Row with max 1s — POTD
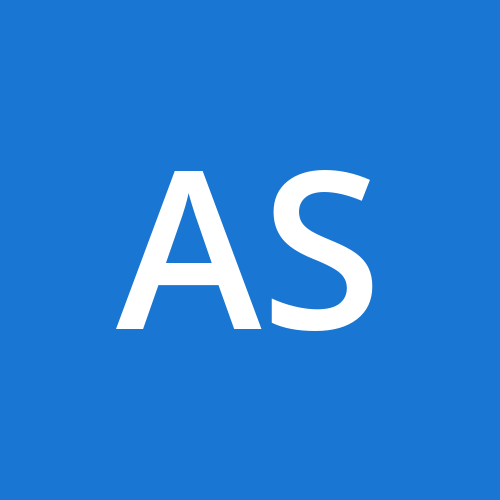
2 min read
Table of contents
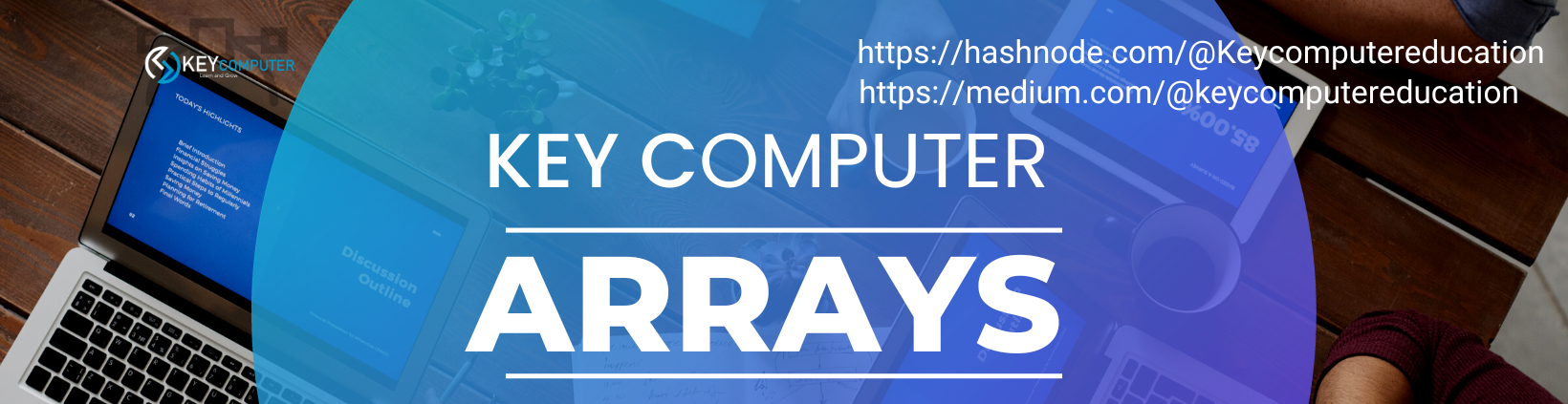
Row with max 1s
Given a boolean 2D array, consisting of only 1’s and 0’s, where each row is sorted. Return the 0-based index of the first row that has the most number of 1s. If no such row exists, return -1.
Examples:
Input: arr[][] = [[0, 1, 1, 1],
[0, 0, 1, 1],
[1, 1, 1, 1],
[0, 0, 0, 0]]
Output: 2
Explanation: Row 2 contains 4 1's (0-based indexing).
Input: arr[][] = [[0, 0],
[1, 1]]
Output: 1
Explanation: Row 1 contains 2 1's (0-based indexing).
Expected Time Complexity: O(n+m)
Expected Auxiliary Space: O(1)
Constraints:
1 ≤ number of rows, number of columns ≤ 103
0 ≤ arr[i][j] ≤ 1
Row with max 1s | Practice | GeeksforGeeks
Given a boolean 2D array, consisting of only 1's and 0's, where each row is sorted. Return the 0-based index of the…
// User function template for C++
class Solution {
public:
int rowWithMax1s(vector<vector<int> > &arr) {
// code here
int m=-1,n=0;
for(int i=0;i<arr.size();i++){
int sum=0;
for(int j=0;j<arr[0].size();j++){
if(arr[i][j]==1){
sum+=arr[i][j];
}
}
if(sum>n){
n=max(n,sum);
m=i;
}
}
return m;
}
};
#User function Template for python3
class Solution:
def rowWithMax1s(self,arr):
# code here
max =0
count=0
rcount=0
for i in arr:
if max < sum (i):
max = sum(i)
rcount = count
count+=1
if max ==0:
return -1;
else:
return rcount;
//{ Driver Code Starts
// Initial Template for Java
import java.io.*;
import java.util.*;
public class Main {
public static void main(String[] args) throws Exception {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int tc = Integer.parseInt(br.readLine().trim());
while (tc-- > 0) {
String[] inputLine;
inputLine = br.readLine().trim().split(" ");
int n = Integer.parseInt(inputLine[0]);
int m = Integer.parseInt(inputLine[1]);
int[][] arr = new int[n][m];
inputLine = br.readLine().trim().split(" ");
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
arr[i][j] = Integer.parseInt(inputLine[i * m + j]);
}
}
int ans = new Solution().rowWithMax1s(arr);
System.out.println(ans);
}
}
}
// } Driver Code Ends
// User function Template for Java
class Solution {
public int rowWithMax1s(int arr[][]) {
// code here
int m=-1 , n=0;
for(int i=0 ; i <arr.length;i++){
int sum=0;
for(int j=0;j<arr[0].length;j++){
if(arr[i][j]==1){
sum+=arr[i][j];
}
}
if(sum > n){
n=Math.max(n,sum);
m=i;
}
}
return m;
}
}
0
Subscribe to my newsletter
Read articles from Akshima Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
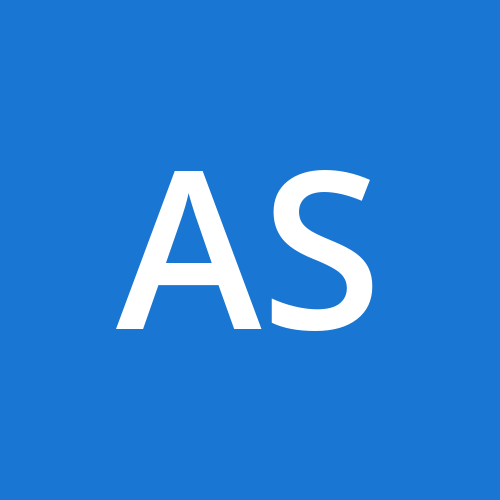