs).pooler_output.detach().numpy()
return embeddings
passages = ["Your text data here..."]
passage_embeddings = encode_passages(passages)
vectors = [{"id": str(i), "values": embedding.tolist(), "metadata": {"context": passage}} for i, (passage, embedding) in enumerate(zip(passages, passage_embeddings))]
index.upsert(vectors)
def retrieve_relevant_docs_dpr(query):
inputs = question_tokenizer(query, return_tensors='pt', padding=True, truncation=True)
query_embedding = question_encoder(**inputs).pooler_output.detach().numpy()[0]
result = index.query(vector=query_embedding.tolist(), top_k=5, include_metadata=True)
return [match['metadata']['context'] for match in result['matches']]
Explanation of the Code Snippets
Loading Pre-trained Models: The code initializes and loads pre-trained DPR models and their respective tokenizers.
Encoding Passages: Passages from the knowledge base are encoded using the context encoder. These encoded vectors are then upserted into the Pinecone index.
Query Encoding and Retrieval: User queries are encoded using the question encoder. The encoded query is used to search the Pinecone index for the top relevant passages.
6. Benefits of Dense Passage Retrieval (DPR)
Dense Passage Retrieval (DPR) offers several significant benefits that enhance the performance of Retrieval-Augmented Generation (RAG) models.
Enhanced Semantic Understanding
Deeper Contextual Grasp: Unlike traditional retrieval methods that rely on keyword matching, DPR models understand the context and semantic meaning of passages. This capability allows them to retrieve information that is contextually relevant, even if the exact keywords are not present.
Improved Response Quality: By focusing on semantic similarities, DPR ensures that the retrieved passages are more aligned with the intent of the query, leading to higher quality and more accurate responses.
Higher Accuracy
Precision in Retrieval: DPR models are trained to capture semantic nuances, which enhances their ability to fetch the most pertinent passages from a large corpus. This precision results in more accurate and relevant answers.
Reduction of Noise: Traditional methods often retrieve irrelevant documents that match the keywords but not the context. DPR minimizes such noise, improving the overall accuracy of the retrieval process.
7. Hybrid Retrieval Methods
Combining sparse and dense retrieval methods leverages the strengths of both approaches to optimize the performance of RAG models.
Overview of Hybrid Retrieval Methods
Hybrid retrieval methods integrate both sparse (e.g., BM25) and dense (e.g., DPR) retrieval techniques. This combination allows the system to quickly filter a large corpus using sparse methods and then refine the results using dense methods.
Benefits of the Hybrid Approach
Efficiency: Sparse retrieval methods like BM25 can rapidly narrow down the corpus to a manageable number of passages. This initial filtering step is computationally efficient and sets the stage for more detailed dense retrieval.
Improved Relevance: Once the corpus is reduced, dense retrieval methods like DPR can refine the results by focusing on semantic content. This two-step process enhances the relevance and accuracy of the final retrieved passages.
8. Implementing Hybrid Retrieval Methods
Initial Retrieval with BM25
BM25 is a well-known algorithm for sparse retrieval that ranks documents based on their relevance to a query using term frequency and inverse document frequency.
Introduction to BM25 Algorithm
Term Frequency (TF): The number of times a term appears in a document.
Inverse Document Frequency (IDF): Measures how common or rare a term is across all documents.
Relevance Score: BM25 calculates a relevance score for each document based on the TF and IDF of the query terms.
Using BM25 for Initial Passage Retrieval
from rank_bm25 import BM25Okapi
from sklearn.feature_extraction.text import CountVectorizer
# Sample corpus
corpus = ["Your text data here..."]
vectorizer = CountVectorizer().fit(corpus)
bm25 = BM25Okapi(vectorizer.transform(corpus).toarray())
def retrieve_with_bm25(query, top_k=50):
query_vector = vectorizer.transform([query]).toarray()[0]
scores = bm25.get_scores(query_vector)
top_indices = np.argsort(scores)[::-1][:top_k]
return [corpus[i] for i in top_indices]
Refinement with DPR
Once the initial set of passages is retrieved using BM25, DPR can be used to further refine these passages by leveraging its deep semantic understanding.
