Exploring the New Features in Angular 18

Table of contents
- 1. TypeScript 5.4 Support
- 2. Defer Views
- 3. Router Enhancements
- 4. Zone-less Change Detection
- 5. Improved Forms API
- 6. Fallback Content for ng-content
- 7. Template Local Variable with @let Block
- 8. Core Improvements
- 9. Enhanced Angular DevTools
- 10. Support for Web Components
- 11. Enhanced Internationalization
- 12. New Router API
- 13. HttpClientModule Deprecation
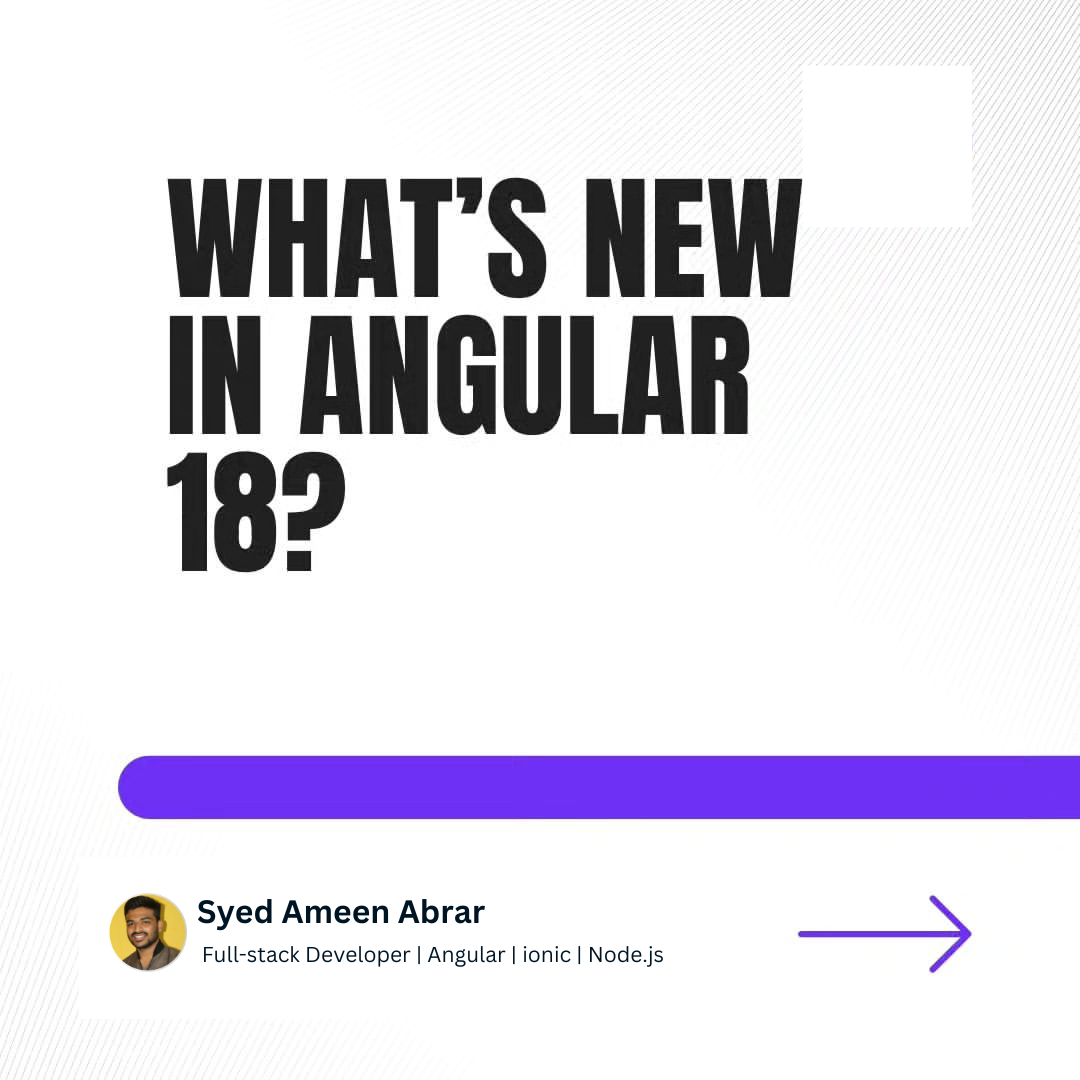
Angular 18 has introduced a range of exciting new features and enhancements designed to improve the framework's performance, flexibility, and developer experience. In this blog post, we'll delve into these new features with detailed descriptions and examples to help you understand how to leverage them in your Angular applications.
