JavaScript and TypeScript Bundlers
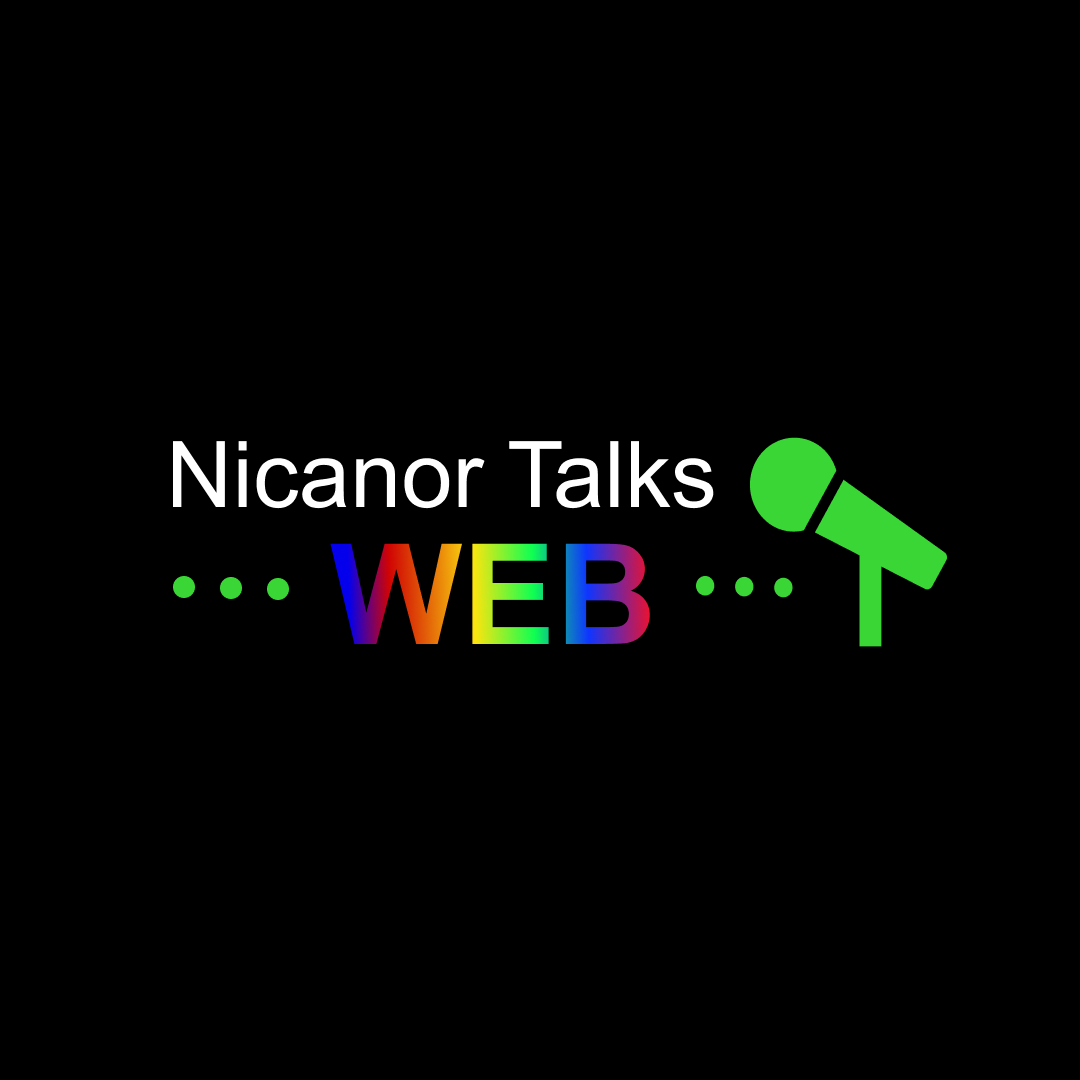
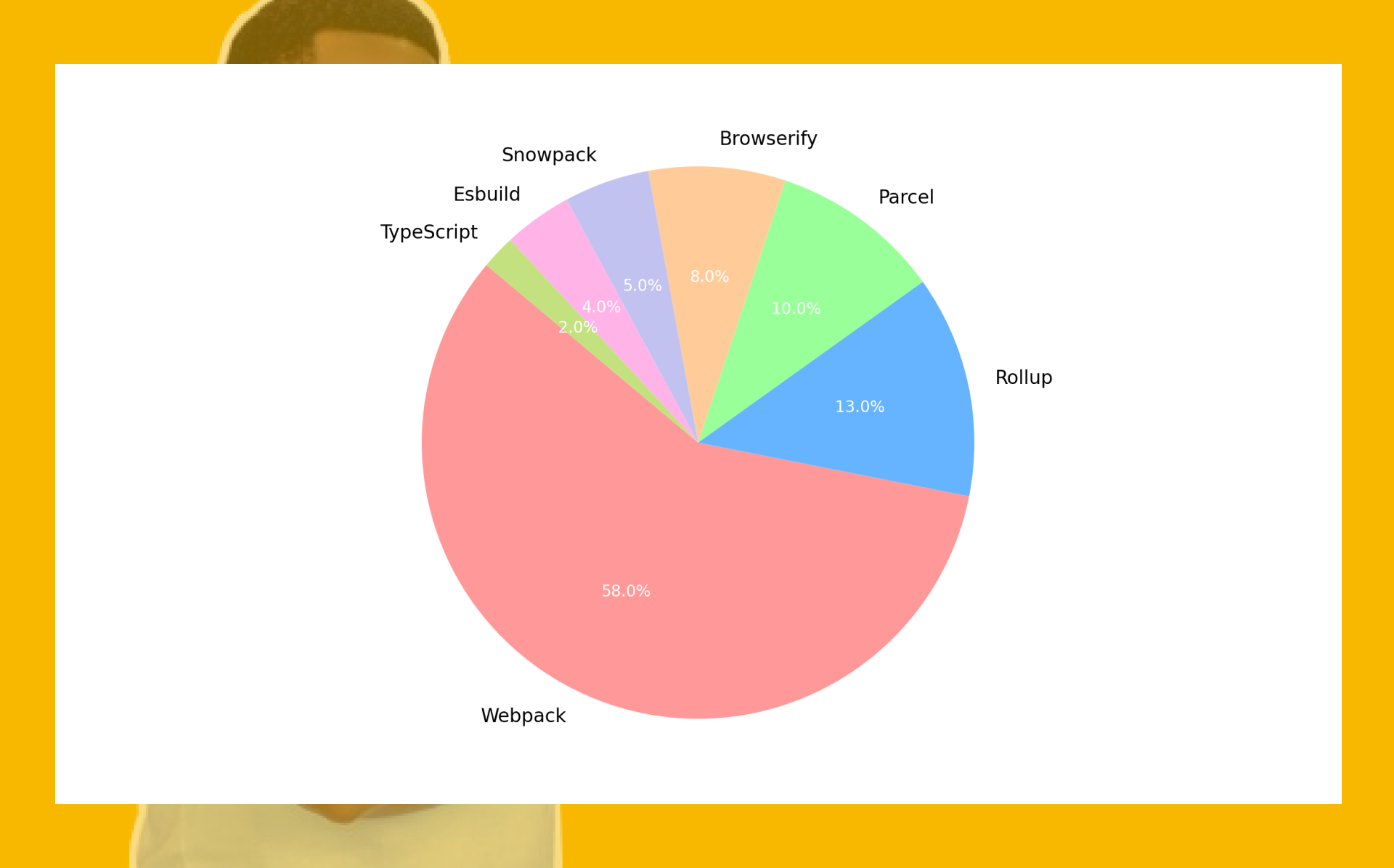
I recently took on a #WeekendBuild challenge idea to create an autocomplete/autosuggestions input package, nicanor-autocomplete. Of course, it seems like a stupid idea, but the end game was learning and building a package-ready product.
In the process, putting this package into a performant ready-to-use app proved to be a challenge and I had to dig around bundling to achieve my goal. This is why am writing the in-depth article, to break down what I did find out about module bundling in JS/TS apps.
What is Bundling?