Combining BM25 and DPR for Better Results
def hybrid_retrieve(query):
# Initial retrieval with BM25
initial_passages = retrieve_with_bm25(query)
# Encoding passages using DPR
passage_embeddings = encode_passages(initial_passages)
# Encoding the query using DPR
inputs = question_tokenizer(query, return_tensors='pt', padding=True, truncation=True)
query_embedding = question_encoder(**inputs).pooler_output.detach().numpy()[0]
# Calculating similarity scores
scores = np.dot(passage_embeddings, query_embedding)
top_indices = np.argsort(scores)[::-1][:5]
return [initial_passages[i] for i in top_indices]
9. Code Implementation: Hybrid Retrieval Method
Sample Code for Hybrid Retrieval Method
Below is the complete code for implementing a hybrid retrieval method that integrates BM25 and DPR.
from transformers import DPRQuestionEncoder, DPRContextEncoder, DPRQuestionEncoderTokenizer, DPRContextEncoderTokenizer
from pinecone import Pinecone
import numpy as np
from rank_bm25 import BM25Okapi
from sklearn.feature_extraction.text import CountVectorizer
# Initialize Pinecone
pinecone.init(api_key="your-pinecone-api-key")
index_name = 'your-index-name'
if index_name not in pinecone.list_indexes():
pinecone.create_index(index_name, dimension=768, metric='cosine')
index = pinecone.Index(index_name)
# Load pre-trained models and tokenizers
question_encoder = DPRQuestionEncoder.from_pretrained("facebook/dpr-question_encoder-single-nq-base")
context_encoder = DPRContextEncoder.from_pretrained("facebook/dpr-ctx_encoder-single-nq-base")
question_tokenizer = DPRQuestionEncoderTokenizer.from_pretrained("facebook/dpr-question_encoder-single-nq-base")
context_tokenizer = DPRContextEncoderTokenizer.from_pretrained("facebook/dpr-ctx_encoder-single-nq-base")
# BM25 setup
corpus = ["Your text data here..."]
vectorizer = CountVectorizer().fit(corpus)
bm25 = BM25Okapi(vectorizer.transform(corpus).toarray())
def encode_passages(passages):
inputs = context_tokenizer(passages, return_tensors='pt', padding=True, truncation=True)
embeddings = context_encoder(**inputs).pooler_output.detach().numpy()
return embeddings
def retrieve_with_bm25(query, top_k=50):
query_vector = vectorizer.transform([query]).toarray()[0]
scores = bm25.get_scores(query_vector)
top_indices = np.argsort(scores)[::-1][:top_k]
return [corpus[i] for i in top_indices]
def hybrid_retrieve(query):
# Initial retrieval with BM25
initial_passages = retrieve_with_bm25(query)
# Encoding passages using DPR
passage_embeddings = encode_passages(initial_passages)
# Encoding the query using DPR
inputs = question_tokenizer(query, return_tensors='pt', padding=True, truncation=True)
query_embedding = question_encoder(**inputs).pooler_output.detach().numpy()[0]
# Calculating similarity scores
scores = np.dot(passage_embeddings, query_embedding)
top_indices = np.argsort(scores)[::-1][:5]
return [initial_passages[i] for i in top_indices]
Explanation of the Code Snippets
BM25 Retrieval: The code initializes a BM25 retriever using a vectorized corpus. The
retrieve_with_bm25
function performs an initial retrieval to get a manageable number of passages.DPR Refinement: The code then uses DPR to encode the retrieved passages and the query. It calculates similarity scores to refine the initial retrieval results and fetch the most relevant passages.
10. Understanding Pinecone Vector Database
Overview of Pinecone Vector Database
Pinecone is a fully managed vector database optimized for high-performance, scalable similarity search and vector operations. It is designed to handle large-scale vector data, providing an efficient way to store, index, and query vectors.
How Pinecone Handles Vector Storage and Retrieval
Vector Storage: Pinecone stores vectors in a distributed, scalable manner. Each vector is represented as a point in a high-dimensional space, and similar vectors are located close to each other in this space.
Indexing: Pinecone uses advanced indexing techniques to organize and manage vectors. These indices allow for fast and accurate retrieval of vectors based on similarity search.
Querying: Pinecone supports efficient querying of vectors, enabling real-time similarity search. Users can query the database with a vector, and Pinecone returns the most similar vectors from the stored data.
Integration of Pinecone with DPR in the Provided Code
Pinecone is integrated into the DPR implementation to store encoded passage vectors and facilitate efficient retrieval. The process involves encoding passages using DPR and storing the resulting vectors in a Pinecone index. Queries are encoded similarly, and Pinecone is used to find the most relevant passages based on vector similarity.
