Pivot with Laravel
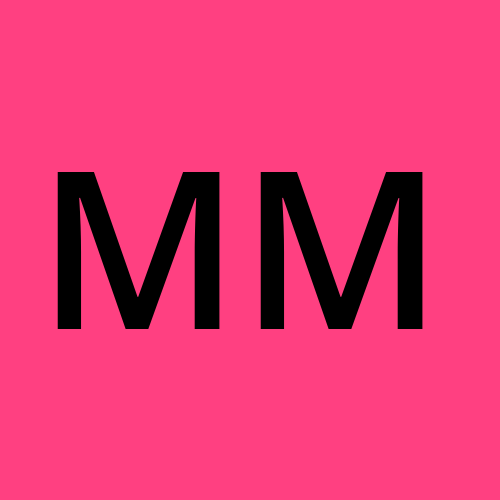
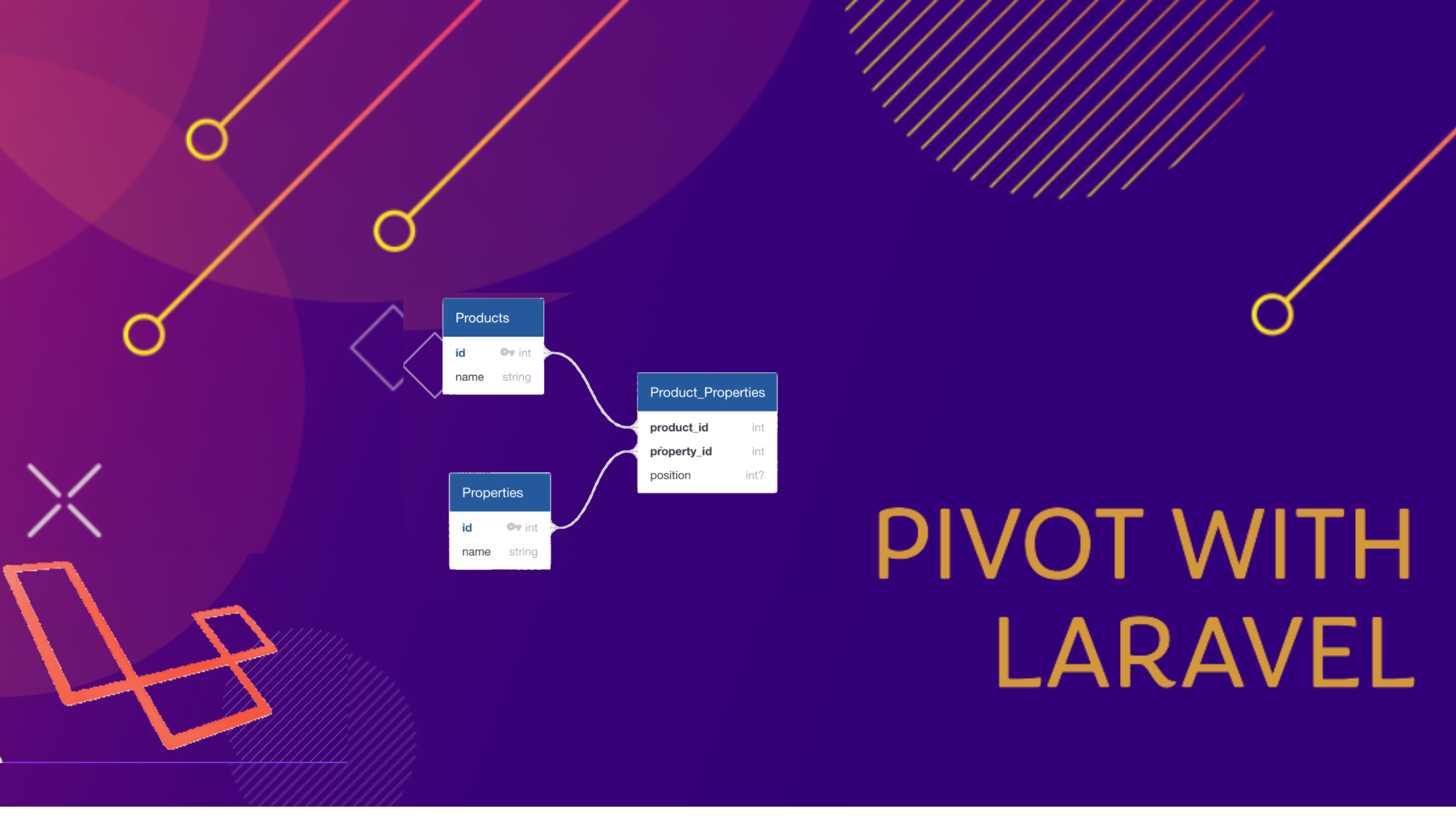
Laravel's pivot feature is a powerful tool for managing many-to-many relationships between models. It allows you to easily create and interact with the intermediate table that connects two models in a many-to-many relationship.
This blog will highlights the pros and cons of using pivot tables, their potential usage, and alternatives with real-world examples.
Pros
Simplifies many-to-many relationships: Pivot tables abstract away the complexity of managing the intermediate table, making it easier to work with many-to-many relationships.
Provides convenient methods: Laravel provides helpful methods like
attach()
,detach()
, andsync()
for managing pivot table data, reducing the amount of boilerplate code you need to write.Allows storing additional data: You can store additional data related to the relationship in the pivot table, such as timestamps, permissions, or any other relevant information.