1. TypeScript 5.4 Support
Description: Angular 18 supports TypeScript 5.4, providing improved language features, better type-checking, and enhanced tooling support. This upgrade ensures compatibility with the latest TypeScript features, helping developers write more robust and maintainable code.
**Example:
**
type User = {
id: number;
name: string;
email: string;
};
const user: User = { id: 1, name: "John Doe", email: "john.doe@example.com"};
2. Defer Views
Description: The defer views feature, previously in developer preview, is now stable. It allows lazy loading of dependencies, which can significantly enhance application performance by loading only the necessary parts of the application when needed.
**Example:
**
import { Component, ViewChild } from '@angular/core';
@Component({ selector: 'app-defer-example', template: <ng-container *ngIf="loadContent"> <app-heavy-component></app-heavy-component> </ng-container> <button (click)="loadContent = true">Load Content</button>
})export class DeferExampleComponent { loadContent = false;}
3. Router Enhancements
Route Redirects with Functions:Description: Angular 18 introduces more flexible route management by allowing functions for redirects. This feature opens up new possibilities for dynamic and conditional routing.
**Example:
**
import { inject } from '@angular/core';
import { Router, UrlTree } from '@angular/router';
import { UserService } from './user.service';
const routes = [ { path: 'legacy-users', redirectTo: (redirectData) => { const userService = inject(UserService); const router = inject(Router); const queryParams = redirectData.queryParams; if (userService.isLoggedIn()) { const urlTree = router.parseUrl('/users'); urlTree.queryParams = queryParams; return urlTree; } return '/users'; } }];
New Redirect Command:Description: Enhances redirection capabilities within guards and resolvers by allowing redirections to be more complex and tailored to specific navigation behaviors.
**Example:
**
import { Router, RedirectCommand } from '@angular/router';
const routes = [ { path: 'users', component: UsersComponent, canActivate: [ () => { const userService = inject(UserService); return userService.isLoggedIn() || new RedirectCommand(router.parseUrl('/login'), { state: { requestedUrl: 'users' } }); } ], }];
4. Zone-less Change Detection
Description: Angular 18 introduces zone-less change detection, allowing change detection to operate independently without relying on zone.js. This enhancement can improve performance and reduce the complexity of managing change detection in large applications.
**Example:
**
import { Component } from '@angular/core';
import { ApplicationRef } from '@angular/core';
@Component({ selector: 'app-zone-less', template: <button (click)="update()">Update</button>
})export class ZoneLessComponent { constructor(private appRef: ApplicationRef) {}
update() { this.appRef.tick(); }}
5. Improved Forms API
Description: Angular 18 enhances the forms API with new properties and events in the AbstractControl
class. These enhancements provide better handling of form control states and events, making form creation and validation more streamlined.
