Build an End-to-end HTTP API with AWS API Gateway
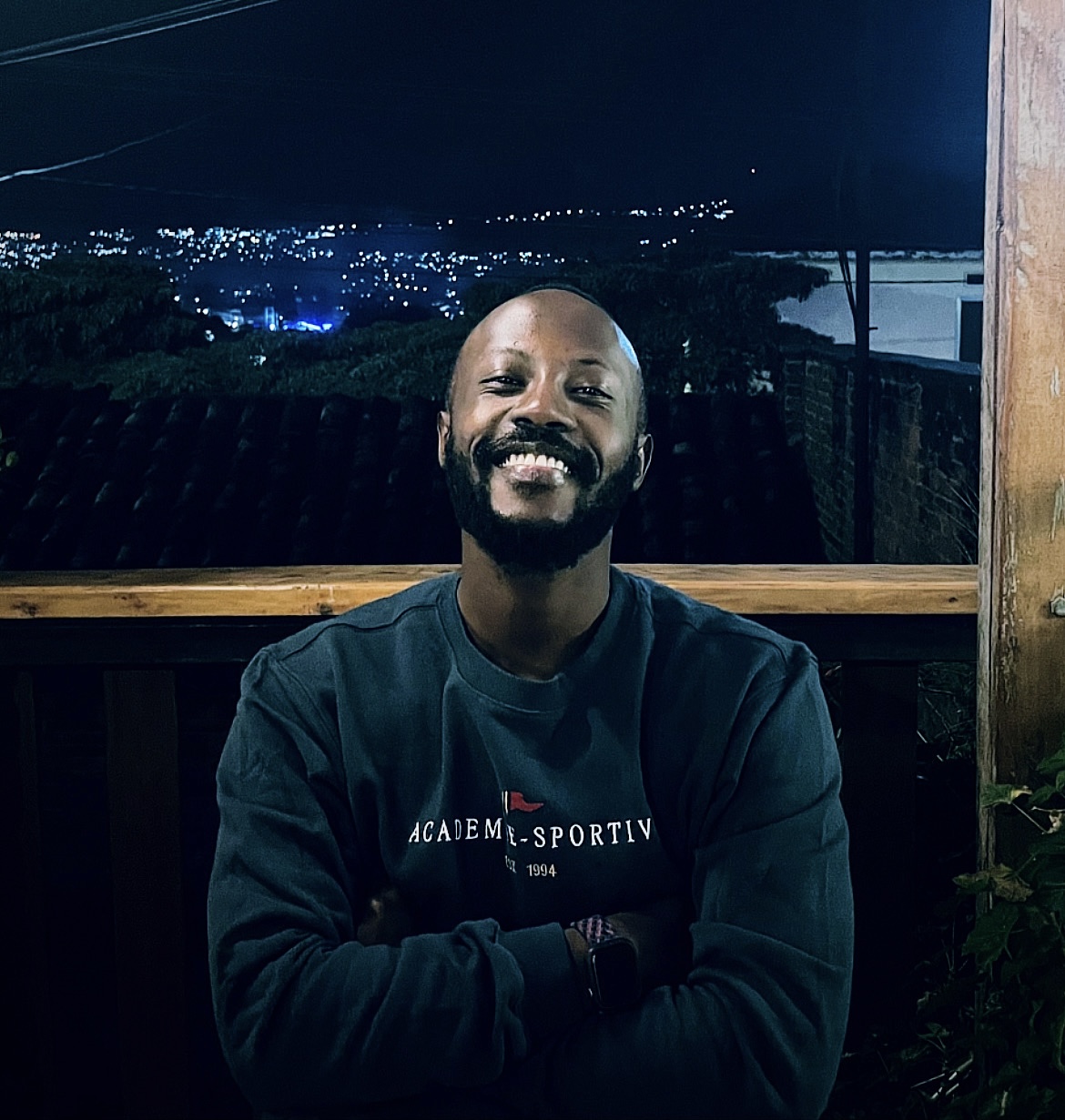
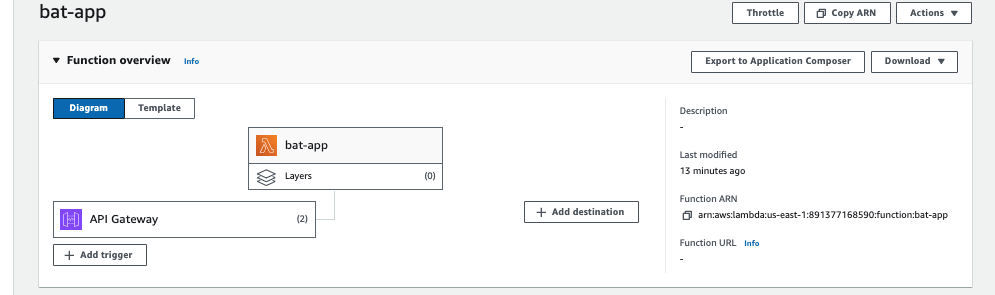
In this article, I will guide you through the process of setting up AWS API Gateway to create an end-to-end HTTP API. This API will allow users to request information from a database and create new data entries. Whether you're a beginner or looking to refresh your knowledge, this detailed guide aims to help you understand and implement AWS API Gateway effectively.
Key Concepts to Understand Before You Start
IoT Communication APIs:
REST Based Communication APIs:
Use HTTP methods such as GET, POST, PUT, DELETE.
A new TCP connection is established for each HTTP request.
WebSocket Based Communication APIs:
Bi-directional communication where messages can be sent and received by both the server and the client.
Utilizes IP address and port number for data retrieval.
Once the connection is set up, messages can be continuously exchanged without interruption.
Understanding HTTP & REST APIs
API (Application Programming Interface):
APIs allow different software applications to communicate with each other.
AWS introduced the first version of API Gateway in 2015, referred to as REST APIs, which include comprehensive features.
HTTP APIs vs. REST APIs:
REST APIs:
Feature-rich and versatile, suitable for complex use cases.
Can be heavier and more costly due to the extensive features.
HTTP APIs:
Introduced by Amazon in 2020 to provide a lightweight alternative to REST APIs.
Designed to be faster and more cost-effective.
Ideal for simple, straightforward API needs.
Setting Up AWS API Gateway
In the following sections, we will cover:
