Patterns ;0
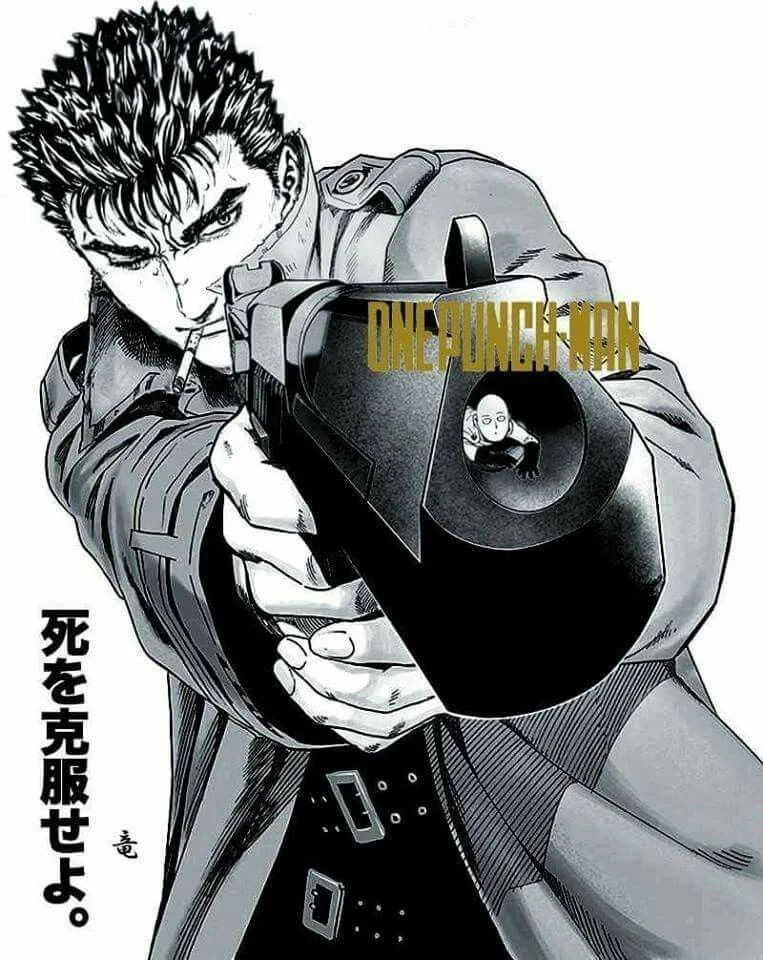
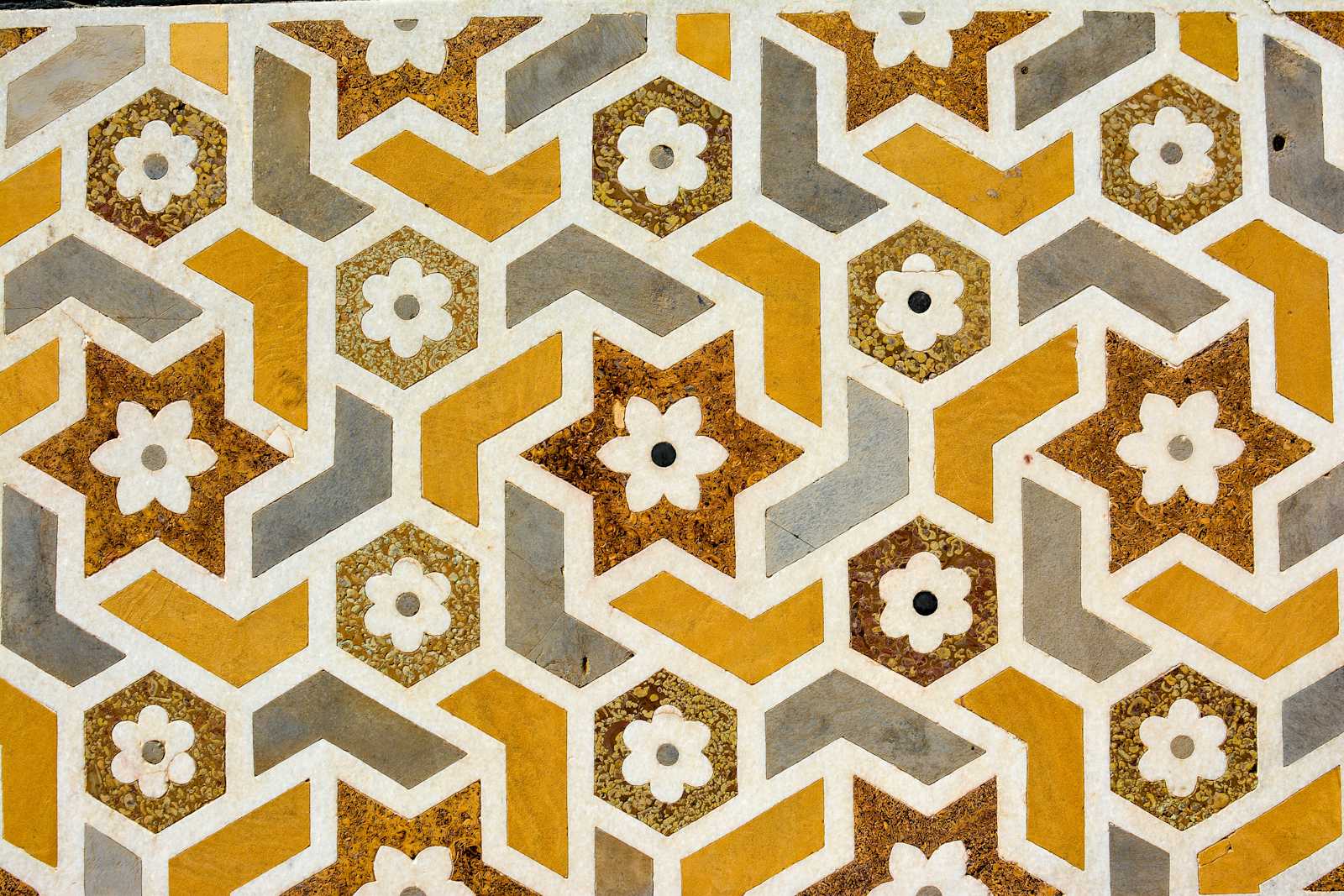
Introduction
Hello, fellow coders! I'm excited to share my journey in mastering Data Structures and Algorithms (DSA) with you. Recently, I embarked on a speedrun through TakeYouForward's comprehensive DSA course, and I’ve just completed the Patterns section. This blog aims to document my experiences, insights, and tips to help you on your DSA learning journey.
My Algo Notebook
Patterns Section
My Experience
The Patterns ;) section was both challenging and rewarding. It involved solving a variety of pattern-based problems that require a strong grasp of nested loops and logic. Initially, some patterns seemed intricate, but with practice and the right approach, they became more manageable. Here's a breakdown of how I approached this section:
Understanding the Problem Statement: Each pattern problem started with understanding the exact output expected. Visualizing the pattern was crucial.
Breaking Down the Logic: For each row and column, I formulated the logic that governs the placement of characters.
Implementing Nested Loops: Most patterns required nested loops, where the outer loop controlled the rows and the inner loop handled the columns.
Four General Rules for Solving Pattern-Based Questions
Nested Loops for Printing Patterns
Always use nested loops for printing patterns. The outer loop handles the number of lines/rows, while the inner loop manages the columns. For example:
for (int i = 0; i < rows; i++) { for (int j = 0; j < columns; j++) { // print pattern } }
Connect Columns to Rows
- Formulate a logic that connects the number of columns to the rows. For each row, determine the required number of columns to be printed. For instance, if the first row has 3 columns, identify the relationship between rows and columns.
Print Inside the Inner Loop
- The actual printing of characters (like
*
