Mastering State Management with React Context: A Comprehensive Guide
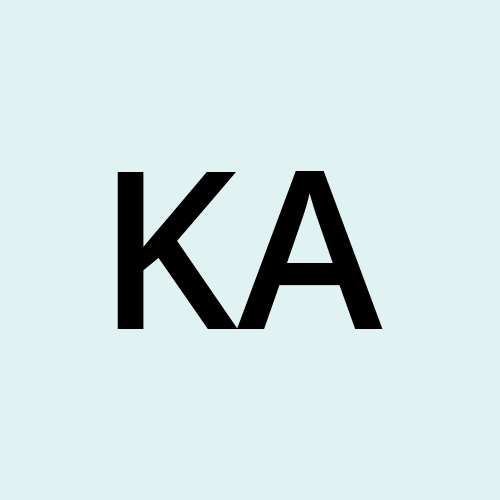
In the dynamic world of React development, managing application state is a critical aspect that often poses significant challenges. As your application grows, the complexity of managing state across multiple components can become overwhelming. One common approach to handling this complexity is through "prop drilling," where data is passed down from parent to child components through multiple layers. While effective in small applications, this method can quickly become cumbersome and error-prone in larger applications.
React Context is a feature designed to address these challenges by providing a way to share state across your entire application without needing to pass props through every level of the component tree. It enables you to create global state objects and make them available throughout your component hierarchy. This can simplify your codebase, reduce boilerplate, and improve maintainability.
In this guide, we’ll explore React Context from the ground up, covering its core concepts, practical applications, and advanced usage scenarios. By the end of this article, you’ll have a thorough understanding of how to leverage React Context to manage state effectively in your applications.
Understanding React Context
What is React Context?
React Context is a built-in feature that allows you to create a context object to hold and share state. This context can be accessed from any component within the tree, making it a powerful tool for managing global state. The core concepts of React Context include:
Context Object: Created using
React.createContext()
, this object will hold the values you want to share.Provider Component: A component that uses the
Context.Provider
to supply the context value to its child components.Consumer Components: Components that consume the context using the
useContext
hook to access the provided values.
Why Use React Context?
Avoid Prop Drilling: Simplifies component hierarchy by eliminating the need to pass props through multiple levels.
Centralized State Management: Provides a single source of truth for global state, making it easier to manage and update.
Enhanced Component Reusability: Allows components to be more modular and reusable by abstracting away the complexity of prop management.
Creating a Basic Context
Step 1: Setting Up the Context
To demonstrate how React Context works, let’s create a simple example: managing user authentication state. This example will guide you through setting up a context for authentication, creating a provider component, and consuming the context in various components.
Initialize a New React Project:
npx create-react-app react-context-demo cd react-context-demo
Create Context and Provider: Create a new file
AuthContext.js
in thesrc
directory. This file will define our context and provider component.
AuthContext.js
import React, { createContext, useState } from 'react';
// Create Context
const AuthContext = createContext();
// Create Provider Component
const AuthProvider = ({ children }) => {
const [authState, setAuthState] = useState({ isAuthenticated: false, user: null });
const login = (user) => {
setAuthState({ isAuthenticated: true, user });
};
const logout = () => {
setAuthState({ isAuthenticated: false, user: null });
};
return (
<AuthContext.Provider value={{ authState, login, logout }}>
{children}
</AuthContext.Provider>
);
};
export { AuthContext, AuthProvider };
Explanation
Creating the Context:
const AuthContext = createContext();
createContext()
creates a context object that holds the shared state. This context will be used to provide and consume the authentication state throughout the application.Provider Component:
const AuthProvider = ({ children }) => { const [authState, setAuthState] = useState({ isAuthenticated: false, user: null }); const login = (user) => { setAuthState({ isAuthenticated: true, user }); }; const logout = () => { setAuthState({ isAuthenticated: false, user: null }); }; return ( <AuthContext.Provider value={{ authState, login, logout }}> {children} </AuthContext.Provider> ); };
The
AuthProvider
component is responsible for managing the authentication state and providing methods to log in and log out. It usesAuthContext.Provider
to pass the state and methods to child components. Thevalue
prop ofAuthContext.Provider
contains the context values that will be accessible to consuming components.
Consuming Context in Components
Step 2: Using Context in Components
With the context and provider set up, you can now create components that consume the context. Let’s build a Login
component and a Logout
component to demonstrate how to interact with the context.
