Integrating SQLAlchemy with Flask for Robust Web Application Development
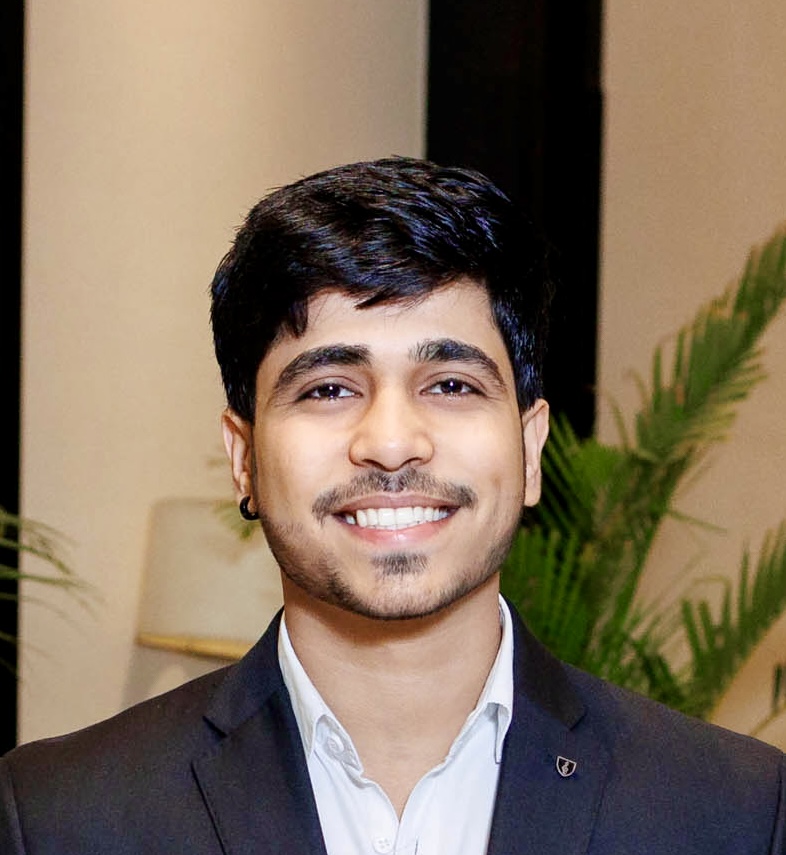
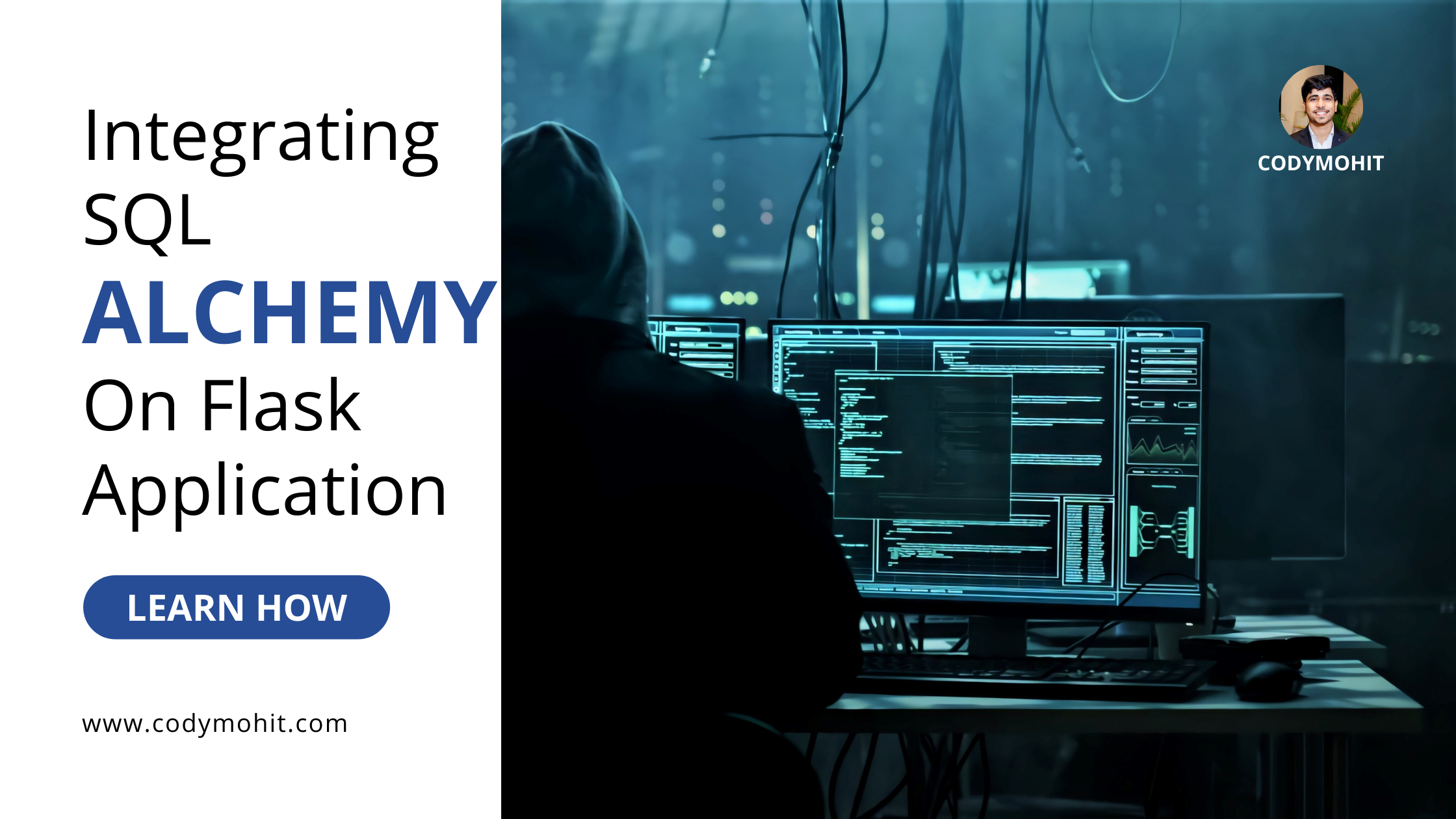
Are you tired of wrestling with complex database management in your Flask applications? Imagine a world where your web app’s backend effortlessly handles data like a pro! If you’re a developer keen on supercharging your Flask projects, then you’re in for a treat. In this blog, we’ll dive into the magic of integrating SQLAlchemy with Flask to create powerful, efficient, and scalable web applications. Whether you're a seasoned pro or just starting out, you’ll discover how SQLAlchemy's ORM capabilities can simplify your data management and make your Flask apps shine. Get ready to transform your development experience and build applications that stand out. So, grab a cup of coffee, and let’s get started!
Overview of Flask and SQLAlchemy
Flask: A Brief Introduction
Flask is a micro web framework for Python. It’s designed to be lightweight and flexible, making it easy to build simple web applications quickly. Think of Flask as a foundation: it doesn’t come with many built-in features but gives you the freedom to choose the tools you need. You can start with just a few lines of code to create a basic web server and then add features as you go along.