Bundling is the process of combining multiple JavaScript or TypeScript files into a single file or a set of files. This is done to reduce the number of HTTP requests needed to load a web page, improving the page load time and overall performance. Bundling also helps in managing dependencies, organizing code, and preparing it for production deployment.
Why Do We Need Bundling?
Performance Improvement: By combining multiple files into one or a few files, bundling reduces the number of HTTP requests, leading to faster load times.
Code Organization: Bundlers help manage dependencies and keep code organized, making it easier to maintain and scale applications.
Minification and Optimization: Bundlers often include features for minifying and optimizing code, which reduces file sizes and improves performance.
Transpilation Support: Modern bundlers support transpiling languages like TypeScript or newer JavaScript syntax into browser-compatible JavaScript.
How Bundling Was Done Before Webpack and Other Modern Tools
Before the advent of modern bundlers like Webpack, Rollup, and Parcel, developers used simpler tools and manual processes to manage and optimize their JavaScript files. Some of the earlier methods included:
Manual Concatenation: Developers manually combine JavaScript files into a single file using shell scripts or build tools like Make or Grunt. This method required a lot of manual effort and was prone to errors.
RequireJS: An early tool that introduced the concept of asynchronous module definition (AMD) to manage dependencies and load JavaScript modules dynamically. While it helped with module management, it did not offer comprehensive bundling or optimization features.
Browserify: A tool that allowed developers to use Node.js-style
require
statements in the browser. It made dependency management easier but still lacked some advanced features of modern bundlers like code splitting and hot module replacement.
As web applications grew in complexity, the need for more sophisticated bundling solutions became apparent. Modern bundlers like Webpack emerged to address these challenges by offering a comprehensive set of features for bundling, optimizing, and managing dependencies in large-scale applications.
Some places like Stackoverflow gave sorts of questions and answers e.g.
why do we still need module bundlers when we have native ESM support in browsers now
https://stackoverflow.com/questions/40308674/why-to-use-module-bundlers-over-es6-module
and many others
Let's now dive into the most commonly used module bundlers
1. Webpack
Webpack is a powerful and flexible bundler, widely used for both small and large-scale applications. It provides a rich set of features and plugins that make it ideal for complex applications. Webpack is used together with Babel, to transpile modern JavaScript into browser-compatible versions
webpack.config.js
const path = require('path');
module.exports = {
mode: 'production',
entry: './src/index.js',
output: {
filename: 'bundle.js',
path: path.resolve(__dirname, 'dist'),
},
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: 'babel-loader',
},
{
test: /\.css$/,
use: ['style-loader', 'css-loader'],
},
],
},
plugins: [
// Add any plugins here
],
};
Pros
Feature-Rich: Supports code splitting, hot module replacement, and more.
Community Support: Extensive documentation and plugins.
Flexibility: Highly configurable for different project needs.
Cons
Complexity: This can be overwhelming for small projects due to its complexity.
Configuration: Requires a configuration file which can become complex.
How to Bundle a Production-Ready App using Webpack
Install Webpack and its dependencies:
npm install webpack webpack-cli babel-loader @babel/core @babel/preset-env style-loader css-loader --save-dev
Create
webpack.config.js
as shown above.Build the project:
npx webpack --config webpack.config.js
2. Rollup
Rollup is a module bundler designed for ES6 modules. It is highly efficient in tree-shaking, making it an excellent choice for library bundling.
rollup.config.js
import resolve from '@rollup/plugin-node-resolve';
import commonjs from '@rollup/plugin-commonjs';
import babel from '@rollup/plugin-babel';
import { terser } from 'rollup-plugin-terser';
export default {
input: 'src/index.js',
output: {
file: 'dist/bundle.js',
format: 'cjs',
},
plugins: [
resolve(),
commonjs(),
babel({ babelHelpers: 'bundled' }),
terser()
],
};
Pros
Tree Shaking: Efficiently removes unused code.
Simplicity: Easier configuration for smaller projects.
ES6 Modules: Designed with modern JavaScript in mind.
Cons
Less Suitable for Large Apps: Lacks some advanced features of Webpack.
Plugin Ecosystem: Smaller compared to Webpack.
