How to Mint an NFT on Polygon using Ethers.js
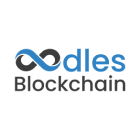
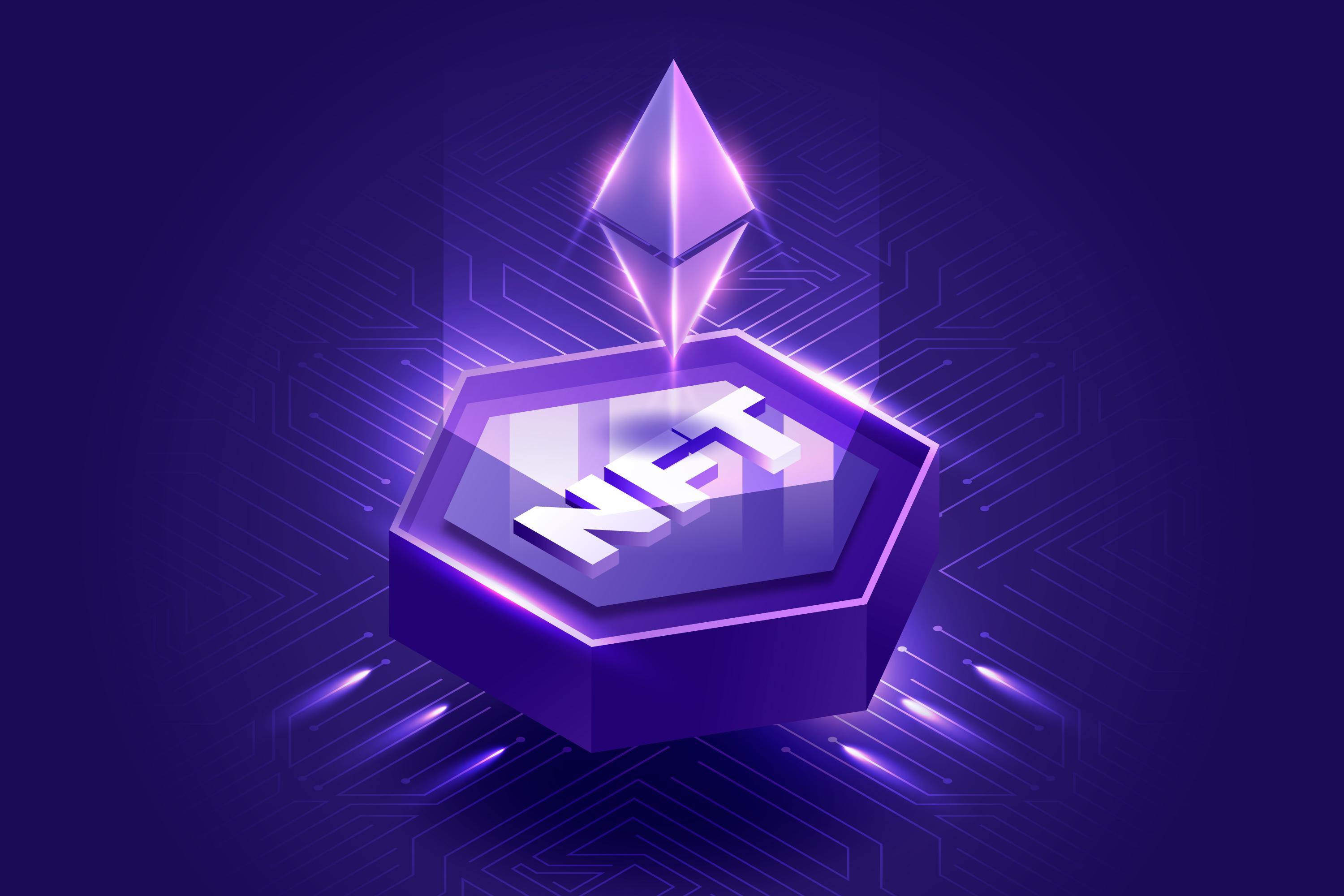
Minting a Non-Fungible Token (NFT) on the Polygon network using Ethers.js is a simple and straightforward process. In this article, explore step-by-step how to do so. Ethers.js is a JavaScript library that helps us interact with smart contracts. It also allows us to call contract functions, enabling us to mint NFTs using JavaScript code easily. For more related to NFT, visit our NFT development services.
Setup
To interact with the Polygon network, we need to install the following dependencies in our code:
Ethers.js: It is the JavaScript library that provides methods and functions to interact with the blockchain.
dotenv: For managing environment variables securely, such as the private key, which is of utmost importance to keep secure.
Additionally, we need the following:
Private Key: A private key is necessary to sign transactions and authenticate the actions performed by your wallet. It must be kept secure to prevent unauthorized access to your account and assets.
Contract Address: The contract address specifies the location of the deployed smart contract on the Polygon network. It is required to interact with the specific contract where the NFT minting functions are defined.
ABI (Application Binary Interface): The ABI defines the functions and events available in the smart contract. It is essential to correctly call the contract functions and interpret the responses.
Here’s how you can set up your project and interact with the smart contract to mint an NFT on the Polygon network using Ethers.js:
Installation
Install the necessary dependencies:
-npm install ethers dotenv
Interacting with the Smart Contract
The provided code demonstrates how to interact with an NFT smart contract on the Polygon chain using ethers.js. It starts by loading environment variables for the private key and contract address. Ensure that the private key belongs to the owner who deployed the contract, as only they are allowed to mint the NFT. A connection to the Polygon network is established via a JSON-RPC provider, and a wallet is created with the owner’s private key. The script then initializes an instance of the NFT contract using the contract address and ABI. It attempts to mint an NFT to a specified address by calling the safeMint function, logging the transaction hash and success status.
require('dotenv').config();
const { nftAbi } = require('./abi');
const { ethers } = require('ethers');
const provider = new ethers.JsonRpcProvider('https://polygon-amoy.drpc.org');
console.log('Connected to Polygon network.');const wallet = new ethers.Wallet(process.env.PRIVATE_KEY, provider);
console.log('Wallet address:', wallet.address);const contractAddress = process.env.CONTRACT_ADDRESS;
console.log('Contract address:', contractAddress);const nftContract = new ethers.Contract(contractAddress, nftAbi, wallet);async function main() {
const recipientAddress = '0xE4cF33fA257Ec61918DC7F07a8e6Ece1952C01D0'; try {
console.log(`Minting NFT to address: ${recipientAddress}`);
const tx = await nftContract.safeMint(recipientAddress);
console.log('Transaction hash:', tx.hash);
console.log('NFT minted successfully!');
} catch (error) {
console.error('Error minting NFT:', error);
}
}main().catch((error) => {
console.error('Script error:', error);
process.exit(1);
});
Conclusion
Minting NFTs on the Polygon network using Ethers.js is a straightforward process that leverages JavaScript’s capabilities to interact with smart contracts. By setting up your environment with the necessary dependencies and securely managing your private key, you can easily work with NFT contracts. This guide walks you through the essential steps, from installing the required libraries to executing code that interacts with your smart contract. With the provided example, you can see how to connect to the Polygon network, create a wallet, and call the safeMint function to mint NFTs to any specified address. If you have a similar project in mind that you want to bring into reality, connect with our skilled NFT developers to get started.
Subscribe to my newsletter
Read articles from Mohd Arslan Siddiqui directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
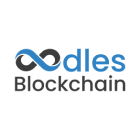
Mohd Arslan Siddiqui
Mohd Arslan Siddiqui
Expert blockchain writer with a knack for simplicity. Blends technical depth with engaging storytelling.