Here’s a super basic example of a Flask application:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return 'Hello, Flask!'
if __name__ == '__main__':
app.run(debug=True)
This code sets up a basic web server that responds with "Hello, Flask!" when you visit the root URL.
SQLAlchemy: An Introduction
SQLAlchemy is an Object Relational Mapper (ORM) for Python. It lets you interact with your database using Python objects instead of writing raw SQL queries. This can make your code cleaner and easier to understand. SQLAlchemy abstracts away the details of SQL and lets you work with Python classes and objects.
Here’s a simple example of how SQLAlchemy models look:
from flask_sqlalchemy import SQLAlchemy
db = SQLAlchemy()
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
def __repr__(self):
return f'<User {self.username}>'
In this example, User
is a class that represents a table in the database. Each instance of User
represents a row in the table.
Why Integrate SQLAlchemy with Flask?
Benefits of Using SQLAlchemy with Flask
Simplicity: SQLAlchemy simplifies database interactions by allowing you to use Python objects instead of writing SQL queries directly. This can make your code more readable and maintainable.
Flexibility: Even though Flask is a micro framework, it doesn’t force you into a specific way of doing things. SQLAlchemy complements this by providing a flexible ORM system that can adapt to various database structures.
Productivity: Using SQLAlchemy can speed up development because it handles common tasks, like creating tables and querying data, which means you can focus on writing your application logic.
Consistency: SQLAlchemy helps maintain consistency in how you interact with your database. It ensures that all your database interactions are handled in a uniform way.
Common Use Cases and Scenarios
Simple Web Applications: If you’re building a web app that needs to store and retrieve data, integrating SQLAlchemy with Flask can simplify the process. For instance, a blog or a user management system can benefit greatly from this integration.
Rapid Prototyping: When you’re quickly developing a new application or feature, SQLAlchemy’s ORM can help you get things up and running faster, thanks to its easy-to-use syntax and automated database management.
Complex Applications: Even in more complex applications where you need to handle multiple tables and relationships, SQLAlchemy provides tools to manage these complexities efficiently.
Educational Projects: If you’re learning web development or databases, using Flask with SQLAlchemy can give you hands-on experience with both web frameworks and ORMs.
Code Example: Integrating Flask with SQLAlchemy
Here’s a basic example of how you can integrate Flask and SQLAlchemy in a single application:
from flask import Flask
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///mydatabase.db'
db = SQLAlchemy(app)
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
def __repr__(self):
return f'<User {self.username}>'
@app.route('/')
def home():
return 'Hello, Flask with SQLAlchemy!'
if __name__ == '__main__':
db.create_all() # Creates the database tables
app.run(debug=True)
In this example, app.config['SQLALCHEMY_DATABASE_URI']
is used to specify the database. db.create_all()
creates the necessary tables based on the models you’ve defined.
3. Setting Up a Flask Project
Installing Flask
To start working with Flask, you first need to install it. Flask is a lightweight web framework for Python that makes it easy to build web applications. You can install Flask using pip
, Python's package installer. Here’s how you do it:
Open your terminal or command prompt.
Run the following command:
pip install Flask
This will download and install Flask along with its dependencies. If you’re using a virtual environment (which is a good practice), make sure to activate it before running the installation command.
Creating a Basic Flask Application
Once Flask is installed, you can create a basic web application. Let’s create a simple "Hello, World!" app:
Create a new directory for your project. For example:
mkdir my_flask_app cd my_flask_app
Inside this directory, create a file named
app.py
. This file will contain your Flask application code. Openapp.py
in your favorite text editor and add the following code:from flask import Flask app = Flask(__name__) @app.route('/') def hello_world(): return 'Hello, World!' if __name__ == '__main__': app.run(debug=True)
Here’s a quick rundown of what this code does:
from flask import Flask
: Imports the Flask class.app = Flask(__name__)
: Creates an instance of the Flask class.__name__
helps Flask locate resources.@app.route('/')
: Defines the route for the root URL (/
). When accessed, it calls thehello_world
function.def hello_world()
: This function returns the text "Hello, World!" when the root URL is accessed.app.run
(debug=True)
: Runs the application with debug mode enabled, which helps during development by showing detailed error messages.
To run the application, go back to your terminal and execute:
python app.py
You should see output indicating that the server is running. Open your web browser and go to
http://127.0.0.1:5000/
to see the "Hello, World!" message.
4. Flask Project Structure
Organizing your Flask project properly is crucial for maintainability and scalability. Here’s a typical project structure and some best practices:
Directory Layout
Here’s a simple but effective layout for a Flask project:
my_flask_app/
├── app/
│ ├── __init__.py
│ ├── routes.py
│ ├── models.py
│ ├── templates/
│ └── static/
├── tests/
│ └── test_basic.py
├── config.py
├── requirements.txt
└── run.py
Explanation:
app/
: Contains your application’s code.__init__.py
: Initializes your Flask app and brings together components like routes and models.routes.py
: Contains route definitions. It’s where you handle different URLs.models.py
: Defines your data models if you’re using a database.templates/
: Holds your HTML files (templates) for rendering views.static/
: Contains static files like CSS, JavaScript, and images.
tests/
: Holds your test files to ensure your code works correctly.test_
basic.py
: An example of a basic test file. You can add more tests here.
config.py
: Stores configuration settings for your app. This file might include database connection info, secret keys, etc.requirements.txt
: Lists all the dependencies your project needs. You can create it with:pip freeze > requirements.txt
run.py
: The script to start your application. It imports the app fromapp
and runs it.
Best Practices
Modularize Your Code: Keep your application modular by separating concerns. For example, routes, models, and configurations should be in different files.
Use Blueprints: If your application grows, consider using Flask Blueprints to organize routes into modules.
Configuration Management: Store configuration settings in
config.py
and use environment variables to manage sensitive data.Testing: Always include a
tests
directory and write tests for your code to ensure its reliability.
5. What is SQLAlchemy?
SQLAlchemy is a powerful and flexible Object-Relational Mapping (ORM) library for Python. It provides a way to interact with databases in a more Pythonic way by mapping Python classes to database tables, and instances of those classes to rows in the tables.
Core Concepts of SQLAlchemy
ORM (Object-Relational Mapper): This is a way to interact with a database using Python objects. Instead of writing raw SQL queries, you work with Python classes and objects. SQLAlchemy takes care of translating these objects into SQL queries and vice versa. This helps to write cleaner and more maintainable code.
Session: This is an object that manages the conversation between your Python application and the database. It tracks changes to objects, handles transactions, and commits or rolls back those changes as necessary.
Model: A model in SQLAlchemy represents a table in the database. Each model is a Python class that inherits from
Base
, and its attributes map to columns in the table.Querying: SQLAlchemy provides a powerful query interface that allows you to perform database operations like SELECT, INSERT, UPDATE, and DELETE using Python code.
Schema: This defines the structure of your database tables and their relationships. It includes tables, columns, primary keys, foreign keys, and other constraints.
SQLAlchemy vs. Other ORMs
Django ORM: Django's ORM is tightly integrated with the Django framework and is more opinionated, meaning it has more built-in functionality and conventions. SQLAlchemy is more flexible and can be used with various frameworks.
