Stepping Stone to Containers

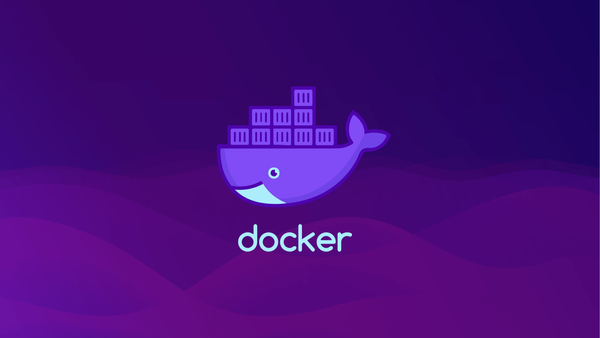
Containers 🫙
Containers are lightweight, standalone executable units that package an application and its dependencies, ensuring it runs consistently across different computing environments. They encapsulate everything needed to run the application, including the code, runtime, libraries, and system tools. Unlike virtual machines, containers share the host system's kernel, making them more efficient regarding resource usage and startup times.
Containers vs Virtual Machines(VM) 🖥️
Advantages of Containers
Lightweight: Containers share the host system's kernel and do not require a full operating system, making them much smaller and faster to start compared to VMs.
Efficiency: Containers consume fewer resources because they share the OS kernel. This allows you to run many more containers on a single host compared to VMs.
Portability: Containers encapsulate the application and its dependencies, ensuring consistent behavior across different environments (development, testing, production).
Rapid Scaling: Due to their lightweight nature, containers can be easily scaled up or down to handle varying loads.
Continuous Integration/Continuous Deployment (CI/CD): Containers support CI/CD practices by allowing developers to build, test, and deploy applications consistently across different environments.
Demerits of Containers
Isolation: Containers provide less isolation than VMs because they share the same OS kernel. This can lead to potential security vulnerabilities.
Compatibility: Containers are dependent on the host OS; they work best with Linux hosts, although solutions like Docker for Windows and macOS exist.
Persistence: Managing persistent storage in containers can be complex compared to VMs.
Virtual Machines (VMs)
Advantages of Virtual Machines
Isolation: VMs provide strong isolation because each VM runs a complete operating system, including its own kernel. This makes them more secure.
Compatibility: VMs can run different operating systems on the same physical hardware, providing flexibility to use any OS that best suits the application's needs.
Mature Tooling: VMs have been around longer and come with a wide array of tools for management, monitoring, and orchestration.
Demerits of Virtual Machines
Resource Intensive: VMs require more system resources (CPU, memory, storage) because each VM runs a full OS. This leads to higher overhead compared to containers.
Slower Boot Times: VMs take longer to start because they need to boot up a full operating system.
Scaling: Scaling VMs is slower and more resource-intensive compared to containers.
Consider building a MicroService Architecture
Using Containers: Each microservice can be containerized, allowing it to run independently on the same host with minimal overhead. Containers enable rapid deployment and scaling, ensuring efficient resource usage.
Using Virtual Machines: Each microservice would require a separate VM, each with its own OS. This setup provides strong isolation but at the cost of higher resource usage and slower performance. Scaling up means provisioning more VMs, which can be slow and resource-intensive.
Choose According to Your Needs
Containers: Are ideal for applications that need to be deployed quickly, scaled rapidly, and run consistently across different environments with minimal overhead. you can spinup your containers over your Virtual Machines too. They are particularly useful in microservices architectures and CI/CD pipelines.
Virtual Machines: Are more suitable for scenarios requiring strong isolation, different operating systems on the same host, or when running applications that require a complete OS environment. They provide robust security and compatibility but at the cost of higher resource usage and slower performance.
Choosing between containers and VMs depends on the specific needs of your application and environment, balancing the trade-offs between efficiency, isolation, and resource consumption.
Docker
The Docker Containerization Platform is transforming the way applications are built, shipped, and run. By encapsulating apps and their dependencies into lightweight, portable containers, Docker ensures they work seamlessly across any environment. Experience unparalleled efficiency, scalability, and consistency with Docker's cutting-edge container technology, empowering developers to innovate faster than ever before.
Docker's architecture consists of several key components that work together to enable the creation, deployment, and management of containerized applications. Here's an overview of the primary components:
1. Docker Client
Description: The Docker client is a command-line tool that allows users to interact with the Docker daemon. It sends commands to the Docker daemon through a REST API.
Common Commands:
docker build
,docker run
,docker pull
,docker push
.
2. Docker Daemon (dockerd)
Description: The Docker daemon (
dockerd
) runs on the host machine and manages Docker containers, images, networks, and storage volumes. It listens for API requests from the Docker client and manages Docker objects.Role: Responsible for the heavy lifting of building, running, and managing Docker containers.