) happens inside the inner loop. Ensure that the logic for placement is accurate to achieve the desired pattern.
- The actual printing of characters (like
Observe Symmetry
- Many patterns exhibit symmetry. Identifying and leveraging this symmetry can simplify your logic. Additionally, some patterns are combinations of two or more simpler patterns, so breaking them down can make them easier to solve.
Challenges and Solutions
One of the main challenges was optimizing the code to handle edge cases and large inputs efficiently. Here are some techniques I found helpful:
Merging Loops: Whenever possible, I merged loops to make the code more concise and reduce runtime.
Debugging with Line Numbers: Assigning line numbers and using placeholders (like dashes instead of spaces) during debugging helped track the flow of the program.
Handling Edge Cases: Special cases like n = 1 or n = 0 were handled separately to avoid unnecessary computations.
Tips and Tricks
Here are some tips that made the patterns section easier to tackle:
Use Functions Instead of
int main()
- In online contests and compilers, it's often more efficient to use functions directly rather than relying on
int main()
. This can help streamline your code and make it more modular.
- In online contests and compilers, it's often more efficient to use functions directly rather than relying on
Break Down and Debug Efficiently
- Assign line numbers and carefully observe both test cases to identify patterns. Breaking down complex problems into smaller, manageable parts makes debugging faster and easier.
Merge Loops
- Whenever possible, merge loops to make your code more concise. For instance, if you have separate loops for odd and even rows, consider combining them to reduce runtime and improve space complexity.
Simplify Conditions
- Simplify your conditional statements to avoid extra cases. For example, use
else if (i1 % 2 != 0)
instead of multiple if-else statements to streamline your logic.