Peewee: Peewee is a simpler and lightweight ORM compared to SQLAlchemy. It is easier to use but lacks some advanced features and flexibility found in SQLAlchemy.
Tortoise-ORM: This is an asyncio ORM, which means it's designed for asynchronous programming. SQLAlchemy also supports async operations, but Tortoise is more focused on this use case.
6. SQLAlchemy Components
Overview of SQLAlchemy ORM and SQLAlchemy Core
SQLAlchemy ORM (Object-Relational Mapper): This component allows you to use Python classes to represent database tables. It abstracts away the SQL queries and provides a high-level interface to interact with the database. You define your models (tables) as classes, and SQLAlchemy takes care of generating the SQL queries to perform database operations.
Example Code:
from sqlalchemy import create_engine, Column, Integer, String from sqlalchemy.ext.declarative import declarative_base from sqlalchemy.orm import sessionmaker # Define the database engine engine = create_engine('sqlite:///example.db') # Create a base class for declarative models Base = declarative_base() # Define a User model class User(Base): __tablename__ = 'users' id = Column(Integer, primary_key=True) name = Column(String) age = Column(Integer) # Create the database schema Base.metadata.create_all(engine) # Create a session Session = sessionmaker(bind=engine) session = Session() # Add a new user new_user = User(name='John Doe', age=30) session.add(new_user) session.commit()
SQLAlchemy Core: This component provides a lower-level, SQL expression language for constructing SQL queries. It's more flexible and allows fine-grained control over the SQL being executed. It is useful for cases where you need to execute raw SQL or perform operations that are not easily handled by the ORM.
Example Code:
from sqlalchemy import create_engine, MetaData, Table, Column, Integer, String # Define the database engine engine = create_engine('sqlite:///example.db') # Create a metadata object metadata = MetaData() # Define a table users = Table('users', metadata, Column('id', Integer, primary_key=True), Column('name', String), Column('age', Integer)) # Create the table metadata.create_all(engine) # Insert a new user with engine.connect() as connection: connection.execute(users.insert().values(name='Jane Doe', age=25)) # Query the table result = connection.execute(users.select()) for row in result: print(row)
7. Installing SQLAlchemy
To use SQLAlchemy with Flask, you first need to install the necessary packages. Here’s how you can do it:
pip install Flask-SQLAlchemy
This command installs both Flask and SQLAlchemy, plus the integration package Flask-SQLAlchemy
which simplifies using SQLAlchemy with Flask.
8. Configuring SQLAlchemy with Flask
After installing, you need to configure SQLAlchemy in your Flask application. Here’s a step-by-step guide:
Basic Setup: In your Flask app’s main file (usually
app.py
ormain.py
), you’ll set up SQLAlchemy with Flask like this:from flask import Flask from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) # Configuration app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///example.db' app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False db = SQLAlchemy(app)
Here,
SQLALCHEMY_DATABASE_URI
is the URI for your database. In this case, it’s using SQLite. For other databases like PostgreSQL or MySQL, you’d use a different URI.Configuration Options:
SQLALCHEMY_DATABASE_URI
: Specifies the database connection string.SQLALCHEMY_TRACK_MODIFICATIONS
: Disables Flask’s modification tracking to save resources. This is not needed for most cases.
Best Practices:
Store your database URI and other sensitive information in environment variables rather than hard-coding them.
Regularly update your dependencies and review configuration settings for security and performance improvements.
9. Creating a Database Schema
To define your database schema, you use SQLAlchemy’s ORM capabilities to create models. Here’s how you can do it:
Defining Models: Create a
models.py
file where you define your database schema:from app import db class User(db.Model): id = db.Column(db.Integer, primary_key=True) username = db.Column(db.String(80), unique=True, nullable=False) email = db.Column(db.String(120), unique=True, nullable=False) def __repr__(self): return f'<User {self.username}>'
In this example,
User
is a model with three columns:id
,username
, andemail
. The__repr__
method is used for debugging and provides a string representation of the model instance.Using Flask-Migrate for Schema Migrations: Flask-Migrate is an extension that handles SQLAlchemy database migrations for Flask applications. First, you need to install it:
pip install Flask-Migrate
Then, integrate it into your application:
from flask_migrate import Migrate migrate = Migrate(app, db)
Initializing Migrations: Run these commands to set up migrations:
flask db init flask db migrate -m "Initial migration." flask db upgrade
flask db init
: Initializes a new migration repository.flask db migrate -m "Message"
: Generates a new migration script.flask db upgrade
: Applies the migration to the database.
Best Practices:
Regularly commit your migration files to version control.
Review migration scripts before applying them to ensure they don’t introduce unwanted changes.
10. Defining Models and Relationships
In SQLAlchemy, models represent your database tables. Defining these models is a way to map your database schema into Python classes. Let’s dive into how you can define models and set up relationships between them.
Creating Model Classes
Here’s a simple example to show how to define a model in SQLAlchemy:
from flask_sqlalchemy import SQLAlchemy
db = SQLAlchemy()
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
email = db.Column(db.String(120), unique=True, nullable=False)
def __repr__(self):
return f'<User {self.username}>'
In this example, User
is a model with three columns: id
, username
, and email
. db.Column
is used to define each column's type and attributes.
Defining Relationships
You can also define relationships between models. For example, if you have a Post
model that needs to relate to the User
model, you can set up a one-to-many relationship:
class Post(db.Model):
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(120), nullable=False)
content = db.Column(db.Text, nullable=False)
user_id = db.Column(db.Integer, db.ForeignKey('user.id'), nullable=False)
user = db.relationship('User', backref=db.backref('posts', lazy=True))
def __repr__(self):
return f'<Post {self.title}>'
Here, each Post
is linked to a User
via user_id
, and db.relationship
is used to establish this relationship. The backref
allows you to access posts from a user object, like user.posts
.
11. CRUD Operations with SQLAlchemy
CRUD stands for Create, Read, Update, and Delete. These are the basic operations you’ll perform on your database records.
Creating Records
To create a new record, you instantiate a model and add it to the session:
new_user = User(username='john_doe', email='john@example.com')
db.session.add(new_user)
db.session.commit()
Reading Records
You can read records using query methods:
# Get all users
users = User.query.all()
# Get a single user by ID
user = User.query.get(1)
Updating Records
To update a record, first retrieve it, then make changes, and commit:
user = User.query.get(1)
user.username = 'john_doe_updated'
db.session.commit()
Deleting Records
To delete a record, retrieve it, delete it from the session, and commit:
user = User.query.get(1)
db.session.delete(user)
db.session.commit()
12. Querying Data
SQLAlchemy provides both basic and advanced querying capabilities.
Basic Querying
For simple queries, use filter
and filter_by
:
# Filter by a specific column
users = User.query.filter(User.username == 'john_doe').all()
# Filter using keyword arguments
users = User.query.filter_by(username='john_doe').all()
Advanced Querying
Advanced queries can involve joins, aggregations, and more complex filters:
from sqlalchemy import func
# Count the number of users
user_count = db.session.query(func.count(User.id)).scalar()
# Join two tables
posts = db.session.query(Post).join(User).filter(User.username == 'john_doe').all()
Here, func.count
is used for aggregation, and join
is used to retrieve posts that belong to a specific user.