How to Bundle a Production-Ready App Using Rollup
Install Rollup and its dependencies:
npm install rollup @rollup/plugin-node-resolve @rollup/plugin-commonjs @rollup/plugin-babel rollup-plugin-terser --save-dev
Create
rollup.config.js
as shown above.Build the project:
npx rollup -c rollup.config.js
3. Parcel
Parcel is a zero-configuration bundler that offers a fast and efficient way to bundle applications. It automatically detects and configures the required plugins.
Pros
Zero Configuration: No configuration is needed for most use cases.
Fast: Uses multicore processing and file caching.
Out-of-the-Box Support: Supports a wide range of file types and frameworks.
Cons
Limited Customization: Less flexibility for custom configurations.
Smaller Community: Compared to Webpack and Rollup.
How to Bundle a Production-Ready App
Install Parcel:
npm install parcel --save-dev
Build the project:
npx parcel build src/index.html
4. Browserify
Browserify lets you use require
it in the browser by bundling up all dependencies. It's a classic tool that works well for smaller projects.
package.json
{
"scripts": {
"build": "browserify src/index.js -o dist/bundle.js"
}
}
Pros
Simple: Easy to get started with.
Node Compatibility: Allows using Node modules in the browser.
Cons
Performance: Not as efficient as newer bundlers.
Feature Set: Lacks advanced features like tree-shaking.
How to Bundle a Production-Ready App
Install Browserify:
npm install browserify --save-dev
Add build script
package.json
as shown above.Build the project:
npm run build
5. Snowpack
Snowpack is a lightning-fast frontend build tool, designed for modern web development. It leverages JavaScript’s ES module system to eliminate the need for bundling during development.
snowpack.config.js
module.exports = {
mount: {
src: '/dist',
},
plugins: [
'@snowpack/plugin-babel',
],
buildOptions: {
out: 'build',
},
};
Pros
Speed: Extremely fast due to unbundled development.
Modern: Uses ES modules and supports modern JavaScript out of the box.
Cons
Newer Tool: Smaller ecosystem compared to Webpack.
Bundling: Requires additional configuration for production bundling.
How to Bundle a Production-Ready App
Install Snowpack:
npm install snowpack --save-dev
Create
snowpack.config.js
as shown above.Build the project:
npx snowpack build
6. Esbuild
Esbuild is an extremely fast bundler and minifier. It is written in Go and offers high performance, making it suitable for large-scale applications.
build.js
require('esbuild').build({
entryPoints: ['src/index.js'],
bundle: true,
minify: true,
outfile: 'dist/bundle.js',
}).catch(() => process.exit(1));
Pros
Performance: Extremely fast build times.
Simplicity: Simple API for bundling and minifying.
Cons
Features: Still evolving, with fewer features compared to Webpack.
Community: Smaller community and plugin ecosystem.
How to Bundle a Production-Ready App
Install Esbuild:
npm install esbuild --save-dev
Create
build.js
as shown above.Build the project:
node build.js
7. TypeScript
TypeScript can be used directly to compile TypeScript code into JavaScript. It integrates well with other bundlers to add type safety to your projects.
tsconfig.json
{
"compilerOptions": {
"target": "es5",
"module": "commonjs",
"outDir": "./dist",
"rootDir": "./src",
"strict": true,
"esModuleInterop": true,
"skipLibCheck": true,
"forceConsistentCasingInFileNames": true
}
}
Pros
Type Safety: Provides static type-checking to reduce bugs.
Modern JavaScript: Supports modern JavaScript features.
Cons
Learning Curve: Requires learning TypeScript if you're not familiar.
Configuration: Needs a
tsconfig.json
file for configuration.
How to Bundle a Production-Ready App
Install TypeScript:
npm install typescript --save-dev
Create
tsconfig.json
as shown above.Compile TypeScript to JavaScript:
npx tsc
Choosing the right bundler depends on your project's needs:
For complex applications with extensive requirements, Webpack is a powerful choice
Rollup is excellent for libraries and smaller projects due to its efficient tree-shaking
Parcel offers a zero-configuration setup, making it ideal for quick development.
Browserify is simple and works well for Node-based project
Snowpack provides a modern, fast development experience
Esbuild offers unmatched performance
TypeScript adds type safety and integrates well with other bundlers.