- Create a Login Component: Create a new file
Login.js
in thesrc
directory.
Login.js
import React, { useContext, useState } from 'react';
import { AuthContext } from './AuthContext';
const Login = () => {
const { authState, login } = useContext(AuthContext);
const [username, setUsername] = useState('');
const handleLogin = () => {
login({ name: username });
};
return (
<div>
{authState.isAuthenticated ? (
<p>Welcome, {authState.user.name}!</p>
) : (
<div>
<input
type="text"
value={username}
onChange={(e) => setUsername(e.target.value)}
placeholder="Enter username"
/>
<button onClick={handleLogin}>Login</button>
</div>
)}
</div>
);
};
export default Login;
Explanation
Using
useContext
:const { authState, login } = useContext(AuthContext);
The
useContext
hook allows you to access the context values provided byAuthContext
. This hook returns the context value, which includes the authentication state and methods.Handling Login:
const handleLogin = () => { login({ name: username }); };
The
handleLogin
function calls thelogin
method from the context, updating the authentication state to indicate that the user is logged in.Conditional Rendering:
{authState.isAuthenticated ? ( <p>Welcome, {authState.user.name}!</p> ) : ( <div> <input type="text" value={username} onChange={(e) => setUsername(e.target.value)} placeholder="Enter username" /> <button onClick={handleLogin}>Login</button> </div> )}
The component renders a welcome message if the user is authenticated. Otherwise, it displays a login form that allows the user to enter a username and login.
Step 3: Adding a Logout Component
- Create a Logout Component: Create a new file
Logout.js
in thesrc
directory.
Logout.js
import React, { useContext } from 'react';
import { AuthContext } from './AuthContext';
const Logout = () => {
const { authState, logout } = useContext(AuthContext);
return (
<div>
{authState.isAuthenticated ? (
<button onClick={logout}>Logout</button>
) : (
<p>You are not logged in.</p>
)}
</div>
);
};
export default Logout;
Explanation
Using
useContext
:const { authState, logout } = useContext(AuthContext);
This hook accesses the context values provided by
AuthContext
, including thelogout
method and the current authentication state.Handling Logout:
{authState.isAuthenticated ? ( <button onClick={logout}>Logout</button> ) : ( <p>You are not logged in.</p> )}
The component renders a logout button if the user is authenticated. If not, it displays a message indicating that the user is not logged in.
Integrating Context into the Application
Step 4: Using the Provider in the Application
To ensure that the context is available throughout your application, wrap your components with the AuthProvider
in the main App
component.
App.js
import React from 'react';
import { AuthProvider } from './AuthContext';
import Login from './Login';
import Logout from './Logout';
function App() {
return (
<AuthProvider>
<div className="App">
<h1>React Context Authentication</h1>
<Login />
<Logout />
</div>
</AuthProvider>
);
}
export default App;
Explanation
Wrapping Components with Provider:
<AuthProvider>
<div className="App">
<h1>React Context Authentication</h1>
<Login />
<Logout />
</div>
</AuthProvider>
By wrapping Login
and Logout
components with AuthProvider
, you ensure that they have access to the context values. This setup makes the authentication state and methods available.
Advanced Usage of React Context
Context for Theming
React Context can be utilized not just for state management but also for theming, which allows you to apply global styles and manage themes across your application seamlessly. This approach simplifies theme toggling and ensures that theme-related data is easily accessible from any component.
Setting Up a Theme Context
- Create a Theme Context: Let’s define a new context for managing theme preferences. Create a new file
ThemeContext.js
in thesrc
directory.
ThemeContext.js
import React, { createContext, useState } from 'react';
// Create Context
const ThemeContext = createContext();
// Create Provider Component
const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme((prevTheme) => (prevTheme === 'light' ? 'dark' : 'light'));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
};
export { ThemeContext, ThemeProvider };
Explanation
Creating the Context:
const ThemeContext = createContext();
createContext()
creates a context object for managing the theme. This context will hold the current theme and a method to toggle between themes.Provider Component:
const ThemeProvider = ({ children }) => { const [theme, setTheme] = useState('light'); const toggleTheme = () => { setTheme((prevTheme) => (prevTheme === 'light' ? 'dark' : 'light')); }; return ( <ThemeContext.Provider value={{ theme, toggleTheme }}> {children} </ThemeContext.Provider> ); };
The
ThemeProvider
component maintains the theme state and provides a function to toggle between light and dark themes. Thevalue
prop ofThemeContext.Provider
makes the theme and thetoggleTheme
method available to all child components.