13. Introduction to Flask-Migrate
Flask-Migrate is an extension for Flask that handles database migrations using Alembic, a lightweight database migration tool for SQLAlchemy. It's super handy for managing changes to your database schema over time. When you're developing a Flask app and you need to update your database schema—like adding a new table or modifying existing columns—Flask-Migrate helps you track these changes and apply them safely.
Why use Flask-Migrate?
Version Control: It keeps track of your database schema changes in a systematic way, so you can revert or upgrade easily.
Consistency: Ensures that the schema changes are applied consistently across different environments (like development, testing, and production).
Automation: Automates the process of applying and rolling back schema changes, reducing the risk of human error.
14. Setting Up Flask-Migrate
To get started with Flask-Migrate, you'll first need to install it. Here’s how:
Installation: Use pip to install Flask-Migrate.
pip install Flask-Migrate
Configuration: Integrate Flask-Migrate with your Flask app. First, import and initialize it in your application.
from flask import Flask from flask_sqlalchemy import SQLAlchemy from flask_migrate import Migrate app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///mydatabase.db' db = SQLAlchemy(app) migrate = Migrate(app, db)
In this example, we’re using SQLite, but you can use any database supported by SQLAlchemy.
Initialize Migrations: Run the following command to create a migration repository.
flask db init
This command creates a
migrations
directory where migration scripts will be stored.
15. Creating and Applying Migrations
With Flask-Migrate set up, you can now create and apply migrations. Here’s how:
Creating Migrations: Whenever you make changes to your models (like adding a new model or changing an existing one), generate a migration script with:
flask db migrate -m "Description of the changes"
The
-m
flag lets you add a message describing what the migration is about. This helps in keeping track of what each migration does.Applying Migrations: To apply the generated migrations to your database, use:
flask db upgrade
This command applies all the migrations that haven’t been applied yet.
Rolling Back Migrations: If something goes wrong, you can rollback the last migration with:
flask db downgrade
This command reverts the most recent migration.
16. Understanding Transactions
Basics of Transactions and Their Importance
Think of transactions like a way to bundle a bunch of operations into one neat package. When you’re working with a database, a transaction ensures that a series of operations either all succeed together or fail together. This is super important for keeping your data consistent and reliable.
Why is this important? Imagine you’re transferring money from one account to another. If the process fails halfway, you could end up with money missing from one account but not added to the other. Transactions make sure either both the debit and credit happen or neither do.
Key Concepts:
Atomicity: This means "all-or-nothing." Either every operation in the transaction is completed successfully, or none of them are. If something goes wrong, the transaction rolls back, undoing all changes made.
Consistency: This ensures that the database always moves from one valid state to another. It maintains the rules and constraints of your database schema.
Isolation: Each transaction operates independently. This means changes made by one transaction are not visible to others until the transaction is complete.
Durability: Once a transaction is committed, the changes are permanent and will survive any system crashes.
17. Using SQLAlchemy Sessions
Managing Sessions and Transactions in Flask Applications
SQLAlchemy sessions are like the workhorses that manage your transactions. They handle the communication between your Flask app and the database, ensuring that your operations are performed in a controlled manner.
Basic Session Management:
Creating a Session: You need to create a session to start interacting with the database. Here’s a basic example:
from sqlalchemy.orm import sessionmaker from sqlalchemy import create_engine engine = create_engine('sqlite:///mydatabase.db') # Replace with your database URL Session = sessionmaker(bind=engine) session = Session()
Using the Session: Now you can use this session to add or update records. Here’s how you add a new record:
from mymodels import MyModel # Import your model new_record = MyModel(name="John Doe") session.add(new_record) session.commit() # This saves the new record to the database
Handling Transactions: If you want to make sure multiple operations are handled as a single transaction, you can use the session within a
try...except
block:try: # Do some database operations session.add(new_record) session.commit() except Exception as e: session.rollback() # Undo changes if something goes wrong print(f"An error occurred: {e}") finally: session.close() # Always close the session when done
Querying Data: You can also use sessions to fetch data:
result = session.query(MyModel).filter_by(name="John Doe").first() print(result)
18. Performance Optimization
When it comes to optimizing database queries and performance, you can make a big difference by paying attention to a few key strategies. Here are some tips:
Use Indexes Wisely
Indexes can speed up query performance by allowing the database to find data faster. For example, if you often query a table based on theemail
column, creating an index on that column can improve speed.from sqlalchemy import Index Index('idx_email', User.email)
But be careful—too many indexes can slow down insert and update operations.
Optimize Queries
Write efficient SQL queries. AvoidSELECT *
and specify only the columns you need. This reduces the amount of data transferred and processed.# Less efficient result = session.query(User).all() # More efficient result = session.query(User.id, User.email).all()
Use Pagination
When dealing with large datasets, use pagination to limit the number of records returned at a time. This avoids overwhelming the database and the application.from sqlalchemy.orm import sessionmaker Session = sessionmaker(bind=engine) session = Session() page = 1 per_page = 10 results = session.query(User).offset((page - 1) * per_page).limit(per_page).all()
Profile and Analyze
Regularly profile your queries to find and fix bottlenecks. Tools like SQLAlchemy’secho
mode or database-specific profiling tools can help you understand which queries are slow.from sqlalchemy import create_engine engine = create_engine('sqlite:///example.db', echo=True)
19. Error Handling and Debugging
Handling errors and debugging effectively can save you from a lot of headaches. Here are some common errors and how to handle them:
Database Connection Errors
These errors occur if your application cannot connect to the database. Ensure that your connection string is correct and that the database server is running.try: engine = create_engine('sqlite:///example.db') except Exception as e: print(f"Connection error: {e}")
Integrity Errors
These happen when there are issues with data constraints, like unique constraints or foreign key violations.from sqlalchemy.exc import IntegrityError try: new_user = User(email='duplicate@example.com') session.add(new_user) session.commit() except IntegrityError: session.rollback() print("This email is already used.")
Query Errors
Errors in queries can arise due to syntax issues or invalid data. Check your query syntax and data types.try: result = session.query(User).filter(User.age > 'string').all() # Error: 'string' is not a valid age except Exception as e: print(f"Query error: {e}")
Debugging Tips
Use logging to track and debug issues. Set up logging to capture errors and important events.import logging logging.basicConfig(level=logging.INFO) logger = logging.getLogger(__name__) try: # Some database operation pass except Exception as e: logger.error(f"An error occurred: {e}")
20. Security Considerations
Securing your database interactions is crucial to protect your application from malicious attacks, especially SQL injection. Here’s how to do it:
Use Parameterized Queries
Parameterized queries help prevent SQL injection by separating SQL code from data. Always use this approach instead of concatenating SQL strings.from sqlalchemy.sql import text query = text("SELECT * FROM users WHERE email = :email") result = session.execute(query, {'email': 'example@example.com'})
Sanitize User Input
Always validate and sanitize user inputs. Ensure that data is clean before using it in queries.def sanitize_input(user_input): # Basic sanitization example return user_input.replace("'", "''") sanitized_input = sanitize_input(user_input)
Limit Database Privileges
Grant the minimum necessary privileges to your database users. Avoid using database accounts with high-level permissions for everyday operations.GRANT SELECT, INSERT, UPDATE ON my_database.* TO 'app_user'@'localhost';
Use Secure Connections
Ensure that your database connections use encryption (e.g., SSL/TLS) to protect data in transit.engine = create_engine('postgresql+psycopg2://user:password@localhost/dbname', connect_args={"sslmode": "require"})
21. Setting Up Testing Environment
When it comes to testing your Flask applications with SQLAlchemy, setting up the right environment is crucial. Here’s a step-by-step guide on configuring your testing settings and test databases:
Create a Test Configuration: Start by creating a separate configuration file for your tests. This allows you to use different settings from your production or development environment. For example, create a
config_
test.py
file with the following content:class TestConfig: SQLALCHEMY_DATABASE_URI = 'sqlite:///test.db' SQLALCHEMY_TRACK_MODIFICATIONS = False TESTING = True
Here, we’re using an in-memory SQLite database (
test.db
) for simplicity.Set Up Test Database: Ensure your test database is created and properly configured. For SQLite, it’s straightforward as shown in the config. If you’re using other databases, make sure to set up appropriate database fixtures.