- Simplify your conditional statements to avoid extra cases. For example, use
Combine Codes
- Look for opportunities to combine similar logic or repeated code. For example, if you have
4+9
, consider combining them into a single calculation or function call to reduce redundancy.
- Look for opportunities to combine similar logic or repeated code. For example, if you have
Write Logic in Steps
When developing your logic, write it out step-by-step, such as:
outer loop inner loop space star space
This helps you clearly visualize and implement the pattern or logic.
Handle Edge Cases Separately
Always take care of critical points like
n=1
orn=0
separately to avoid unnecessary computations and potential errors. For example:if (n == 1) { cout << 1; } else { // whole code }
Optimize Variable Usage
- Reduce the number of variables by reusing existing ones whenever possible. This can have a major impact on the loop performance and overall efficiency of your code.
Debug with Placeholders
- Use placeholders like dashes instead of spaces during debugging to track the flow of your program more effectively.
Practice and Play
- Start with smaller problems and gradually work your way up. Practice is key, so play around with different problems and keep debugging to improve your skills.
Insights and Learnings
This section reinforced the importance of a methodical approach to problem-solving. I learned to:
Break Down Problems: Decompose complex problems into smaller, manageable parts.
Think Efficiently: Always consider the runtime and space complexity of your solutions.
Debug Quickly: Efficient debugging techniques are crucial for success in programming contests.
Good Ques ;)
Next Steps
With the Patterns section completed, I’m now moving on to the next segment of the TakeYouForward DSA course. My goal is to continue improving my problem-solving skills and efficiency. Stay tuned for more updates as I progress through the course!
My Algo Notebook
Conclusion
The journey through the Patterns section has been a game-changer for my understanding of nested loops and pattern recognition. I hope these insights and tips help you in your own DSA journey. Happy coding
Subscribe to my newsletter
Read articles from kintsugi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
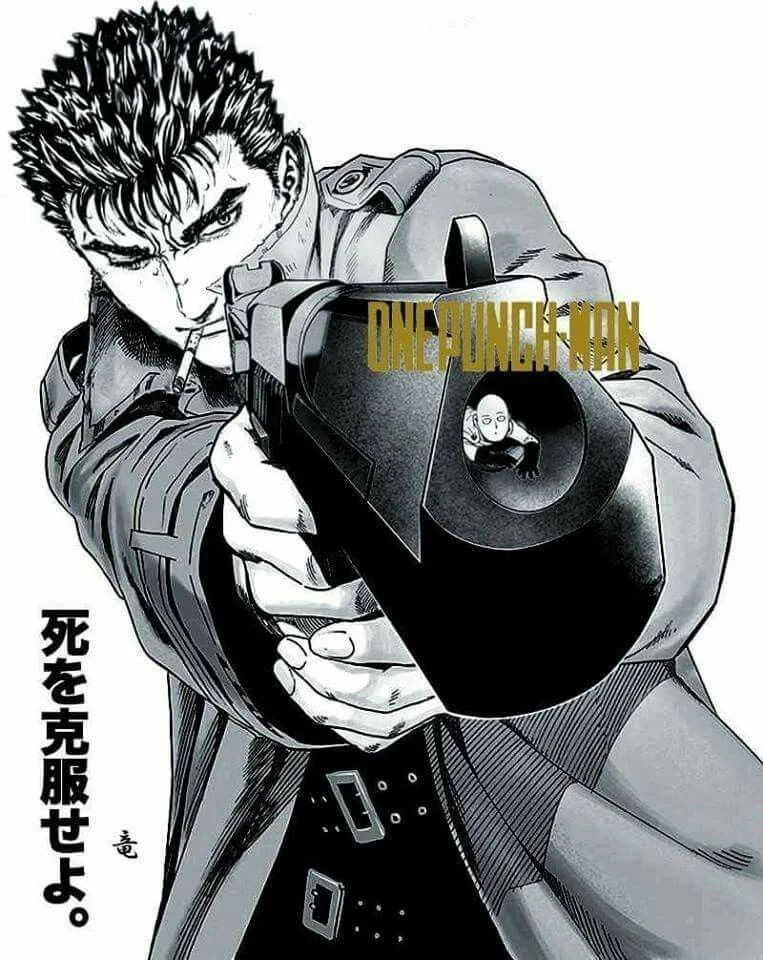
kintsugi
kintsugi
Siddhant Bali, an aspiring tech entrepreneur, is an Undergraduate Research Scholar at IIIT Delhi, currently pursuing a B.Tech in Computer Science Engineering with a focus on design (CSD). Excelling in college activities and event management, Siddhant's entrepreneurial spirit propels him into innovative ventures. Connect on LinkedIn or reach out at siddhant22496@iiitd.ac.in for more info.