**Example:
**
import { Component } from '@angular/core';
import { NonNullableFormBuilder, TouchedChangeEvent, PristineChangeEvent, StatusChangeEvent, ValueChangeEvent, FormResetEvent, FormSubmitEvent } from '@angular/forms';
@Component({ selector: 'app-forms-example', template: <form [formGroup]="userForm"></form>
})export class FormsExampleComponent { userForm = this.fb.group({ login: ['', Validators.required], password: ['', Validators.required] });
constructor(private fb: NonNullableFormBuilder) { this.userForm.events.subscribe(event => { if (event instanceof TouchedChangeEvent) { console.log('Touched:', event.touched); } else if (event instanceof PristineChangeEvent) { console.log('Pristine:', event.pristine); } else if (event instanceof StatusChangeEvent) { console.log('Status:', event.status); } else if (event instanceof ValueChangeEvent) { console.log('Value:', event.value); } else if (event instanceof FormResetEvent) { console.log('Form reset'); } else if (event instanceof FormSubmitEvent) { console.log('Form submit'); } }); }}
6. Fallback Content for ng-content
Description: Angular 18 allows defining fallback content inside ng-content
tags if no content is projected. This makes component templates more flexible and robust.
Example:
<div class="card">
<div class="card-body">
<h4 class="card-title">
<ng-content select=".title">Default title</ng-content>
</h4>
<p class="card-text">
<ng-content select=".content"></ng-content>
</p>
</div>
</div>
7. Template Local Variable with @let
Block
Description: Angular 18 introduces the @let
block, which enhances the flexibility of template syntax by allowing the declaration of local variables within templates.
**Example:
**
<div *ngFor="let item of items; let index = index">
<p>Item {{ index }}: {{ item }}</p>
</div>
8. Core Improvements
Description: Angular 18 includes ongoing refinements in change detection, rendering, and dependency injection to improve performance and maintainability.
**Example:
**
import { Component, Inject } from '@angular/core';
@Component({ selector: 'app-core-improvements', template: <h1>Core Improvements</h1>
})export class CoreImprovementsComponent { constructor(@Inject('SomeService') private someService) {}}
9. Enhanced Angular DevTools
Description: Various improvements have been made to Angular DevTools, simplifying the debugging process and providing more insights into Angular applications.
**Example:
**
// No code example needed for DevTools enhancement as it is an external tool.
10. Support for Web Components
Description: Angular 18 enhances support for using web components within Angular applications, making it easier to integrate and use web components seamlessly.
**Example:
**
import { Component, CUSTOM_ELEMENTS_SCHEMA } from '@angular/core';
@Component({ selector: 'app-web-component-example', template: <my-web-component></my-web-component>
, schemas: [CUSTOM_ELEMENTS_SCHEMA]})export class WebComponentExampleComponent {}
11. Enhanced Internationalization
Description: Improved capabilities for building multilingual applications, making it easier to handle multiple languages and locales.
**Example:
**
import { registerLocaleData } from '@angular/common';
import localeFr from '@angular/common/locales/fr';
registerLocaleData(localeFr, 'fr');
12. New Router API
Description: Angular 18 introduces a new router API, providing more flexible and powerful routing configurations.
**Example:
**
import { provideRouter, RouterModule } from '@angular/router';
@NgModule({ imports: [ RouterModule.forRoot(routes) ], providers: [ provideRouter([ // New router API configurations ]) ]})export class AppRoutingModule {}
13. HttpClientModule Deprecation
Description: Moving towards standalone components, the HttpClientModule
and related modules are deprecated in Angular 18, encouraging developers to adopt standalone components for better modularization.
Angular 18 brings a wealth of new features and enhancements that make it a powerful and flexible framework for modern web development. By leveraging these new capabilities, developers can build more efficient, maintainable, and scalable applications.
Fallow me for more such content,
Email me at : syedameenabrar092@gmail.com,
Website : https://syedameenabrar.blogspot.com/p/home.html
Blogs : https://syedameenabrar.hashnode.dev/
Instagram : https://www.instagram.com/syed_ameen_abrar/
Linkedin: https://www.linkedin.com/in/syed-ameen-abrar-61a67217a/
Facebook : https://www.facebook.com/syedameenabrar92/
Subscribe to my newsletter
Read articles from Syed Ameen Abrar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