Initialize the Flask App for Testing: Modify your test setup to use the test configuration. This typically involves creating a
conftest.py
file or similar in your test directory:import pytest from yourapp import create_app, db @pytest.fixture(scope='module') def app(): app = create_app('config_test') with app.app_context(): yield app db.drop_all() @pytest.fixture(scope='module') def client(app): return app.test_client()
This code sets up a test app and ensures the database is dropped after tests are complete.
22. Writing Tests for SQLAlchemy Models
Testing your SQLAlchemy models involves both unit tests and integration tests. Let’s break down how to write these tests:
Unit Tests for Models: Unit tests focus on testing individual components. Here’s an example of how to test a SQLAlchemy model:
from yourapp.models import User from yourapp import db def test_user_model(): user = User(username='testuser', email='test@example.com') db.session.add(user) db.session.commit() assert user.id is not None assert user.username == 'testuser'
In this test, we’re creating a
User
instance, adding it to the session, committing it, and then verifying that the user has been properly created.Integration Tests: Integration tests check how various parts of your application work together. For SQLAlchemy, this often means testing the interaction between models and the database. Here’s an example:
def test_user_creation(client): response = client.post('/create_user', json={'username': 'newuser', 'email': 'new@example.com'}) assert response.status_code == 201 user = User.query.filter_by(username='newuser').first() assert user is not None assert user.email == 'new@example.com'
This test sends a POST request to your endpoint for creating users and verifies that the user is correctly added to the database.
A Few Key Points:
Always use a separate test database to avoid polluting your production data.
Use fixtures to manage the setup and teardown of your database.
Make sure your tests are isolated and repeatable.
23. Preparing for Deployment
Deploying a Flask application with SQLAlchemy can seem daunting, but following some best practices can make the process smoother and more reliable. Here’s a guide to help you prepare your app for deployment:
**1. Configuration Management:
Separate Configurations: Use environment variables or configuration files to manage different settings for development, testing, and production. This ensures sensitive information like database credentials are not hard-coded in your source code.
Example:
import os class Config: SQLALCHEMY_DATABASE_URI = os.getenv('DATABASE_URL') SECRET_KEY = os.getenv('SECRET_KEY')
**2. Dependency Management:
Requirements File: Ensure all your project dependencies are listed in a
requirements.txt
file. This allows you to recreate the exact environment in production.Generate Requirements File:
pip freeze > requirements.txt
**3. Testing:
- Run Tests: Before deploying, run all your tests to catch any issues that might arise. Make sure your unit tests and integration tests are comprehensive and passing.
**4. Static Files:
- Collect Static Files: If your Flask app serves static files (like CSS or JavaScript), make sure they are collected and served properly in production. You might need to use a web server like Nginx for this.
**5. Logging:
Set Up Logging: Implement logging to capture errors and important events. This helps in debugging and monitoring the application’s performance.
Example:
import logging logging.basicConfig(filename='app.log', level=logging.INFO)
**6. Security:
- Secure Your App: Use HTTPS, set security headers, and regularly update dependencies to avoid vulnerabilities.
**7. Database Migration:
Apply Migrations: Ensure all database migrations are applied before starting your application. Use Flask-Migrate for managing migrations.
Example:
flask db upgrade
**8. Scaling:
- Plan for Scaling: Consider how your app will handle increased traffic. Use load balancers, and consider horizontal scaling of your app servers.
24. Managing Database in Production
Managing your database in production is crucial to ensure your app runs smoothly and data is safe. Here are some strategies:
**1. Backups:
Regular Backups: Schedule regular backups of your database to avoid data loss. Automate the process if possible.
Example (PostgreSQL):
pg_dump mydatabase > mydatabase_backup.sql
**2. Monitoring:
Monitor Database Performance: Keep an eye on database performance metrics like query times and connection counts. Use tools like
pgAdmin
orMySQL Workbench
for this.Example:
SELECT * FROM pg_stat_activity;
**3. Replication:
Database Replication: Set up replication to ensure high availability. This helps if your primary database fails.
Example: Use master-slave replication for databases like MySQL.
**4. Scaling:
- Scale Your Database: As your app grows, you may need to scale your database. Consider sharding or using a managed database service that scales automatically.
**5. Security:
Secure Access: Restrict database access to only necessary applications and users. Use strong passwords and encryption for sensitive data.
Example:
GRANT SELECT, INSERT, UPDATE ON mytable TO 'user'@'host';
**6. Error Handling:
- Handle Database Errors: Implement error handling in your application to manage database connection issues or query failures gracefully.
**7. Regular Maintenance:
Database Maintenance: Perform regular maintenance tasks like indexing and vacuuming (for PostgreSQL) to keep the database performant.
Example:
VACUUM ANALYZE;
25. Real-world Applications
Flask and SQLAlchemy in Action
Flask and SQLAlchemy are a powerful combo used in many real-world applications due to their flexibility and ease of use. Here are some examples:
Blog Platforms: Many blog platforms use Flask and SQLAlchemy to handle dynamic content. The simplicity of Flask allows for quick development of web apps, while SQLAlchemy manages the database interactions, such as storing and retrieving posts and user comments.
from flask import Flask, request, jsonify from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///blog.db' db = SQLAlchemy(app) class Post(db.Model): id = db.Column(db.Integer, primary_key=True) title = db.Column(db.String(100), nullable=False) content = db.Column(db.Text, nullable=False) @app.route('/posts', methods=['POST']) def add_post(): title = request.json['title'] content = request.json['content'] new_post = Post(title=title, content=content) db.session.add(new_post) db.session.commit() return jsonify({'message': 'Post created'}), 201 if __name__ == '__main__': app.run(debug=True)
E-commerce Websites: E-commerce platforms use Flask to create a smooth and responsive user experience while SQLAlchemy handles the complex relationships between products, orders, and users.