Subscribe to my newsletter
Read articles from Nicanor Talks Web directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
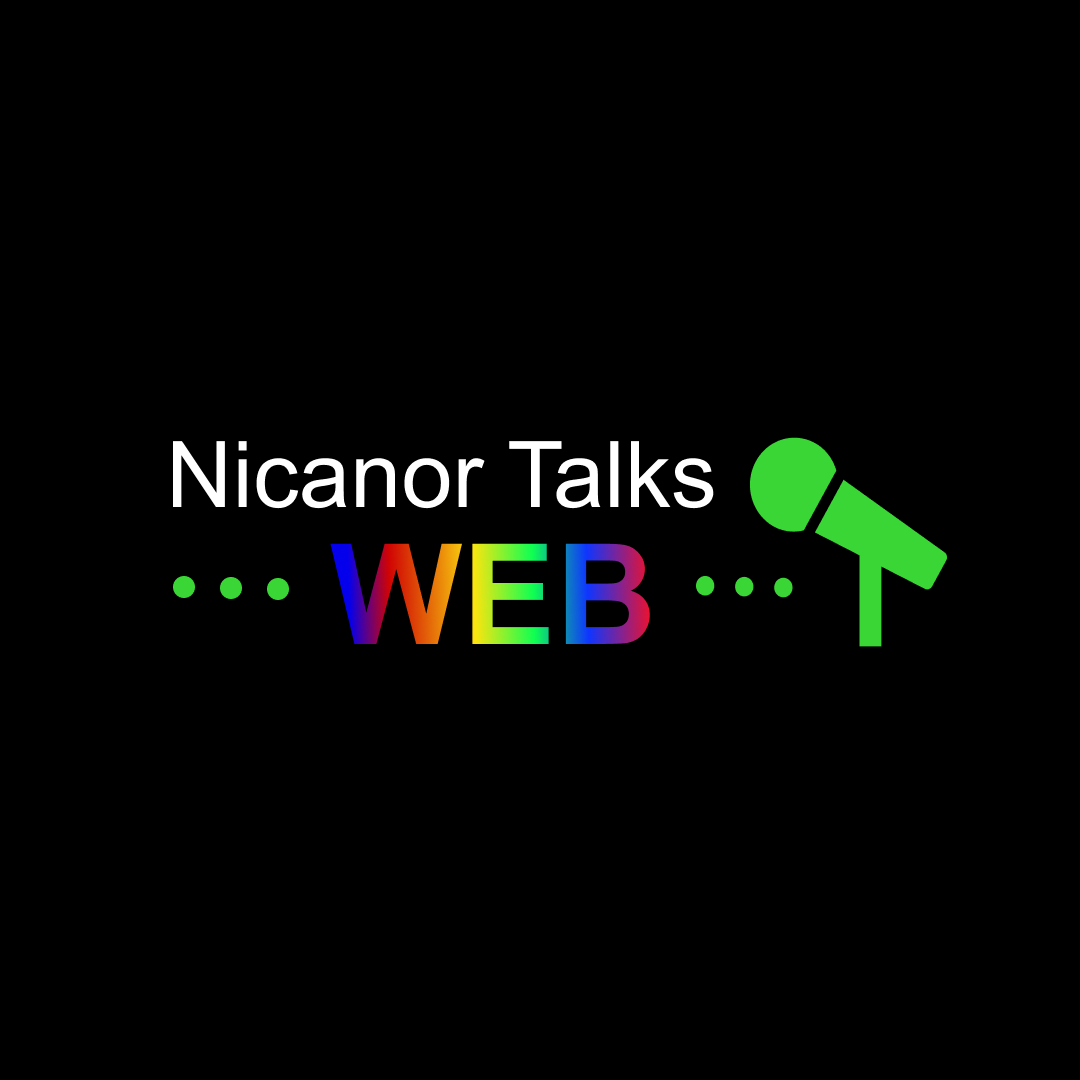
Nicanor Talks Web
Nicanor Talks Web
Hi there! I'm Nicanor, and I'm a Software Engineer. I'm passionate about solving humanitarian problems through tech, and I love sharing my knowledge and insights with others.