Creating an HTTP API: Step-by-step instructions to set up an HTTP API using AWS API Gateway.
Connecting to a Database: How to configure your API to interact with a database for retrieving and storing data.
Handling HTTP Requests: Implementing GET and POST methods to allow users to retrieve and create data.
Security and Permissions: Setting up appropriate security measures to protect your API and data.
Let’s create an HTTP API using AWS API Gateway right away.
1. Create a Lambda function
Here we’ll implement the requests in Lambda function. Since we haven’t built one. I’ll create a lambda function for further configuration.
Click Lambda service>Functions>Create function
Defining your function name, select the latest python version that is listed on Runtime, others leave as default. Then click Create function.
Click Lambda service>Functions>Create function
Defining your function name, select the latest python version that is listed on Runtime, others leave as default. Then click Create function.
2. Create an HTTP API
Click API Gateway service\> APIs>select HTTP API> Build
Select Lambda, Region and the lambda function name we just created. Define your own API name, then click Next
Adding Routes to the API
Define POST Route:
Navigate to the "Routes" section in API Gateway.
Add a route for
/createPerson
and select the POST method.Integrate this route with the
createPerson
Lambda function.
Define GET Route:
Add a route for
/getPerson
and select the GET method.Integrate this route with the
getPerson
Lambda function.
Step 4: Deploying the API
Create a Deployment Stage: Define a stage (e.g., "dev" or "prod") for your API deployment.
Deploy API: Deploy your API to the chosen stage.