from flask import Flask, request, jsonify from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///shop.db' db = SQLAlchemy(app) class Product(db.Model): id = db.Column(db.Integer, primary_key=True) name = db.Column(db.String(100), nullable=False) price = db.Column(db.Float, nullable=False) @app.route('/products', methods=['GET']) def get_products(): products = Product.query.all() return jsonify([{'id': p.id, 'name': p.name, 'price': p.price} for p in products]) if __name__ == '__main__': app.run(debug=True)
Internal Tools and Dashboards: Many companies use Flask and SQLAlchemy to build internal tools for managing data and generating reports. The simplicity of Flask makes it easy to create dashboards, and SQLAlchemy efficiently manages the underlying data.
from flask import Flask, render_template from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///dashboard.db' db = SQLAlchemy(app) class Metric(db.Model): id = db.Column(db.Integer, primary_key=True) name = db.Column(db.String(100), nullable=False) value = db.Column(db.Float, nullable=False) @app.route('/') def index(): metrics = Metric.query.all() return render_template('dashboard.html', metrics=metrics) if __name__ == '__main__': app.run(debug=True)
26. Common Challenges and Solutions
Challenges and Fixes
Challenge: Handling Database Migrations
Problem: Keeping track of changes to your database schema can be tricky. If you update your models but forget to apply migrations, it can lead to inconsistencies between your code and database.
Solution: Use
Flask-Migrate
to handle database migrations. It integrates with SQLAlchemy and provides commands to upgrade and downgrade your database schema.flask db init # Initialize migration repository flask db migrate # Create migration scripts flask db upgrade # Apply migrations to the database
Challenge: Managing Relationships Between Models
Problem: Defining and querying relationships between models (like one-to-many or many-to-many) can be complex and error-prone.
Solution: Use SQLAlchemy's built-in relationship handling. For example, use
relationship
andbackref
to simplify these interactions.class Author(db.Model): id = db.Column(db.Integer, primary_key=True) name = db.Column(db.String(100), nullable=False) books = db.relationship('Book', backref='author', lazy=True) class Book(db.Model): id = db.Column(db.Integer, primary_key=True) title = db.Column(db.String(100), nullable=False) author_id = db.Column(db.Integer, db.ForeignKey('author.id'), nullable=False)
Challenge: Handling Large Data Sets
Problem: Loading large amounts of data into memory can cause performance issues or crashes.
Solution: Use pagination and lazy loading to handle large datasets efficiently. SQLAlchemy provides methods to query data in chunks.
@app.route('/posts') def get_posts(): page = request.args.get('page', 1, type=int) per_page = 10 posts = Post.query.paginate(page, per_page, False) return jsonify([{'id': p.id, 'title': p.title} for p in posts.items])
Challenge: Security Concerns
Problem: Exposing your database directly to the internet can lead to security issues like SQL injection.
Solution: Always use parameterized queries and avoid directly including user input in your queries.
@app.route('/search') def search(): query = request.args.get('query', '') results = Post.query.filter(Post.title.contains(query)).all() return jsonify([{'id': r.id, 'title': r.title} for r in results])
27. SQLAlchemy’s Advanced Querying
SQLAlchemy’s advanced querying features allow you to perform complex operations and retrieve data in sophisticated ways. Here’s a breakdown of some key features:
1. Joins and Relationships: You can join tables and query related objects using SQLAlchemy’s ORM capabilities. For example, if you have a User
and Post
model with a relationship defined, you can easily join them:
from sqlalchemy.orm import joinedload
# Query users and their posts
query = session.query(User).options(joinedload(User.posts))
users = query.all()
for user in users:
print(user.name)
for post in user.posts:
print(f" - {post.title}")
2. Subqueries: Subqueries allow you to nest queries within another query. This can be useful for complex filters:
from sqlalchemy import select
# Create a subquery for posts with more than 10 comments
subquery = select([Post.id]).where(Post.comment_count > 10).alias('subquery')
# Main query using the subquery
query = session.query(User).join(subquery, User.id == subquery.c.id)
users = query.all()
3. Complex Filters: You can use SQLAlchemy’s filtering capabilities to create more complex queries:
from sqlalchemy import and_
# Query users who have made posts in 2023
query = session.query(User).filter(and_(User.posts.any(Post.created_at >= '2023-01-01'), User.posts.any(Post.created_at <= '2023-12-31')))
users = query.all()
4. Aggregations: Aggregations such as count()
, sum()
, and avg()
can be performed easily:
from sqlalchemy import func
# Count the number of posts per user
query = session.query(User.name, func.count(Post.id)).join(User.posts).group_by(User.name)
results = query.all()
for name, count in results:
print(f"{name} has {count} posts")
28. Working with Multiple Databases
Handling multiple databases and schemas in SQLAlchemy can be a bit tricky but is manageable with the right approach.
1. Multiple Engines: You need separate Engine
instances for each database:
from sqlalchemy import create_engine
# Create engine for primary database
engine1 = create_engine('sqlite:///primary.db')
# Create engine for secondary database
engine2 = create_engine('sqlite:///secondary.db')
2. Multiple Sessions: Each engine needs its own Session
:
from sqlalchemy.orm import sessionmaker
Session1 = sessionmaker(bind=engine1)
Session2 = sessionmaker(bind=engine2)
session1 = Session1()
session2 = Session2()
3. Working with Different Schemas: When dealing with multiple schemas within a single database, you can specify the schema in your model definitions:
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy import Column, Integer, String
Base = declarative_base()
class User(Base):
__tablename__ = 'users'
__table_args__ = {'schema': 'main'}
id = Column(Integer, primary_key=True)
name = Column(String)
class ArchiveUser(Base):
__tablename__ = 'users'
__table_args__ = {'schema': 'archive'}
id = Column(Integer, primary_key=True)
name = Column(String)
4. Switching Between Databases: Sometimes you might need to switch between databases in your code. Ensure you handle transactions carefully when doing so:
# Using session1 to interact with the primary database
with session1.begin():
# perform operations
# Switch to session2 for the secondary database
with session2.begin():
# perform operations
In summary, advanced querying in SQLAlchemy enables powerful data retrieval with features like joins, subqueries, complex filters, and aggregations. Handling multiple databases involves managing multiple engines, sessions, and schemas. Both features provide flexibility but require careful management to ensure smooth operations.
29. SQLAlchemy vs. Django ORM
SQLAlchemy and Django ORM are both popular tools for interacting with databases in Python, but they have different philosophies and use cases. Here’s a comparison to help you choose the right one for your needs:
SQLAlchemy
Flexibility: SQLAlchemy provides a lot of flexibility. It offers two layers: Core and ORM. Core is for those who want to write raw SQL or use SQL expression language, while ORM is for those who prefer object-relational mapping.
Use Cases: Great for projects where you need fine-grained control over database interactions or when you’re not using a specific web framework.
Integration: Works well with various web frameworks and can be used in non-web applications too.
Example Code:
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
Base = declarative_base()
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
# Database setup
engine = create_engine('sqlite:///example.db')
Base.metadata.create_all(engine)
Session = sessionmaker(bind=engine)
session = Session()
# Adding a user
new_user = User(name='John Doe')
session.add(new_user)
session.commit()
Django ORM
Tight Integration: Django ORM is tightly integrated with Django’s web framework. It’s designed to work seamlessly with Django’s features like models, forms, and views.