Consuming Theme Context in Components
- Create a Theme Toggle Component: Create a new file
ThemeToggle.js
in thesrc
directory.
ThemeToggle.js
import React, { useContext } from 'react';
import { ThemeContext } from './ThemeContext';
const ThemeToggle = () => {
const { theme, toggleTheme } = useContext(ThemeContext);
return (
<button onClick={toggleTheme}>
Switch to {theme === 'light' ? 'Dark' : 'Light'} Mode
</button>
);
};
export default ThemeToggle;
Explanation
Using
useContext
:const { theme, toggleTheme } = useContext(ThemeContext);
The
useContext
hook allows you to access the current theme and thetoggleTheme
method fromThemeContext
.Handling Theme Toggle:
<button onClick={toggleTheme}> Switch to {theme === 'light' ? 'Dark' : 'Light'} Mode </button>
The button allows users to toggle between light and dark themes. The text on the button dynamically changes based on the current theme.
Applying Theme Styles
- Create a Theme Wrapper Component: To apply theme-specific styles, you might create a wrapper component that adjusts styles based on the current theme.
ThemeWrapper.js
import React, { useContext } from 'react';
import { ThemeContext } from './ThemeContext';
const ThemeWrapper = ({ children }) => {
const { theme } = useContext(ThemeContext);
const themeClass = theme === 'light' ? 'light-theme' : 'dark-theme';
return (
<div className={themeClass}>
{children}
</div>
);
};
export default ThemeWrapper;
Explanation
Using
useContext
:const { theme } = useContext(ThemeContext);
The
ThemeWrapper
component usesuseContext
to get the current theme and applies the appropriate CSS class to a wrappingdiv
.Applying Theme Styles:
const themeClass = theme === 'light' ? 'light-theme' : 'dark-theme';
The component dynamically assigns a class based on the current theme. You can define the styles for
light-theme
anddark-theme
in your CSS files.
Integrating Theme Context into the Application
- Using the Theme Provider in the Application: To make the theme context available throughout your application, wrap your components with
ThemeProvider
in the mainApp
component.
App.js
import React from 'react';
import { ThemeProvider } from './ThemeContext';
import ThemeToggle from './ThemeToggle';
import ThemeWrapper from './ThemeWrapper';
function App() {
return (
<ThemeProvider>
<ThemeWrapper>
<div className="App">
<h1>React Context Theming</h1>
<ThemeToggle />
</div>
</ThemeWrapper>
</ThemeProvider>
);
}
export default App;
Explanation
Wrapping Components with Provider:
<ThemeProvider> <ThemeWrapper> <div className="App"> <h1>React Context Theming</h1> <ThemeToggle /> </div> </ThemeWrapper> </ThemeProvider>
By wrapping your components with
ThemeProvider
andThemeWrapper
, you ensure that the entire application can access and apply theme settings. TheThemeToggle
component allows users to switch between themes, andThemeWrapper
applies the appropriate styles based on the current theme.
Conclusion
React Context is a powerful tool for managing global state and sharing data across your application without the need for prop drilling. In this guide, we explored the basics of creating and using React Context, demonstrated how to build a simple authentication system, and delved into advanced use cases such as theming.
By incorporating React Context into your applications, you can simplify state management, improve code maintainability, and create a more intuitive development experience. As your applications grow and evolve, mastering React Context will be a valuable asset in your toolkit.
If you have any further questions or need additional examples, feel free to reach out or explore the official React documentation for more advanced use cases and best practices.
Subscribe to my newsletter
Read articles from Kate Ahmed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
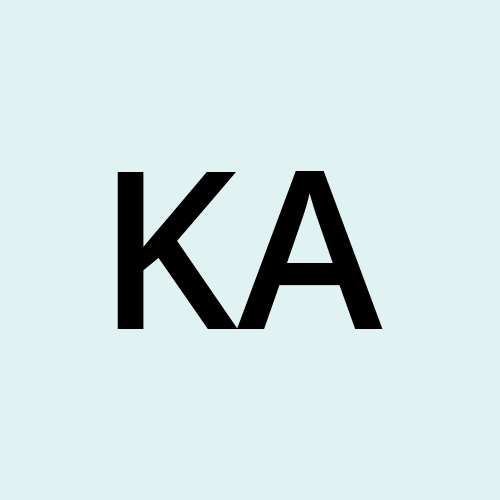