What are Docker Volumes?
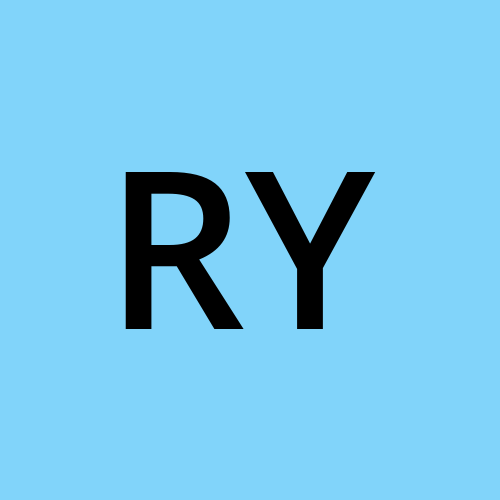
Docker volumes are a way to persist and share data between Docker containers and the host machine. They are essentially directories that exist outside of the container’s Union File System. Docker volumes offer several advantages over other methods of data management:
Persistence: Data stored in volumes persists beyond the lifecycle of a container. This means that even if a container is removed, the data stored in the volume remains intact.
Performance: Volumes are typically faster and more efficient than bind mounts for managing container data. This is because volumes are managed by Docker itself and can be optimized for performance.
Isolation: Volumes can be managed independently of containers, providing better isolation and management of data. This allows for easier backup, restore, and migration of data between containers.
How Docker Volumes Work
Docker volumes are managed by the Docker daemon and can be created, inspected, and manipulated using Docker CLI commands or Docker Compose configurations. When you create a volume and mount it to a container, Docker ensures that the data stored in that volume is persisted even if the container is removed or replaced.
Now that we have a basic understanding of Docker volumes, let's move on to a practical example to see how they can be used to manage data persistence effectively.
Practical Example: Managing Data with Docker Volumes
Suppose we have a simple Python application that connects to a MySQL database. We want to containerize this application and ensure that both the application data and the MySQL data are persisted reliably.
Step 1: Dockerfile Configuration
First, let's create a Dockerfile for our Python application. This Dockerfile will define how our application is built and packaged into a Docker image.
# Use an official Python runtime as the base image
FROM python:3.9-slim
# Set the working directory in the container
WORKDIR /app
# Install required packages for system
RUN apt-get update \
&& apt-get upgrade -y \
&& apt-get install -y gcc default-libmysqlclient-dev pkg-config \
&& rm -rf /var/lib/apt/lists/*
# Copy the requirements file into the container
COPY requirements.txt .
# Install app dependencies
RUN pip install mysqlclient
RUN pip install --no-cache-dir -r requirements.txt
# Copy the rest of the application code
COPY . .
# Specify the command to run your application
CMD ["python", "app.py"]
Step 2: Docker Compose Configuration
Next, let's create a Docker Compose configuration to define and manage our application's services and their dependencies.
version: '3'
services:
backend:
build:
context: .
ports:
- "5000:5000"
environment:
MYSQL_HOST: mysql
MYSQL_USER: admin
MYSQL_PASSWORD: admin
MYSQL_DB: myDb
depends_on:
- mysql
volumes:
- mysql-data:/app/data # Mount volume for storing application data
mysql:
image: mysql:5.7
ports:
- "3306:3306"
environment:
MYSQL_ROOT_PASSWORD: root
MYSQL_DATABASE: myDb
MYSQL_USER: admin
MYSQL_PASSWORD: admin
volumes:
- ./message.sql:/docker-entrypoint-initdb.d/message.sql # Mount SQL script for initialization
- mysql-data:/var/lib/mysql # Mount volume for MySQL data storage
volumes:
mysql-data:
In this configuration:
We define a volume named mysql-data which will persist MySQL data beyond container restarts.
The backend service mounts this volume at /app/data, ensuring that application data is persisted.
The mysql service also mounts the same volume at /var/lib/mysql to store MySQL data persistently.
Step 3: Explanation of Configuration
Let's break down the Docker Compose configuration:
We define two services: backend for our Python application and mysql for the MySQL database.
The backend service depends on the mysql service, ensuring that the database is available before the application starts.
Volumes are used to persist data for both services. For the backend service, we mount a volume named mysql-data at /app/data to store application data. For the mysql service, we mount the same volume at /var/lib/mysql to store MySQL data.
Step 4: Running the Application
Now that we have our Dockerfile and Docker Compose configuration in place, we can build and run our application using the following commands:
docker-compose build docker-compose up -d
This will build the Docker images and start the containers in detached mode, allowing them to run in the background.
Step 5: Testing Data Persistence
To test the data persistence, we can make some changes to our application data and MySQL database and then restart the containers using the following command:
docker-compose restart
After the containers have restarted, we can verify that our changes are still present in the application and database, confirming that the data persistence is working as expected.
Conclusion
In this article, we've explored Docker volumes and how they enable us to manage and persist data within containers effectively. By leveraging Docker volumes, we can ensure that our application data and databases remain intact even in dynamic containerized environments. Understanding these concepts is essential for building resilient and scalable containerized applications.
Follow me on LinkedIn for more DevOps insights and tutorials. Let's continue our journey towards mastering containerization and infrastructure as code!
#DevOps #Docker #Containerization #DataPersistence #InfrastructureAsCode
Subscribe to my newsletter
Read articles from Ranjan Yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
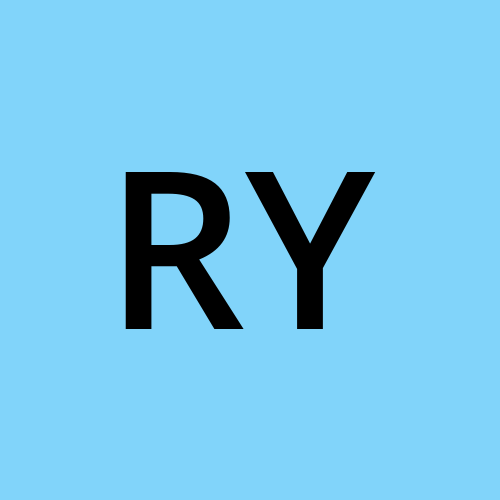