Select GET method and edit the Recource path to /getPerson;
Add one more POST method and edit the Recource path to /createPerson, then click Next, leave the setting on the page default, click Next and then Create.
Now we have deployed to both prod and dev environments
Dev
Prod
Building a GET Method Response
Step-by-Step Guide
Step 1: Setting Up Postman
- Download Postman: If you haven't already, download and install Postman from here.
Step 2: Configure the GET Request in Postman
Open Postman: Launch the Postman application.
Create a New Request: Click on the
+
button to create a new tab.Set Request Type: Change the request type to
GET
from the dropdown menu.Enter the URL: Copy and paste the appropriate URL into the request URL field based on your deployment:
Staging:
https://xl274ucrd8.execute-api.us-east-1.amazonaws.com/dev/getPerson
Production:
https://xl274ucrd8.execute-api.us-east-1.amazonaws.com/prod/getPerson
Step 3: Add Query Parameters
Add Query Parameter: Click on the
Params
tab below the URL field.Enter Key-Value Pair: Add the key
personid
and the value123
to simulate a request. This will look like:Key:
personid
Value:
123
Step 4: Send the Request
Send Request: Click the
Send
button to send the GET request.View Response: The response from the API will be displayed in the lower section of Postman. Here you should see the data associated with the
personid
123 if it exists in your database.
Example Postman Setup
Request Type: GET
URL:
https://xl274ucrd8.execute-api.us-east-1.amazonaws.com/dev/getPerson
orhttps://xl274ucrd8.execute-api.us-east-1.amazonaws.com/prod/getPerson
Query Parameters:
Key:
personid
Value:
123
this is the response we get to ensure the API is working
We are going to update the Lambda function to get a better response
this is the source code
import json
def lambda_handler(event, context):
GET_RAW_PATH = "/getPerson"
if event['rawPath'] == GET_RAW_PATH:
# GetPerson Path
print("Start Request for GetPerson")
personId = event['queryStringParameters']['personid']
print("Received request with personId=" + personId)
if personId == '123':
person_details = {
"firstName": "John",
"lastName": "Goodman",
"email": "myEmail@gmail.com"
}
return {
'statusCode': 200,
'body': json.dumps(person_details),
'headers': {
'Content-Type': 'application/json'
}
}
else:
return {
'statusCode': 404,
'body': json.dumps({'message': 'Person not found'}),
'headers': {
'Content-Type': 'application/json'
}
}
# Test event example for local testing
if __name__ == "__main__":
test_event = {
'rawPath': '/getPerson',
'queryStringParameters': {
'personid': '123'
}
}
test_context = {}
print(lambda_handler(test_event, test_context))
Explanation
Import JSON: Import the
json
module to handle JSON serialization.Define the
lambda_handler
Function: This function is the entry point for the Lambda function.Check the Path: Verify if the request path matches
/getPerson
.Extract
personid
: Retrieve thepersonid
from the query parameters.Response Based on
personid
:If
personid
is123
, return the person's details with a 200 status code.If the
personid
is not123
, return a 404 status code with a "Person not found" message.
Set Headers: Include the
Content-Type
header in the response to specify JSON format.