11. Implementing Pinecone Vector Database in DPR
Setting Up Pinecone API
To use Pinecone, you need to set up the Pinecone API and create an index for storing vectors.
!pip install -qU sentence-transformers pinecone-client transformers
from pinecone import Pinecone
import numpy as np
import os
from sentence_transformers import SentenceTransformer
from transformers import pipeline, AutoModelForCausalLM, AutoTokenizer
# Initialize Pinecone
pc = Pinecone(api_key="your-pinecone-api-key")
index_name = 'qa-bot-384'
if index_name not in [index['name'] for index in pc.list_indexes()]:
pc.create_index(
name=index_name,
dimension=384,
metric='cosine',
spec=ServerlessSpec(
cloud='aws',
region='us-east-1' # Using AWS region as per Pinecone's quickstart guide
)
)
index = pc.Index(index_name)
Creating and Configuring an Index
When creating an index, you specify the dimensions of the vectors and the metric for similarity (e.g., cosine similarity). Pinecone allows for flexible configuration based on your needs.
Upserting Vectors into the Pinecone Index
Encoded vectors are upserted (inserted or updated) into the Pinecone index, allowing for efficient storage and retrieval.
def encode_passages(passages):
inputs = context_tokenizer(passages, return_tensors='pt', padding=True, truncation=True)
embeddings = context_encoder(**inputs).pooler_output.detach().numpy()
return embeddings
passages = ["Your text data here..."]
passage_embeddings = encode_passages(passages)
# Create a list of vectors to upsert into the Pinecone index
vectors = [{"id": str(i), "values": embedding.tolist(), "metadata": {"context": passage}} for i, (passage, embedding) in enumerate(zip(passages, passage_embeddings))]
# Upsert vectors into the Pinecone index
index.upsert(vectors)
Querying Pinecone for Relevant Vectors
When a query is made, it is encoded into a vector, and Pinecone is used to find the most similar vectors in the index.
def retrieve_relevant_docs_dpr(query):
inputs = question_tokenizer(query, return_tensors='pt', padding=True, truncation=True)
query_embedding = question_encoder(**inputs).pooler_output.detach().numpy()[0]
result = index.query(vector=query_embedding.tolist(), top_k=5, include_metadata=True)
return [match['metadata']['context'] for match in result['matches']]
Performing Efficient Vector Searches
Pinecone's indexing and querying capabilities ensure that vector searches are efficient, even with large datasets. The combination of fast retrieval and high accuracy makes Pinecone an excellent choice for enhancing RAG models.
12. Fine-Tuning Language Models
Importance of Fine-Tuning
Fine-tuning language models is crucial for adapting pre-trained models to specific tasks or domains. This process involves training the model on a task-specific dataset to improve its performance and accuracy in that context.
Techniques for Preparing High-Quality Datasets
Data Collection
Diversity: Collect data from various sources like books, articles, websites, and social media to ensure the model can handle diverse contexts.
Relevance: Ensure the data is closely related to the task. For instance, for a question-answering task, include datasets with questions and corresponding answers.
Volume: Gather a substantial amount of data, ensuring it is diverse and not redundant.
Data Cleaning
Removing Noise: Eliminate irrelevant or low-quality data, such as broken sentences, incorrect information, and data with excessive noise (e.g., advertisements).
Normalization: Standardize text by converting it to lowercase, removing special characters, and normalizing whitespace.
Tokenization: Properly tokenize the text into words, subwords, or characters based on the model's requirements.
Data Labeling
Manual Labeling: Ensure high accuracy by manually labeling critical datasets, despite being time-consuming.
Automated Labeling: Use pre-trained models for automated labeling to save time, with manual review to ensure quality.
Data Augmentation
Synonym Replacement: Increase dataset size and variety by replacing words with their synonyms.
Back Translation: Generate paraphrased versions by translating text to another language and back to the original language.
Noise Injection: Introduce minor errors, such as spelling mistakes, to make the model robust to noisy inputs.
Balancing the Dataset
Class Balance: Ensure even distribution of classes in classification tasks to prevent bias towards the majority class.
Length Balance: Include examples of varying lengths to help the model handle inputs of different sizes.
Validation and Testing
Holdout Sets: Create separate validation and testing sets to evaluate the model's performance.
Cross-Validation: Use cross-validation techniques to assess the model's performance across different subsets of data.
Subscribe to my newsletter
Read articles from Debanjan Chakraborty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