3. Docker Images
Description: Docker images are read-only templates that contain the instructions for creating a container. An image is built from a Dockerfile and can include the application code, runtime, libraries, and dependencies needed to run the application.
Usage: Images are used to create containers with
docker run
.
4. Docker Containers
Description: Containers are lightweight, portable units that run applications. They are created from Docker images and share the host system's kernel but run in isolated user spaces.
Characteristics: Containers can be started, stopped, moved, and deleted using Docker commands.
5. Docker Registry (Docker Hub)
Description: A Docker registry is a storage and distribution system for Docker images. Docker Hub is the default public registry, but private registries can also be set up.
Functions: Users can push and pull images to and from registries.
6. Docker Compose
Description: Docker Compose is a tool for defining and running multi-container Docker applications. It uses a YAML file to configure the application’s services, networks, and volumes.
File: The configuration is typically defined in a
docker-compose.yml
file.
7. Docker Swarm
Description: Docker Swarm is Docker's native clustering and orchestration tool. It turns a pool of Docker hosts into a single, virtual Docker host, allowing for the management of multiple containers across multiple hosts.
Features: It provides features like load balancing, scaling, and desired state management.
8. Docker Network
Description: Docker provides a networking system that allows containers to communicate with each other and with the outside world. It includes several drivers, such as bridge, host, and overlay
Purpose: Networks provide isolation and security for containers.
9. Docker Volume
Description: Volumes are the preferred mechanism for persisting data generated by and used by Docker containers. They can be shared and reused among containers.
Types: Named volumes and anonymous volumes.
Now Let's Get our hands Dirty👷♂️
Here the Objective is to Containerize a simple Java-based SpringBoot WebApp
I have already built a simple web app here.
You could easily clone it and follow the below-mentioned Steps.
The Dockerfile is the cornerstone of Docker's containerization magic. It serves as a blueprint, detailing every step needed to assemble a Docker image, from the base OS to the application itself. This ensures reproducibility, consistency, and streamlined deployment, making Dockerfile an essential tool for seamless DevOps workflows.
I have written DockerFile for the respective web-app
FROM koushalakash/myjava-maven:latest
ARG artifact=target/Koushal-0.0.1.jar
WORKDIR /app
COPY src /app
COPY pom.xml /app
RUN mkdir src && \
mv main src/ && \
mv test src/ && \
mvn clean install && \
cp $artifact app.jar
ENTRYPOINT ["java"]
CMD ["-jar", "app.jar"]
The Base Image mentioned in the above DockerFile " koushalakash/myjava-maven:latest" is a Linux based platform with Java 22 and Maven. You could also follow the same Base Image or Try a Different one with Java and Maven
For this Hands-on Purpose, I am using a New AWS EC2 instance
you could see the instance doesn't have any Java or Maven installed itself.
I have cloned the repository in this Instance
The next Step is to install the Docker
sudo apt update && sudo apt upgrade -y
sudo apt install docker.io -y
Don't rush and execute the docker installation it mostly throws error.It is always good practice to update the packages and their respective index before installing any software using the package manager.
Before Executing any docker commands
check if docker is up and running
systemctl status docker
if in case Docker is not active
systemctl enable docker
systemctl start docker
Next Try running Docker Commands such as docker ps
if you got an error such as
it means the user ubuntu has no rights to execute the docker command so to resolve this error you need to add the respective user to the docker group
sudo usermod -aG docker ubuntu
Logout from the system once and login again.This steps will clear the above mentioned error
Now let's create a docker image of our application
docker build -t koushalakash/demoapplicationcontainer:v1
you will get output similar like this
Before running the image we are running the spring-boot application it runs default on port 8080 so you need to edit the inbound rules to allow the traffic via that port
Next will run the respective image
docker run -p 8080:8080 -it koushalakash/demoapplicationcontainer:v1
Here -p 8080:8080 is used because we are mapping the Docker port 8080 to Host Port 8080
Now let's check the website is up or not
That's Right the Website is UP 🌟⚡. we containerized a web application and ran it Successfully😎.
If you want store your Docker image Remotly and use it in future .you could use Docker Registry (Docker hub) which is Explained above in Docker Components Section. you need to sign up to the Docker Hub before.It was just a regular login process
docker login
Enter your username and password and Get your login Succeeded
docker push koushalakash/demoapplicationcontainer:v1
Now Let's Verify in the Docker Hub Repository
Now you can push and pull your image anytime you want and deploy your application irrespective of the host's Platform🌟.
That's it for today ✌️.Happy Exploring.⚡
Subscribe to my newsletter
Read articles from Koushal Akash RM directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