Subscribe to my newsletter
Read articles from Bruno Gatete directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
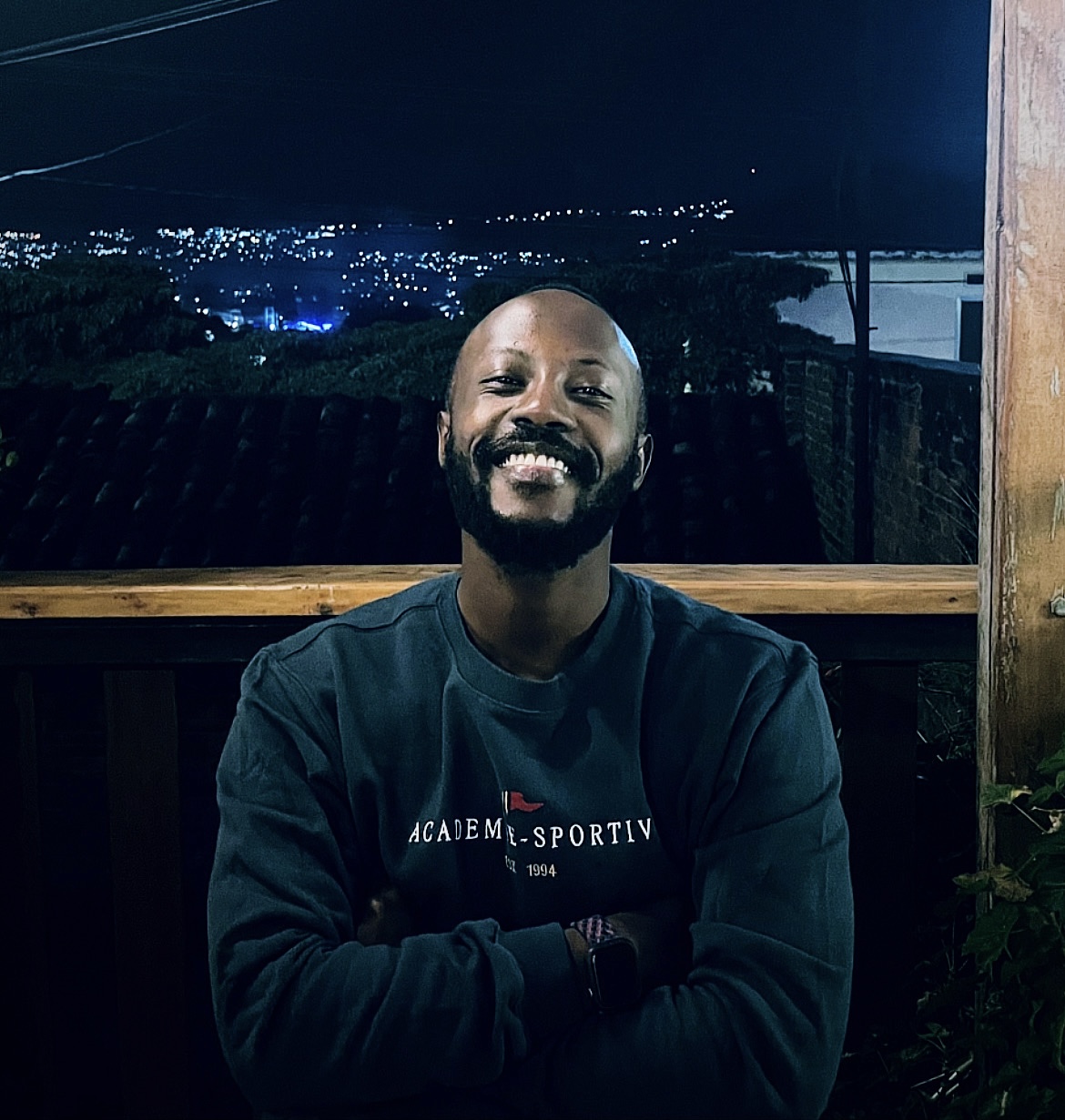
Bruno Gatete
Bruno Gatete
DevOps and Cloud Engineer Focused on optimizing the software development lifecycle through seamless integration of development and operations, specializing in designing, implementing, and managing scalable cloud infrastructure with a strong emphasis on automation and collaboration. Key Skills: Terraform: Skilled in Infrastructure as Code (IaC) for automating infrastructure deployment and management. Ansible: Proficient in automation tasks, configuration management, and application deployment. AWS: Extensive experience with AWS services like EC2, S3, RDS, and Lambda, designing scalable and cost-effective solutions. Kubernetes: Expert in container orchestration, deploying, scaling, and managing containerized applications. Docker: Proficient in containerization for consistent development, testing, and deployment. Google Cloud Platform: Familiar with GCP services for compute, storage, and machine learning.