Use Cases: Ideal for Django-based web projects where you want an easy, out-of-the-box solution for database operations. Less flexible than SQLAlchemy but easier to use within Django’s ecosystem.
Configuration: Automatically generates SQL queries for you based on your models. Less control but more convenience.
Example Code:
from django.db import models
class User(models.Model):
name = models.CharField(max_length=100)
# Adding a user
User.objects.create(name='John Doe')
Key Differences:
Flexibility: SQLAlchemy is more flexible and can be used with various frameworks or standalone. Django ORM is more opinionated and integrated with Django.
Control: SQLAlchemy gives you more control over SQL queries and database schema. Django ORM abstracts a lot of that for simplicity.
Complexity: SQLAlchemy might require more setup and configuration, while Django ORM is more straightforward if you're already using Django.
30. SQLAlchemy vs. Peewee
SQLAlchemy and Peewee are both ORM libraries, but they cater to different needs and complexities. Here’s a comparison:
SQLAlchemy
Complexity: SQLAlchemy is a powerful and complex ORM with a steep learning curve. It’s suitable for large-scale applications where fine control over database interactions is required.
Use Cases: Ideal for complex applications where you need advanced querying and schema management. It’s also more suitable for applications that might need to integrate with multiple databases or advanced features.
Example Code:
# Similar to the SQLAlchemy example above
Peewee
Simplicity: Peewee is a simpler and lightweight ORM. It’s designed to be easy to use and configure, making it a good choice for smaller projects or when you need something straightforward.
Use Cases: Best for small to medium-sized applications where you don’t need the extensive features of SQLAlchemy. It’s easier to get started with and has a gentler learning curve.
Example Code:
from peewee import Model, CharField, SqliteDatabase
db = SqliteDatabase('example.db')
class User(Model):
name = CharField()
class Meta:
database = db
# Create table
db.connect()
db.create_tables([User])
# Adding a user
User.create(name='John Doe')
Key Differences:
Complexity: SQLAlchemy is more complex and feature-rich, suitable for large projects. Peewee is simpler and more lightweight, suitable for smaller projects.
Features: SQLAlchemy offers advanced querying and schema management. Peewee offers a simpler API with less overhead.
Learning Curve: SQLAlchemy has a steeper learning curve due to its complexity, while Peewee is easier to pick up and use quickly.
In summary, choose SQLAlchemy if you need a powerful and flexible ORM for complex applications, Django ORM if you’re using Django and need a tight integration with its features, and Peewee if you prefer a lightweight and simpler solution.
31. SQLAlchemy Documentation and Tutorials
When diving into SQLAlchemy, having good resources is essential for mastering this powerful ORM. Here’s a guide to help you find the best documentation and tutorials:
SQLAlchemy Official Documentation
The SQLAlchemy Documentation is the best place to start. It provides detailed explanations of SQLAlchemy’s features, components, and best practices. The documentation is structured in a way that guides you through the core concepts, from basic usage to advanced techniques.SQLAlchemy Tutorial by Real Python
Real Python offers an excellent SQLAlchemy Tutorial that covers the basics and walks you through practical examples. It’s great for beginners and also includes some advanced tips.SQLAlchemy Migration Documentation
For learning about schema migrations, check out the Flask-Migrate Documentation. This resource helps you understand how to handle database schema changes effectively.YouTube Tutorials
Platforms like YouTube have numerous video tutorials on SQLAlchemy. Channels like Corey Schafer provide hands-on videos that can help you grasp the concepts more interactively.Books and eBooks
Books like "Mastering SQLAlchemy" and "SQLAlchemy: Database Access Using Python" are valuable resources for in-depth learning. They provide comprehensive coverage of SQLAlchemy features and usage.
32. Flask and SQLAlchemy Community Support
When you run into issues or have questions, these communities and forums are fantastic places to seek help:
Stack Overflow
Stack Overflow is a popular platform where developers ask and answer questions about Flask and SQLAlchemy. You can search for existing answers or post your own questions if you need specific help.Reddit
Subreddits like r/flask and r/sqlalchemy are useful for engaging with other developers. You can share your experiences, ask for advice, or simply follow discussions related to Flask and SQLAlchemy.Flask and SQLAlchemy Discord Channels
Discord has various servers dedicated to Python, Flask, and SQLAlchemy. These real-time chat environments are great for getting quick answers and networking with other developers. Look for servers like Python Discord or specific Flask/SQLAlchemy channels.GitHub Issues
If you encounter bugs or need features, checking the GitHub Issues page for SQLAlchemy or Flask can be helpful. You can also report issues or contribute to discussions about ongoing problems.Official Flask and SQLAlchemy Mailing Lists
Both Flask and SQLAlchemy have mailing lists where you can ask questions and get support from the community. These lists are often monitored by the developers and experienced users who can provide insightful feedback.
By utilizing these resources and engaging with the community, you can enhance your knowledge and resolve issues more efficiently. Remember, learning from others and sharing your own experiences can significantly speed up your growth as a developer!
33. Recap of Key Points
Integrating Flask with SQLAlchemy is a powerful way to build robust and scalable web applications. Here’s a quick rundown of the key steps and best practices for integrating these two tools:
1. Setting Up Your Project
First, you need to install Flask and SQLAlchemy. This can be done with pip:
pip install Flask SQLAlchemy
2. Configuring Flask
You must configure your Flask application to work with SQLAlchemy. Here’s a simple setup:
from flask import Flask
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///example.db'
db = SQLAlchemy(app)
3. Defining Models
Create your database models by subclassing db.Model
. Each class represents a table in your database:
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
4. Handling Migrations
Use Flask-Migrate to manage database schema changes. Install it with:
pip install Flask-Migrate
Initialize migrations:
from flask_migrate import Migrate
migrate = Migrate(app, db)
And then you can run migration commands:
flask db init
flask db migrate -m "Initial migration."
flask db upgrade
5. Best Practices
Use SQLAlchemy’s session management to handle transactions and rollbacks.
Keep models and database logic separate from business logic to maintain clean code architecture.
Regularly test your models and migrations to catch issues early.
34. Future Directions and Trends
Looking ahead, several trends are emerging in the Flask and SQLAlchemy ecosystem:
1. Enhanced ORM Capabilities
SQLAlchemy is continuously evolving, with future versions expected to bring more powerful and intuitive ORM features. This includes better support for advanced querying and optimization techniques.
2. Integration with Modern Frameworks
Flask is often used in conjunction with other modern technologies like FastAPI and async frameworks. Expect to see more integrations that make Flask work seamlessly with these tools.
3. Improved Performance and Scalability
As applications grow, so does the need for performance tuning. New trends in Flask and SQLAlchemy will likely focus on enhancing performance and scalability, making it easier to handle large volumes of data and high traffic loads.
4. More Comprehensive Documentation and Tools
The community around Flask and SQLAlchemy is growing, leading to improved documentation and more tools to simplify development. Keep an eye out for new tutorials, plugins, and best practices emerging from the community.
5. Emphasis on Security
With increasing concerns about web security, future developments will likely focus on enhancing security features in Flask and SQLAlchemy. This includes better handling of sensitive data and integration with modern authentication and authorization systems.
FREQUENTLY ASKED QUESTION:
What are the main advantages of using SQLAlchemy with Flask?