Integrates well with Eloquent: Pivot tables are seamlessly integrated with Laravel's Eloquent ORM, allowing you to leverage its powerful features like eager loading, scopes, and events.
Potential Usage of Pivot Tables
- User roles and permissions: A common use case for pivot tables is managing user roles and permissions. You can create a many-to-many relationship between users and roles, and store the specific permissions for each role in the pivot table.
class User extends Model
{
public function roles()
{
return $this->belongsToMany(Role::class)->withPivot('permissions');
}
}
- Product categories: In an e-commerce application, you can use pivot tables to associate products with multiple categories. This allows a product to belong to multiple categories and a category to have multiple products.
class Product extends Model
{
public function categories()
{
return $this->belongsToMany(Category::class);
}
}
- Event attendees: For an event management system, you can use pivot tables to keep track of event attendees. Each event can have multiple attendees, and each attendee can attend multiple events.
class Event extends Model
{
public function attendees()
{
return $this->belongsToMany(User::class)->withTimestamps();
}
}
Cons
Limited functionality: Pivot tables are designed to be simple and lightweight, which means they have limited functionality compared to regular Eloquent models. You can't define relationships, scopes, or events on pivot tables.
Difficulty in querying: Querying data from pivot tables can be challenging, especially when you need to filter or sort based on pivot table columns.
Lack of flexibility: Pivot tables are tightly coupled with the models they connect, making it difficult to reuse the same pivot table for different relationships.
Problem I encountered and its solution
Issue
I had a table job which will have many to many relation ships with many cities. So there was there tables where job holds the job data, city holds the city data and job_city table acts as pivot to connect job and city. The features that we get with laravel pivot was working fine unless the request to the server was made with huge payload that includes cities data. The issue at the time was execution of queries to perform transaction to delete and add city data related to job on pivot table. I was using sync function to implicitly resolve the delete and addition of related cities for job into pivot table.
Using Sync
// Syncing city to a job
$job = Job::find(1);
//supposed we have cities already aatched to the job
// 1.2.3
// Sync city with IDs 1, 2, and 4
$job->cities()->sync([1, 2, 4]);
Here sync function first checks for all given ids related to a job on pivot table, then deletes the ones not in city array. But after detaching the unnecessary city ids , now it prepares query to insert city data for a job in pivot table individually. This was taking long time to complete due to transactions in huge amount and causing timeout issue.
Solution
So instead of using sync method, manual insertion and deletion seemed to be better optimised solution. Here is the implementaion
$cityIds =[1,2,4];
$cities = City::whereIn('id',cityIds)->get();
// it return a job cities that were previously selected [1,2,3]
$jobCities = $job->cities->pluck('pivot')->pluck('city_id')->toArray();
//this return the city ids that are in $jobCities but not in $cityIds
$newCityIds = array_diff($cityIds, $jobCities); // return 4
$newCities = $cities->whereIn('city_id', $newCityIds); // filters city detail based on diffrentiated city ids which for 4
//Prepare a array for bulk insert
$newCities = $newCities->map(function ($item) {
$item['job_id'] = $this->job->job_id;
return $item;
})->toArray();
// insert the new cities for job in bulk
JobCity::insert($newCities);
// detach the cities for a job which were previously selected but not this time
$removedCityIds = array_diff($jobCities, $cityIds);
//here I have used the detached methods as it does delete the city id for a job in bulk
$job->cities()->detach($removedCityIds);
Alternatives to Pivot Tables
- Custom Eloquent models: If you need more functionality than pivot tables provide, you can create custom Eloquent models to represent the intermediate table. This allows you to define relationships, scopes, and events on the intermediate model.
class RoleUser extends Model
{
public function role()
{
return $this->belongsTo(Role::class);
}
public function user()
{
return $this->belongsTo(User::class);
}
}
- Polymorphic relationships: Laravel's polymorphic relationships allow you to create a single intermediate table that can connect to multiple models. This can be useful when you have a many-to-many relationship that involves more than two models.
class Comment extends Model
{
public function commentable()
{
return $this->morphTo();
}
}
References
https://serversideup.net/managing-pivot-data-with-laravel-eloquent/
https://laracasts.com/discuss/channels/eloquent/how-and-why-to-use-pivot-model
Conclusion
In conclusion, Laravel's pivot feature is a powerful tool for managing many-to-many relationships, but it has its limitations. Understanding the pros and cons, potential usage, and alternatives can help you make suitable decisions when designing application's data model. Leveraging pivot tables or exploring alternatives, you can create more efficient and maintainable applications with sustainable database structure to maintain the many-to-many relationships.
I hope this blog was helpful ๐. Please feel free to ask me any other questions you may have. I am always happy to help ๐ค.
Happy Pivoting.
Subscribe to my newsletter
Read articles from manish maharjan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
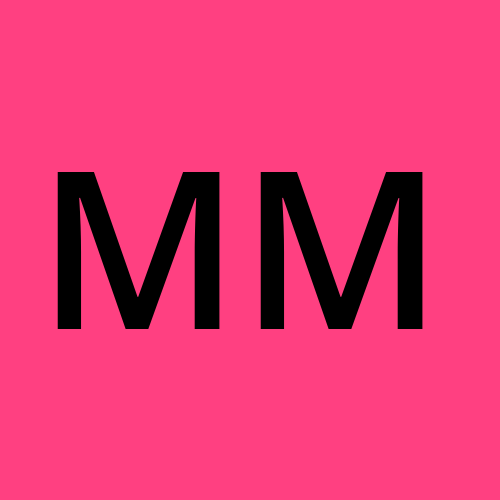