Using SQLAlchemy with Flask offers several benefits:
Powerful ORM: SQLAlchemy’s ORM (Object-Relational Mapping) allows you to work with your database using Python objects, making data manipulation easier and more intuitive.
Flexibility: SQLAlchemy provides both high-level ORM and low-level SQL expression capabilities, giving you flexibility in how you interact with your database.
Migration Support: With Flask-Migrate, SQLAlchemy integrates seamlessly for managing database schema changes over time.
Performance Optimization: SQLAlchemy's caching and connection pooling features help improve performance and manage database interactions efficiently.
How do I install and configure SQLAlchemy in a Flask application?
Installation: You can install SQLAlchemy and Flask-SQLAlchemy using pip:
pip install SQLAlchemy Flask-SQLAlchemy
Configuration: In your Flask application, you’ll need to configure the SQLAlchemy extension. Here's a basic setup in your
app.py
or equivalent file:from flask import Flask from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///example.db' db = SQLAlchemy(app)
Replace
'sqlite:///example.db'
with your preferred database URI.
What is Flask-Migrate and how do I use it for database migrations?
Flask-Migrate is an extension that handles database migrations for Flask applications using SQLAlchemy. It provides a way to upgrade and downgrade your database schema over time.
Installation:
pip install Flask-Migrate
Setup: Initialize Flask-Migrate in your application:
from flask_migrate import Migrate migrate = Migrate(app, db)
Commands:
Initialize migration repository:
flask db init
Create a migration:
flask db migrate -m "Initial migration"
Apply migrations:
flask db upgrade
How can I handle transactions and sessions with SQLAlchemy in Flask?
In SQLAlchemy, you manage transactions and sessions using the Session
object.
Session Management: SQLAlchemy’s
sessionmaker
creates a new session for each request:from sqlalchemy.orm import sessionmaker Session = sessionmaker(bind=db.engine) session = Session()
Transactions: You can manage transactions using
session.commit()
andsession.rollback()
:try: # Perform database operations session.commit() except: session.rollback() raise
What are some best practices for optimizing SQLAlchemy performance?
Use Session Scoped to Requests: Ensure you create and manage sessions within the scope of a request to avoid overhead.
Optimize Queries: Use SQLAlchemy’s query optimization techniques like eager loading and proper indexing.
Connection Pooling: Configure connection pooling to manage database connections efficiently.
Lazy Loading: Avoid unnecessary data loading by using lazy loading and selective querying.
How do I write and run tests for SQLAlchemy models in a Flask application?
Testing Setup: Configure a separate test database and use Flask’s test client for testing:
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///test.db'
Writing Tests: Use a testing framework like
pytest
and write tests for your models:def test_user_model(): user = User(name='Test User') db.session.add(user) db.session.commit() assert User.query.count() == 1
Running Tests: Execute your tests using:
pytest
What are the common challenges when integrating SQLAlchemy with Flask, and how can I resolve them?
Database Connection Issues: Ensure proper configuration of your database URI and handle connection pooling.
Session Management: Manage sessions properly to avoid data inconsistencies and leaks.
Migration Conflicts: Resolve conflicts in migrations by carefully managing schema changes and using Flask-Migrate effectively.
How does SQLAlchemy compare to other ORMs like Django ORM and Peewee?
Django ORM: Django ORM is tightly integrated with the Django framework and provides a high-level abstraction. SQLAlchemy, on the other hand, is more flexible and can be used with various frameworks.
Peewee: Peewee is a simpler and lightweight ORM compared to SQLAlchemy, which offers more advanced features and greater flexibility.
What are some real-world examples of Flask applications using SQLAlchemy?
Blog Platforms: Many blog platforms use Flask and SQLAlchemy for managing posts, comments, and user data.
E-commerce Sites: Flask applications for online stores often use SQLAlchemy for handling product inventories, orders, and customer information.
APIs: RESTful APIs built with Flask leverage SQLAlchemy for data storage and retrieval.
Where can I find additional resources and community support for Flask and SQLAlchemy?
Documentation: Check out the Flask documentation and SQLAlchemy documentation for comprehensive guides and API references.
Community Forums: Engage with the Flask and SQLAlchemy communities on forums like Stack Overflow and Reddit.
GitHub Repositories: Explore open-source projects and examples on GitHub to see how others use Flask and SQLAlchemy.
Subscribe to my newsletter
Read articles from Mohit Bhatt directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
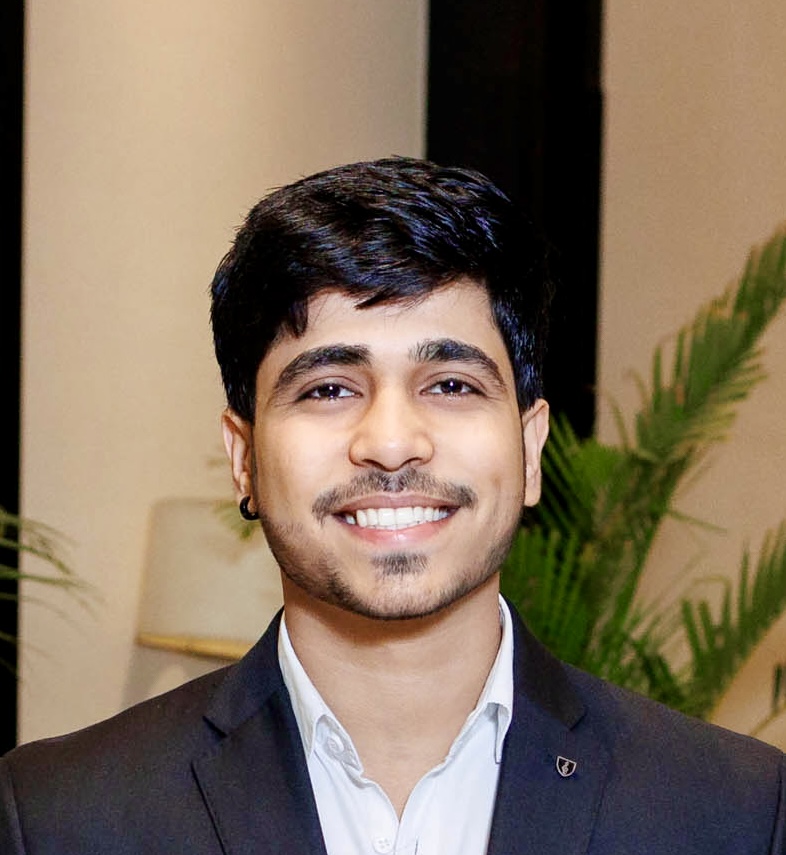
Mohit Bhatt
Mohit Bhatt
As a dedicated and skilled Python developer, I bring a robust background in software development and a passion for creating efficient, scalable, and maintainable code. With extensive experience in web development, Rest APIs., I have a proven track record of delivering high-quality solutions that meet client needs and drive business success. My expertise spans various frameworks and libraries, like Flask allowing me to tackle diverse challenges and contribute to innovative projects. Committed to continuous learning and staying current with industry trends, I thrive in collaborative environments where I can leverage my technical skills to build impactful software.